Fetching on the Server
Here we'll learn how to prefetch data based on the route
This lesson preview is part of the React Data Fetching: Beyond the Basics course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to React Data Fetching: Beyond the Basics, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
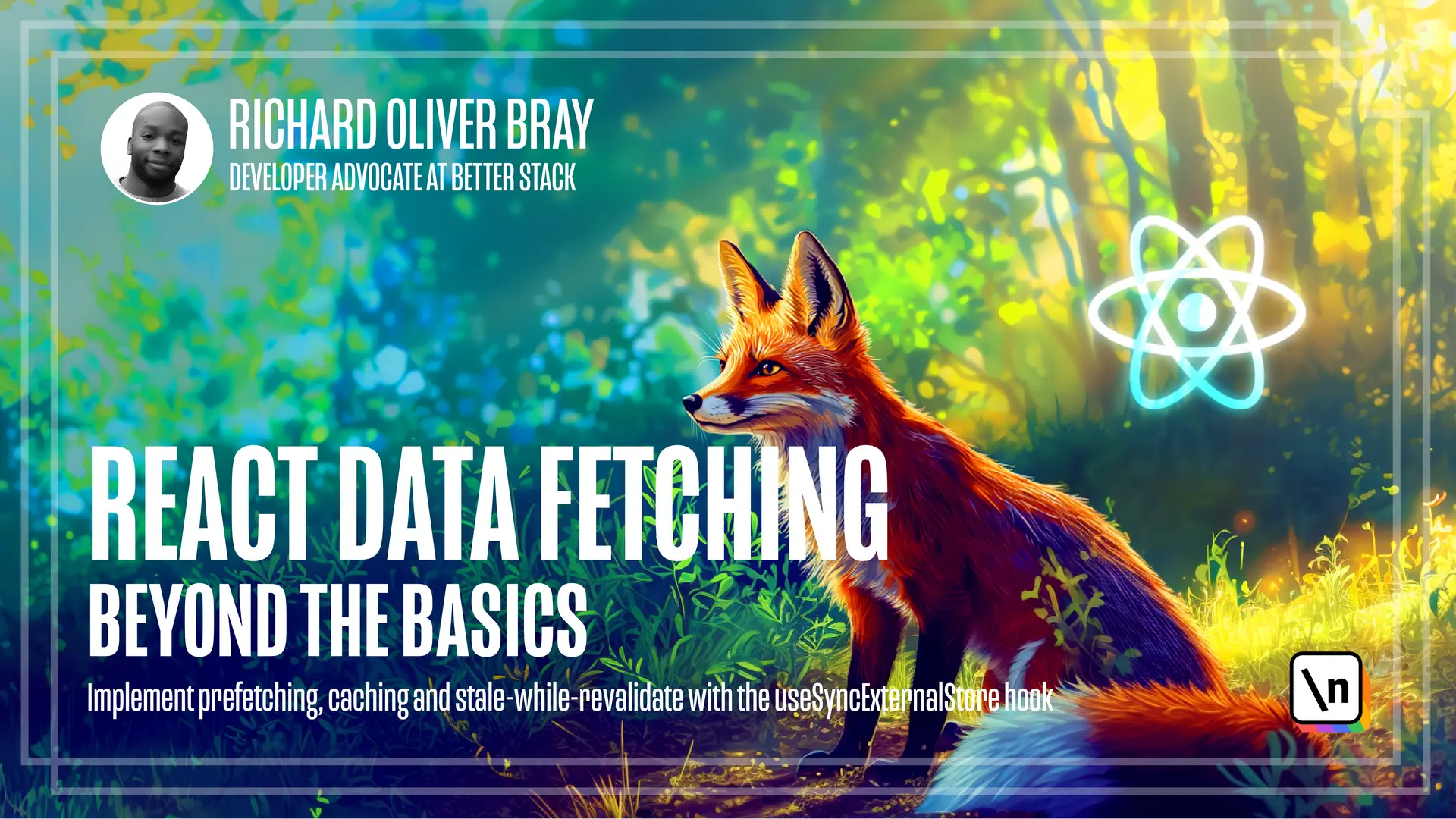
[00:00 - 00:09] Throughout this course, we've been addressing problems with the use effect hook , especially when it comes to data fetching. And these problems have been raised in the React documentation.
[00:10 - 00:16] We've addressed most of these problems apart from one. Which is, effects don't run on the server.
[00:17 - 00:29] This is because the scope of this course is to focus on client-side data fetch ing. However, I thought it would make sense to have a video that would at least talk about how fetching could be done on the server.
[00:30 - 00:43] Particularly with React Server Components, which at the time of recording this video is only production ready in next JS. For other frameworks like Waku, my framework of choice, it's still in development.
[00:44 - 00:56] And even in the Canary version of React, so all this stuff is pretty new. Server Components are either built on the server for a dynamic page or on build time for a static page.
[00:57 - 01:12] They can't use any React state, so use state or use reducer, and they can't use any rendering live cycles, so use effect or use layout effect. They also can't use any browser APIs or any hooks that depend on state and effect.
[01:13 - 01:22] So if you want to use these features for a more reactive page, you'd have to create a client's component. Which needs a use client directive at the top of the page.
[01:23 - 01:48] So if we rebuilt our Pokemon app with React Server Components, the homepage wouldn't need a loading spinner since it would fetch the data on the server before rendering the page to the client. This is different from client side rendering, which will get the HTML from the server and then render the page after the JS has loaded, which will then fetch data from the external source and then re-render the page once the data has been fetched.
[01:49 - 02:11] This means that if we were to click the show details page for a specific Pokemon using server components, then the server would make an API call to an external source , get the data, and then render the page after it has the data. Which means by default it won't cache the data, or it won't be able to fetch the data on an event like click or hover.
[02:12 - 02:29] This also means that server side rendering won't give you the race condition issue, but you could still have network waterfalls. Basically server components don't fix all the issues with data fetching, but it 's possible to put a client component inside a server component which will create a client boundary.
[02:30 - 02:45] Inside the client boundary we could use our data loading library to cache data and fix issues with network waterfalls and race conditions. But this means that if a user were to visit a new page like the details page, then the server would need to refresh the data.
[02:46 - 03:24] One way this could be addressed is to cache the data on the server using something like Redis or memcache, which means a call from the client to the server would cache the data so that when it's needed again it could be retrieved quicker, but the client still needs to make an API call to get the cached data. This means that we could pre-fetch on the client by making an API call to the server which would then fetch the data and cache it so that if another call is made from the client to the server it could check if the data is in the cache and then get the data again instead of making another call to an external service.
[03:25 - 04:05] But the problem with this whole process is that the client needs to make API calls each time to get cached data from the server instead of having its own cache which will be quicker because you don't have to make a call to the server. Alternatively we could just do the initial fetch on the server, it would make a fetch call to the first 150p and render that on the client, but then if any further calls need to be made, so for example if you hover over the details button then that API call could be done on the client and then stored in the client cache so that when the page is rendered it won't be done on the server, it will be done on the client instead and the client could make use of the data cache.
[04:06 - 04:24] So if we went with this approach we'd have to have a wider client boundary which would rely on JavaScript for reactivity. But we can still keep part of the site server rendered, so for this heading if it doesn't change that much then it could be rendered from the server and this bit over here could be rendered by the client.
[04:25 - 04:36] This means that if we click on the button then only the client boundary will change to show the data from the view details component. Now the approach you take will depend on the type of app you want to build.
[04:37 - 05:00] If you want an app that heavily relies on SEO and doesn't have that much reactive or dynamic information then it could mostly be server rendered or even static site generated. But if you have a lot of reactivity and you need a lot of JavaScript like a real time app or a social media app that needs instant feedback when you use it clicks then you could use server boundaries for most of your application.
[05:01 - 05:20] As previously mentioned, react server components are fairly new and the more people use them the more ways people will discover how to optimize them. Currently they've been implemented in next JS, Raku and will be introduced into Remix and I'm sure there'll be many other react server component frameworks in the future.
[05:21 - 05:35] So there may be a really good solution for data fetching on the server and the client side when it comes to react by the time you're watching this video. For now I think our data loading library is pretty good for client side data fetching.
[05:36 - 05:44] Going in at just under 120 lines of JavaScript code. Of course there are many ways our library could be improved.
[05:45 - 06:04] We could add optimistic updates or add help us for pagination and infinite scroll or even do some refetching when a user comes back online after being offline and we could have some logic to invalidate the cache manually in case you want to force a refetch . But for now, I think it's a good place to stop and wrap things up.