Building The User Context
Learn how to build the user context for the event management application using shadcn/ui
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Sleek Next.JS Applications with shadcn/ui course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Sleek Next.JS Applications with shadcn/ui, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
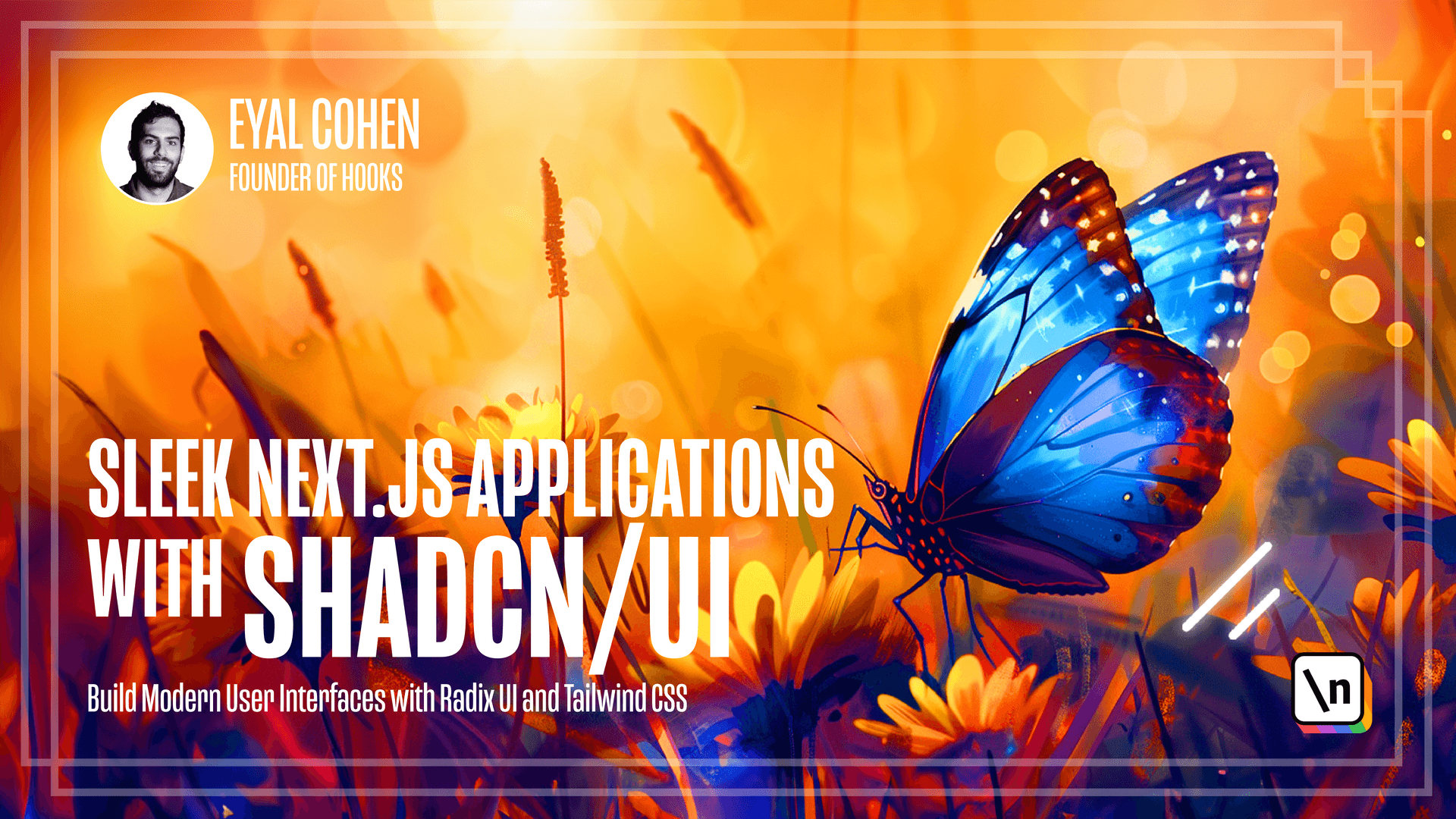
[00:00 - 00:18] In the previous module, you build a public-facing site of the event management application where users can view upcoming events. In this module, you will build a user authentication flow and a registration functionality, while learning about forms in shadcn/ui.
[00:19 - 00:28] Before users can register to the app, they need to sign up and sign in. To implement a complete authentication experience, you need a few things.
[00:29 - 01:08] A user context to all the user authentication status and to send login and register requests to the API, an authentication form to end the user input and send it to the user context, a login button to show the authentication form in a dialogue, and a conditional render inside the RSVP button to show the RSVP button if the user is logged in, and the login button if the user is logged out. To keep track of the user's indication state, you need to create a new React context called user context, navigate to the code editor, and create a new file in hooks called user context.
[01:09 - 01:42] Let's create the hooks folder as well. Create by declaring it as a client component because a context must be a client component, and then import form React object, React node, which is a type for the children , create context function, use effect, and the use date function, all from React.
[01:43 - 01:48] Then create a type for the user context state. Start with the user object, which is pretty simple.
[01:49 - 02:07] We have user name and email, and then the UserContextPropsState. It includes the user object, which is the name and the email.
[02:08 - 02:26] It should be nullable for logged out users, for logged out users, a token string to all the user authentication token. It's also nullable.
[02:27 - 03:33] A set user function, which is a React dispatch function, it receives a user. I set token function, that receives a string, a login function to make a network request to the server with the user input, so it receives the user, which is user name and password, and it returns a promise with either a void or a string, and the register function, which accepts the same parameters as the login one, but for creating a user.
[03:34 - 04:37] Now initiate the context with its initial state, so export const UserProvider the user context state, and define the initial user, initial token, and we can define all the function to be an empty function. Now create the provider component with the values implemented, so expert context, user provider, it stipies a React functional component that receives children, as React node, can pass you the procs with children, and it returns, and let's start defined the values.
[04:38 - 11:20] The first value is the user, the second is the token, which is a string, or null, both will be initiated with null, the login and register function are pretty similar, both take a user name and password with the input, and make a network request to the API to authenticate the user, let's write them, the login function, async function, that receives a user, which is a user name with a string and a password, we'll use the native fetch function, and the API returns, and the API provide us with a user/login route, then we want to pass the method, property, the headers should be content type, application JSON, and the body, which is a JSON of the user input provided here. I can usually make a reusable function out of this make requests function, but for now that's fine, because we only do it twice, and then I want to turn the terresp onse into a JSON, if we don't have the user, we'll return string, if we don't have a user property, on the data that returns from the request, we'll return the data message, this means that there was an error, and we want to show it to the user, and if there is, we'll want to call the set user function, and we also want to save the token, we don 't only want to set it to the state, we also want to save it, so let's create a new function called save token, with the token return from the API, it's a JWT, and the const, and the save token function will do two things, it receives a token of course, and the first thing it will do is set the token to the state, the second thing is to set the local storage item, set item, with new events token, and save the token there, now implement the register function, we can copy and paste the login, replace the requests with a simple user call, and then we want to return the, and then we want to render the user context provider with the value of the functions and the saved state, so return a user context dot provider, and make it value equal to user token, set user, set token, login and register, then we'll render anything inside which is the children, forgot to declare them, now there is one more thing we want the context to do, once the application is running we want to load the token from local storage, well we save it, we'll use the user effect for that with the empty dependency array, and then we'll call an init user function, let's create a init user function, it's an asynchronous function, the doesn't receive any problems, it loads the token from the storage , and it makes a request to the server to get the user, paste on the token that we saved to local storage, we send the token via the headers, through the authorization header, and we add the beware prefix, now turn the request into a JSON, and set the token if it returned, and set the user, great, we don't need to add it to the value because it's internal, the last piece of this context is the use user hook, which encapsulates the use context call to the user context, so let's export a user hook, if there is no context that means that we call the use user hook outside the provider, we haven't rendered the user provider, on top of the component that we are calling the use user hook in, so we'll draw an error, if there is a context we'll simply return it, now navigate to app layout, and import the user provider, then render it under the theme provider, so any component will be able to call the use user hook, in the next lesson you will check if the user is logged in when it tries to register for an event, if the user is not logged in you'll show them the login button instead, .