Front End Layouts - Creating Reusable Parent Components
Creating a Front End Layout for Admin Pages
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
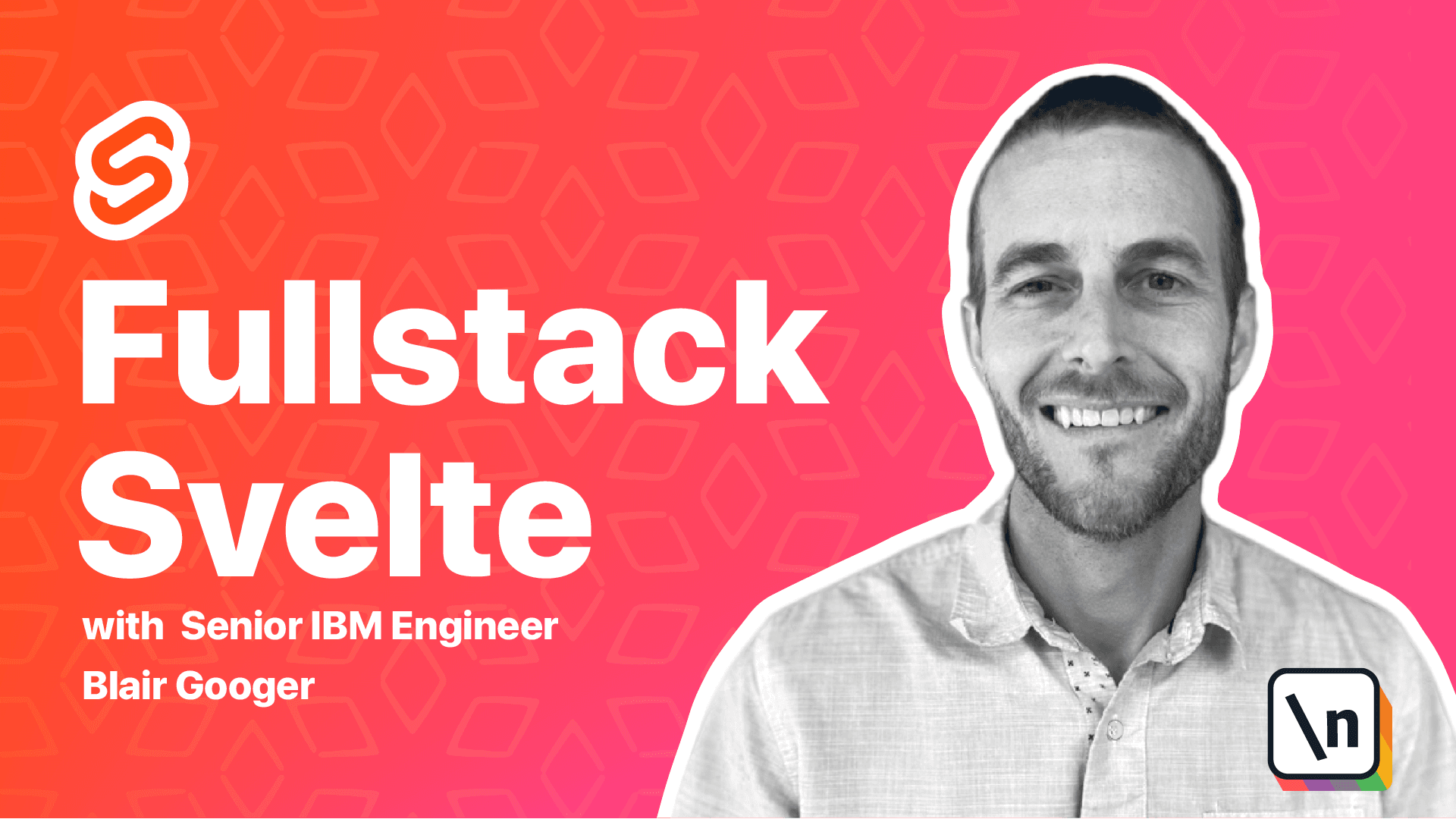
[00:00 - 00:15] In this lesson, we're going to continue with the spelt front end and work on an admin layout. So layouts are a technique for creating a common parent component that can be reused across multiple child components.
[00:16 - 00:27] So we could use it, for example, for something like a nav bar that would be at the top of all admin components. And we can also use it to run shared initialization logic.
[00:28 - 00:39] For our school lunch app, we'll create a admin layout, and we'll use that for all of our admin pages. And then we'll use it to initialize a user object.
[00:40 - 00:54] And later on, when we add authentication, we'll enhance it further to handle authentication logic. We can get started by creating a dummy import into our routes file.
[00:55 - 01:07] So we're going to adjust our routes a little bit to use the admin layout. So we need to reconfigure the routes to use a nested approach.
[01:08 - 01:22] So we're going to replace this section that we had before with a slightly different configuration. So what we've done here is we've continued with the admin manage menus route.
[01:23 - 01:39] But then the component that that will directly point to will be our admin layout. And then our admin layout will route to a nested route, which is our lunch menu admin component that we created before.
[01:40 - 02:02] In this case, index is special, and it just refers to root in a web app. So you can kind of think of this index as referring being the same thing as saying index here would be the same thing as leaving it blank.
[02:03 - 02:24] We imported the file, but we haven't actually created it yet. So let's create admin layout dot spelt under source views admin.
[02:25 - 02:46] And here's the code for that file. So what we've got here is another layer in between app dot view, which handles the main routing, then all admin routing will flow through admin layout and then route to the proper admin page under that.
[02:47 - 03:03] So similar to what we did in app dot spelt, where we imported the router and then we have the router component. Now in admin layout, we import route and then have the route component and we pass down the current route.
[03:04 - 03:12] So it's kind of like a pass through. And then we just put the words admin layout at the top.
[03:13 - 03:25] So go ahead and start the local dev environment if it's not already started. And come back to the browser and check out the app.
[03:26 - 03:32] So now we've got the words admin layout. And then our actual lunch menu admin component.
[03:33 - 03:47] So the admin layout is working so far. Next we're going to talk about stores and life cycle functions.
[03:48 - 04:01] So it has a concept of stores, which are objects that hold state that can be used by any other spelt component. So this is real similar to Redux and react and view X in view.
[04:02 - 04:24] And the admin layout file that we just created is a good place to initialize a user store that we can use throughout the application. To do this, we'll create a simple file called stores.js in the source directory .
[04:25 - 04:38] And it will export a writeable store called a user. And then we'll work with it in the admin layout.
[04:39 - 04:55] Another big concept that's very useful in spelt apps are life cycle functions. So there's a few life cycle functions that can be used to run code at specific points when loading and rendering a spelt component.
[04:56 - 05:05] The on mount function runs just after a component is rendered. So as something is loaded to the browser, the on mount life cycle function runs .
[05:06 - 05:28] And it's a good spot to load data from a server or populate user state in our case. So we're going to update our admin layout to use the on mount life cycle function and then import the user store and then set an object in the user store.
[05:29 - 05:41] So let's copy this over. Go to our admin layout.
[05:42 - 05:54] Now we've removed, just removed the text admin layout that was here. And to step through this, we're still importing route like before and we've still got route in the markup.
[05:55 - 06:11] And then we're importing the on mount life cycle function and we're importing our user store that we just created over here in stores.js. So here's where we implement the on mount function.
[06:12 - 06:32] So we refer to it here inside the script and then inside the callback, we use an arrow function to run the code that we want to load. So what we're going to do here is use user.set and set an object into that store.
[06:33 - 06:41] So this will be the value of the user store. And then later in other components, we can use this user store.
[06:42 - 06:57] So anytime we load a page that uses the admin layout, this code will run. And another very useful thing in Svelte is conditional rendering.
[06:58 - 07:12] At this point, we're setting our user state in the admin layout. But if we tried to use it in another component, there could be a timing issue because of the nature of asynchronous JavaScript code.
[07:13 - 07:25] Sometimes our page may load, but the user state may still be initializing. We can make sure that the admin layout is done initializing by using conditional rendering.
[07:26 - 07:37] To accomplish this in Svelte, we use something called an if block. We'll create a state variable called initialized.
[07:38 - 07:57] Then when the on mount is finished, we'll set it to true. And then in the markup, we'll use an if block to make sure that we don't pass the application doesn't render the next route in the chain until after initialized is finished.
[07:58 - 08:08] So let's copy this over. So back in our admin layout file, this is where we can declare the state value.
[08:09 - 08:35] So once we're done with user set, we'll set initialized to true. And then down in the markup, we'll add the if block.
[08:36 - 09:00] So this felt framework will automatically track the initialized state. And when it's toggled to true, it will render this portion, which will then trigger routing down to the component that we requested.
[09:01 - 09:16] So we're setting our user object in the admin layout. So next, let's actually use it and display some information from it in our lunch menu admin component.
[09:17 - 09:37] So it's very easy to refer to state that's in a Svelte store by just using the dollar sign store name. So copy this and head back to the lunch menu admin component.
[09:38 - 09:47] And we'll get rid of this temporary code that we were playing around with. And we'll add import user from the user store.
[09:48 - 09:57] Then all we have to do is use dollar sign user. And then we can refer to anything in that store.
[09:58 - 10:09] So we'll use dollar sign user.school name. And so this will display the school name here at the top of the lunch menu admin page.
[10:10 - 10:30] So with our hot loading dev environment, save that file, it automatically refreshes the app with the changes and we can see test school here. That's it for this lesson.
[10:31 - 10:40] We created an admin layout and we covered several important Svelte concepts. So we're using the admin layout to initialize our state.
[10:41 - 10:52] Later on, we're going to use it to handle authentication and initialize the actual user from Auth0. So we're just kind of laying the groundwork for that now.
[10:53 - 11:14] In our admin layout, we used a Svelte store and so we wrote that user object to the store and then we can use it in any other component by importing the store and then using dollar store name to refer to it. And we use the on mount lifecycle function to do that initialization.
[11:15 - 11:34] And then lastly, we used conditional rendering to make sure that the initialization was complete before rendering down the chain to the route that was asked for. The app doesn't look like too much yet, but it's set up with a basic architecture to support various design and admin functions as we move forward.