Using React Context API to Pass Data to React Components
Context API provides a way to pass data through the component tree - no more prop-drilling!
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to React Native for JavaScript Developers using TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to React Native for JavaScript Developers using TypeScript, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
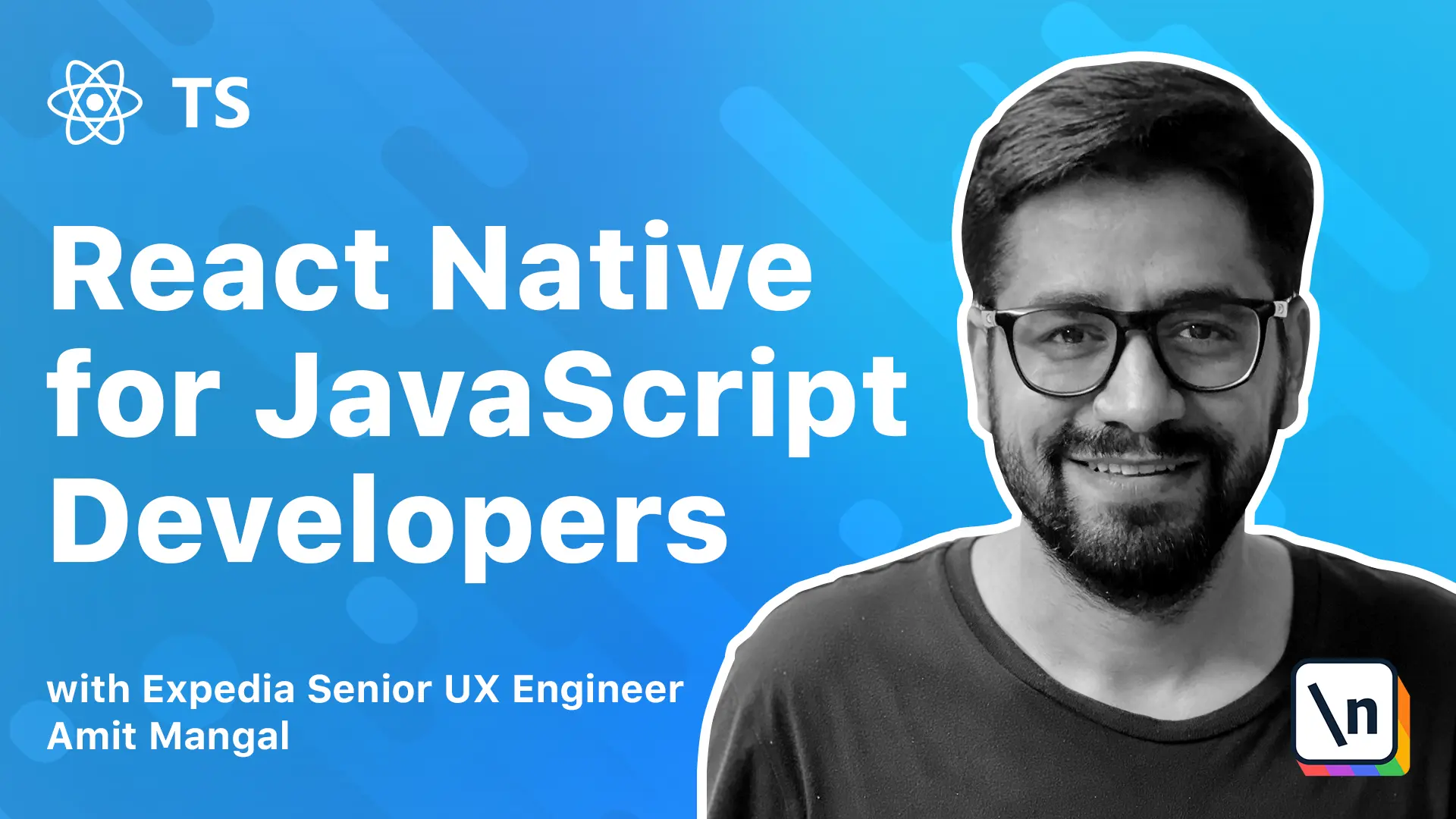
[00:00 - 00:12] The context API is from react provide a way to pass data through the components without manually passing the props down each component. Now this is popularly called prop drilling.
[00:13 - 00:30] A good example of that would be let's say that an application has the user information like the user object with name, surname and email address and other things at the root level of the application. And we want to display a username, say hi, username and then show an avatar badge for that.
[00:31 - 00:55] It might happen that we create a homepage and then inside the homepage we have a header and then probably a sidebar and then the user profile component where we want to show this particular information. What we've seen so far, what we have to do is since the data lives on the homepage, we'll pass that to header component and then the header component will pass it to sidebar and the sidebar will finally pass it to user profile.
[00:56 - 01:10] This is popularly known as prop drilling since we are passing the prop that is required by a deeply nested component from a parent component through all the levels down the children. Now react context API is provide a way to avoid prop drilling.
[01:11 - 01:29] What we can do is we can create a context for the user at the parent component and then just wrap the parent component with the context provider. And now what we can do is we can go to the user profile, which is four levels nested into the parent component and we can just say that we are a consumer of that user context.
[01:30 - 01:45] So we'll say user context dot consumer and now we have the same user object with name and surname and we can use that information in this particular component. So the react context API is primarily used for this use case.
[01:46 - 01:59] This does help in avoiding the prop drilling scenario and that can happen a lot in large scale react or reactative applications. Though they should be used sparingly and the reason for that is let's look at this example.
[02:00 - 02:08] Now we have a user profile component that uses a user context consumer. This particular component gets tied to this implementation.
[02:09 - 02:22] So it impacts the reusability of this component. However, this component is used it expects that a user context will be available to it whenever it is used on the UI so that way it gets tied to it.
[02:23 - 02:39] Let's go ahead and use that in our application and see how it works. What we'll do is we'll go back to our search screen and let's say that we want to search for a particular text that we came from a Google deep link or universal link and we need to populate the text box with that.
[02:40 - 02:49] So what we need to do for that is we need to basically pass the text into the search input component that we have. So here we are on the search screen.
[02:50 - 03:03] So let's first create the context for that. Declare this variable and we'll say dot create context and we can pass whatever we want to.
[03:04 - 03:12] So let's say search term earphones. So that's it.
[03:13 - 03:20] Now we need to pass this as a provider. Let's do that for this entire page or view.
[03:21 - 04:08] We'll say search context dot provider value equals and we need to pass the value to it. So let's declare this and we can pass the same to the provider.
[04:09 - 04:24] Now all the children will have access to this particular context. So we'll go inside header and now we want to go inside search input and now we want to say that we'll pass the text to see text input.
[04:25 - 04:49] So what we want to do is something like we save and we get test there but we want to use the term that has been derived from the parent component that probably got it from a state or a deepening or somewhere. So all we need to do is we need to wrap this particular component into the consumer of that context.
[04:50 - 05:27] So we can do, okay it's not showing up because we have not exported that. So let's do export and we'll do search context dot consumer and we'll do.
[05:28 - 05:56] So we need to wrap the children inside and pass that variable to it. So we'll just say user and now we can access that particular, sorry it was not user it was a search term.
[05:57 - 06:43] So let's just say search and what we can do is value equals search dot search term and what's we save we can see the earphones. So your context really enables avoiding prop printing and we can pass the context to any level that we want and it really keeps the code clean that way though as I told earlier if we want this particular value let's say this is not a mandatory field but let's say that this was a mandatory field like in case for user and we want to display a username and we use this particular pattern for that then wherever we will have that particular component that gets tied to the context and the provider for that.
[06:44 - 06:50] So that is a limitation with this. Another use case can be using multiple consumers.
[06:51 - 07:42] What we need to do is we just need to wrap it inside another context. So let's say that we had another context and it was some kind of a user context and what we could simply do was we could wrap the whole thing inside our user context and to access this what we could do is we could simply say we want the user context dot consumer and very similarly we could access it since this starts to get a little clumsy the alternate way of doing that is declaring a hook and let's see that.
[07:43 - 08:56] So we'll see that for search context itself we'll go back and pass this as a function and we don't need all of this and what we can do is declare search react dot use context and we'll say search context and now search context it will act the same way. If we go and change the value over here to let's say in earphones it will do that.
[08:57 - 09:22] So now if we have multiple context what we need to do is declare different variables for that and that way we can avoid that clumsy implementation of the UI. So I'll just remove this.
[09:23 - 09:52] Now as we saw react context API is help us avoid fraud drilling but it's not really dealing with state management. The reason for including react context API under state management is because there is a very common use case where since we can have a particular state now we have this object it can also be called a state for this particular tree what we can also do is extend this particular implementation to an app level.
[09:53 - 10:12] So we have one root implementation that's app and what we do is we wrap this app around the context for the entire application that works as its state. Now we should not do that the reason for that is first of all it's not built for that.
[10:13 - 10:38] Secondly it becomes very difficult to track what attribute has been changed in what part of the application like we just said that we are doing search term in earphones but what we can actually do is we can also change the search term to be something else and that also works. So this becomes very very dangerous.
[10:39 - 11:16] Now in the entire application if there's in context that moving around and anyone can change that context it becomes very difficult to debug what particular action or what particular piece of code changed that value of state and in enterprise application finding that kind of a bug can become really really challenging. Now that is where state management libraries like Redux and mob x and other come into picture which really helped track down which particular action caused a change in state and what was the change and how we can backtrack that if there is a bug in our code or something that we want to trace.
[11:17 - 11:41] And look at that in the next lesson. So that was all for React context it has a very specific use case and it should be used sparingly like I said it's good to avoid propped drilling but it can also tie a component to a particular implementation.
[11:42 - 11:52] One common and great use kids where yet context can be really used is changing theme of the app. We have a light theme in a dark theme based on the time of the day.
[11:53 - 12:14] So with context API is what we can do is we can go to our typography and we can define two different variables for light and for dark and then based on the context our global styles can pick different values based on time of the day. So that becomes a good use case that's all for this module.
[12:15 - 12:16] Thank you and I'll see you in the next one.