How to Use and Customize Tailwind CSS in React Native
This lesson preview is part of the Building React Native Apps for Mac course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Building React Native Apps for Mac, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
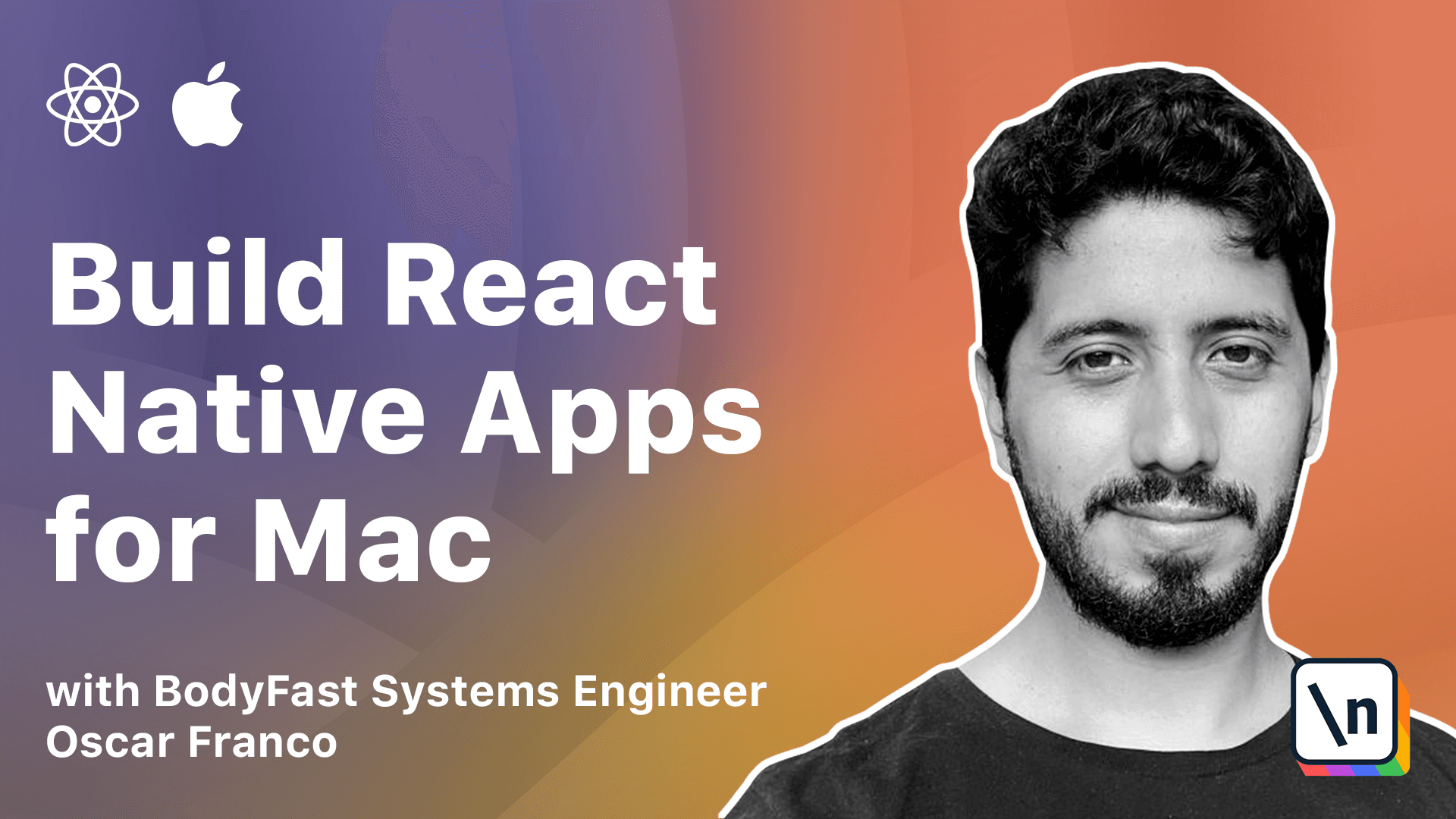
[00:00 - 00:19] In the previous lesson, we started customizing the UI of our application. We didn't use any custom colors or grids, we only used the integrated classes from Tailwind, but now we will exactly explore how to add our own colors and our own spacings and our own fonts to it.
[00:20 - 00:46] So the first thing that I'm going to do is on my route of the project, I'm going to create a new file and this is going to be called tailwind.config.js. So basically Tailwind is going to read this file and all of its configurations and it will generate a set of styles that we can import into our application.
[00:47 - 01:05] So basically here on the theme section, I have a extent key, which means I'm not going to replace the base colors that Tailwind offers, but I'm just going to extend them. So here I have the colors key and I'm now adding two more colors, one cyan and tangerine.
[01:06 - 01:17] So basically you can extend a lot of different properties of Tailwind. I would recommend that you go over the documentation, the official website, so you can have a better idea what's possible and what's not.
[01:18 - 01:38] There's also plugins available for Tailwind that extend its functionality, but bear in mind, we're not using pure Tailwind because we're using our React Native port, so maybe not everything is going to work out of the box. It's something that you might have to try in your own.
[01:39 - 01:55] So I actually took this from the lessons in the course, but there's one other command that you could do in order to initialize a blank template, which is NPX, Tail wind CSS in it, right? So it will just create this file for you, but since we already have it, we don 't need it.
[01:56 - 02:09] So now that we have our base configuration file, we can actually generate, or we can tell Tailwind to generate all the styling for us. So it will generate the JSON file, which we will be able to import into our application.
[02:10 - 02:33] So in order to do this, we're going to do NPX create tailwind RN. So you will see now we have a style.json file, and these are all the styles that will contain our changes as well.
[02:34 - 02:36] So how do we take care of this? How do we make it work?
[02:37 - 02:54] So I'm going to go to my source folder, and I'm going to create a tailwind.ts file. So one more time, I'm just going to take the contents of the lesson.
[02:55 - 03:09] And basically, I have a create function, which I'm going to import from tail wind RN from our port of tailwind. Then I'm going to import the JSON file that was created for me.
[03:10 - 03:17] And I'm just going to pass the styles into the create function. So this create function is going to return an object with some properties.
[03:18 - 03:33] The ones that are important are tailwind itself, which is equivalent to the function that we have been using so far to style our component and get color. This is particularly useful if you have any other component that doesn't work with tailwind, but you need to match the colors.
[03:34 - 03:46] So instead of hard coding your color, again, you can just call this function. So now that we have these two, we're just going to export them, and we can use them from our different components.
[03:47 - 03:55] So in order just to give you an example, I already have the app open. From the last lesson, I only move the elements a little bit, so it looks a little bit better.
[03:56 - 04:08] So I'm just going to go back into my component one more time. And this time, instead of importing TW directly from the tailwind package, I am just going to import it from our package.
[04:09 - 04:16] And now this is a named import. So I'm just going to change, let's say, for example, our button.
[04:17 - 04:24] So instead of saying background bloom 500, since I added the color, there you go. That's nice.
[04:25 - 04:31] You will also add the Tangerine. So basically, that's how you create your own colors for your application.
[04:32 - 04:45] You can create your own pairings, your own grid, so you can style the elements. You can create a bunch of different properties, font sizes, animations.
[04:46 - 04:58] I think animations right now are not working with tailwind with this port, but maybe in the future, you should also be able to do that every single detail of tailwind. You can just customize it at once.
[04:59 - 05:04] Great. So that kind of takes care of custom colors.
[05:05 - 05:20] Now there is one more topic about colors that we need to take care of. So let's say, for example, right now I'm using the white theme of my Mac, and let's say I switched to the dark theme.
[05:21 - 05:34] So here it works kind of nicely, mostly because the text I didn't style, I didn 't add any style into my text, and it works occasionally. But sometimes you will see these kind of things, right?
[05:35 - 05:45] Like the placeholder here disappeared. Or if I say, add my favorite books, I actually give it a color, and I said, you know, this is a text created 100.
[05:46 - 05:53] And it doesn't change automatically with my OS setting. And this is kind of not very nice, right?
[05:54 - 06:02] Because nowadays a lot of people, they use the theming options on their phones and also on their computers. So you kind of want your app to work everywhere.
[06:03 - 06:09] So we need to add some sort of dynamic theming. We could do it on application start.
[06:10 - 06:26] That does, let's say, you know, the application starts and then we query for the OS and then we see which theme is the user running at the moment, and we kind of stick to that. But a lot of the applications nowadays, you know, they try to be as smooth as possible.
[06:27 - 06:38] In this regard, React Native macOS has some way of dealing with this, but it's not properly documented. And it also doesn't play very nicely with tailwind itself.
[06:39 - 06:49] So we're going to go with a different approach and we're going to do it via a dynamic hook. So my source folder, I'm going to create a new folder.
[06:50 - 06:57] I'm going to call it leaps. And inside of it, I'm going to create a file that's called hooks.
[06:58 - 07:08] I'm just going to take one more time, the code from the lesson. And basically, there is this function that is provided by React Native itself.
[07:09 - 07:20] It's called use color scheme, which is a hook. So basically, you can put this inside of your component and it's going to return to you the current theme that the OS is running with.
[07:21 - 07:34] So we're going to take advantage of that and we're going to create our very first custom hook. Our hook is just a function that returns another function and internally uses the use color scheme function.
[07:35 - 07:48] So it's going to take two values. The first value is going to be the dark theme value and the second value is going to be the light theme value independent on which team we're using, which is going to return either of them.
[07:49 - 07:57] Great. So I'm just going to follow our structure to support our modules.
[07:58 - 08:07] And now I'm going to use it here. It was use dynamic color.
[08:08 - 08:21] So I'm just going to import it and dynamic color. Let's do something like this.
[08:22 - 08:31] So I'm going to create a dynamic color for, let's say, our button. Yeah, our button looks fine.
[08:32 - 08:36] Yeah, let's start with that. Let's say, bottom color.
[08:37 - 08:48] So my dynamic theme, I'm just going to give it a value. So let's say, when it's on the dark, I like the orange or tangent color.
[08:49 - 09:00] And on the light theme, I want our cyan color. And on my button, I'm going to replace my class.
[09:01 - 09:04] So here's what I meant. It works nice with tailwing classes, right?
[09:05 - 09:16] Because basically we need to compile or we need to provide everything as strings. We can just use string template, which is just concatenating strings, just a JavaScript feature.
[09:17 - 09:23] And now if we save this, let's see if we get any error. Yeah, right.
[09:24 - 09:39] So because react native hot swaps, the code, your code at runtime, sometimes you might get into these small errors with hooks, right? Hooks are also some sort of global state, right?
[09:40 - 09:54] So what happens if you render your component the first time and then on the next render cycle, there is one less hook or one more hook, then react. Cannot know what happened to that state, to that piece of state.
[09:55 - 09:59] And that kind of produced this error. So this is not not a major thing.
[10:00 - 10:04] You can just dismiss it. And if we reload the wrap, then we should be good to go.
[10:05 - 10:06] Yes. Right.
[10:07 - 10:16] So now if I switch one second, if I switch my OS, then I switch my OS. The theme.
[10:17 - 10:20] Okay. Probably small, small debug.
[10:21 - 10:40] That's how you customize. That's the mechanism that we're going to use to customize colors within our app .
[10:41 - 10:45] yeah