Beginner's Guide to React useContext Hook
Learn about the useContext Hook, its use cases and implementation.
This lesson preview is part of the Beginner's Guide to Real World React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Beginner's Guide to Real World React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
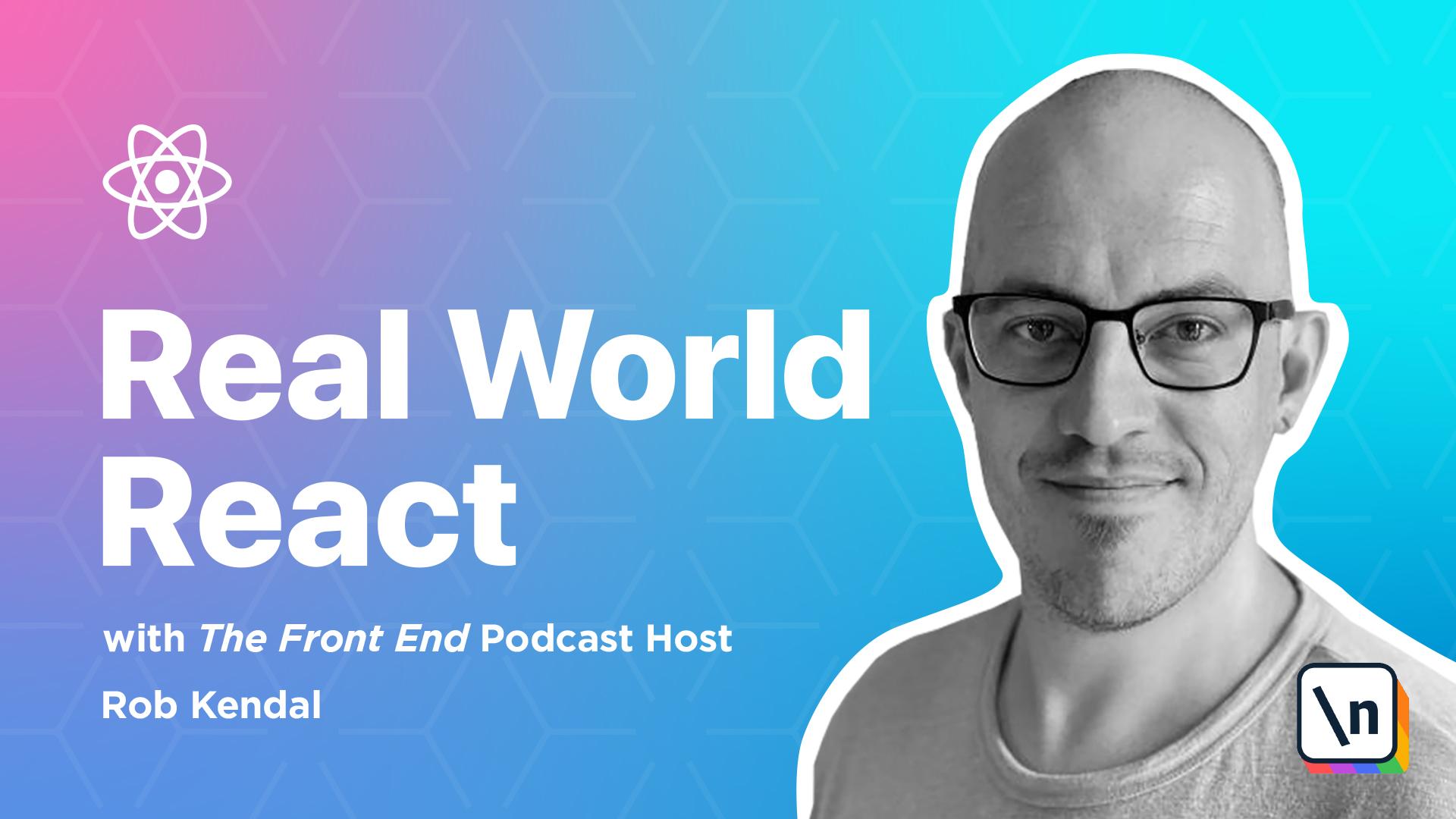
[00:00 - 00:11] Lesson 3. The Use Context Hook The first hook we'll explore in this module full of advanced hooks is Use Context. Context in React is defined very clearly in the official React documentation.
[00:12 - 00:28] Context is designed to share data that can be considered global for a tree of React components, such as the current authenticated user, theme, or preferred language. By making various items of data available in a global sense, context solves a familiar problem that we saw in the first lesson of the previous module, Proptralin.
[00:29 - 00:46] Proptralin is the process of passing data down through multiple layers of child components, and it often looks like this. So you can see we have the main app component receives a user object, and then it passes it down to the header component, which passes it down to the navigation, which in turn passes it onto the avatar and so on down the tree.
[00:47 - 00:59] In this really contrived and oversimplified example, it's not much to look at, but you can already start to see how this could get messy if we had a number of different items of data to pass through multiple child components. Also, this practice creates a lot of redundancy.
[01:00 - 01:09] In essence, a lot of these parent components become the middleman for the user object, then merely pass it on down the component tree. With context, we can create a context provider to wrap our app component in.
[01:10 - 01:18] This provider would then carry our user data across the components until we hit the avatar component. At this point, we grab the context using a consumer wrapper.
[01:19 - 01:27] This non-hook version of React context looks like this. Straight forward enough, but it looks a little clunky.
[01:28 - 01:35] Things only get worse when you start to use multiple slices of context. You have to wrap providers inside providers and do the same at the consumer end .
[01:36 - 01:44] The use context hook offers a much more efficient experience when dealing with context. Much of the way you define a context object is the same when using use context.
[01:45 - 01:49] This holds true for the provider wrapper too. The main difference is how you consume the context.
[01:50 - 01:55] That's where use context comes in. Let's walk through the steps needed to create and consume context in React.
[01:56 - 02:03] The first step is to define your context object. This is the default value for your context, and it can be anything you like.
[02:04 - 02:12] A primitive value, a function, an object, you name it. Next, you'll want to wrap whatever your top-most component happens to be in a context provider.
[02:13 - 02:21] The top-most component might be your app's entry point. It might be a specific area within your app that contains a specific group of components, such as an account management area.
[02:22 - 02:30] Next, you'll want to consume the context with use context. To grab the context and use whatever value it contains, you implement the use context hook like this.
[02:31 - 02:42] If for whatever reason you needed to access multiple contexts from within a component, the use context hook makes it really easy to do so. You simply call use context as many times as you need, one for each context object that you want to consume.
[02:43 - 02:47] Like this. Looks nice and clear and clean, doesn't it?
[02:48 - 02:59] No more wrapping consumers within consumers to access multiple context objects. Note that the use context hook is awesome, but it still requires the use of a provider further up the tree to provide the context that it consumes.
[03:00 - 03:06] So when should you use context? Context is a great fit for some use cases, theming being one of them.
[03:07 - 03:19] Its primary purpose is to provide a means to share data between many components within a nested structure. However, its use can be abused and you may be tempted to use context when some component refactoring, or component composition changes could achieve the same result.
[03:20 - 03:39] Using context is fine, but it can make components less reusable as they're more inherently tied to their context providing parents higher up the component chain. The official React documentation on context has a great example on using component composition, i.e. how you create your components, over using context, and I'd recommend checking those out.
[03:40 - 03:54] Context also offers a simple alternative to something like Redux. You want to study Redux in detail in an upcoming module, but for now just know that whilst more complex in its implementation, Redux essentially offers the same end result of providing access to a more broad idea of state.
[03:55 - 04:08] It's also important to note at this point that context is not very suitable for two-way communication between components. You can facilitate data back upstream by passing event handles and functions down via context, and you can see an example of this very thing in the official context docs.
[04:09 - 04:17] But its primary purpose is to provide access to a global state data across portions of your app without the need for prop drilling. This is generally a one-way street.
[04:18 - 04:33] Now that we have a better understanding of React's context and how to use the use context hook, let's build a simple example that uses context to change themes. Open up your project, we set up in the previous lesson and open the styles files, styles.css.
[04:34 - 04:41] So far it only has some basic general styles in here. We're going to add a few more for the use context example component just to illustrate the theming idea.
[04:42 - 04:56] Add these styles to the bottom of the file. We're going to be creating a layout container that houses a sidebar with some navigation and a main article element that holds some dummy content.
[04:57 - 05:08] The styles here add a little bit of background color to the sidebar to distinguish it, as well as adding a little bit of padding and spacing, and as well as setting the display type as flex on the container. We'll see why this is important in a moment.
[05:09 - 05:22] This is a familiar layout job that you'll see on just about every website you visit. The difference here is that we're going to control both the position of the sidebar and the type of theme, i.e. light or dark, that's applied to the content, all with your friend of mine, use context.
[05:23 - 05:28] With the styles taken care of, open the example component, use content example. jsx.
[05:29 - 05:45] As usual, we'll start with our import section, importing React, use content and create context. You don't have to import create context, you can just write react.createContext as we did in the earlier examples, but it's a nice idea I'm implying here as it's an alternative option.
[05:46 - 06:00] Next, we're going to define a couple of plain JavaScript objects, one for some styles and another for some layout information. You can see with the styles object we have light and dark properties filled, and a couple of familiar CSS key value pairs.
[06:01 - 06:15] The light one having a light background with dark text and the dark one vice versa. The layout object offers a couple more CSS values, but these will translate to flex direction values when we inject them into our layout container JSX in a little while.
[06:16 - 06:33] Next, we're going to define our initial context object and default values. We'll name our variable theme context as it is the convention for context objects, i.e. name of your context, then context.
[06:34 - 06:42] Then we have react create context function passing in some defaults. In our case, we're passing in an object with the light styles and the left direction for the layout.
[06:43 - 06:51] Now for the start of the show, the main component that will consume our context . We'll worry about providing the context in the default exported component later on.
[06:52 - 07:03] For now, however, we'll define a skeleton component called layout. The first thing we're going to do here is consume the context using the use context hook.
[07:04 - 07:20] That looks like this. We call the use context hook, just like in the mini examples from earlier in this lesson, and we pass it the theme context object we created earlier.
[07:21 - 07:37] The theme variable will now have access to whatever was passed into the context provider, or if that isn't available, whatever was passed to the context object as a default. In our case, we haven't defined the context provider yet, but we'll have that passed in the default values, so our theme variable will look like this.
[07:38 - 07:58] Next, it's time for some JSX. Everything within the section element, which is our layout container, is pretty standard HTML.
[07:59 - 08:09] We have a sidebar with a nav element and some dummy links. Inside the article element, we have a title and some good old fashioned lorem ipsen placeholder text.
[08:10 - 08:18] In the section element, however, is where we're consuming our context data. Using React inline style attribute, the one that looks like style and then a set of curly braces.
[08:19 - 08:34] We're setting background color, color and flex direction CSS properties. The values for those properties are being provided from the theme context we've pulled in using the use context hook.
[08:35 - 08:47] Pretty neat, and very little effort. Okay, we've defined some context and consumed it in the layout component, but the use state hook won't do a thing unless we provide the context to the components looking to consume it.
[08:48 - 09:15] For our default export, we'll create a super simple inline function that returns our provider wrapper and the layout component as a child. To provide context to child components, we'll also wrap them in a provider component which takes the form of context object dot provider.
[09:16 - 09:30] Since our context object was created as theme context, our provider wrapper component looks like this. ThemeContext.provider. The value attribute is where we pass whatever values we want into the context object to be consumed later.
[09:31 - 09:41] In our case, we're passing the value attribute and object, but it could be a primitive value such as an integer or a string. Finally, inside our provider, we add the layout component.
[09:42 - 10:01] Just as with any other component, however, we could add more child components in here if we wanted and each would have access to the context values using the use context hook. So with a heavy lifting done, head over to the app.js file and make sure all the other components that are imparted are commented out, except for the use context example component.
[10:02 - 10:15] Ideally, at this point, your app component should look like this. Update and save the file if needed and open your terminal window. Type the good old yarnstart command and view the result in the browser.
[10:16 - 10:33] So you should see a dark text on white background theme with the sidebar on the left. Let's change things up and alter the theme details that the default component uses.
[10:34 - 10:56] Back in the use context example, right at the bottom of the file where we're exporting a default component, change the contents of the value attribute passed to the theme context dot provider wrapper to styles dark value for the color scheme and layout dot right for the direction. Back in the browser, refresh the page if it hasn't hot reloaded for you already and take a look.
[10:57 - 11:08] Well, look at that. We've got a light text on a dark background and the sidebar is now on the right hand side and all we've done is update two values. And that's all there is to the use context hook and indeed reacts context in general.
[11:09 - 11:19] It's a fairly simple yet elegant based. I'd encourage you to have a play around with this example and see what else you can pass around using the context mechanism. I'll try providing and consuming multiple contexts.
[11:20 - 11:22] Next up, we'll be looking at the use ref hook.