Customizing React Native Elements With Global Style
We'll look at the Atomic design pattern's atoms and molecules; what they are and how they help build the design system of the app.
This lesson preview is part of the The newline Guide to React Native for JavaScript Developers using TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to React Native for JavaScript Developers using TypeScript, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
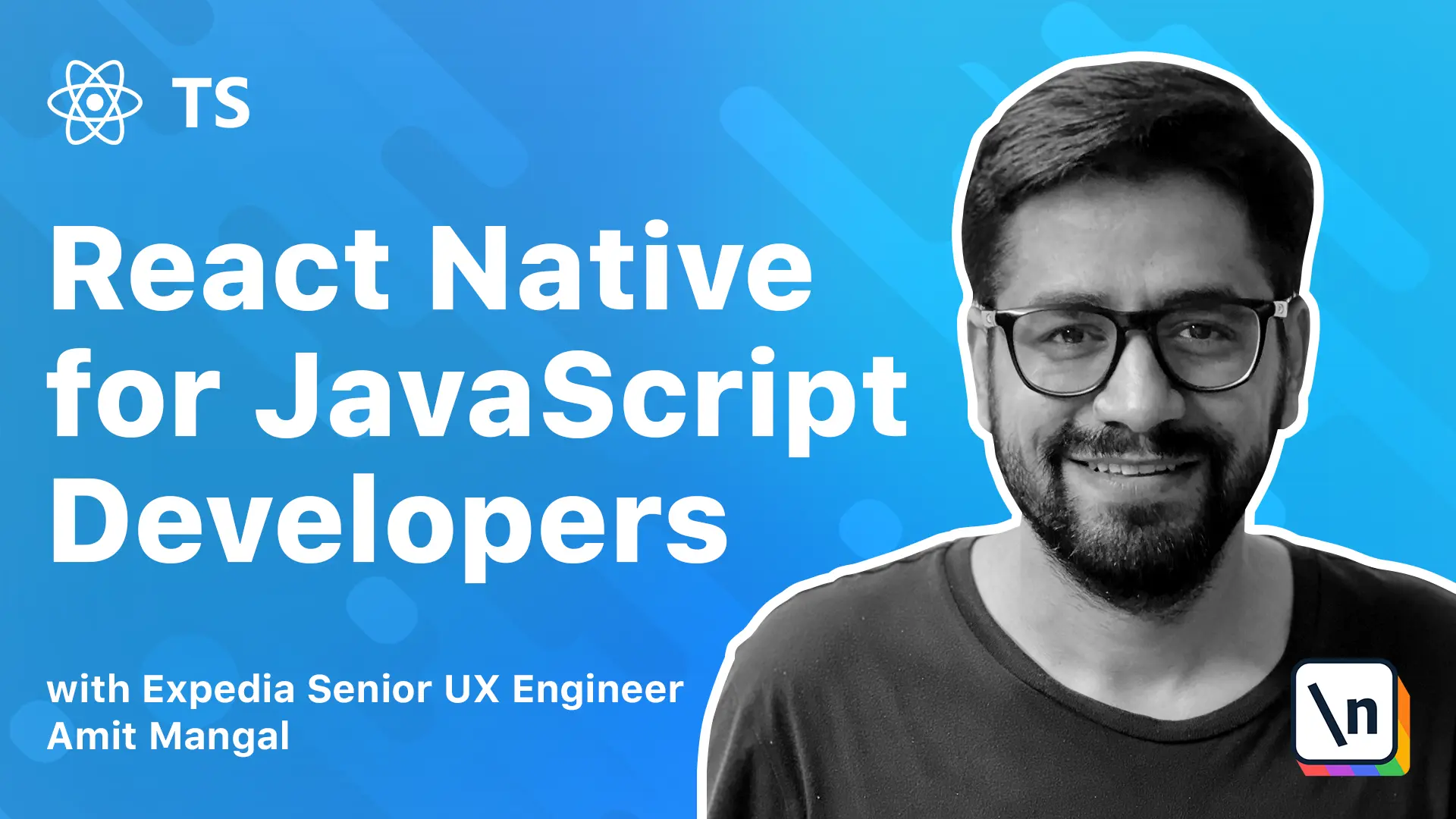
[00:00 - 00:09] In this lesson, we'll look at atoms and molecules. Now, if you would recall from the atomic design pattern lesson, we had five different components for any view.
[00:10 - 00:28] We had atoms, the smallest building units of any view, then we had molecules which were atoms grouped together to form a certain functionality, like a search box. Then we had organisms, they were the complete components that would provide a certain feature, and then temperature in pages.
[00:29 - 00:47] So, what I generally tend to do is categorize atoms and molecules, the smallest building blocks of any view as elements, and organisms as widgets. As we go forward, you will see that the differentiation between these, though, are very fundamental, but can sometimes overlap.
[00:48 - 00:55] Let's say that we have an icon, and it's going to be an atom, right? Then we have a button. It's going to be an atom.
[00:56 - 01:03] But what if a button is also displaying an icon with a text? Now, that might fall in the category of a molecule.
[01:04 - 01:11] Similarly, it can also be for a search bar. We have a simple search bar with an icon. We have two atom elements that combine together to form a molecule.
[01:12 - 01:29] So, we'll be covering them as elements, and in widgets, we'll put everything that we're going to categorize as organisms, basically complete functionalities , like an entire header, or an entire product carousel, and similarly. Let's look at these from our design, the app that we're going to bend.
[01:30 - 01:45] This is the view. Let's look at what the elements are going to be. So, if you look at this, we have these icons, and they're used in a lot of places for building these buttons on a search bar on this particular camera icon that takes an image and does search based on that.
[01:46 - 01:57] Molecules would be this entire search bar, the text input along with the icon. Then we can also have these buttons with the text as molecules and so on.
[01:58 - 02:05] So, let's break the UI down into atoms and molecules first. These are some of the examples on the left that we see.
[02:06 - 02:13] We have these icons, the title of any particular section, the input box. All of these can be categorized as atoms.
[02:14 - 02:30] Here we also have more examples, like a badge, how a badge we're supposed to be displaying, generally the pattern is to display it with a red color, and that tends to be a common experience across the apps to reduce a user's cognitive load. Then we can have buttons, we can have icons, which we're going to have for this app.
[02:31 - 02:40] An image, now what do I mean here by an image? Even the native elements that we're going to use in the app, the text, the input box, and image, we're going to create a very simple wrapper over them.
[02:41 - 02:54] And the reason for that is, that makes them very, very configurable going forward. Like for example, we want to show a text box in a particular format with a particular background, and we wanted to have a font of certain size.
[02:55 - 03:06] Now going forward, we decide that no, we need to change the size of the font for the text for any XYZ reason. If you're using native elements, we'll have to go and change that in every view that we have.
[03:07 - 03:14] Instead, if we have very thin wrappers, they can allow us to customize that particular element. Also, if we need to make changes, we just need to do that at one place.
[03:15 - 03:35] Similarly, we're going to do for images, inputs, and we don't have links in this design, but we can guess if we have links, we could also do that for them. If you notice all of these are very basic elements, they themselves don't tend to provide a feature or a functionality, but they'll help us build the page in general.
[03:36 - 03:58] These are the molecules that we see, the search box along with the icon, and once we click on it, we'll be taken to the search page or will be shown the result, the category icon along with the text icon was an atom, and we used another atom to create this particular molecule. And similarly, we had the heading and the C all, so we created this particular molecule using those atoms.
[03:59 - 04:12] Those would be our atoms and molecules, and what we see on the right are going to be our widgets, or the organisms in atomic design pattern terminology. We'll look at those in the next lesson.
[04:13 - 04:21] So, let's start building some of those. If you go back to the application under SRC and view, we will have elements.
[04:22 - 04:38] The boilerplate already defines some of the basic elements that you would have seen through the lessons that we have gone so far. For example, C text, this is a custom text, and it's a wrapper over the text element, and we are simply displaying the text that is passed to it.
[04:39 - 04:50] But what it helps us to achieve is we can now style the text element depending upon our style guide. And if we want to change that going forward, change the font or anything, we can do that.
[04:51 - 05:01] Similarly, we also have one for text input, and it's again quite a similar implementation. We'll be building the text input as per the design that we have over here.
[05:02 - 05:07] Let's also look at the buttons. We already have two buttons, a button, a primary and a button, a secondary.
[05:08 - 05:14] We'll also be defining the category icons. They also seem to be buttons, because we can click on them and go to a different page.
[05:15 - 05:22] So, let's get started with that. We will move to Home, Component, and let's display a text input.
[05:23 - 05:34] So, we'll do C text input, and we will simply display it. And once the app refreshes, we'll see that we have this input box.
[05:35 - 05:50] Now, it might not be visible because of the current styling, and let's make it look like how we want it on the UI. Now, ideally, we would have these typography and styles provided to us by designers, but let's still go ahead and try that.
[05:51 - 06:02] So, we have this text input, and if we go to the definition, we are applying some global text input default styles. Let's update that.
[06:03 - 06:18] The first thing we'll need is probably a background color, and we'll pick it from typography. Next, let's also add border radius.
[06:19 - 06:39] For a radius, typography.fond.input, and then we'll do. We don't have a border radius, we should probably add that, but ideally, this will come from design.
[06:40 - 06:50] And let's say that it's basically going to be half of whatever the size of the text box is. So, let's just add 10 for now, and we'll do.
[06:51 - 06:56] Dot. Okay, I did not name that correctly.
[06:57 - 07:06] And let's just save it. It refreshes.
[07:07 - 07:12] And we already have the background color. Next, we should have the border radius, and that's mostly it.
[07:13 - 07:17] I think the height is supposed to be different. Let's also add that.
[07:18 - 07:27] We'll have height as typography. Dot.
[07:28 - 07:31] Dot. Input.
[07:32 - 07:41] Okay, we don't have a height, so let's go and specify that. So, we'll say height, and probably 32.
[07:42 - 07:50] Save it. Height.
[07:51 - 07:57] And now once we save it, we should be able to see the changes on the UI. There's our input box.
[07:58 - 08:03] I think the height is okay. It can increase a little, but the border needs to be more.
[08:04 - 08:18] So, what we'll do is, let's do the height as 36, and border can be 18. We're just looking to build the atom, the basic input box here.
[08:19 - 08:29] So, we'll not work on the icon part. Icons can be considered as atoms, and once the app refreshes, you can see the input box now looks like what we want to build.
[08:30 - 08:43] So, if we type into this, let's just also style that out. We'll just say react, native app, and I don't think we want the text to be in clean.
[08:44 - 08:49] Let's also do that. We'll go back to our custom elements, and this is the input box.
[08:50 - 09:07] We're going to say, we'll probably need some padding, and we want a bigger font size. We'll say font family and font size, it says 12, and let's make it 14.
[09:08 - 09:13] And we'll also need to add some padding. The text is starting a little too early.
[09:14 - 09:21] So, let's also do that. Padding horizontal will be fine.
[09:22 - 09:45] We'll just do typography.font.input. Padding horizontal.
[09:46 - 09:53] So, let's go ahead and add that. We'll just say padding horizontal.
[09:54 - 09:59] 8 for now. We'll save this.
[10:00 - 10:05] Okay, I think we also need to change the color. So, let's just do it.
[10:06 - 10:16] default is white, default selected is the dark one. There's the one that close it to black. Let's do that.
[10:17 - 10:24] Let's save, and once the app refreshes, we'll see the changes. And that looks better.
[10:25 - 10:28] Although we could add more padding. So, again, yes.
[10:29 - 10:36] Something that needs to come from design. So, we have our input box.
[10:37 - 10:42] Next, let's look at creating one of these icons. So, these can probably be buttons.
[10:43 - 10:54] And I've already added the SVGs that are required for these to the application. You can find them in the SVGs file.
[10:55 - 11:03] And what I've basically done is I've created a separate file that's called category.tsx and all the category-related icon. This is like a category list.
[11:04 - 11:08] And the category-related icons are all inside this. Let's go ahead and do that.
[11:09 - 11:17] We'll create another button. So, we'll go to button.tsx and let's create that.
[11:18 - 11:28] We can copy this over. Let's call it button category.
[11:29 - 11:36] And export it already. And let's just write down the whole page so that we can see the changes.
[11:37 - 11:45] We'll just copy this one over. But this time we'll say that it's a button category.
[11:46 - 11:50] We'll have the button. But let's just go ahead and style that.
[11:51 - 12:18] What we'll do is we'll need to definitely add the icon first. So, let's remove the text and we will say import SVG icons from assets/svgs.
[12:19 - 12:23] And let's start with the first one. So, that's a shoe.
[12:24 - 12:31] Let's go ahead and display that SVG icons. Let's do shoes.
[12:32 - 12:37] Save it. And we don't want these styles.
[12:38 - 12:42] Let's create a style for this. That's because the style is incorrect.
[12:43 - 12:56] Let's go ahead and add styles to this. So, we'll go to our globals.tsx and we'll create a style for this.
[12:57 - 13:03] So, let me do that. Let me add the styles and we'll continue from there.
[13:04 - 13:06] Okay. That's our icon.
[13:07 - 13:19] What I've basically done is I went to typography and I created a typography for it by the name category. And then I went to global and for category icons I added the styles that are required.
[13:20 - 13:28] Basically, whenever we use this particular type of button, all the styles are coming from our design system. Let's do one more thing.
[13:29 - 13:34] The icon is sort of hard coded into this particular button. We can make that as a parameter.
[13:35 - 13:44] What we'll do is we'll take icon as a prop and we'll pass it to this one. So, whenever we want to show a button of typography, we need to pass an icon for it.
[13:45 - 13:52] And the rest remains the same. We'll copy over the props.
[13:53 - 14:19] And we can call it icon props. And we'll take icon and it will be here in the Act element.
[14:20 - 14:35] We'll just assign it to, we'll say icons. And we'll just say icon over here.
[14:36 - 14:51] Let's save that and now we need to pass it from the Home component. We don't take a title anymore, it takes icon and we need to pass the icon over this.
[14:52 - 15:02] We already have SVG icons, SVG icons, start, category icons, start, show. We'll save that and we get the icon.
[15:03 - 15:09] It seems a little off, but once I refresh the eye, I would be fine. Let me just go ahead and try and create those icons.
[15:10 - 15:17] We have a show and some stilettos and then a pulp. So, let's quickly do that.
[15:18 - 15:25] We'll do a till a dose and we'll do a pulp. We'll save.
[15:26 - 15:35] And if we refresh the view, there we have our icons, which we can use as buttons. So, as we've reconqued from the beginning of this lesson, we talked about building the elements.
[15:36 - 15:42] We have done that. We already have the title in the app.
[15:43 - 15:48] Then these icons are already there. This is what we could also build, but the process is going to emit quite the same.
[15:49 - 15:53] We have the input boxes. So, these are all the atoms and molecules that we're going to build for now.
[15:54 - 15:57] I'll add the rest to the repository. And there can be more.
[15:58 - 16:04] We can keep building as we go forward into the app. As you look at the view, we have quite a lot of it.
[16:05 - 16:10] We have the title, the subtitle, the text, the icons. Now the app is starting to take shape.
[16:11 - 16:13] Thank you and I'll see you in the next module.