How to Store User Login Data With React Native Async Storage
AsyncStorage is an asynchronous, unencrypted, persistent, key-value storage system for React Native.
This lesson preview is part of the The newline Guide to React Native for JavaScript Developers using TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to React Native for JavaScript Developers using TypeScript, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
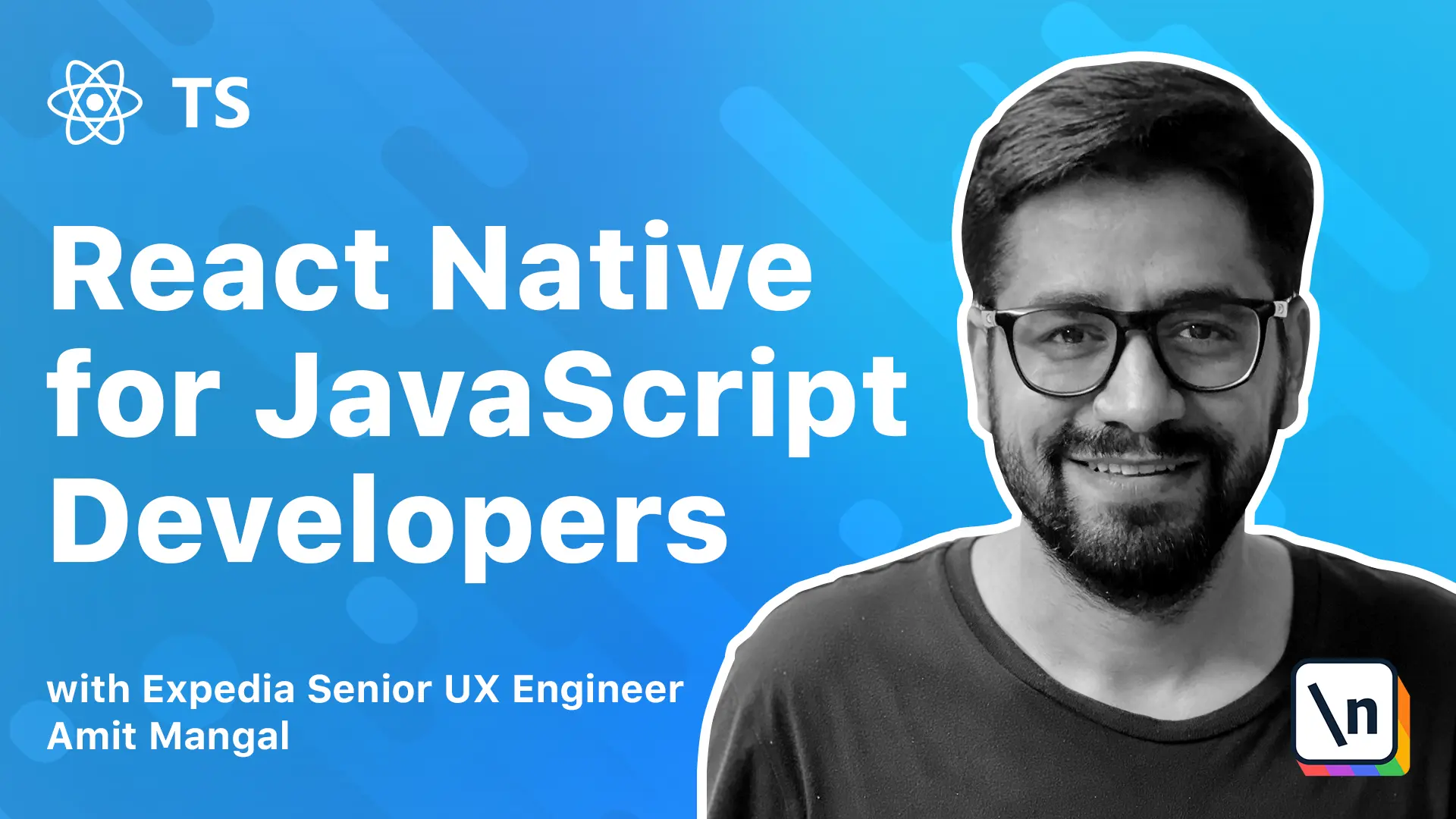
[00:00 - 00:13] Or the data that we have collected so far in the app, like the recent search terms, the card, everything is in memory. And as soon as we would refresh the app, we would lose that information.
[00:14 - 00:21] We don't have the card anymore. The recent searches are there because we have it hard coded over here.
[00:22 - 00:34] But let's say that we wanted to store this and we wanted to get back the card information, the recent searches information. And I would ideally want to store this information in the persistent storage.
[00:35 - 00:45] So that is when the user kills and comes back to the app, we are able to retrieve that information and show it to the user. And this can also include the user token.
[00:46 - 00:52] So we'll also be able to continue the user session from there. This is where async storage comes in.
[00:53 - 01:06] Using storage is a persistent key value store, it's a very simple format. What it does is it stores the data in the app on iOS and Android and it basically takes a key for the value that we want to store.
[01:07 - 01:24] And the value is any string that we want to store. Generally, what we do is we take a JSON and we stringify it and store it and then pull it back into the application state when the app relaunches or whenever we want to.
[01:25 - 01:29] Using this is quite simple. We install the async storage library from the React Native community.
[01:30 - 01:39] This was a part of the core React Native. But since the Lean Core initiative by React Native team, they have started moving out a lot of these optional dependencies.
[01:40 - 01:45] It is not absolutely required to have async storage. It's based on the use case.
[01:46 - 02:01] So that way it is great and it keeps the core React Native as light as possible . So we'll just add this async storage library to our application and then just we just need to do a part install for iOS and from there, it's pretty simple.
[02:02 - 02:11] So let's do that. Let's open the package.json and we would want this independently.
[02:12 - 02:20] Okay, we have it installed. Let's go to the iOS directory and do pod install.
[02:21 - 02:25] Let me come back when this is done. So it installed React Native asian storage.
[02:26 - 02:42] We'll just start the Metro bundler again. And what we'll do is we'll go to the core services and like we implemented the API service, we'll implement the storage service over here.
[02:43 - 02:50] It's a part of the core service. So that will abstract out the implementation from the rest of the application.
[02:51 - 03:06] Let's start doing that. const storage export default storage.
[03:07 - 03:21] And if you look at the async storage API, it provides a lot of methods like get item, let item, merge, remove items. What we'll do is we'll implement get item and set item and the rest of these are best based on the use cases.
[03:22 - 03:45] So let the get and we need a key that we want to get the data for. So let's go to the core and storage from React Native asian storage.
[03:46 - 03:57] And we'll write this in try catch block. Return this to get item.
[03:58 - 04:13] And we need to pass them key to it. For error block, let's log it to a congun for now.
[04:14 - 04:29] Once we have a network where a dashboard set of like century, we're going to look in on the upcoming lessons, we can push it to that too. So we'll say async storage get field.
[04:30 - 04:38] It's also long to keep. First we error.
[04:39 - 04:44] Next is also implement the set. Before we can get a key we need to set it.
[04:45 - 04:54] We pass the value, which will be of type any between any JSON object. It doesn't let me to have a particular structure.
[04:55 - 05:12] So we'll say async storage dot set item and what this teach is a key and a value as a string. So we'll convert that JSON to a string and handle that over here.
[05:13 - 05:26] So the app is still passing an object. They set field for key.
[05:27 - 05:31] So that's it. Let's now go ahead and use it.
[05:32 - 05:44] So what we can do is we can either save the card that we want. So we have a thumb for that for saving the card or we can save the recent surgeon.
[05:45 - 05:55] Because once we kill the application, we'll use these searches. So let's go to our home page.
[05:56 - 06:08] And we're doing an add to cart over here. So right here, we're from the SQL and calling this add item to cart from thumb go to our thumb and save this card to persistent storage also.
[06:09 - 06:17] So let's do that. Let's go to the phone for saving the scar, which is this one.
[06:18 - 06:27] So right here, we're saving it to the cart after this. Let's get the card, which is this and then also saving to Ethan's stories.
[06:28 - 07:03] So we're going to take for students, so from services slash, or slash, storage and call it just storage also, so it's a storage, the storage dot set and we fast the key. So let's lay a key for that.
[07:04 - 07:12] We use a hardware value. So we'll go to content and we'll say.
[07:13 - 07:36] Key card, let's cut. So in for the config, the code config from the feed at the config from services slash config.
[07:37 - 07:51] And we'll say config.keys.card and we also need to pass the venue. So the store is the card state.
[07:52 - 08:01] So we'll say store. Keep them and now when we add items to cart, it will also say it to the stores.
[08:02 - 08:14] And there's no direct way to see what data is stored into the Asian storage, although there's a tool that's called react to Tron. Clear allows me to see the network call and also what's inside the storage, but all and we can use that.
[08:15 - 08:20] What we'll do is also application launches. We get the item back and console it so that we can also see it.
[08:21 - 08:28] So let's do that. We do the app come this fast V launch and as it launches, we get the item from the card.
[08:29 - 09:14] So we'll say storage.get and we pass the key. Config.keys.card save it and it returns up from it.
[09:15 - 09:22] So we do const. Card.
[09:23 - 09:41] Wait and push it to the application card, but let on add item to card, but we 'll do that. But before that, let's console that for now.
[09:42 - 09:49] For this error because we added Asian storage and this is not pure JavaScript library. There's also some need code that library elements.
[09:50 - 09:56] So what we need to do is we need to build the application and launch it again. So I just do that.
[09:57 - 10:18] So I'll sneak yarn, I will and I'll get back to the point where this goes to application. So the win took some time, but we're back and it features three rounds the app and we look at the value of the console.
[10:19 - 10:27] Clicking this also open map. Start the app.
[10:28 - 10:33] And as we can see, the card is coming in. It's not because there's nothing in there right now.
[10:34 - 11:10] So let's go ahead and click that add to card button on the phone piece and soon it's add to the card and then we'll try and check the launching the app. So we'll say, and since we are now saving this for the, which is Asian stories, now add to our card button, we should have that.
[11:11 - 11:24] So we'll go back to our app and re-launch it. And now if we look at the console.
[11:25 - 11:31] We have that card. So as we saw, we kill the app and then content, we get the card back.
[11:32 - 11:52] So next what we need to do is we need to set it back to our application state. The one way to do that would be we dispatch the same actual, but this actual is defined for only one item and and using that my mess with an antique next city also have some analytics written over it that fires a particular pixel on listening to the active card action.
[11:53 - 11:56] So that will also get fired. So let's do that.
[11:57 - 12:14] What we'll do is we'll write a separate action for it that says add items to card and that will say initiate card. And then we know that this is the way that the action that happened in the, the application.
[12:15 - 12:26] So we'll fail. In it cars, this is for that and I just go and add this action.
[12:27 - 12:56] So we'll say unit card. And we'll see that and we'll go to reducer.
[12:57 - 13:26] And we'll add an individual. And what we can do is we don't have any items when the application launches in the application state in the now, so what do is that action for data to end and we 'll see if that.
[13:27 - 13:56] So we also need to add data to this, which is for beer and it will be a type. Card state, which is area of card item and see it that and now all you do is we need to go back to our thumb and this past data.
[13:57 - 14:23] This pants and we will say, we need for the card schemes. So we'll say item and then card.
[14:24 - 14:35] So the error is if we need to fast and item array and what we're doing is the first thing because it returns a string. What we could do is before running the value, we can pass this as G1 and give it back.
[14:36 - 15:02] That you can say, what we can do is if we have something in value, we can say return. Instant but fast, when you are, will definitely turn.
[15:03 - 15:27] That and now tank cost this and any of our card item, which is what our card state is. So let's say that and now when we check the state, let's restart the app and let's come in.
[15:28 - 15:32] We already have an item. So click it.
[15:33 - 15:45] Let's go back to the next one. So let's go back to the next one.
[15:46 - 16:21] And let's restart there. And once we continue, we'll have the two items that we added to card.
[16:22 - 16:33] There you have it. So let's see for my engine look is the badge on the card was said.
[16:34 - 16:55] This was not happened when we did that from the launch screen and I'll explain that in one second. So let's just relaunch that.
[16:56 - 17:07] So now our card will be persisting. Now about updating this bank, we card from our recent storage on the splash screen.
[17:08 - 17:14] And then that's also where we set the state where it's available. But this tab navigation is not available on the splash screen.
[17:15 - 17:23] It happens only after that. If we go back to the navigator, we'll see that that once we continue from our splash we get the tab navigation.
[17:24 - 17:34] So what we need to do is when we set that navigation, we need to get this where we need to get the value from the store and set the value of the batch. So let's do that.
[17:35 - 18:16] We'll go to navigator, then we get the store and set this value for not for settings but for card over here. So we go to the top and we'll import store and port, store from, share, store.
[18:17 - 18:57] Let's go to cart icon. So, and store dot, date, dot card, dot items, dot meant.
[18:58 - 19:19] And say that now once the app is selected, it will fetch the data from the store and set that to state and when the tab navigation is shown, we could also fill the bad for the card item. So let's re-wrote the app and see that.
[19:20 - 19:35] Okay, we need to make this as a string. The batch does not accept numbers.
[19:36 - 19:52] We used to. Now that we go to the tab navigation, we'd also have the bad with the number of items in the car.
[19:53 - 20:09] So next, what we can do is you can also save these instance searches. This is right now, far-quoted into the search, but we can save this to 18 students and I leave this up to the students to implement.
[20:10 - 20:26] So what we've done is we can't implement the get and the set for our async storage. We can also implement other methods that are required for to have mostly the move or the remove all methods in the required so that we can clear the car.
[20:27 - 20:37] In some cases, when we are locking our danger, so we won't clear the storage for that token and also for other things that we can have stored for the particular user. So that is useful.
[20:38 - 20:47] The other thing is that we can do a little bit of this. We can do a little bit of this.
[20:48 - 20:53] So we can do a little bit of this. So we can do a little bit of this.
[20:54 - 20:57] So we can do a little bit of this. So we can do a little bit of this.
[20:58 - 21:01] So we can do a little bit of this. So we can do a little bit of this.
[21:02 - 21:08] So we can do a little bit of this. So we can do a little bit of this.
[21:09 - 21:14] So we can do a little bit of this. So we can do a little bit of this.
[21:15 - 21:20] So we can do a little bit of this. So we can do a little bit of this.
[21:21 - 21:24] So we can do a little bit of this. So we can do a little bit of this.
[21:25 - 21:28] So we can do a little bit of this. So we can do a little bit of this.
[21:29 - 21:36] So we can do a little bit of this. So we can do a little bit of this.
[21:37 - 21:40] So we can do a little bit of this. So we can do a little bit of this.
[21:41 - 21:44] So we can do a little bit of this. So we can do a little bit of this.
[21:45 - 21:49] So we can do a little bit of this. So we can do a little bit of this.
[21:50 - 21:53] So we can do a little bit of this. So we can do a little bit of this.
[21:54 - 22:01] So we can do a little bit of this. So we can do a little bit of this.
[22:02 - 22:05] So we can do a little bit of this. So we can do a little bit of this.
[22:06 - 22:09] So we can do a little bit of this. So we can do a little bit of this.
[22:10 - 22:13] So we can do a little bit of this. So we can do a little bit of this.
[22:14 - 22:17] So we can do a little bit of this. So we can do a little bit of this.
[22:18 - 22:21] So we can do a little bit of this. So we can do a little bit of this.
[22:22 - 22:36] And if the application does some kind of a login, we also talk about fetching that user token and validating the user token. So there's quite a few things that's going on here and having something like this inside the new turnprint sense.
[22:37 - 22:44] And it also clutches that you with a lot of business logic that's not required. So these middleware are really, really fantastic.
[22:45 - 22:52] And you can also control the order right here. So if you want to validate the user and then fetch the card, we can also do that.
[22:53 - 22:56] So that's all for this module. And I'll see you in the next one.