How to Build an Event Signup App with React Redux Thunk
Learn how to build our very own Event Sign-Up App using the React Redux library with a centralized state management store.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Beginner's Guide to Real World React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Beginner's Guide to Real World React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
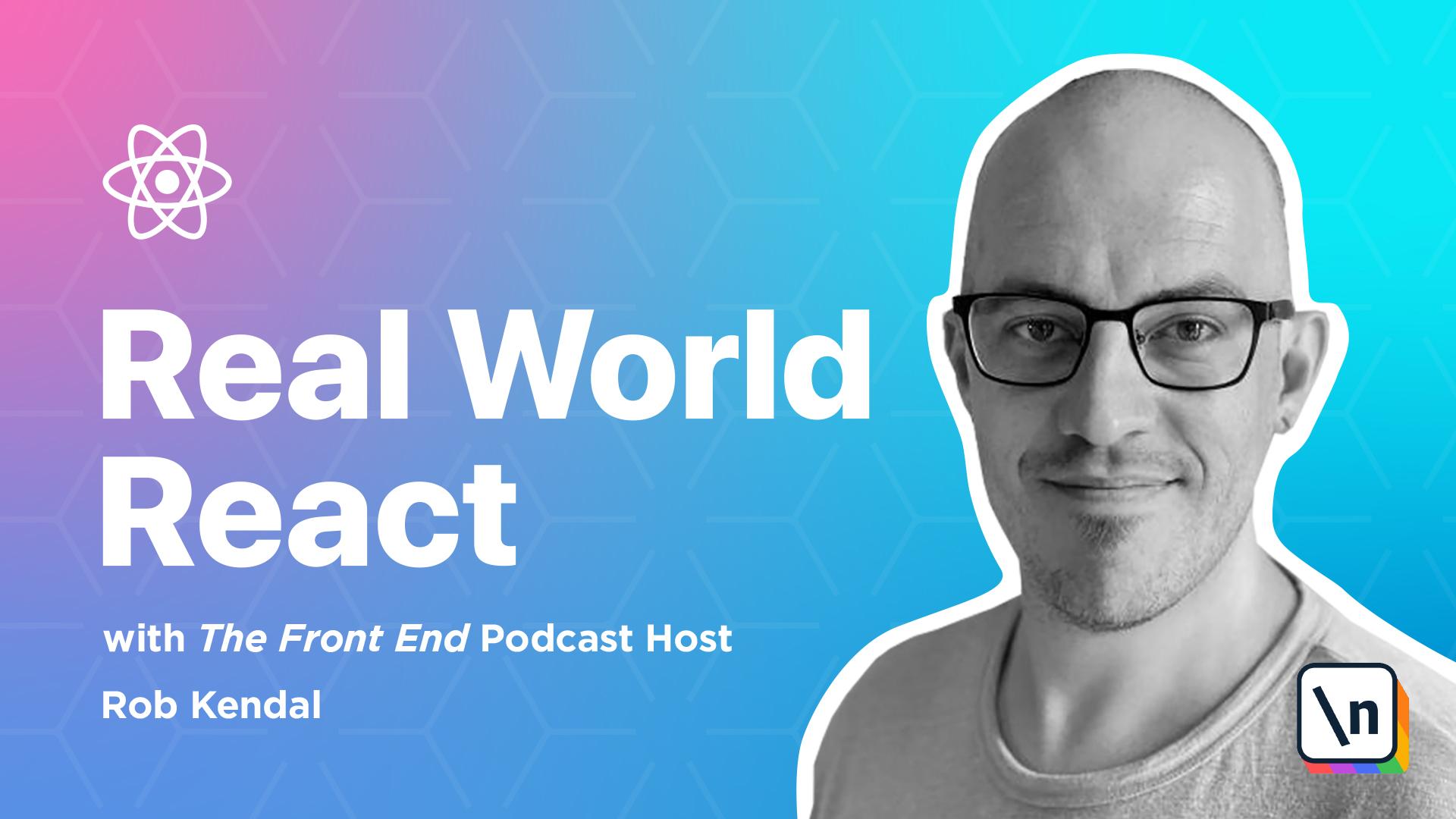
[00:00 - 00:11] Lesson 4, Building the Event Sign-Up App For the remaining three lessons in this module, we'll be building three approaches to using Redux. In this first lesson we're going to build, arguably the most complex of the three approaches.
[00:12 - 00:24] We'll be using the React Redux Library to help us before transitioning into the Redux Toolkit for the next lesson. Finally, we'll see how to implement the Redux pattern without any additional libraries using React, built-in, use, reduce the hook and the context system.
[00:25 - 00:32] First, let's get going with building the Event Sign-Up App and the Vanilla React Redux package. Onto the project setup.
[00:33 - 00:42] We're using Create React App for the remaining lessons and this process should be starting to feel familiar by now. Open up a terminal window to the parent folder you want to create the new project in and run the following command.
[00:43 - 01:00] Yarn, Create, React-App, Event-SignUp-Demo. Once the command finishes and our App base is installed, run it to test it setup correctly with CD, Event-SignUp-Demo and Yarn Start.
[01:01 - 01:10] View the running starter app in your browser at localhost port 3000. Just like in the previous lessons, let's clean up the starter app by doing the following.
[01:11 - 01:21] Open index.js and remove the reference to import index.css. Delete the index.css and app.css files.
[01:22 - 01:33] Open the main app.js file and empty the file so we can add our demo specific code later on. Before we create the files we need for the demo, let's bring in the required dependencies.
[01:34 - 01:41] The first dependency is to add our Redux, React Redux and the Redux THUNK. We'll explain this last one when we come to configure our Redux store.
[01:42 - 01:53] Back in a terminal window, make sure you're in the root project location and enter the following command. Yarn, Add, Redux, React-Redux and Redux- THUNK.
[01:54 - 02:04] With that done, we can access the various methods and helpers that the React Redux provides us. Since we'll be adding Event attendees to our fake event, it's good practice to give each of them a unique ID value.
[02:05 - 02:18] We could just increment the ID to the next highest value in the array of Event attendees, but to make it more realistic we'll assign a uniquely generated ID instead. To do that, let's impart the very helpful React UUID package with the following command.
[02:19 - 02:31] Yarn, Add, React-UUID. Next, we'll stick to the node version of Bulma for this demo, adding it to our project using the command, Yarn, Add, Bulma.
[02:32 - 02:41] Finally, we'll also add in node SAS to compile our .scss files for us. Bringing the file package as a dev-only dependency as follows.
[02:42 - 02:51] Yarn, Add, Dash-dev, Node-Sas. With all our dependencies added, let's create all the files we need for the project and build them out.
[02:52 - 02:58] Index.js. We'll need to make a scant few changes in here to wire up our Redux store and the rest of the application.
[02:59 - 03:17] App.js, the main starting point of our app, /assits/style.scss, will add in some basic styling niceties in the SCSS version of SAS. /components/event-details.jsx, a presentational component that will display details of an individual event.
[03:18 - 03:38] Components/events-signup-form.jsx, Bulma-styled-form-component, whose logic will enable us to add new attendees to the global star via Redux methods. Components/events-signup-list, the other side of the event-form coin, this component will allow administrators to edit attendees, remove them and see their details at a glance in a list format.
[03:39 - 03:56] Components header.jsx, another presentational component with scant logic that will act as a navbar and display a message to the user if they're logged in. Config/configure-store.js, a small but mighty JavaScript file that configures the Redux store at the heart of the Redux pattern.
[03:57 - 04:12] Data/initial-state.js, a starting point for the global state in our app. Reduces/event-reducer.js, this will hold all reducer functions, state-altering- magic, and actions for dealing with the event portion of our app's state.
[04:13 - 04:20] And finally, reducers/reducers.js. This will be the main reducer file that will associate with our store and app's Redux.
[04:21 - 04:29] Effectively, it acts as a container for the other more specific reducers such as the event reducer. With all the files created, let's work through them and add in the details needed.
[04:30 - 04:46] Open up assetsstyle.scss and copy in the following styles. The import statement at the top of the file brings the Bulma CSS framework styles into the project, and next we set a couple of SCSS variables and a couple of styles to bring a little nizity to our base app.
[04:47 - 05:01] Our app's state represents a central data storage facility that we can use to house any relevant data we need to share and use across our app. When we create our Redux star, we'll supply it with an initial data set that represents a starting point, the initial state of our state, as it were.
[05:02 - 05:16] In the data-initial-state.js file, that's where we're going to house such a starting point, so open it up and enter the following. There's very little here, just a plain vanilla JavaScript object that we're exporting to use elsewhere.
[05:17 - 05:31] You'll notice that we've created an event's property in the object that will hold the state data relevant to the event's section of the app. You can define your app's state however you like, but I prefer to have a single initial state file and split it into slices like this.
[05:32 - 05:37] Now let's tackle the reducers.js file. A Redux star can only deal with a single reducer.
[05:38 - 05:52] This presents a problem if we have a larger app working on different slices of the app's state with a very different reducer statements and actions and other moving Redux parts. If we were to only have a single reducer, you can imagine this would grow huge over time and become a maintenance nightmare for us developers.
[05:53 - 06:01] A much better approach would be to have multiple reducers that only deal with a distinct area of the app. However, we would then fall foul of the single reducer to a star rule.
[06:02 - 06:18] Fortunately for us, the React Redux library provides us with a handy function " combine reducers", which allows us to define our reducer files separately and combine them into a single master reducer of sorts that we can then pass into the reducer. That's what we'll be doing with the reducers.js file.
[06:19 - 06:28] First we'll import the "combine reducers" function from the Redux package. And then import the "event reducer" from the reducer's "event reducer.js" file.
[06:29 - 06:43] In a bigger app you might have more reducers, but we've only got one here. Finally, we'll export the result of the "combine reducers" function, which accepts an object of key-value pairs, each key being the reference name of the slice of state the reducer affects, each value being the reducer that affects the value of the reducer.
[06:44 - 06:56] In our case, they're one and the same thing, so we can use the shorthand "Java Script Object" properly notation. Now we come to the main event, even though we're only a couple of files into the demo and have few more to complete.
[06:57 - 07:10] However, logically it makes sense to work through the "event reducer" file now and cement the key concepts about reducers in place before creating and wiring up the other parts of the reducer's puzzle. Open up the reducer's "event reducer.js" file and let's begin with the "imports ".
[07:11 - 07:23] We're pulling in the "uuid library" in our initial state file here. The "uuid" package will be used to generate a unique ID value when we come to add a new event, "attendee" into state via the "upcoming reducer" function.
[07:24 - 07:31] Next, onto the action types. Action types are simple string constants that describe the type of action we want to perform against state.
[07:32 - 07:39] You don't have to do this or use action types at all, they're just a well-ad opted convention. If you choose not to use them, then you'll have to pass around string values here and there.
[07:40 - 07:48] This introduces a lot of potential for mistakes, such as spelling errors, and that's without considering that you might want to change one of those strings. In this case, we'll only have to change them in one place.
[07:49 - 07:58] Let's define some action types with the "event reducer" now. There we go, nothing but a simple JavaScript object whose properties describe the action we want to take.
[07:59 - 08:15] Actions are different from action types, which are simple describing strings. Actions are functions that return both the type of change that we want to perform against our app state, such as adding a new user, deleting a row or fetching a result, and a payload, an object containing the changes to state.
[08:16 - 08:35] For example, if we wanted to add a new user, we'd create an action that had a type of "add new user" and a payload that was a new user object. Again, you don't have to actually do this, however ultimately we're going to call a dispatch function, provided to us by the Redux library, which dispatches a notification to the reducer to make some changes to our app state.
[08:36 - 08:47] This dispatch function requires a type of action, as we've already created, and a payload. Without creating any of the action functions, we'd have to call each and every dispatch like this.
[08:48 - 09:01] As it stands, this might not look like much, but imagine that you have a bunch of these updates in the same component and across multiple areas of the app. It'll start to become cumbersome and bloated to keep doing this as time goes on , plus we're going back to the potential for errors and making it harder to make changes.
[09:02 - 09:14] If we wanted to change the payload here, we'd have to find every single instance of this and change it across multiple files. Compare this with having a single function call that has passed any data changes of parameters, like this.
[09:15 - 09:31] It's much easier and cleaner to define an export, a series of action functions in each relevant reducer file, that accepts one or more state data changes as parameters, and then return a nicely formatted action object. That's what we'll do now. We're going to create three action functions here.
[09:32 - 09:50] Add event attendee, for adding new event attendees, toggle event attendance, for toggling whether or not someone is coming to an event, and delete event attendee to remove anyone who's no longer needed from our event signup list. We're exporting each function so we can call it across our app in whichever component needs to use it.
[09:51 - 10:13] You'll see that there are pretty straightforward error functions. There's nothing specific to redox here, but we formatted the return type with a type property using one of the action types we defined earlier, and a payload, which contains any relevant data that needs to be used by the upcoming reducer to affect our state. The reducer is where all roads point to, and this is responsible for making the changes and updates instead.
[10:14 - 10:23] Remember, it's important to understand that app state must be immutable, that is, we do not change it. When we talk about changes or updates, it's mainly for conversational convenience.
[10:24 - 10:34] In reality, the whole redox setup is centered around making a new copy of state with changes applied to it. It's going to look a little unwieldy at first, but stick with it and you'll soon make sense of it as the pattern becomes very familiar.
[10:35 - 10:45] We'll also be looking at the redox toolkit in the next lesson, which reduces a lot of this initial complexity in writing reducers. Let's define the reducer function with just a single action handler in it.
[10:46 - 11:02] Again, the reducer is a standard arrow function that accepts a value for state and an action. These values will be passed to the reducer by the React Redox library, when everything is wired up, so the only thing we have to do at this stage is supply an initial value for state.
[11:03 - 11:14] In our case, this will be the events portion of the initial state object we imported earlier. The main body of the reducer will be a switch statement that checks the incoming action arguments type property for a match.
[11:15 - 11:26] When we find a match, in this case when action.type=actions@eventattendy, we can perform some updates to state. First, we pull out the ID and content values from the actions payload property via destructuring.
[11:27 - 11:36] Then, we need to return a new updated copy of state without changes applied. Notice that we're including a copy of existing state using the .dot.spread syntax.
[11:37 - 11:48] Also, keep in mind that this reducer will be acting upon the events portion of overall state. This is wired up via the Redux library, but it stems from our inclusion of the state equals initial state.events, when we define this reducer function.
[11:49 - 12:03] We want to update the event attendees properly and array, so we use the spread syntax again and add the new event attendee info from the content object. We'll add the ID value to and set a new property attending to true, because when someone signs up for the first time, we assume they're attending.
[12:04 - 12:18] Next, we'll sort out the code to handle the toggle event attendance and delete event attendee actions. OK, so things are starting to look a little messy, and that's a fair criticism that's leveled at Redux from time to time.
[12:19 - 12:29] However, you can start to see a similar pattern between these different action matches. For the toggle event attendance case, we pull out the ID, value, and use it to search for a matching event attendee using a red.map loop.
[12:30 - 12:41] When we find a match, we update the attending property to reverse. And finally, we return a new state copy once more with the updated event attend ee's variable assigned to the event attendee's state property.
[12:42 - 12:55] With delete event attendee, we're performing a very similar process to the one we've just done. Only this time, the updated event attendee's variable will be populated via a red.filter, removing the event attendee whose ID value matches the one we supplied to the reducer.
[12:56 - 13:08] Well done, you'll be pleased to know that we're done with the reducer file and it's all downhill from here as we'll build out the remaining files. With all the code in, the finished and complete event reducer JSL file looks like this.
[13:09 - 13:24] Open up the config configur.js file and fill out the following code. What we're doing in this file is stitching together our root reducer, initial state values, and what we call enhancers.
[13:25 - 13:40] Enhancers are higher order functions that enhance a redook store, adding in middleware, persistence capabilities or other additional capabilities that we may want our store to have. In our case, we're adding a very common middleware, readups thunk, which we pulled into our project in the dependencies section.
[13:41 - 13:53] The thunk middleware allows for asynchronous actions during readups operations. Granted, it's not something we're doing here in this demo, but you'll use this frequently, especially when dealing with API calls or other asynchronous mechanisms in larger projects out in the real world.
[13:54 - 14:05] So, we pull in our imports, the root reducer and the initial state. Next, we define an error function that creates a middleware enhancer by calling the apply middleware function and passing it the thunk middleware import.
[14:06 - 14:22] The composed enhancers is a little redundant here as we only have the one, but we call the compose function from readups and pass it the result of the middle ware enhancer variable. All that remains is to call the create star function and pass it our reducer, starting state and the composed enhancers variable, returning the star value to the call.
[14:23 - 14:38] You can find more information on applying middleware, the official readups documentation, especially in the glossary section. It might look a little alien at this point, but for now, just be aware that applying middleware to your readups stars is quite common in a lot of projects, and the process is usually the same.
[14:39 - 15:04] You define a star configuring function, within it, apply the middleware you need, compose an enhancer from the applied middleware, create a readups star that wires up your reducers, initial state enhancers, and then return the star for use. Our header component will be a straightforward website header with some navigation elements in it. Open up the components header.jsx file, after this, let's define the entire presentational component like this.
[15:05 - 15:18] We're destructuring three properties from props here. Its signed in will be a boolean value to determine if we're logged into the admin side of things. Handle sign-in click and handle sign-out click will be used to handle button clicks for signing in and I have it respectively.
[15:19 - 15:42] With the JSX we have a nav element styled up with Bulma classes. In the nav bar dash end section, we toggle the display of a welcome message for a signed in user, depending on the value of is signed in. Finally, we display either a signed in or sign out button depending on is signed in's value. Each button click event is attached to one of the click handlers we pulled from props, and this is fired up to the parent component on the actual button click.
[15:43 - 16:04] Over in the components event details.jsx file, we're going to create another purely presentational component as follows. We bring in React, and then we define a card element using Bulma's HTML structure and CSS classes, and in the JSX we're adding in a cute puppy placeholder image and adding in some fabricated event details, such as a title, name, or description.
[16:05 - 16:15] Save this file and let's move on. Now things are getting a little interesting. Open up the component event sign- up list.jsx file.
[16:16 - 16:24] This component will be the first one where we'll be using all of our hard work in setting up the various Redux elements. Let's start with the import.
[16:25 - 16:35] So, we've got React and this new connect function from the React Redux library. What connect allows us to do is quite literally connect components with both our Redux state and the dispatch mechanism.
[16:36 - 17:00] When using connect the common convention is to define our component and then create two other functions that map state and dispatch to the component, passing both those and the component itself into the connect function before exporting that as the files default export. After this, we're pulling in our action functions from our event reducer.js file, and we'll use these shortly to tell the Redux system what actions we'd like to perform against our app state.
[17:01 - 17:14] Following on with tradition, let's define the event sign-up list component body name. We're extracting event attendees and delete event attendee and toggle event attendance from props.
[17:15 - 17:26] These will be passed to the component later by the connect function. Event attendees is the state array of the currently attending sign-ups to the event whilst the remaining two are self-explanatory events that we defined in the reducer.
[17:27 - 17:41] Next, we have the Bulma box elements whose contents involve a short message highlighting the number of sign-ups followed by a regular old table element. Inside the table we map each event attendee to a table row, extracting various properties such as name, ID and phone number into table cells.
[17:42 - 17:50] The key point of note here is that we have two buttons, one to toggle attendance and one to remove this attendee from state. See how we're assigning the props functions to the buttons click handlers?
[17:51 - 18:05] Because of the action creating functions we made earlier in our event reducer, all we have to do here is pass the correct parameter values, in this case each item's ID. Without the action creator function we'd have to pass the action type and a payload object into this function.
[18:06 - 18:12] However, on their own these functions won't trigger anything in the Redux pipeline. To do that we need to wire them up to the Redux's dispatch.
[18:13 - 18:21] To do that we're going to move on to our Redux mappings. To wire our Redux system into this component we need to create two mapping items.
[18:22 - 18:36] So we have two items here, a map state to props function and a map dispatch to props object. The point of both of these items is to wire up the Redux state and dispatch mechanisms to our component.
[18:37 - 18:55] Starting with the mapped state to props, this is a function that will be passed our app state and should return an object that contains any items from state that our component needs. In this case we only want the event attendees array, but we could take anything we need from state and it will be passed our components props object under whatever property name you choose to give it.
[18:56 - 19:11] With map dispatch to props we create an object filled with properties that will be functions to have Redux's dispatch mechanism wired up to them automatically and pass the long to our components props. You don't have to do this but it provides a very declarative approach in keeping with React's overall principles.
[19:12 - 19:26] Without this approach we'd have to pass dispatch into the component and then call it directly passing in our action function. For example instead of calling toggleventattendenceitem.id we'd have to call dispatch toggleventattendenceitem.id.
[19:27 - 19:43] Another bonus with this approach is that it gives us an at a glance look at what's wired into the Redux system and provided to props without actually having to scan the component and work things out. With our two mapping items present and correct the last piece of the puzzle is to create our default export for the components.
[19:44 - 19:54] We call the connect function which accepts a state mapping function and then a dispatch connection object. The function returns a wrapper function that takes our component and wraps it with additional props we need.
[19:55 - 20:08] That's what we do after calling it, adding our event signup list component which completes the component code and why is it all up to the Redux style. With everything in place here's what the entire component should look like.
[20:09 - 20:20] Now on to the event signup form. Over in our event signup form component we're going to have a few more moving parts but you'll start to see a very familiar pattern emerging in how we use the Redux actions and mapping functions.
[20:21 - 20:27] Let's start with our imports. We're bringing in React and the connect function from React Redux.
[20:28 - 20:40] Next, just like in the event signup list component we're bringing in one of our action functions, in this case add event attendee. We'll be using this very shortly in order to dispatch an action to the Redux system telling it to add a new event signup.
[20:41 - 20:53] After that we're imparting a simple presentational component event details we defined earlier. Before we define the main body we first need to create a quick base form field object.
[20:54 - 21:06] This object will represent the default fields and values that we want to capture in our event signup form. We're going to use it here as a kind of reset object so that once the form is submitted we can put it back to its beginning state for another submission to be made with clean data.
[21:07 - 21:15] Now on to the event signup form component body. This is our skeleton component body.
[21:16 - 21:24] We've destructed props to grab the add event attendee action function that we passed to the component via Redux mapping function we'll define later. Just like in the previous component.
[21:25 - 21:33] Let's add in the variables and component functions now. We have two sets of variables and three functions.
[21:34 - 21:43] Starting with the variables we have two uses of the use state hook. The first signup complete holds a simple boolean value that we'll use to show or hide some part of the UI depending on the value set.
[21:44 - 21:51] With form fields this should look familiar from our forms in previous lessons. We use this to capture and edit any field values that are entered into the form by the user.
[21:52 - 22:01] With the functions the first one handle on change should look familiar from previous forms in other lessons. It will be assigned the unchanged event of each of our form fields to update the fields value instead.
[22:02 - 22:10] With handle form submit we're going to capture the form submission event. It cancels the default behavior first which will prevent unwanted page refres hes.
[22:11 - 22:25] Next we call our first Redux action function add event attendee. It will take care of the behind the scenes reduct work for us creating an action object with a type and a payload and calling the Redux dispatch function to eventually arrive at our event reducer where state will be updated.
[22:26 - 22:44] However all we have to worry about is passing an object to add event attendee and for that we'll be creating a new object using the spread syntax and the values from the form fields object instead. The function reset forms is exactly what it says in the tin. It updates the local component state values for form fields and sign up complete back to their starting points.
[22:45 - 22:56] Notice how we're using that base object values variable we created earlier, base form fields. With the logic out of the way we can define the components JSX.
[22:57 - 23:07] It looks like there's a lot going on here but that's always the case with forms and form HTML. This is doubly true when using a CSS framework. They always add a little more markup to achieve their styling aims and Bulma's no exception.
[23:08 - 23:20] We first have the event details presentational component that will display the details of the event to the user. Next we contain the entire form and development with a dot box class that will give it just a little border and some shadow styling.
[23:21 - 23:31] We check the sign up complete value instead and if it's false we then show the form. The form value itself holds fields for the name, email address and phone number of our event guest.
[23:32 - 23:42] Notice that the three inputs value and unchanged attributes are wired to their respective values in the components state and handle on change event. Also take note that we're not hooking up the forms default buttons or anything.
[23:43 - 23:50] You can see at the end of the form. By default generated HTML trigger forms submission event just by clicking this button without us having to wire up a click event.
[23:51 - 24:11] We do however connect the forms on submit event to the handle form submit we defined earlier. The last thing to take a look at is this final logic brackets here where we check the sign up complete value again and if it's true, i.e. the user is submitted a form, then we show a little thanks for submission style message and a button that triggers the reset form function to do exactly that.
[24:12 - 24:23] With everything done the last thing to take care of is wiring up the reduck style with its component via the mappings functions. The map dispatch to props function works exactly like it did in the event sign up list component.
[24:24 - 24:35] We pass it functions that we want connecting to the reduck dispatch mechanism and the connect function here does the magic for us. In our case we imported the add event attendee function and we want that wiring up so we add it here.
[24:36 - 24:47] Since we don't need access to any state values from the global star we're not going to define a map state to props function like we did in the last component . Instead we'll simply pass null to the connect function which we'll define now.
[24:48 - 25:08] Just like in the last component we call connect, passing it a null value for the state to props mapping and the map dispatch to props function and then we wrap it all in the event sign up form component before exporting the whole lot. Once we've done everything the entire component should look like this.
[25:09 - 25:19] We're on the home stretch now as we define our final component app. All of the complex reducks work happens in the files we've already built out so the remaining work in the app component will be relatively straightforward.
[25:20 - 25:28] Open the app.js file and let's do our imports. First up is react and use state shortly followed by our app styles.
[25:29 - 25:37] The last things to import here are the main components we've been busy defining up until this point. The app components body looks like this.
[25:38 - 25:53] Now authentication is a complex business and well outside the scope of this course so we're going to fake the sign in process with a simple boolean value. In this case user signed in will be used to hold a value in local component state to indicate if we've clicked the sign in button we created in the header component.
[25:54 - 26:08] Speaking of which we add the header component first, passing in the user signed in value. For the handle sign in click and handle sign out click event handlers we're passing in an inline arrow function that just updates the user signed in directly instead.
[26:09 - 26:27] Next we show a welcome message and then use two logical operator shortcut statements with user signed in to either display the list of event sign ups or the sign up form to simulate what an admin user might see if they were signed in. With everything in place the complete app component looks like this.
[26:28 - 26:36] Right, the very last thing we need to do to make any of this happen is to wrap our app in the Redux store. Everything we've done so far hinges on this last step being complete.
[26:37 - 26:45] It is the magic that makes all the Redux system tick. Open up the index.js file and let's import two new things.
[26:46 - 26:53] We bring in the Redux provider from React Redux first. We'll need this to wrap around our app to provide access to the Redux store dispatch functions instead.
[26:54 - 27:08] Speaking of the Redux store that's what we'll bring in next, the configure store function we created right at the beginning of the lesson. Next we're going to call the configure store function to provide us with a shiny new store that we can pass to the provider we imported a moment ago.
[27:09 - 27:20] We call it like the function that it is and stash the result in a store variable. Next up we just need to amend the react.random method to add in our provider and store.
[27:21 - 27:32] The provider we imported works just like the context.provider component we saw during the use context hooks lesson. We use it just like any component and pass it to the store variable via the store attribute.
[27:33 - 27:44] And that, believe it or not, is it? We're all done so now it's time to test our hard work and sign up for some events. Fire up the terminal and pop in the yarn start command waiting until it loads in your browser.
[27:45 - 27:55] All things being equal you should see our glorious sign up app staring back at you. Let's sign up two or three times as new people who would like to come along to our puppy petting party.
[27:56 - 28:12] Notice how the display changes between sign up form and the thanks message as we sign up and then reset the form. There's not much to see for now but once we hit the sign in button and in that bar this is where the magic is.
[28:13 - 28:20] We're now ostensibly logged in and so our app switches are showing us a list of event attendees. Using the event sign up list component.
[28:21 - 28:29] See how we have our guests all populated in a nicely formatted list? They're coming directly from the global redox state not from an API or for the component itself.
[28:30 - 28:41] Let's make some changes to the list removing someone and changing the attendance date of another. Notice how the change is persisted as we swap back to the sign up form and then back into the list component.
[28:42 - 28:52] Note that if you refresh the page the global state will be wiped. Redox on its own is not responsible for persisting any state data to a more permanent place.
[28:53 - 29:06] This might be local browser storage under certain circumstances but it's more likely to be a data star via an API call or two. We've come a long way since our humble beginnings with a simple greeting app but you should give yourself a huge pat on the back for making it through this topic.
[29:07 - 29:19] Redox is not always easy to get to grips with and it's one of the most common stumbling blocks for any new React developer. It requires a lot of work up front before you can really see any payoff and some of the terminology and mechanisms can seem a bit alien and confusing.
[29:20 - 29:37] However, what we've achieved in this lesson is to create a new Redux star, action functions that describe the changes to state and an entire reducer that carries out those changes for us. Not only that but we've used arguably the most challenging implementation of the Redux pattern using React Redux's very unappininated skeleton framework.
[29:38 - 29:47] In the next lesson we'll be modifying what we have here to use the Redux tool kit, a much more opinionated approach to Redux that removes a lot of the setup and state modification complexity.