A Guide to Handling DOM Events and Input Binding with Svelte
Using Svelte to handle DOM events and bind inputs
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
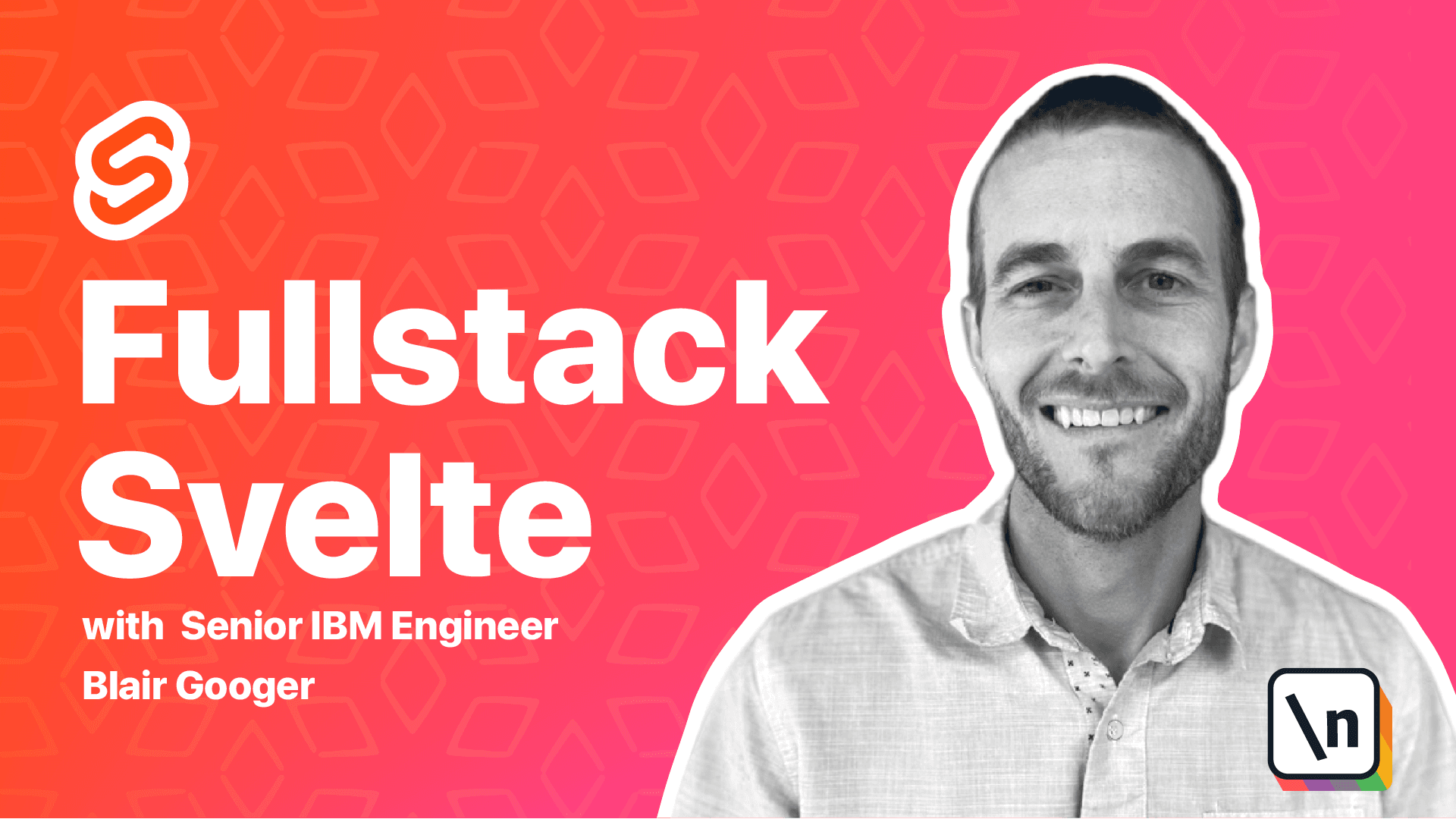
[00:00 - 00:12] Okay, welcome back. When we left off last time, we finished creating our landing page, but our landing page doesn't have any interactivity.
[00:13 - 00:29] So even though we could type into these inputs or click these buttons, nothing happens. So we'll start addressing that in this lesson and we'll cover the concepts of input binding and event handling.
[00:30 - 00:51] When we say input binding, we mean taking the value of an HTML input and updating an underlying reference variable in JavaScript, and then inversely taking values from JavaScript variables and displaying them in the inputs. Sometimes this is called two-way binding because it will work in either direction.
[00:52 - 01:06] First felt makes it pretty easy. All we have to do is use bind value equals var name. And we just put that directly on the relevant HTML input.
[01:07 - 01:19] In the case of our landing page, we've got two inputs right now, the email address input in the school name. So we'll create two state variables, and then we'll bind our inputs to those variables.
[01:20 - 01:30] So let's grab these two variables and go over to home.self. So we don't have a script section at this point.
[01:31 - 01:40] We only have markup. So we'll add a script section to the top.
[01:41 - 01:50] And we'll put those two variables in there. And then we need to add our bind directives.
[01:51 - 01:58] So I'm just going to copy this little bit here. And this will be for the email address input.
[01:59 - 02:07] So right down here in the markup, find the email address input. And we'll add that directive there.
[02:08 - 02:22] And then likewise, on the school name input, we'll add another one. And this time, we'll use the school name variable.
[02:23 - 02:35] And so just for demonstration purposes, let's say we set this to [email protected]. So we've set the email address variable.
[02:36 - 02:50] And we should see it now in the form. So there you can see that that underlying variable has been bound to the input.
[02:51 - 03:06] And here's another way to visualize this. We can anywhere in a svelte file in the markup section, we can add JavaScript by using curly braces.
[03:07 - 03:25] So let's just say, let's say div email address is, and then we'll add curly braces and we'll refer to the email address variable. This is just temporary code, just to test it and show the concept.
[03:26 - 03:40] And then we can do the same thing for the school name. So now we've got email addresses, test, school name is undefined.
[03:41 - 04:09] And so as soon as we start typing into this input, you can see that svelte is automatically two-way binding the value from this input to our variable and then reactively showing it up here. And then if we change this one, same thing will happen there.
[04:10 - 04:18] So that's input binding. And so basically we're getting the values of our inputs bound to a variable in our JavaScript.
[04:19 - 04:25] And then we can do things with it. Like for example, we could send that information to a server to save it in a database.
[04:26 - 04:32] Now that we have it in the JavaScript variable. So let's clear this.
[04:33 - 04:38] Get rid of this. Okay.
[04:39 - 04:46] Here's another scenario. Imagine that we have a clear function.
[04:47 - 05:06] So we might have something like function clear. And we would say email address equals null school name equals null.
[05:07 - 05:17] So this would be something we would use maybe after the form has been completed and we want to clear it back off. And so when we did that, again, svelte would automatically update those inputs and clear them.
[05:18 - 05:21] Okay. So get rid of that sample code.
[05:22 - 05:31] Now let's talk DOM events. So we have a couple of buttons on our landing page, but they won't do anything until we add event handlers to them.
[05:32 - 05:38] And svelte has an easy approach for handling events. We just need to use an on directive.
[05:39 - 05:50] So we say on event name and then the function name that we want to call when that event happens. So we don't have a back end for our application yet.
[05:51 - 06:02] So we're not going to actually register a user, but we'll just stub out a click event handler for the go button. So we're just going to show an alert whenever the go button is clicked.
[06:03 - 06:13] Later on, we'll come back and address this. So let's add function register.
[06:14 - 06:27] So there's our function. At this point, it's just sitting in the script and it's not really going to do anything until we add on click and then call that register function.
[06:28 - 06:34] So I'm copying this little bit and going down. So here's the button.
[06:35 - 06:46] So paste that in there. And so now svelte is going to handle click events and call the register function when a click event happens.
[06:47 - 06:54] So let's give that a try. Now our alert is going to show the values from these inputs.
[06:55 - 07:05] So I'm just putting some test data in there. And so now you can see that events handled and we show an alert email is test and school name is school.
[07:06 - 07:17] Okay. Now one more thing in this lesson, we still have a login button to deal with.
[07:18 - 07:29] And later on, we're going to introduce auth zero. And when a user clicks log in, we'll actually redirect them to auth zero to perform the login and then they'll come back to our app.
[07:30 - 07:42] But that's a little ways away. So for now, we're just going to redirect the user to the lunch menu admin component when they click the login button.
[07:43 - 07:55] So to do that, we're going to use the navigate component from svelte router spa . And that'll let us trigger navigation through our spa routing.
[07:56 - 08:12] So let's import this navigate component here. And then copy this bit of code here.
[08:13 - 08:17] And so you can see kind of the outline of it. So we've got the navigate component.
[08:18 - 08:23] We tell it where to navigate to. And then we put the button inside it.
[08:24 - 08:42] So we'll go paste that there. Now we don't need a click handler in this case because the navigate wrapper component handles app for us.
[08:43 - 09:00] So now when we click login, it'll perform that navigation and drop us straight into the admin area of the app. So those are two other important concepts in Selt.
[09:01 - 09:09] And then one concept from svelte router spa. And this is the last lesson for a little while on the front end.
[09:10 - 09:20] Next in the next module, we're going to work on the backend express application . So I'm going to do a review of everything in the front end that we've covered so far.
[09:21 - 09:30] So just looking at the code, we've initialized our svelte app. So we've got a public folder and inside here has an index dot HTML.
[09:31 - 09:44] And this is the base HTML file for our single page app. So inside this file, we got rid of a few things and we've added a link to the B oma style sheet.
[09:45 - 09:52] And then under source, we've got our app dot svelte file. So this is the main entry point file.
[09:53 - 09:58] And we added a little bit of markup here. We added a section and a container.
[09:59 - 10:04] And then we added our router component here. So everything flows through this entry point.
[10:05 - 10:21] And then the router component renders the appropriate page component for whatever route the user has gone to. And to accomplish that, we're using svelte router spa, which is an open source library.
[10:22 - 10:28] And then we created a routes file. So our routes dot js file looks like this.
[10:29 - 10:42] And it's got the information for each route and what component each route should point to or render when that route is accessed. Then we did something special on the admin part.
[10:43 - 10:55] So whenever we come to a route that starts with admin slash manage menus, then we've got a admin layout. And then underneath that admin layout, then we've got nested routes.
[10:56 - 11:08] And so far, we only have one, which is our index route or the root route directly at admin slash manage menus. And we call the lunch menu admin component for that.
[11:09 - 11:13] So our admin layout looks like this. And it's kind of similar to app dot view.
[11:14 - 11:22] It's kind of another entry point to all the admin pages. And so we've done a little bit of initialization here.
[11:23 - 11:32] Inside the admin layout, we use the on mount life cycle function. And so this will run whenever that admin layout is first accessed.
[11:33 - 11:42] And then we also use a svelte writeable store to write a little bit of state. So we initialize our user state here.
[11:43 - 11:54] And it's just dummy data at this point. But it's good to learn the concept and later on will come and actually use real login information from a real user.
[11:55 - 12:02] So we initialize our user state there. And then we set an initialized toggle flag.
[12:03 - 12:13] And we use conditional rendering here. So we only show the route that they're trying to access after initialize has been toggled over to true.
[12:14 - 12:18] So first we start it as false. And so therefore this won't be shown or rendered.
[12:19 - 12:29] And then when on mount is completed, we toggle it over to true. And then this will render the route that the user is trying to access.
[12:30 - 12:35] And so that's basically it so far. We've also got our lunch menu view page.
[12:36 - 12:45] And that's just kind of an empty page sitting there, a placeholder that we'll deal with later. Our home.svelte file we were just working in.
[12:46 - 12:52] And this is our landing page. So this is where we did input binding and event handling.
[12:53 - 12:58] And then lastly we've got our lunch menu admin page. And we haven't done a whole lot here.
[12:59 - 13:07] But we did import the store. And then we're accessing the store using dollar, store name, dot property name syntax.
[13:08 - 13:15] And then we added some breadcrumbs here to make it look a little bit nicer. So this is really good progress.
[13:16 - 13:21] We've got a functioning front end application. And we've got pages set up.
[13:22 - 13:26] We've learned about conditional rendering. We've learned about input binding.
[13:27 - 13:30] We've learned about event handling. We've learned about stores and routing.
[13:31 - 13:37] So we've covered quite a lot. And we're going to continue next after this with the back end.
[13:38 - 13:44] And so we'll kind of switch gears for a little while and start working on the back end portion of the application. So thank you.
[13:45 - 13:45] And we'll look forward to seeing you next time.