The 7 Core React Hooks [with examples]
We'll be taking a deep dive walkthrough of the most common Hooks in React, including their use cases and how to work with them.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Beginner's Guide to Real World React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Beginner's Guide to Real World React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
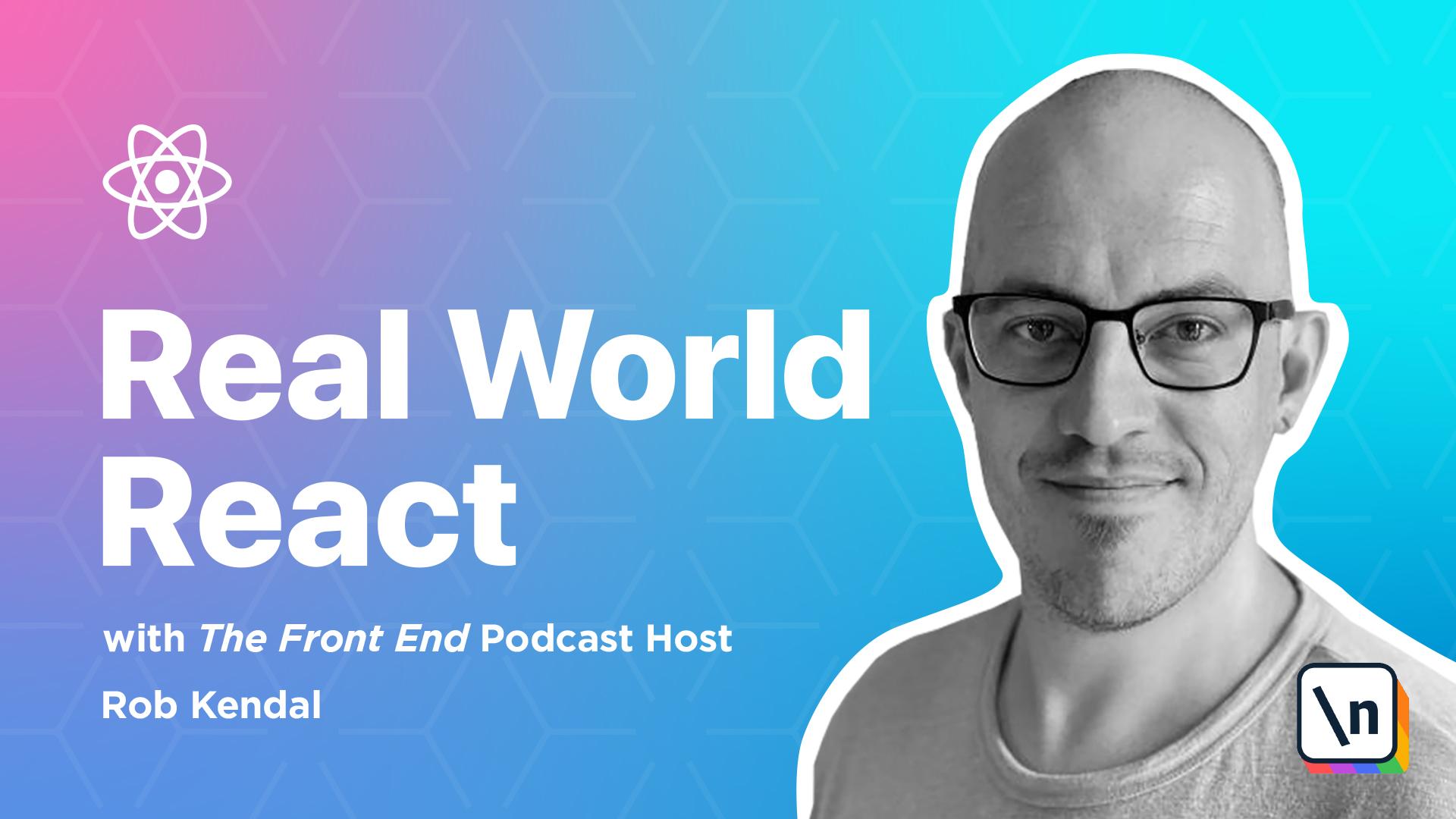
[00:00 - 00:17] Module 7 - React Hooks Deep Dive We've covered hooks fairly lightly up to this point and mainly focused on the two most used, namely, YouStid and News Effect. As we've previously discovered hooks were introduced into the React core library in version 16.8, the current version at the time of writing this module has just passed 17.
[00:18 - 00:27] Their concept is simple, they allow us to hook into various React features without using JavaScript classes. In this module we're going to take a deeper dive into the available hooks React provides out of the box.
[00:28 - 00:34] Before we do that however, there are a few things to note about hooks from the Hook's official documentation. There are 100% opt-in.
[00:35 - 00:44] Even though hooks use is quite widespread, you don't have to use them. In fact, depending on the types of projects you end up tackling with React, you might come across class-based components which can't use hooks.
[00:45 - 00:53] There are 100% backwards compatible. Hooks offer a separate layer to react to hook into React's core functionality, but they don't introduce any breaking changes.
[00:54 - 01:05] Again, depending on the projects you find yourself involved in, you may well see a mixture of class-based components and function-based components using hooks as they are gradually adopted. Classes aren't in danger of lack of support.
[01:06 - 01:14] Facebook themselves have tens of thousands of components written using classes. They're not likely to drop support for class-based components, so you don't have to scramble to rewrite all of your existing components.
[01:15 - 01:25] Hooks don't change React's core concepts. Hooks allow us to use part of React's core features such as state and the rendering lifecycle outside of classes, but they don't alter the concepts behind them.
[01:26 - 01:37] For example, whether you use U-State Hook or this.state.xyz, for a class, the fundamental principles and concepts remain the same. Just like the film gremlins, there are a few rules to caring for and using hooks.
[01:38 - 01:48] Fortunately, they're not as complicated as looking after Gizmo, and there are only two rules you have to be aware of. Number one, you can only call hooks at the top level, and number two, you can only call hooks from React functions.
[01:49 - 01:58] The first rule means that you can't call hooks from within loop blocks conditional statements or nested functions. As a quick example to hammer home the point, let's take a look at the following code.
[01:59 - 02:10] Notice how in the first example a U-State Hook is used at the top level, so an immediate descendant of the good example function. Note that they don't have to physically be at the top of a component, but this is a good idea for a readability point of view.
[02:11 - 02:28] We call setMyValue within a conditional if statement, but this isn't calling the hook merely a value of variable returned from the U-State Hook. However, in the second example, we're declaring a variable and calling the U- State Hook inside of the conditional statement, if.props another condition, which violates rule 1 of hooks.
[02:29 - 02:44] The second rule is short and simple. You can only use hooks from React functions that is either function-based components or within your own custom hooks, which we'll talk about in the moment. I'm going to keep banging the drum for the official React documentation, as it 's comprehensive, well-written, and a superb result for beginners.
[02:45 - 02:54] It details hooks rules there too and provides some instructions for setting up lint in plugins such as ES lint to watch out for and then fast the rules above. Go and check them out if you haven't already.
[02:55 - 03:00] Available hooks. React comes with quite a few hooks built in, and these are the ones we're going to explore throughout this module.
[03:01 - 03:09] Here they are at a glance. We've got U-State, U-Effect, Use Context, Use Reducer, Use Callback, Use Memo, and Use Ref.
[03:10 - 03:24] We're not going to cover U-State or U-Effect, as we've already looked a little deeper at those in earlier modules. Similarly, with U-State, we're not going to cover that here because it will have a dedicated module, let it in the cast, and really, we need to cover the Redux topic before we can understand how to effectively use Reuse Reducer.
[03:25 - 03:36] There are a couple of hooks that we've not mentioned here, namely, Use Imper ative Handle, Use Layer Effect, and Use Debug Value that we're going to leave alone this time around as they're not very common hooks that you'll come across . Let's take a look at the Use-State hook.
[03:37 - 03:48] We've been using the Use-State hook a lot in lessons up to this point. It's quite simple in its implementation, which looks like this. You can access the value in-state by referencing the first variable returned from the Use-State array.
[03:49 - 03:58] In this case, we'd reference the value variable. To update this value in-state, we use the second variable returned from Use- State, the updating method that looks like this.
[03:59 - 04:08] Using a realistic code example for, say, a pageing component, its use would look like this. The Use-Effect hook.
[04:09 - 04:19] Straight out of the gates here, I'm going to once again recommend Amelia Watten berger's superb article on thinking in hooks. It really does a great job of explaining the mind shift you need to take to approach the Use-Effect hook.
[04:20 - 04:29] The Use-Effect hook can be thought of as an initial replacement for other React lifecycle events that you'd seen a class-based component. Events such as component did mount or component will unmount.
[04:30 - 04:43] However, a better way to think of it, as per Amelia's article, is a way to keep various bits of data in sync. React components are concerned primarily with shuffling all the pieces into place to render some slices of the UI to the user.
[04:44 - 04:55] Anything that depends on mutation, subscriptions, timers, logging, or similar code that we refer to as a side effect needs to live inside of the Use-Effect hook. By default, Use-Effect runs on each and every render of the component.
[04:56 - 05:06] You can prevent this, however, by passing an optional argument and array of dependencies. Now, the Use-Effect contents will only be run when any of the items in the dependency array changes.
[05:07 - 05:14] Another optional aspect is return function. You don't have to include a return function, but there are some times when you want to perform some sort of cleanup between Use-Effect firings.
[05:15 - 05:23] It might be that you need to remove an event listener or a subscription to a data handler or API. If you've left these sort of things in place, you can end up with memory leaks in your app.
[05:24 - 05:36] Fortunately, you can return a function from Use-Effect that is called for each time the component unmounts or is removed from the UI. The Use-Reduce-A-Hook The Use-Reduce-A-Hook is a different approach to managing state.
[05:37 - 05:47] It's similar to the Use-Effect hook, but intended more for complex state management situations. For example, where you need to manage multiple sub-values or deal with the state updates that depend on previous values.
[05:48 - 06:00] The Use-Reduce-A-Hook is heavily tied to the Redux state management pattern, which can get tricky to get your head around at first, so we're leaving that until a later module. Once we start covering Redux, we'll be covering the Use-Reduce-A-Hook in lots of depth there.
[06:01 - 06:19] Custom Hooks Of course, you're not just limited to hooks that React provides you, you can create your own custom hooks to abstract common functionality that you want to be able to share across your apps and various components. A custom hook might sound daunting, but really they're just regular JavaScript functions, whose name begins with Use, and that provides some sort of common functionality.
[06:20 - 06:44] For example, here's a custom hook for storing values in the browser's local storage. [ Silence ]