Fetching Data on an Event
We will learn how to fetch data on an event
This lesson preview is part of the React Data Fetching: Beyond the Basics course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to React Data Fetching: Beyond the Basics, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
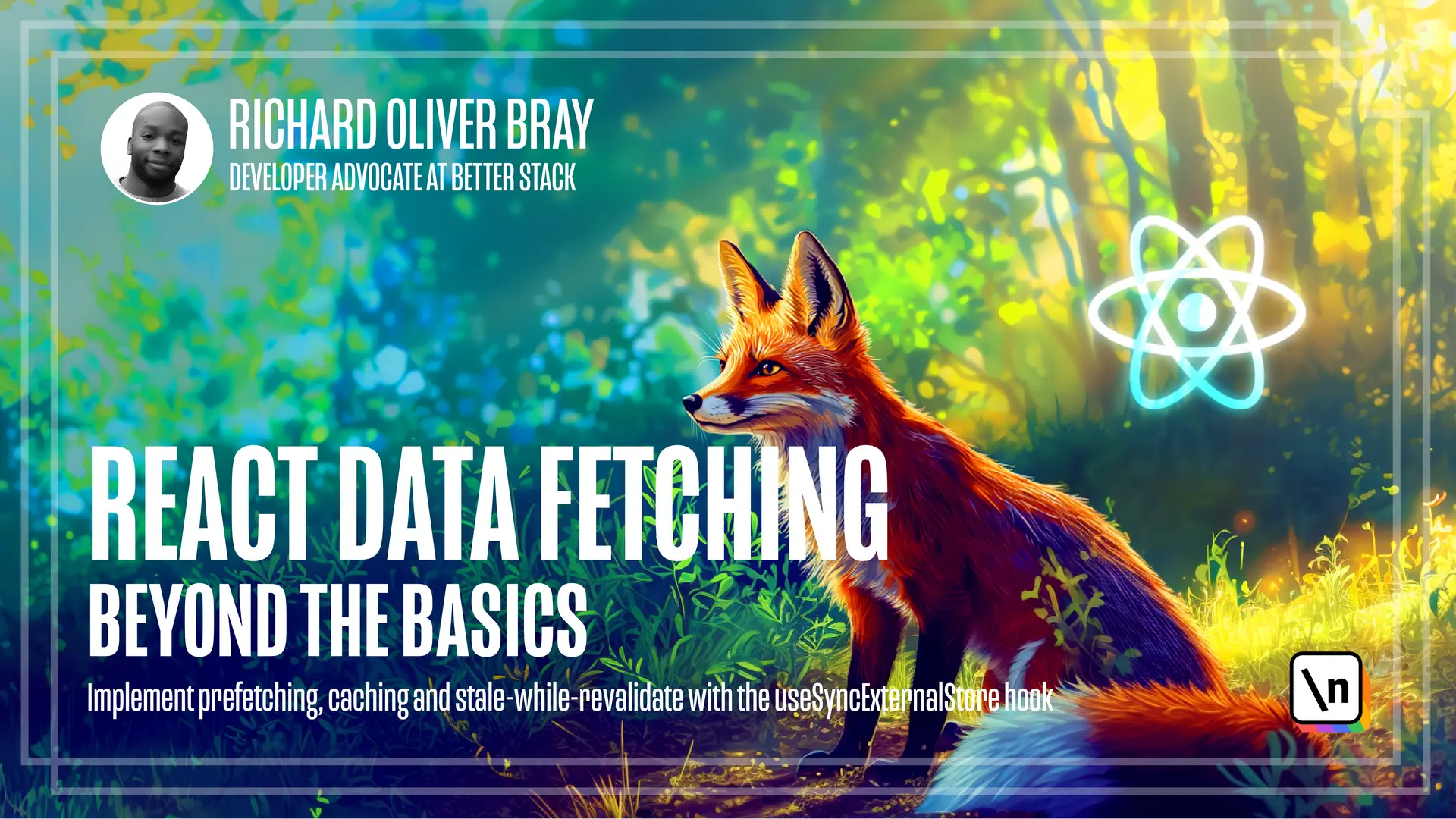
[00:00 - 00:15] In the last lesson, we added some simple caching to our data loader and in this lesson, we'll look at how to fetch data on an event and add it to the cache. But let's first talk about why we might want to fetch data on an event.
[00:16 - 00:49] Right now, our application is prefetching homepage data, but if we click on a specific Pokémon, the data is being fetched only after the component has loaded with a use effect hook, but we could be a bit more clever. Since we already know the URL of the data we need to fetch, we can start fetch ing on click, so if I go back, we can start fetching when I click this button, or we can even fetch the data when we hover over this button, which will start the fetching process before a user has clicked on the button.
[00:50 - 01:05] Let's go into our code and implement this. Just to heads up that in my previous videos, the format on save option was turned off, but going forward, I'm going to turn it on so that whenever I save a file, it will format with prettier automatically.
[01:06 - 01:09] Cool. Let's go to our data loader.js file.
[01:10 - 01:31] Below our prefetch function on line 14, let's create a new function, so I'm going to go to the end of line 19, hit enter a few times and write export function, and we 're going to call this function prefetch on event. Let's add parentheses and inside the parentheses, we're going to give it two arguments of key and function, and then let's add curly braces.
[01:32 - 01:45] What we want to do here is to first check if the data is already in the cache and return it if it is. So inside the curly braces, let's hit enter, and in fact, I'm going to press enter a few times, so we're not looking at the bottom of the screen.
[01:46 - 01:59] And here on line 22, I'm going to write an gift statement and inside the parentheses, we're going to write data cache dot has add parentheses, and we're going to give it an argument of key. So if it does have the key, then we just want to return.
[02:00 - 02:09] And if data isn't in the cache, then we can run our prefetch data function. So at the end of line 22, hit enter and write prefetch data.
[02:10 - 02:14] And here we want to pass arguments of key and function. Nice.
[02:15 - 02:23] And this should be it. Now let's go to our home component to trigger the fetch on hover, but scroll down to our link component, which should be online 21.
[02:24 - 02:31] And here after the two prop, let's create a new prop. And we're going to call this on mouse enter.
[02:32 - 02:39] And to prevent us from strolling left and right on this page, I'm going to open the command palette and toggle word wrap. Cool.
[02:40 - 02:47] We're going to make our new prop equal curly braces. And here we're going to give it an arrow function, which will run our prefetch on event function.
[02:48 - 02:57] And this is going to take in two arguments, the key and the function we want to run. So as I had parentheses, an R key is going to be Pokemon dot name.
[02:58 - 03:09] And the function is going to be an arrow function that will run the get Pokemon details function. And this takes an argument, which would be our Pokemon dot name.
[03:10 - 03:16] Sweet. Before we check this in the browser, let's quickly run through what this is going to do.
[03:17 - 03:34] So in our link component, if someone hovers over it, so on mouse enters, it's going to run this function, which is our pre-fed on event, which takes a key, in this case it's Pokemon name and takes a function, which will fetch the Pokemon data. First it's going to check if this key already exists in the cache.
[03:35 - 03:48] And if it does, it will just return that data. And if this key doesn't exist, then it's going to create a new entry in our cache with that key and it's going to run this function and save this data inside our cache.
[03:49 - 03:55] Cool. Make sure that both prefetch on event and get Pokemon details are imported correctly at the top of our file.
[03:56 - 03:59] Now let's go ahead and test this in the browser. Okay.
[04:00 - 04:07] So when the page loads, all Pokemon data is being fetched. And if I hover over Ivysore, you can see that data is being fetched as well.
[04:08 - 04:24] Now let's go ahead and update the code in our details component to use the cache data, instead of fetching it again with the use effect hook. Let's first copy all of line eight from the home component, since we're going to be using it in the details component as well.
[04:25 - 04:39] Now in the details component, at the end of line eight, press enter and paste the code to be copied from the home component. Let's change the word Pokemon to Pokemon and we can delete all the code on line eight.
[04:40 - 04:52] We can then copy all the code from line 12 to 11, press command X to cut it and paste it above line eight. What line eight and line nine are doing is getting the query peram from the URL .
[04:53 - 05:13] So in this case, the query peram will have the URL of the Pokemon name extracting the name, so that's what is going on on line nine, and we're using that as an argument to fetch the data. Now because by this stage, the data should already be fetched with the prefect on event function, all we have to do is use the Pokemon name as a key to get the data from the cache.
[05:14 - 05:28] So we can copy this code from line nine and we can get rid of everything else. Then on the new line nine, with our use data hook, we can get rid of this key of Pokemon and paste in the key of the Pokemon name.
[05:29 - 05:39] Before I forget, let's make sure we're importing used data from our data loader file and we can see that's been added on line six and we can get rid of all the code from line 15 to line 12. Nice.
[05:40 - 05:47] We can also get rid of all the code on line one and line two. Now let's go over to the browser and test this out.
[05:48 - 06:07] Let's quickly refresh the page to make sure data is being fetched correctly and we can see it is and if I hover over Iveysaw that data is being fetched and if I click on view details for Iveysaw, that data is being populated on this details page. If I go back and click on Iveysaw again, that data is not being refetched.
[06:08 - 06:13] It's only being fetched once and being retrieved from the cache. We can also test this for a different Pokemon.
[06:14 - 06:22] So let's try Bobosaur hover over and that's being fetched here. Click on Bobosaur and the data is being populated on the page.
[06:23 - 06:35] Very cool. This already makes the app feel a lot faster and if only written around 26 lines of code but there's still a lot we could do to our custom data loader.
[06:36 - 06:40] In the next lesson we're going to look at how we can handle error and loading states.