Getting Started With React Native Navigation
Stacked app or tabbed navigation, drawer menus and modals, and what's the best place to load user sessions, and other application data.
This lesson preview is part of the The newline Guide to React Native for JavaScript Developers using TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to React Native for JavaScript Developers using TypeScript, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
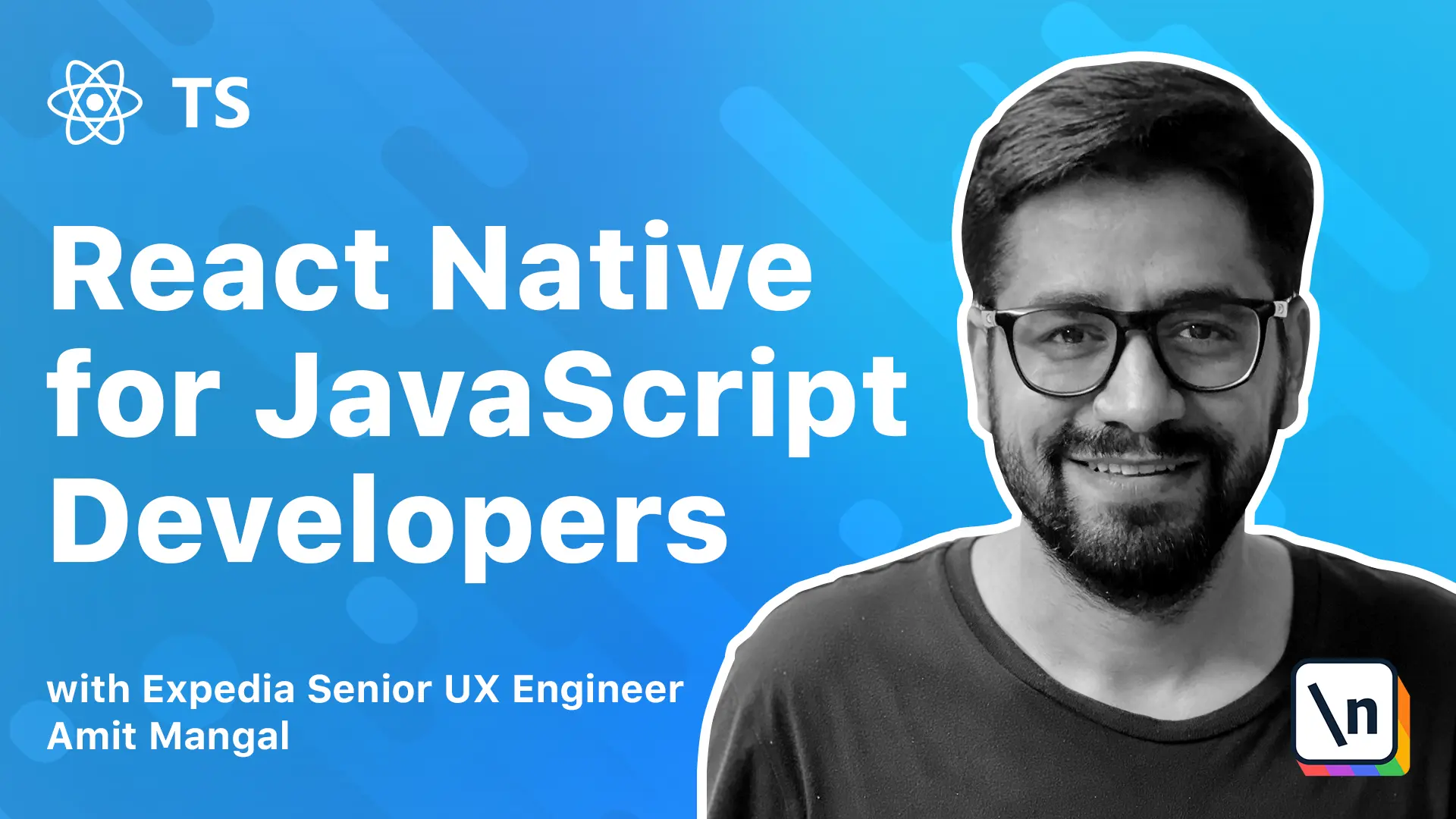
[00:00 - 00:09] We'll be using React Native Navigation to build our app. There are a few other solutions available too and also very popular, like React Navigation is one of them.
[00:10 - 00:26] Though from my experience, I recommend React Native Navigation as it provides a 100% native navigation on both the platforms. What that means is, it doesn't take up those extra event loops on the JavaScript thread.
[00:27 - 00:37] Most apps have two types of setup. Either they are a single screen app, which are also called the Stacked Apps or a Tap-based Navigation.
[00:38 - 00:45] The choice is totally dependent on what we are looking to build. In this lesson, we'll look at how to set up both of these.
[00:46 - 01:05] Stacked apps also tend to have a side menu or a drawer and React Native Navigation provides out of the box support for that too. These types of apps are generally called Stacked Apps because the screens that are navigated to are pushed onto the base screen, creating a sort of stack.
[01:06 - 01:23] The animation on both the platforms are configured to have the familiar native experience, that is the new screen slides from the right on iOS while it fades in from the bottom on Android. Tap Navigation are all very popular and are seen on most of the apps.
[01:24 - 01:34] It provides for easy access to different sections of the app from a single location, the Tapbar. The boilerplate code starts with a single screen app, a single screen setup.
[01:35 - 01:50] It is called the splash screen in the code and then it moves to a tab navigation. Now this kind of setup is great to have some initial marketing screens or load user data or ask for login and then take the user to the main experience.
[01:51 - 02:08] The drawers on the side menu are like partial screens that pop out on top of the current screen from the left or the right depending on the configuration, not fully covering it. This is very similar to what we have seen traditionally on mobile websites.
[02:09 - 02:25] It can be accessed by either swiping from extreme side to the left or the right or you can have an accessible button like a burger menu. So let's jump into the code and apart from the two navigation setups, we'll also look at using modals.
[02:26 - 02:46] Okay, so let's start the macrobundler and we can run it on iOS or Android using the simple code that we have. It's already configured on the scripts.
[02:47 - 03:13] So let's just do yarn iOS. Now if you remember from the navigation index to TSX, we were listening for a launched event and then we'll just show the splash screen.
[03:14 - 03:27] Now right now it's just showing a simple screen as we will see once the app installs and runs. Let's, it's already built so it shouldn't be long.
[03:28 - 03:35] Yeah. And we have our modaler running.
[03:36 - 03:42] Okay. Now this is a splash screen.
[03:43 - 03:51] Right now what it does is if you click the button, it takes us to the tab navigation, but let's say we don't want that. We want a stacked app.
[03:52 - 04:01] So the first thing that we need to do is we need to go to the navigation and then right now it's just a simple screen. We need to tell it that we need, we want to build a stack app.
[04:02 - 04:24] So what we'll do is instead of passing the component directly, we'll see that we want stack and then as children, we'll just pass. Sorry, just pass the component of splash screen.
[04:25 - 04:38] So id screens.plash and name. Okay.
[04:39 - 04:55] So now this is a stack app. Let's go to the splash screen and try in and instead of moving to the tab navigation, let's try and move to a different screen.
[04:56 - 05:19] We'll just call this function and we'll need the component id for this. And we can get this from, it's available in the props for every screen.
[05:20 - 05:38] So we'll just do navigation dot since we want to push screen, we'll do push. We have the component id and we'll just pass what screen we want to see.
[05:39 - 05:56] Now for now I have a dummy screen created, let's just do that. And okay, it's giving an error because I have not declared it in props.
[05:57 - 06:06] The moon or the pane of TypeScript. And let's just click.
[06:07 - 06:12] Yeah. So now as you see what's happening, the screen is being pushed onto the base screen.
[06:13 - 06:22] Now if we move to the second screen and now push more screens over it, we can see how a stack has been created, right? So that's why this is called a stack navigation.
[06:23 - 06:31] Now we've directly called the navigation here. I recommend moving all these routings to the router that we created.
[06:32 - 06:40] So what we'll do is I already have a route defined for pushing the dummy screen . Let me just call that from here instead of doing this.
[06:41 - 06:55] So we'll just do router dot. So push screen and it needs the component id.
[06:56 - 07:07] So we'll pass that. And that should do it.
[07:08 - 07:14] Great. So it also keeps our component really clean, right?
[07:15 - 07:26] So let's add a side menu or a drawer to the screen. So for that again, we need to go to the route, the navigation.
[07:27 - 07:43] And in route, we need to say that we add a side menu and a side menu will have a left or a right and in a center. So we'll say it's a left and on the left, the component that we want to show.
[07:44 - 07:57] So I've already created a simple screen name drawer. So if we go to it, it's nothing.
[07:58 - 08:11] It's a very, very simple screen just shows two texts. And let's add the id and the left is the drawer and what do we have in the center?
[08:12 - 08:22] We need to tell it that. So we'll have center and we can simply copy that we'll need the entire stack.
[08:23 - 08:25] And if we save. Yeah.
[08:26 - 08:39] So it's not visible right now, but if you pull from extreme left, there it is, the drawer menu. Now we can also add an icon to access this.
[08:40 - 08:52] Let's just do that very quickly. Let's go to the splash component and let's add a button.
[08:53 - 09:07] We will add a burger icon, which I've already added to the assets. So there's a burger menu.
[09:08 - 09:26] So let's just do that. Burger menu image and pressable is basically the new version of touchable opacity, which is we basically, we can use to handle any kind of touch events.
[09:27 - 09:37] So what we'll do is we just need to add the on press event to it. And we'll say that we'll call show burger menu.
[09:38 - 09:42] That's a method that we have read declared. So we just do navigation dot merge options.
[09:43 - 09:52] And this is the idea that we need to pass. It should be the same as what we declared for the draw screen.
[09:53 - 10:05] So let me just make it same. Once I save it, it's taking space, but it's not really visible that because the image we need to pass some kind of height and width.
[10:06 - 10:14] So let's just call it menu. Let's go and declare height.
[10:15 - 10:22] Let's say 44 width. It's a 44 and save it.
[10:23 - 10:25] We need to save this too. And there it is.
[10:26 - 10:36] It's coming in the center because the container has a line center. So let's just put it out of the container.
[10:37 - 10:55] Yeah, that does it. And let's add some space so that looks better.
[10:56 - 11:01] So we have our image and it should work now. There.
[11:02 - 11:10] Yeah, that's the side menu. The drawer is actually just a screen.
[11:11 - 11:16] We could build anything on top of it. We could build a list of navigation menus we could build.
[11:17 - 11:22] Profile user settings. So yeah, it's very extensible that way.
[11:23 - 11:36] Next let's look at tab navigation. So if we just go to navigator and index and instead of doing show splash, let's just call the tab navigation.
[11:37 - 11:48] Now this is a method that we have defined that sets up tab navigation using reactive navigation. It's not something that comes out of the box.
[11:49 - 11:58] And if you just save it, our app now boots into a tab navigation. This is also great though I do recommend keeping a splash screen and then moving to a tab navigation.
[11:59 - 12:16] The reason for that is sometimes we need to fetch user data from an API or do some kind of loading from our async storage or SQLite and that may take a couple of seconds. Which doesn't seem long, but from a user expectation for an app two seconds is a long time.
[12:17 - 12:43] So the splash screen really helps in putting some kind of a placeholder like a Jeff or a small video that can keep the user busy and while the data loads and then we can move to a tab navigation. So if you look at the setup of tab navigation, it's quite similar to what we had for stack.
[12:44 - 12:59] So there's, so we are still using a side menu, but let's just, if you could ignore this for a second, there's the bottom tabs and then it has children. And now each children is actually a stack.
[13:00 - 13:10] So we just putting two tabs right here like home and settings. And each of them is a stack because on each screen we'll be able to push more screens.
[13:11 - 13:15] So we can push screens onto home tab, push screens onto settings tab. That's why we have a stack.
[13:16 - 13:22] And then it's all very similar to the earlier one. We pass in the component ID, this default prop.
[13:23 - 13:36] If you want to initialize a screen with store data, we can do that. Or if you want to pass props from a different screen, like when coming back from some screen to a parent screen, we can also do that.
[13:37 - 13:40] And these are some of the options like the top part. We want it to be visible.
[13:41 - 13:46] Do we want to draw behind it? And these are the bottom tab options that we see.
[13:47 - 13:56] So there's the font size, the text that we want and the text color that we want . Now if you will notice we are using the typography here.
[13:57 - 14:03] We're not using any hard coded hex strings. And if you want to display an icon, we can pass in the icon.
[14:04 - 14:11] Generally this is generally two sets of icons. One is for home and one is while it's active that's that selected.
[14:12 - 14:23] But we can also do that using these attributes, selected text color or selected icon color. And that way the tabs will get highlighted with whatever we pass.
[14:24 - 14:29] So like I said, we'll be building an e-commerce app. So let's add a couple more tabs to it.
[14:30 - 14:41] So we can simply copy one of these. And let's say that we'll have a search screen.
[14:42 - 14:56] But for now let's just pass the setting screen because we need to add that screen to our views and register it in the navigation. If you remember.
[14:57 - 14:59] So we need to register the screen. So we'll do that.
[15:00 - 15:11] But let's just add two more of these and call the third one search. Or let's call the second one search.
[15:12 - 15:24] Third one can be let's say card. And we'll have a fourth one for user profile.
[15:25 - 15:34] Let's call it settings and just save it and reload and there we have it. We have a home search card and settings.
[15:35 - 15:40] So right now they're sharing the screens, but we'll add these screens. That was easy, right?
[15:41 - 15:48] So we've already seen pushing a screen and it automatically provides a back button. But what if we want to go back manually?
[15:49 - 15:50] How to do that? So this is the dummy screen.
[15:51 - 15:56] Let's just open that dummy component. Let's add a button to it.
[15:57 - 16:08] Now we'll be using an element that we want to declare and it's one of the basic elements. We'll talk about elements and widgets in our design system lesson.
[16:09 - 16:41] But let's just say for now it's a default button that we have configured for our application with some default styling and the text we've passed is shown. So we'll just say title equals go back and on click we want to call this dot back navigation.
[16:42 - 16:50] So let's just declare that. We'll again do navigation.
[16:51 - 16:54] Anything related to navigation. Navigation dot.
[16:55 - 17:12] This time we do, last time we did push, now we do pop and we need to pass the component ID, this component is ID because we don't want to pop this, right? So now if we do go back, that works.
[17:13 - 17:27] Let's say that we have pushed three four screens on top of this base screen and we want to come back to not the root screen, but probably the screen one or screen two. So how do you do that?
[17:28 - 17:39] So in that case, you just pass the component ID of the screen that we want to move to. So let me just add a couple more screens and come back and show that.
[17:40 - 17:59] So I've added two more screens. Let's just call this dummy screen one and we will push the screen to have added dummy to dummy three.
[18:00 - 18:15] So let's just push navigation dot push. We'll pass the component ID.
[18:16 - 18:44] Come on. And sorry, the ID screens dot dummy two.
[18:45 - 19:08] And let's call it push two and we need to pass in the name, which is screen dot dummy to save it. So that pushes dummy to, but dummy to has a go back.
[19:09 - 19:30] Then just copy this that thing, go to dummy two and let's push me. So dummy and it needs a screen variable and tidy.
[19:31 - 19:35] Let's do that. It will push three.
[19:36 - 19:42] Now we are at three. Now we want to click this button and we want to go back to the first screen, dummy one.
[19:43 - 19:52] Now right now it's taking us to two, but we want to go to one. So all we need to do is let's just go to dummy three and this is the back one.
[19:53 - 20:15] So we'll just go to navigation and it has a method called Bob to and this will pass the ID of the first screen, the same ID that we called it with. So it was in home dot component and the component ID that we passed was this one, screen dot dummy.
[20:16 - 20:25] Let's just go to three. Let's say two screens dot dummy, save it.
[20:26 - 20:38] Now we have gone back to screen one. So this way we can control the navigation in a in a stack setup.
[20:39 - 20:48] So we can go to let's look at that again. We go to three and from here we go to one, but what if we wanted to go to base screen?
[20:49 - 20:58] Let's say that we have completed our journey and we don't really know how many screens are there. We just want to go back to the base one since the journey is done.
[20:59 - 21:04] How to do that? So for that is another method.
[21:05 - 21:32] Let's call Bob to root and it takes the component ID and let's just save it and you back to the base screen. Let's look at switching tabs.
[21:33 - 21:39] Users can always click on these, but what if we want to do that program matically? Let's say we have a card icon and we click on it.
[21:40 - 21:46] We want to go to the cart page. So let's just reuse this push button.
[21:47 - 22:01] So let's go to home component and on show push screen using this. We just need to call navigation method.
[22:02 - 22:07] Let's do navigation dot. We need to use merge options.
[22:08 - 22:38] Now this will take the component ID and we need to tell it that we need to switch to one of the bottom tabs and make the new index as. So it's called current tab index and we want to do it too and just save it.
[22:39 - 22:47] Now when we click this, it moves to the cart screen. So I've just we don't need the problem in you here.
[22:48 - 23:13] So I'll just add a cart icon and let's just make it cleaner. So the image on the left, let's go to the style and let's say alliance self flex and now this works because the parent container, the special opacity has a style called cart container and which already has display flex.
[23:14 - 23:20] So it's pretty similar to how CSS works. That's the beauty of react native to.
[23:21 - 23:42] So next let's on press. Let's create a function for this and we'll say show cart screen.
[23:43 - 23:46] We can copy this over here. The company ID.
[23:47 - 23:55] We can just copy it over and we'll say show cart screen. Just save it or not.
[23:56 - 24:01] Can this we are going to cut page. Okay.
[24:02 - 24:08] So one last thing. Let's look at models.
[24:09 - 24:18] Those are also components we can render any screen on it. So let's try that.
[24:19 - 24:37] Let's add another button here and it says show model and show model. So let's declare this.
[24:38 - 24:57] So now again, the navigation object, it has a method that's called show model and we need to pass something very similar to what we did navigator. We need to tell what we need to show so we can say if we want to show us that as we can see it supports stack, right?
[24:58 - 25:07] So it's very extensible that way we can show a single screen. We can show stack what that means is we can show a model and then again push screens inside the model.
[25:08 - 25:21] If the flow asks for it, right? Sorry, children will take an array and then we just pass the component that we want to show.
[25:22 - 25:39] Let's say we want to show screens.dummy2. And let's click it and there we have it, our model.
[25:40 - 25:46] Now since we said that this is a stack, the push and also should still work, right? So that's the beauty of it.
[25:47 - 25:55] And if you look at the animation, this is very native, right? The models in the native iOS apps, they behave this way.
[25:56 - 26:00] When we push the screen, it slides in and we will go back. So the animation is all very, very consistent.
[26:01 - 26:09] So let's look at we saw that we can dismiss the model by sliding this down. But what if you wanted to do that programmatically?
[26:10 - 26:37] So let's go to dummy2 and do that dummy2 component and instead of the go back, we say dismiss model and or let's just create a new button. It's quite simple.
[26:38 - 26:52] And let's do it right here. Navigation taught, we have business model, right?
[26:53 - 26:59] And then we just need to pass the component. Just save it.
[27:00 - 27:08] And once we click, the model is just a specific. So to recap, we saw how to do a stack.
[27:09 - 27:30] How to push screens, add a draw to it and a draw is a simple component, a screen. And then how to sort of a tab navigation, push screens, go back to a particular screen and or come back to the base screen and how to use models, how to dismiss them.
[27:31 - 27:37] There just one more option with models. There's also an overlay.
[27:38 - 27:46] So overlays are actually full screen. So whenever you use it, you have to be careful because it will not have that default option of dismissing it.
[27:47 - 27:53] So let's just do that. And so we need to have some kind of a strategy to go back to the previous screen.
[27:54 - 28:11] And there are a couple of other options with navigation for models. Like there's a dismiss all models and there's also a dismiss models, which we can use if we pass an array of model that we want to dismiss and similarly we have dismiss overlays.
[28:12 - 28:22] So that should cover the navigation of the app. For the purpose of our app, the e-commerce app will be doing a tab navigation and will continue building all this app.