What is React Router? A Beginner's Guide
React Router is a routing and navigation library that bolts onto to React. We'll explore what it is and how it works.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Beginner's Guide to Real World React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Beginner's Guide to Real World React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
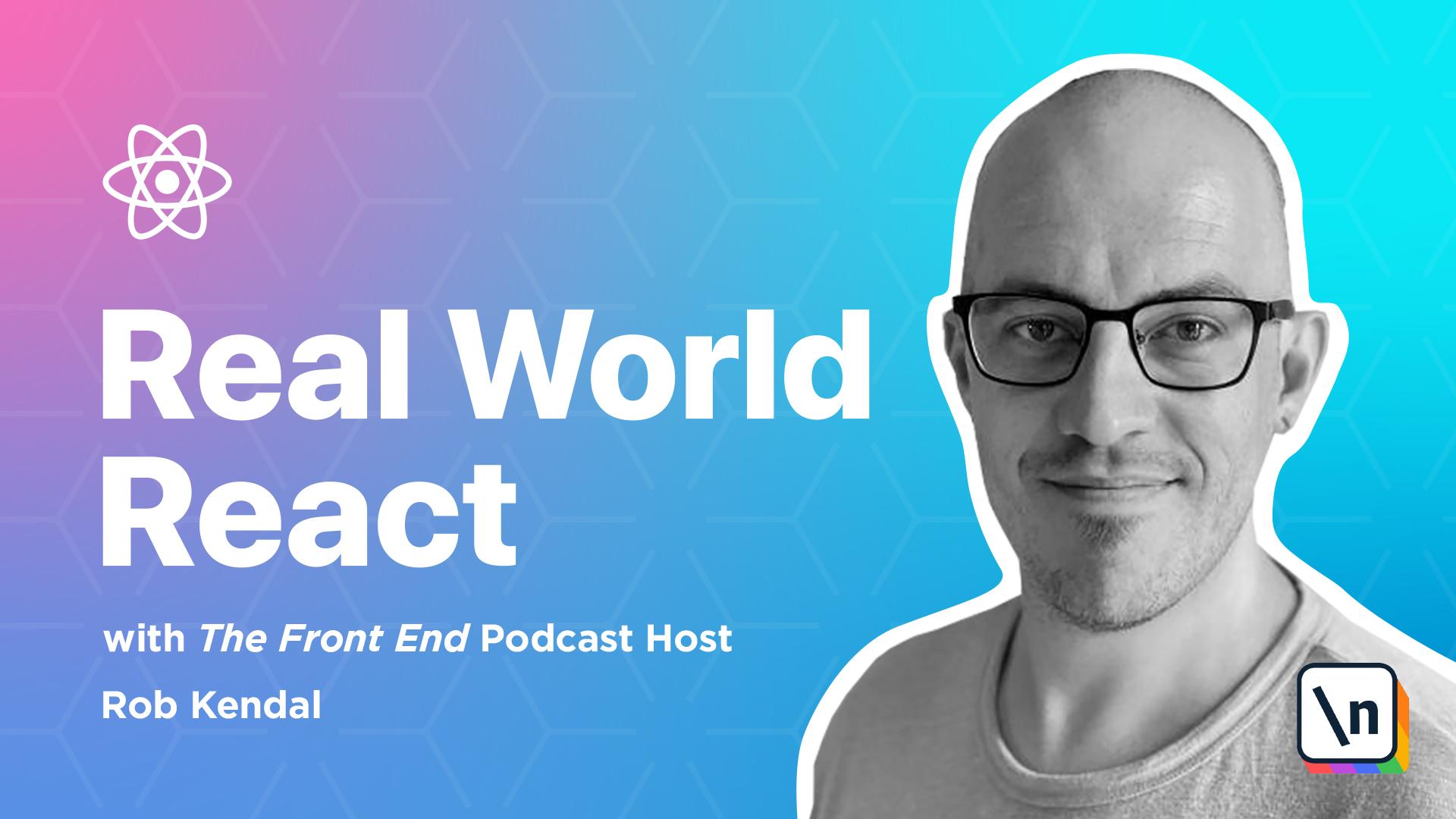
[00:00 - 00:17] Lesson 2 - Introducing React Router React Router is generally the go-to choice for devs looking to implement a solid navigation system into React. It's an open-source product of the React training team which features some impressive and well-known figures in the developer world, including Ryan Florence, Brad Westfall and Cassidy Williams.
[00:18 - 00:32] The React Router library is but one of several choices out there, and I'd always encourage you to review your options and make sure that you make the right choice for you and your project, but arguably very few of them are as well battle-tested and as easy to implement. The React Router has been around for some time.
[00:33 - 00:47] It's very robust and proven to work well in just about any project scenario, playing well with different project types, getting out of your way and allowing for a very flexible implementation. What's more, it has excellent developer docs and I'd recommend you explore them as soon as we're done with our upcoming demo.
[00:48 - 00:59] But when should you use React Router? Well, as we've already seen in the previous lesson, as soon as your app starts to grow in even moderate size and complexity, swapping out components using some sort of state value just isn't going to cut it.
[01:00 - 01:34] With React Router, we get access to a superb routing system that primarily relies on native HTML history API to provide access to browser history, as well as a host of other convenient and helpful features. Features include in Location History, Hash routing, to enable navigation by URLs contained in hashtags, integration with Redux, which we'll explore in a later module, static routes, parameterised URL routing for URLs such as User, User ID, where the user ID pie is dynamic and unknown, non-matching or 4/4 route handling, and support for complex layered navigation such as in sub areas and layouts.
[01:35 - 01:51] You may never need to use many of the features that React Router offers, but it does care to have any routing scenario you can throw at it. Getting to know the far ends of React Router is a course in and of itself, so for now we'll be focusing on the main elements required to employ React Router in your app and getting to know the major elements that make it work.
[01:52 - 02:12] Note, as wonderful as React Router is, for simple projects are those that don't require complex routing or features such as dynamic URL parameters, you may not need anything more than React offers you out of the box. It can be tempting to want to use something when there's little cars too, so assess your projects needs before you add a helpful library such as React Router, you may not need to add the additional complexity or code weight.
[02:13 - 02:28] Using React Router In order to use React Router, we first need to install it into the project, which we'll do in the upcoming lessons as we build our demo, and then for basic use we'll be concerned with three main elements. A browser router, the link or nav link, and route.
[02:29 - 02:41] For each player vital part in the routing landscape, but let's briefly walk through each one to see how it works and what role it occupies. The browser router component, often imported as just router, as shown here, is the main parent of the routing system.
[02:42 - 02:51] It doesn't have many additional options or attributes, and the few it does have you likely rarely use. The most commonly found the router or browser router element wrapped around the main application entry point.
[02:52 - 03:08] For example, in our demos thus far, we'd have wrapped a router component around either the app component in our index.js file, or directly in the app.js file itself. Either way, you can usually find this at the topmost level of your app, so that any child components down the tree have access to the routing system.
[03:09 - 03:21] The link and nav link components replace the more common anchor elements within our JSX, ostensibly offering the same functionality, i.e. you click on them and they route you to another URL. In that sense, they're fairly simple and straightforward.
[03:22 - 03:32] Anywhere you wish to place a link that a user can click on to be transported to another location, you'll use the link component. It accepts a number of attributes, but the primary one to be concerned with for now is the two attribute.
[03:33 - 03:42] You can pass it as simple string value as we have here. So if the user clicks a link with a 'bout us' on it, the links to attribute will navigate the routing system over to the /about us URL.
[03:43 - 03:52] The two attribute isn't limited to just strings though. You can pass it an object containing other root properties such as search or hash values, as well as a function that can determine the destination URL dynamically.
[03:53 - 04:05] You really have a lot of flexibility to define links to various parts of your app as you wish. The main difference with the nav link component is that it provides an active class name and adds styling attributes to the rendered element when it matches the current URL.
[04:06 - 04:15] This is very handy for typical top-level navigation you may find in a page header. The root component is arguably the most important component you use as part of React Router.
[04:16 - 04:21] It's responsible for matching a given root and rendering a specific component. Here it is in its simplest form.
[04:22 - 04:30] We take the root component and wrap it around whichever component we want to render. We provide a path attribute of the URL we want to match, and we're also supplying the optional exact attribute.
[04:31 - 04:50] So if we use an navigate to the URL /fault/ which is usually the home page, this root component will be matched and the component to render component will be displayed. Because we've included the exact attribute, we can be sure that the root that is matched will be exactly / and not another root containing a /such as /about or /pager/1 .
[04:51 - 04:59] For more flexibility, AutoCater for more complex needs, the root component provides several additional attributes as you can see here. Let's talk about them so you can understand how they work.
[05:00 - 05:12] With path, this should contain the URL path that you wish to match against a given root. It can also handle root parameters, so for example, you can see here that we're aiming to match the /account URL that contains a call on ID parameter.
[05:13 - 05:24] So it would match URLs like /account/123 or /account/7, etc. With the exact attribute, this ensures that the path supplied to the path attribute is matched exactly.
[05:25 - 05:35] For component, if you don't wish to supply a child component into the root component, you can supply it via this attribute. This allows you to define inline root elements which can neaten up your code base.
[05:36 - 05:48] Behind the scenes, this uses React.createElement to create a new React element from the given component. And finally, with render, this allows for inline rendering and wrapping without undesired remounting that you will get with the component attribute.
[05:49 - 06:12] Each of the child rendering methods, i.e. a child component wrapped by a root component and the component and render attributes, passes a root props object along to them that contains match, location and history items. We'll look at and use some of these as we build our example routing app in the coming lessons, but for now, just know that if you need to perform some logic around the current location or history information, it'll be passed along to the rendered component by ReactRooter.
[06:13 - 06:18] With the basics under our belt, let's push on to the next lesson and take a look at what we'll be building to cement our knowledge.