Building Svelte Single Page Applications and Serving Static Files
Building and Serving a Svelte App
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
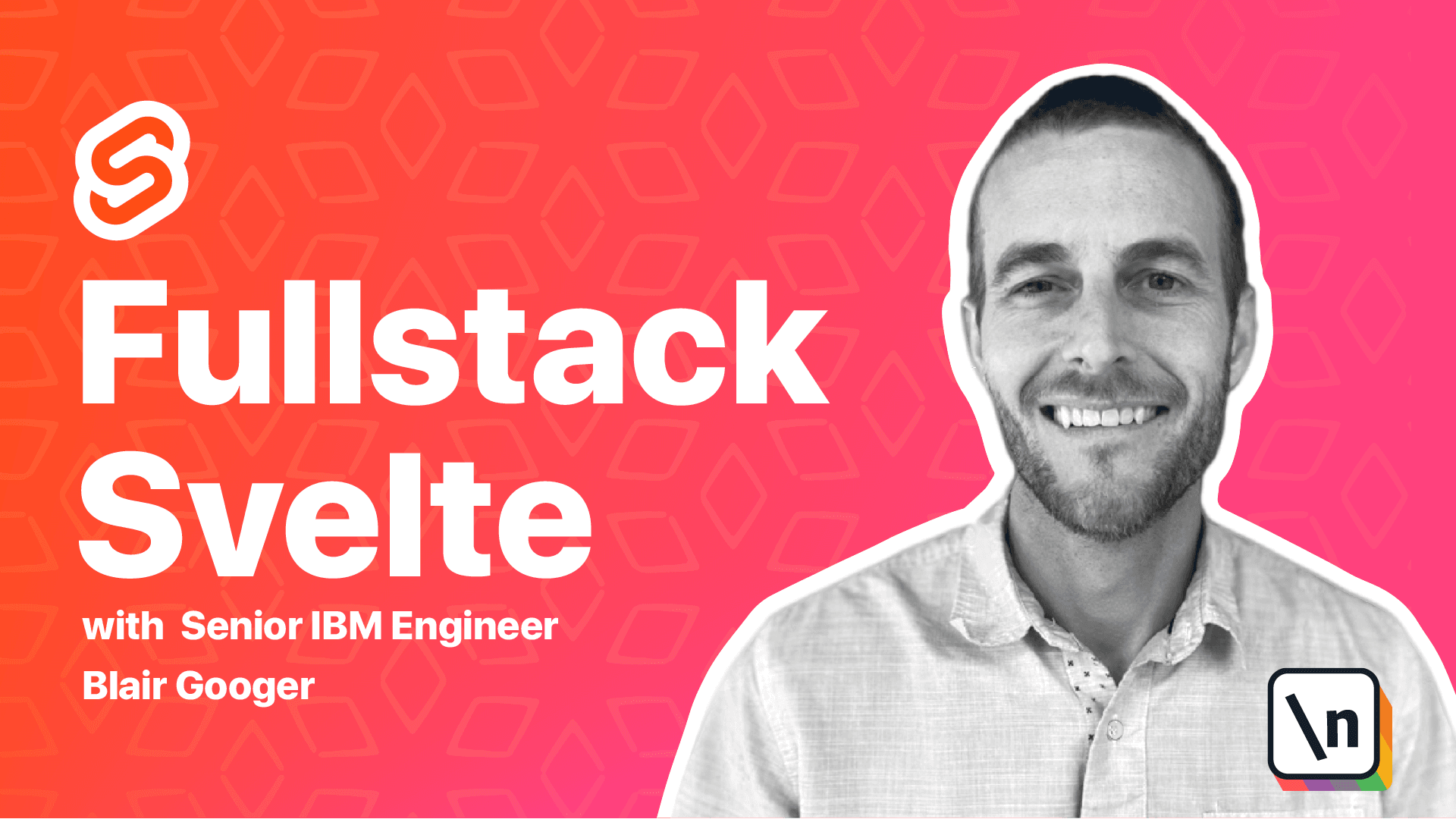
[00:00 - 00:12] Hello, we're back again to talk about production deployment. So the first thing for production deployment that we need to figure out is building a spell tap.
[00:13 - 00:35] Up until now, we've been running our spell tap with a local dev server on port 5000. And as web browsers can't run spell files directly, the spell framework converts our spell files into a single JavaScript file called bundle.js and a single CSS file called bundle.css.
[00:36 - 00:50] And in the local dev environment, the local setup automatically runs a new build after every file change and then hot loads the new build into the browser. And that's awesome for development because you see your changes immediately.
[00:51 - 00:58] But that won't work for production. So to build a spelt app for production, we run the command npm run build.
[00:59 - 01:10] So let's give that a try. So in the front end of the project, npm run build.
[01:11 - 01:26] Okay, so we got created public build bundle.js in 6.3 seconds. Let's get rid of these a 1 1 y errors.
[01:27 - 01:34] These are just accessibility errors. And we just need to add the four attribute to each of these labels.
[01:35 - 01:47] So over in home dot spelt. So where we have a label, just say four equals email.
[01:48 - 02:16] Four equals school name, etc. Okay, let's give this a try again.
[02:17 - 02:31] So npm run build in the front end. And we get the files created in public build bundle.js.
[02:32 - 02:52] Okay, so let's take a look back in VS code and under the front end project, the public directory, here's the files that were built from npm run build. We've got our bundle dot CSS, our bundle dot JS, and then our index dot HTML.
[02:53 - 03:00] And so index dot HTML is going to refer to bundle CSS and bundle JS. Now both of these files are minified.
[03:01 - 03:10] And so this makes the file size much smaller and it's just a performance optimization. And then we've got this bundle JS map file.
[03:11 - 03:27] And this is a source map. And so what this does is it just maps this minified, uglified file to the original source code so that when we see errors in the console, we can click on those and see the original source code and it's kind of nice for debugging.
[03:28 - 03:42] Okay, so all of this stuff here represents the whole entire Svelta app. So it gets built into just these couple of small files.
[03:43 - 03:54] And those files are actually ready to be deployed to a web server. And they're known as static assets and they could be served from any web server .
[03:55 - 04:07] But what we're going to do is use our express app as both our API portion and we're going to serve the static files. So we're going to serve those static spell files from our back end.
[04:08 - 04:16] And then we'll just have one back end that does everything. So we got to do a little bit of setup on our express server to do that, but it 's relatively easy.
[04:17 - 04:26] So kind of the main thing we need to do is copy the files from front end public to back end public. So let's go on the back end public.
[04:27 - 04:34] There's some boilerplate files there. We'll just get rid of those and then we'll copy the files in and go from there.
[04:35 - 05:02] So let's see, let's close this stuff here. So on the back end public, let's get rid of these style sheets and this index dot HTML file delete all this stuff.
[05:03 - 05:21] So we've got just a public directory there and a public directory here. So we're going to pull forward build, babe icon in index dot HTML, copy that there and then paste it into public here on the back end.
[05:22 - 05:25] Okay. So let's give this a try.
[05:26 - 05:41] Now I'm going to recycle this just to show you something. So if we run npm run nodemon now, we're going to get a bunch of errors.
[05:42 - 05:55] And that's because the minified, unglified code doesn't conform to the ES lint rules. So we're going to use an ignore approach so that we don't look at anything in the public directory.
[05:56 - 06:21] So we just need to add ignore patterns public star to our ES lint RC dot JSON file. So on the back end, yes lint RC dot JSON, just add that little bit of configuration there, save that and then try npm run nodemon again.
[06:22 - 06:40] Okay. So previously we were running our front end on localhost 5000.
[06:41 - 06:46] I don't have that even running now. So we're not getting anything there.
[06:47 - 06:58] And our express backend runs on port 3000. So now that we've copied that code over, if we run localhost 3000.
[06:59 - 07:07] Now we see our index file. So this index dot HTML file is now being served from our express backend.
[07:08 - 07:14] So we get that one file. And if we hit log in, we're actually going to get an error.
[07:15 - 07:24] And this is because we haven't allowed localhost 3000 as a callback URL. Inside auth zero.
[07:25 - 07:41] So auth zero is treating port 3000 and 5000 as two different servers. So let's go to auth zero, go to applications, go to our front end.
[07:42 - 08:07] And then in all of these spots where we have 3000, let's add, or excuse me, where we have 5000, let's add 3000. And just use commas to separate them.
[08:08 - 08:30] And then save that down at the bottom. Okay, so let's give that a try.
[08:31 - 08:36] Okay. Now when we log in, this time auth zero allowed it.
[08:37 - 08:41] And so we actually got to our app. Now here's another thing that we have to deal with.
[08:42 - 08:51] So when we hit that log in button, we were redirected here. And that was a JavaScript only redirect.
[08:52 - 09:05] And so from a backend server perspective, we were kind of still on the index route and only the front end knew that we changed routes. But we want to be able to go directly to one of these routes and hit the refresh button.
[09:06 - 09:19] And so when we're doing this, we're sending this call to the backend and the backend doesn't know how to handle that. So we're going to use a technique called a fallback route to deal with that.
[09:20 - 09:34] Okay. So essentially, we need a little rule that if we don't match one of these routes, these are all our API routes, then any other route will just serve up our index dot HTML file.
[09:35 - 09:45] And that way, any other route regardless of how it looks, whether it has an ID at the end, etc, will serve index dot HTML. Okay.
[09:46 - 09:55] So copy this bit of code here for the fallback route. And it needs to go into app JS on the backend.
[09:56 - 10:06] And it needs to go into the bottom because we want to hit the API routes first. And if none of those match, then we'll hit our fallback route.
[10:07 - 10:13] Okay. So let's give that a try.
[10:14 - 10:32] So now when I hit refresh, I'm going to get my index dot HTML file served back to me, which is what I want. And regardless of where I am now, if it doesn't hit an API route, it's going to serve index dot HTML.
[10:33 - 10:40] So now we're back in serving both the API routes and index dot HTML. Okay.
[10:41 - 10:50] All right. So last thing we're going to do here is enhance our spelt build.
[10:51 - 11:05] So earlier we ran npm run build and that created the spelt files, but then we manually copied them to the backend. And this is a good place to use a little bit of scripting to automate that step .
[11:06 - 11:15] That's a step that you could easily forget to do or make an error in doing. So we want to try to automate things like that so that our builds are consistent and work well.
[11:16 - 11:32] So inside the front end package JSON, we're going to run that build and then move the files over with a little bit of a script. So this is what we need right here.
[11:33 - 11:39] So go to the front end package JSON. And so here's our build script.
[11:40 - 12:01] Right now we're just doing es lint and then roll up dash C. So we're going to put in something a little more sophisticated here. So we're going to do those same commands and then we're going to remove the stuff from the public directory and then copy it to the backend.
[12:02 - 12:11] Okay. So it's going to remove what was in the backend previously and then copy the stuff from front end to the backend.
[12:12 - 12:22] Just a small disclaimer, this works on Mac Linux. You may need a slightly different syntax on Windows, but the same concept will work.
[12:23 - 12:33] So let's give this try here. So let's just say, I'm going to change this just briefly and save that.
[12:34 - 12:49] So now if we run another front end build, it should delete all of these files and copy the good files back in. So back to the front end, npm run build.
[12:50 - 13:02] Okay. Now, may not have noticed it, but copy it deleted these files and copy the good files back in.
[13:03 - 13:08] And so now you can see the bundle.js there. And that's it for this lesson.
[13:09 - 13:22] So a little bit of a short lesson and this is just setting the stage for being able to deploy to an actual server. So now we've got our express backend serving both the API and the static files.
[13:23 - 13:31] And so these are, this is a little bit of a tricky area for single page apps, but with just a little bit of express configuration, we can handle what we need to.