Setting Up a Formatter and Linter for Svelte Development
Setting up a Formatter and Linter
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
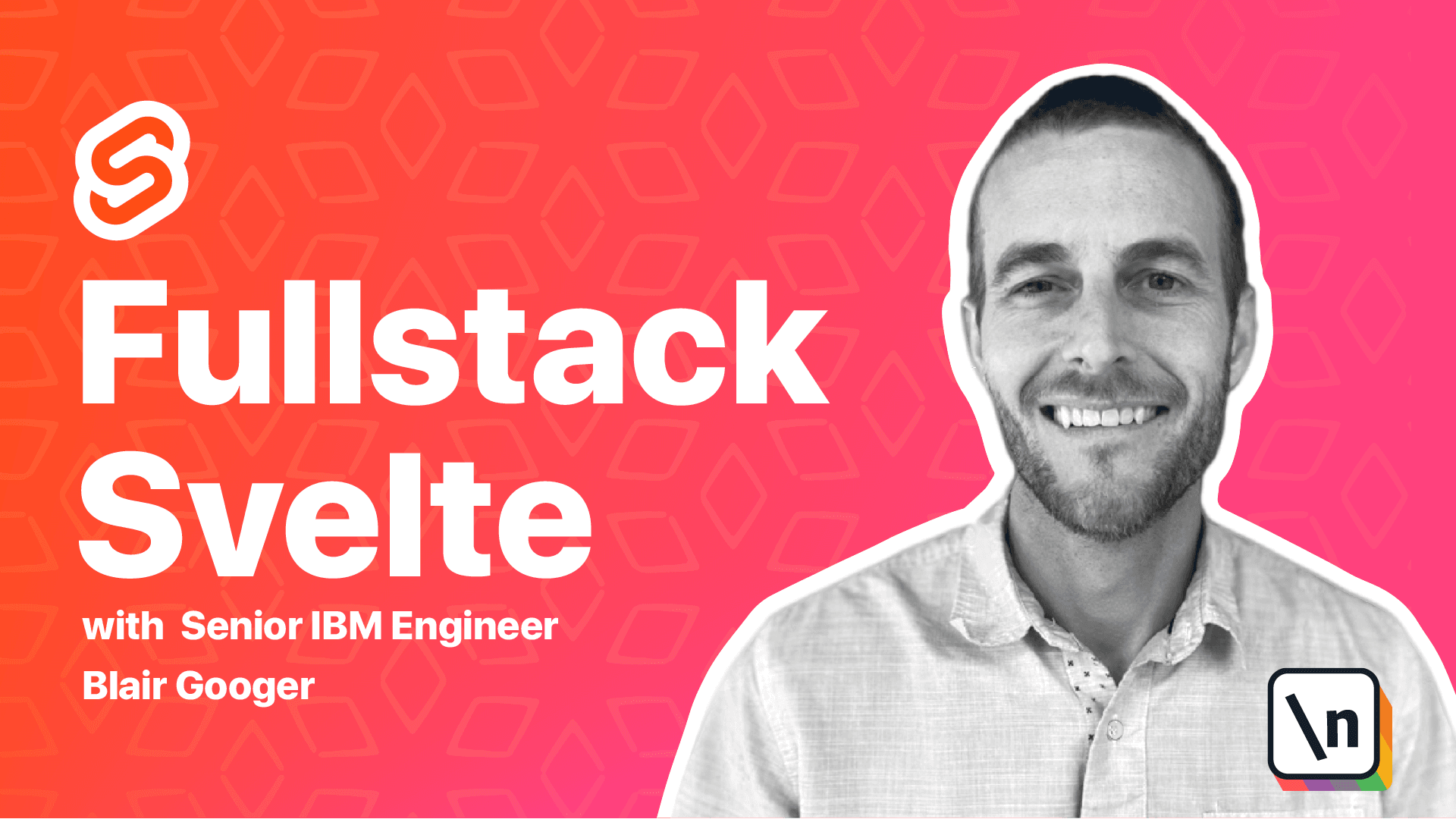
[00:00 - 00:08] In this lesson, we're going to talk about formatting and linting. As developers, we want to craft consistent and readable code.
[00:09 - 00:20] And even though it's a little bit of a pain, it's worth spending some extra time now to set up formatting and linting tools that'll help us keep our code clean. And in some cases, even catch bugs.
[00:21 - 00:29] So formatting is just like what it sounds like. It's a tool that will automatically indent and space the code.
[00:30 - 00:47] And linting is the process of statically analyzing code for problems, such as undeclared variables, unused variables, and other syntax issues. In this lesson, we're going to use the prettier and ES lint extensions for VS code.
[00:48 - 00:57] And then we'll look at a few examples of how the tools actually help. So head over to VS code, and then click the extensions tab.
[00:58 - 01:07] Now I've already installed these extensions and some other ones, and there's tons of different extensions you can use from the marketplace. So I'd encourage you to check those out as well.
[01:08 - 01:15] But the ones we're interested in right now are prettier. So go ahead and search for that one and install it.
[01:16 - 01:25] Go ahead and install the Svelte language support for VS code extension. And then go ahead and install ES lint as well.
[01:26 - 01:44] Now once you have those installed, I think it's really nice to format on save. So you can go to VS code, preferences, and go to settings, and then search for format.
[01:45 - 01:54] And check the format on save checkbox. And so this will just automatically format your files every time you save them.
[01:55 - 02:06] Now with different versions of VS code, sometimes this could be a little bit picky. So you may also need to set prettier in the default form matter box here.
[02:07 - 02:31] And then if you're still having trouble specifically with Svelte files, you may want to click this button here to open settings in JSON mode and add this bit of code right here. And this will specifically point the James Burdles Svelte code formatter, which is integrated with prettier.
[02:32 - 02:41] And it'll specifically set that up with Svelte files. So you may have to tweak it and spend a little bit of time with it to get it just right.
[02:42 - 03:00] But like I said, I think it's worth it for that formatting feature. So another thing that we need to do at this point is install the prettier plug- in Svelte from the command line.
[03:01 - 03:12] So copy this command here and head over to the command line. If you're running your local development environment still, you can hit Ctrl C to stop it.
[03:13 - 03:18] And then I'm in the front end directory. So I'm going to run this npm command.
[03:19 - 03:35] And that'll save this as it'll install it and save it as a dev dependency in package.json. And then heading back to VS code, we're going to set up a prettier RC.json file .
[03:36 - 03:46] And this'll be configuration for prettier. So back in VS code, go to the root of the front end project here.
[03:47 - 03:53] And right click and choose new file. Create this .purdier RC.json file.
[03:54 - 04:04] And then paste this configuration into that file. So these are just some rules that we're giving prettier.
[04:05 - 04:14] And so this will enforce single quotes so that we're consistent with that. And I have mine set up with semicolons as false.
[04:15 - 04:20] I'm not a semicolon and JavaScript guy. Now of course, if you do like those, you can set that to true.
[04:21 - 04:39] And you can play with your tab width and so forth to tailor it to how you like to format your JavaScript code. So now that we have that set up heading back to main JS, if I hit control S right now to save it, you'll notice that the semicolon went away.
[04:40 - 04:51] And also the double quotes went away. So now every time I save a file, even if I add semicolons in, it will automatically remove them.
[04:52 - 05:00] Let's go one step further now and look at ES lint. So we've already installed the ES lint plugin for VS code.
[05:01 - 05:13] And next we need to run an npm command to install ES lint libraries. So go ahead and run that in your terminal.
[05:14 - 05:27] And while that's running, we also need to set up an ES lint RC.json file. So we'll go ahead and set up that in the root of the project.
[05:28 - 05:33] And this will be the configuration that we use for that file. So paste that in there.
[05:34 - 05:45] And so this configuration will use a svelte plugin. And so that'll tell ES lint to actually lint.svelte files, which we want to do.
[05:46 - 05:56] We want to do svelte files in addition to JS files and HTML files and so forth. So this configuration will work for that.
[05:57 - 06:12] Now in order to actually lint the files, we need to run npxes lint and tell ES lint where to lint. And so what we're going to do is add this to our npm scripts.
[06:13 - 06:20] So in package.json, we have a build script and a dev script. And this is what they look like out of the box.
[06:21 - 06:29] So we're going to update them to run our linter first. And this will be an opportunity to catch errors while we start the project.
[06:30 - 06:46] So copy this and open up package.json. And then find the script section and at the front of the build script, add in the npxes lint command.
[06:47 - 07:00] Now this syntax will work for max and Linux machines, but you might have to adjust it slightly for a Windows use case. I don't think that the .slash source will work on Windows.
[07:01 - 07:06] So I think that this would work on Windows. Okay.
[07:07 - 07:15] So now also copy this down to the dev command. And so we'll run our linting process on both start and dev.
[07:16 - 07:20] And so let's give this a try. So back to the command line.
[07:21 - 07:37] Now if we run npm run dev. So as you notice, it ran the linter first and then it ran the npm normal dev startup process.
[07:38 - 07:48] So let's check out and see if our linter is actually working. So one of the things that linting does is it won't let you have undeclared variables.
[07:49 - 08:11] So we can set up a little test case here and try to console log a variable that doesn't exist. So copy this into the app.spelt file and save that and then recycle the local dev environment.
[08:12 - 08:22] So hit control C, then hit the up arrow and run the same command again. And so now you can see an example of a linting error.
[08:23 - 08:35] So this is pretty cool because this would be a bug that we could potentially introduce into our app and the linter caught it. So it tells you what file the error is found in and then what line number.
[08:36 - 08:56] So it's telling me to look at line three in the app.spelt file and it's telling me that this is hitting the no undeath linting rule. So let's get rid of that, save that and now we've got the project cleaned up again.
[08:57 - 09:12] Very good. We installed prettier and es lint and we've got our local dev environment set up a little bit better to make sure that we're formatting our files and also catching some bugs before they make it to production.
[09:13 - 09:43] We've made great progress and in the next module we'll set up a spelt router and stub out some of the pages in our application. [ Silence ]