Optimize React Native with FlatLists and Native Webview
This lesson preview is part of the Building React Native Apps for Mac course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Building React Native Apps for Mac, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
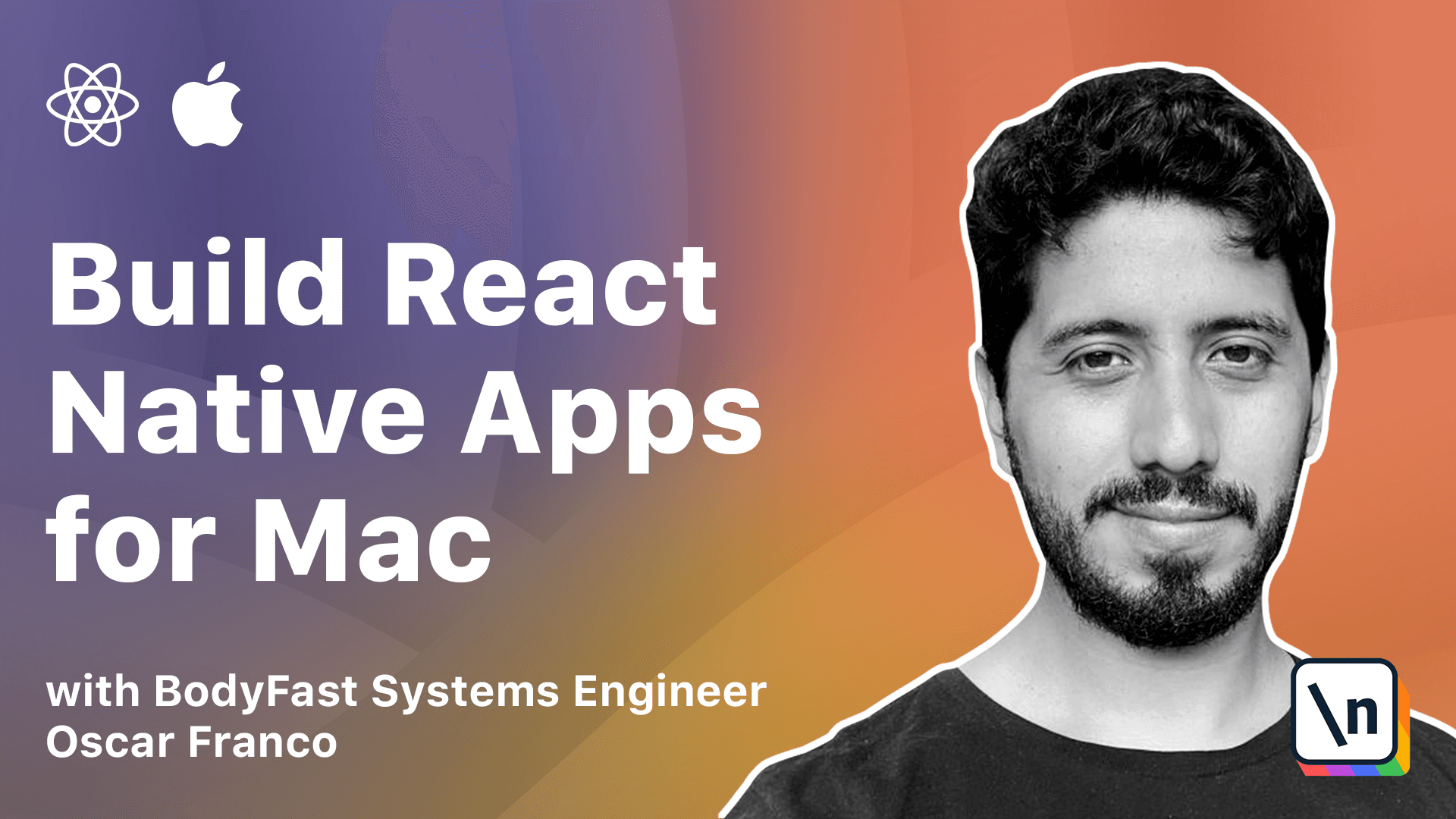
[00:00 - 00:20] We have seen a lot of the basic components of React Native so far. In theory you can just jump in and start creating your own application, but there are of course a lot more advanced components with different APIs.
[00:21 - 00:33] I would like to take a look into two specific components that are going to be very useful as you go down the line and start creating your application. One of them is the flat list component.
[00:34 - 00:48] So far whenever we had to display a list of items we have just placed them in their UI and iterated through them. But this doesn't really work as long as you have a very long list of items.
[00:49 - 00:58] Once you start rendering 100, 200 or maybe 1000 elements then you cannot render all of them at the same time. Your application is not going to work.
[00:59 - 01:13] So in order to solve this React Native provides what's called a flat list. So let's just jump right into the code and we will go exploring the possibilities of the flat list as we move along.
[01:14 - 01:24] So first I'm going to import it from React Native and I'm just going to put it inside the component. So flat list takes a lot of properties.
[01:25 - 01:30] We're just going to create a basic example for now. So one of the required properties is the data.
[01:31 - 01:43] Right this is the array of items the flat list is going to iterate upon. Another component, another property you need to pass is the render item function.
[01:44 - 01:55] So per each element of the array the render item function is going to be involved with that element. So I'm just going to create a placeholder for now.
[01:56 - 02:04] We're going to explore it in a little bit. Then we need to pass a key extractor function.
[02:05 - 02:16] This is going to take the element that will be rendered and we just need to return a unique key. We'll just use the title.
[02:17 - 02:30] One very useful property is the style that's going to be applied to the list. And if you have noticed so far our application doesn't really scale as it grows , we're going to take care of that now.
[02:31 - 02:40] So we're going to pass a flex one property which means it's going to expand. It's going to take as much space as possible without pushing or without messing with other elements.
[02:41 - 02:49] But that also means that we need to also make our container expand to take as much of the view as possible. So that's quite easy to do.
[02:50 - 03:01] We just use flex on both of them. So now that we have that working now is the time to create our function or render book function.
[03:02 - 03:11] So this function is going to be passed an object and inside of the object is going to be an item property. And that's going to be each of the books.
[03:12 - 03:31] It's going to be a book proper from our array. So we're going to type this and let me just see what name is.
[03:32 - 03:44] So the name of the property we're looking for is list render item info. And we're going to use our iBook type.
[03:45 - 03:57] So we need to import our iBook type from our stores. Are you ready to care of this in our UI store?
[03:58 - 04:07] I'm exporting the type or the interface that we have created before. So I couldn't hear it just imported.
[04:08 - 04:18] So this is just going to return that. So now you see the layout has shifted a little bit, but the list is empty because I'm not returning anything yet.
[04:19 - 04:33] So inside of my render book function, I'm just going to take more or less the same contents that I had before. I'm just going to return that as a final element.
[04:34 - 04:48] And instead of book, I have my item, but actually I don't need the key anymore because now it's going to be extracted. It's abstracted away because the flat list is a redirection.
[04:49 - 04:52] And we have that. And there you go.
[04:53 - 04:55] So now you will see the differences. I can actually scroll this.
[04:56 - 05:01] I'm not scrolling the entire interface. I'm just scrolling this part of the UI.
[05:02 - 05:13] And in here, now I could have thousands of books. This doesn't mean that if each of one of the elements is quite heavy to render, it's going to make it faster.
[05:14 - 05:24] It's just not going to render a thousand of them at the same time. It's going to render whatever is visible, maybe a little bit more down or upward so that when the user scroll still feels smooth.
[05:25 - 05:33] But it's going to improve the performance of our component quite a bit. So that's very useful.
[05:34 - 05:39] That's one of the components you want to use. Sometimes you might want to use just a scroll view, right?
[05:40 - 06:05] If you don't have a dynamic list of elements and you just want the UI completely to scroll, what you want to use is a scroll view, which is just as the name says, it's just a scroll view. It doesn't have any virtualization, but it allows your entire, all the stuff that you put in there is going to now be scrollable.
[06:06 - 06:14] Great. Now, let's just take a look at another component, which is a different type of component.
[06:15 - 06:26] This is a dependency. So we're going to add React Native WebView.
[06:27 - 06:32] This is a native package. It has a native component.
[06:33 - 06:51] So we're going to do a poly install. And we're going to rebuild our application.
[06:52 - 07:07] Great. So now that we have that working, let me just, just to be sure, I'll just close it and start it again.
[07:08 - 07:28] So now that we have our WebView component, what we can do is we can just use it inside the four components. So I'm going to go to my book container and I'm going to import WebView from React Native WebView.
[07:29 - 07:51] And now to make our application a little bit more interesting, instead of just displaying the title of the book that was passed into the component, I'm actually going to mount an embedded WebView. Now the WebView takes a source object and within the source object I need a URI .
[07:52 - 08:14] So I'm just going to take, I'm going to do a Google search just to display something interesting to the user. If somebody is taking a look into our favorite books or maybe me myself and I want to get some news or whatever, I can just do a Google search.
[08:15 - 08:22] So I'm just going to save that and now let's see what happens. If I, wait, I made a mistake.
[08:23 - 08:25] This is supposed to go like this. Great.
[08:26 - 08:34] So now if I go into Lord of the Rings, we are right. I need to accept the Google terms and conditions, but it doesn't matter.
[08:35 - 08:41] There it is, right? So this, the WebView component is fairly easy, right?
[08:42 - 08:51] It just mounts a small WebView inside of our, your application. But like this, there are many components which also have a UI element.
[08:52 - 09:08] There are camera components, there are SVG components, there are more image mounting components and so on and so forth. So you can just search for packages and import them and if they have a UI part, you can just display them within your application.
[09:09 - 09:20] One important thing to know here is that not all of them support macOS at the time. All of them support iOS and Android.
[09:21 - 09:35] So you might have to check if the specific component you're looking for actually can run on macOS machines. But I think that's it for the basic part of our JavaScript.
[09:36 - 09:45] Oh, I feel... Yeah that's it, I'll look.