Building a News Site - Navigation
Create the page navigation for NEWSLY, a shadcn/ui news site
This lesson preview is part of the Sleek Next.JS Applications with shadcn/ui course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Sleek Next.JS Applications with shadcn/ui, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
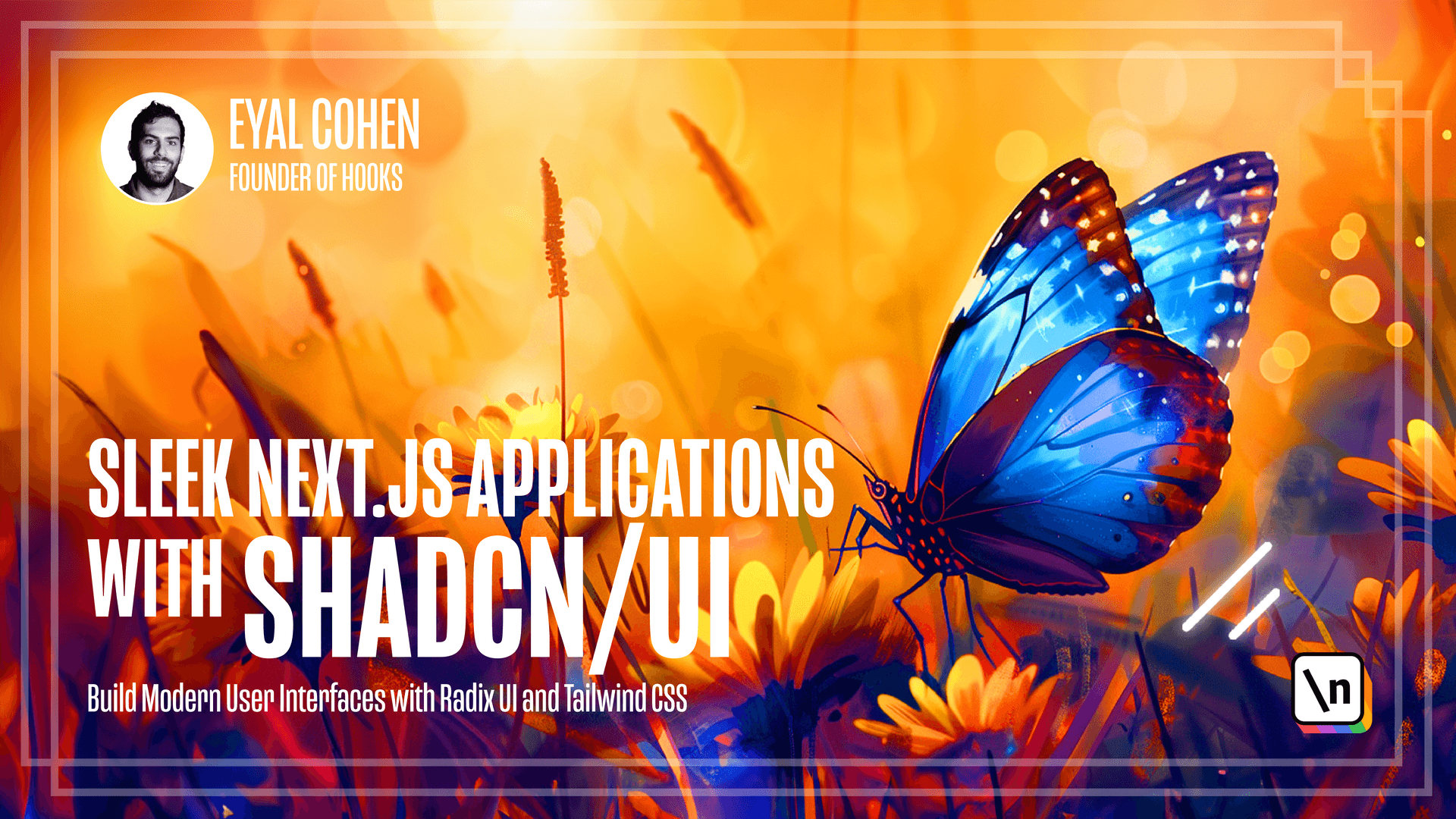
[00:00 - 00:11] In the previous lesson, you've created the site layout and the basics of the header. In this lesson, you will build a page navigation component using shadcn/ui navigation menu and separator components.
[00:12 - 00:19] Start by installing the navigation menu and separator using shadcn/ui CLI. Run this command in your favorite terminal.
[00:20 - 00:36] npx shadcn/ui@latest. Add navigation menu, separator. I already had it exist, but for you, it will just install them.
[00:37 - 00:49] The API you are going to fetch articles from is called newsapi.org, and it has a list of categories that you can use. You can now see them on my screen.
[00:50 - 01:00] The available categories are business entertainment, general health, science, sports and technology. Let's say them as navigation items on the site header.
[01:01 - 01:15] Create a new component inside the components folder called "categories". As mentioned before, you will use lucide.dev icons.
[01:16 - 01:26] You can see the icons on my screen. There are tons of them and they are very easy to use. And even more than that, the packages are already installed with the template you've used to create the app.
[01:27 - 01:44] So now add the following icon imports to your categories.tsx. Import BanknoteIcon, BikeIcon, CpuIcon, Flaskconical, and TvIcon from lucide-react.
[01:45 - 02:07] So const categories = an array label business, icon `BanknoteIcon`. The second one will be "entertainment" with the TV icon.
[02:08 - 02:33] You can feel free to copy and paste this array from the course text. And the last one will be "technology" with a CPU icon.
[02:34 - 02:44] The categories array is your data model for the categories component. Now import shadcn/ui navigation menu component and create a categories component.
[02:45 - 02:50] Let's start with the imports. We want the "Navigation" menu.
[02:51 - 03:11] We want to import that from "component/ui/navigation" menu. We want the "Navigation menu" component, the "Navigation menu" item component, the "Navigation menu" link, the "Navigation menu" list, and the "Last" and "Navigation menu" trigger style.
[03:12 - 03:25] Now create a new component. Don't forget to export it, "called categories". We want to return "Navigation menu" wrapper inside of it, the "Navigation menu" list.
[03:26 - 03:39] And then you want to loop through the categories array with just created and render "Navigation menu" item for each category. Create a wrapping div inside the "Navigation menu" item.
[03:40 - 04:17] And render the icon you've imported and render the icon of the category with the size 4, now add a span that will render the category label and add a text small medium className. You'd now want to see it live, so go back to the "site-header" file and render the categories component after the "H1" tag like this.
[04:18 - 04:29] Render the categories and the "H1" under the "H1". Try to navigate to there, don't forget to run it first.
[04:30 - 04:41] Again, if you have any issue running it, feel free to reach out on this curve. And you should probably see an "L" Next is drawing "L" as a "TUS".
[04:42 - 04:55] Now the problem is that the "Navigation menu" component of shadcn/ui is using a React context inside of it. And you can only use "Create context" inside the "Clite component" versus a " Server Side component".
[04:56 - 05:06] To make the categories component client side only, add the "Use client" line at the top. Now looking back at the site, your header should look similar to this.
[05:07 - 05:19] Now let's design the "Categories" button. You'd want to make the "icon and label" on the same row and "competalize the label" by adding "Flex". Now let's design the "Categories" button.
[05:20 - 05:32] You'd want to make the "icon and label" on the same row and "competalize the label" by adding the "Flex Item Center" justified between "Gap 2" and "Cappetal ize Class Names". So let's do it.
[05:33 - 05:46] Great. Let's see it live and they are now on the same row.
[05:47 - 05:58] The "Categories" button should also be clickable since it leads to a category page. Now shadcn/ui offers a spatial navigation menu link component that plays nicely with the "Next Link" component.
[05:59 - 06:08] So add an "Import" to the "Next Link" component first. Add an "Import" to the "CN" function as well.
[06:09 - 06:24] Now you'd want to end the link inside the "Navigation" menu item. So, wrap the inner div with the link, pass it an "Href" which is a dynamic link to the "Slash" category.Label.
[06:25 - 06:39] Add a "Pass, Href and Legacy Behavior" props to it. Now under the "Link", add the NavigationMenuLink components shadcn/ui offers .
[06:40 - 06:47] To the NavigationMenuLink, pass to the "Class Name". And now you want to pass a "CN" function which will combine the "Class Names" as you remember.
[06:48 - 07:03] With the "Navigation" menu trigger style, which is a spatial function from "Sh ud CNI", you can see the source code of "Itin" inside the "Navigation" menu component. And let's pass our own "Minor Design" adoption.
[07:04 - 07:26] So, in "Minor" settings on the side, we want to make a small transition on "Av ver" which will make the "Background Transparent" and will make the text with an " Deep Orange" color. Great, now don't forget to close the "Navigation" menu link and wrap them both.
[07:27 - 07:42] Great, now the category item should look something similar to this. You can see that on hover, the "Background Transparent" and the text becomes a "Deep Orange".
[07:43 - 07:52] Now let's add a "Separator" that you can see it on the new side and design. Add a "Separator", start by importing it.
[07:53 - 08:04] Now add it between the menu Items. Render it after the "Categories" icon and the label inside the "Item" div.
[08:05 - 08:13] So, the "Separator" is "Even Orientation" prop which you want to make vertical. And then we want to design it a little bit.
[08:14 - 08:21] So, we want it to have a margin left of 4. And height of 8, we want to rotate the angle a bit, so 45.
[08:22 - 08:33] And we want to make it with a bg-orange-700. Great, now don't forget to hide a "Separator" for the last item.
[08:34 - 08:48] We can do so by wrapping the "Separator" in a condition. So, if the index is equal to categories dot length minus 1 We don't want to render anything, but if it doesn't, we do want to render the " Separator".
[08:49 - 08:54] Great, let's check it out. Perfect.
[08:55 - 09:06] Right now, the categories and header are being rendered under the logo. On Desktop, the header should be one row with categories on the right, as you can see on the designs.
[09:07 - 09:24] Let's add a flex-row class name to the header Go to the site-header file, locate the header, and add to it at the beginning a "Flex" with full "Flex" column, "Item Center" and "Justify" between components. That's for "Mobile".
[09:25 - 09:41] For "Desc" top, we want to make it a "Flex" row and add a small border at the bottom of the component. Now, on "Mobile", there's an "issue" though.
[09:42 - 09:48] "Categories" will be wider than the screen width. They will overflow. You can see it here.
[09:49 - 10:00] The "Technology" component is overflowing the content, and the "Business" component is almost invisible. shadcn/ui offers a scroll area component that enters this exact case.
[10:01 - 10:11] The "Component" augments negative scroll functionality for "Castum" "Gos" "Browso" "Stining". “So, let’s install the Scroll Area component from shadcn/ui.
[10:12 - 10:28] Now wrap the categories component in Scroll Area in the site-header file. First, add an input And wrap the "Categories" with the scroll-area.
[10:29 - 10:38] You want to add a "Class Name", which is no "nowrap" for the "whitespace" property. And you also want to add the Scroll Bar because the Scroll Area doesn’t work without the Scroll Bar.
[10:39 - 10:49] without the scroll bar. You want the orientation to be horizontal. And we want to hide it on larger screens because we don't want it to scroll there.
[10:50 - 11:05] Now, you also need to edit the Navigation Menu wrapper inside the Categories component. You want to add a max-width first We will make it 95 VW, which is the screen width.
[11:06 - 11:16] And then we want to add a "Flex", "Item Start" and "Justify Start Class Name" with "Dweet". Now the categories should be scrollable and won't overflow.
[11:17 - 11:28] Perfect. Great work. One thing we need to fix is that the site-header component also needs a "Use Client" at the top of it.
[11:29 - 11:39] So, don't forget to edit. And we have another arrow that we can see on the terminal, which is that we forgot to add a key to the "Categories" item, the Navigation menu item.
[11:40 - 11:47] So, you want to pass the "Categories" label into the Navigation menu item. And now the code compiles without an arrow.
[11:48 - 11:53] In the next lesson, you will learn how to fetch articles from the API and render them on the page.