How to Read NFC Tags and Write NDEF Data With NfcManager
This lesson preview is part of the The newline Guide to NFCs with React Native course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to NFCs with React Native, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
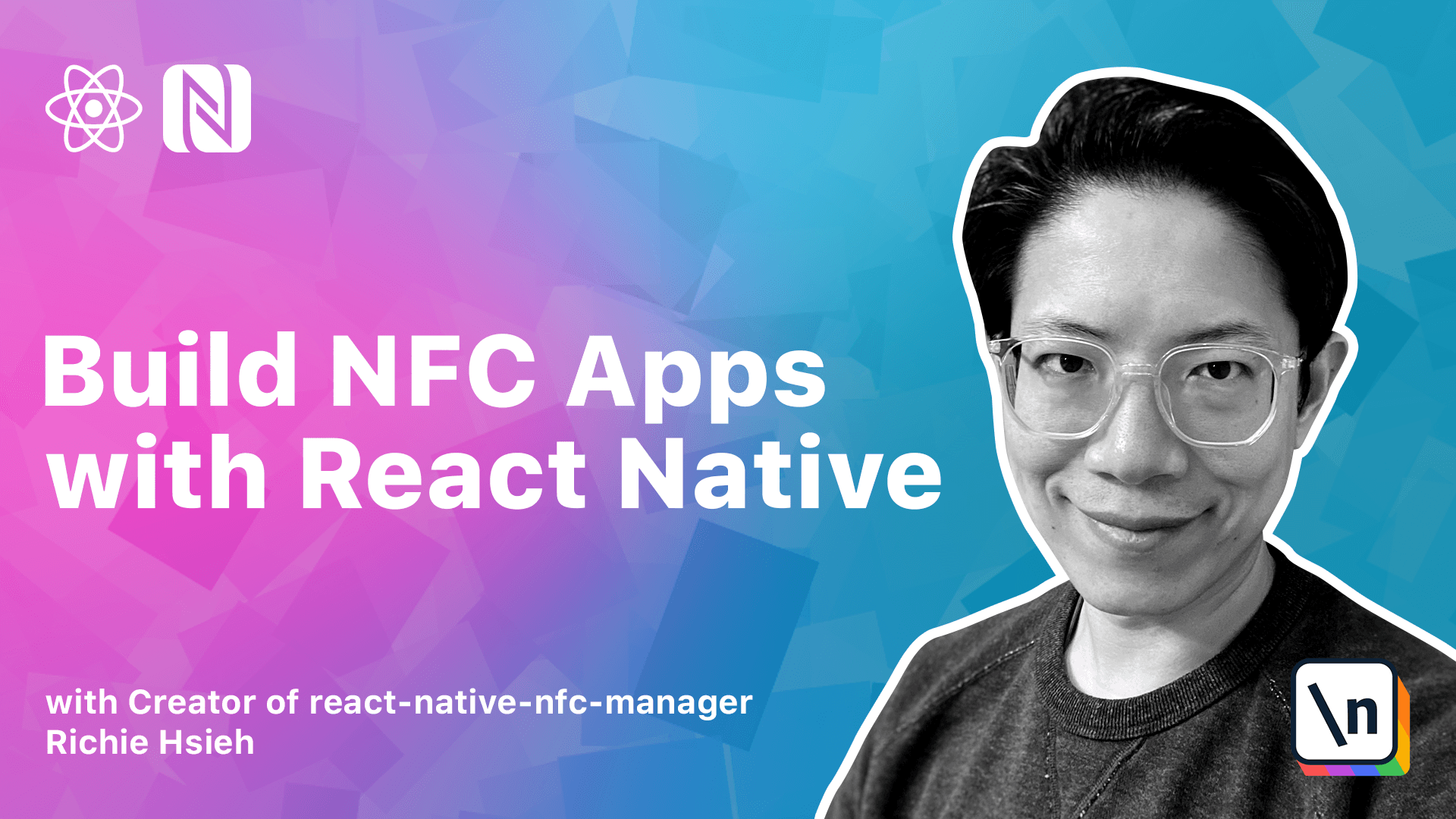
[00:00 - 00:25] Hello! In this lesson, we will focus on how to deal with n-death, covering, reading and parsing the n-death from NFC tags, building and writing n-death into NFC tags. Before handling n-death, let's first add some codes to check the NFC feature availability.
[00:26 - 00:39] First, open app.js from the previous Tech Countergame app. Paste the import NFC manager line back to our home screen.
[00:40 - 00:55] Then, migrate the use effect hook. So now we have the has NFC state to check if the NFC feature is available.
[00:56 - 01:06] And the enable state to check if the NFC is enabled. After that, we create a render NFC buttons function.
[01:07 - 01:26] And paste the original UI code into it. Then we go back to Tech Countergames app.js and copy the conditional rendering codes into our render NFC buttons function.
[01:27 - 01:38] Oh, it looks like we forgot to import touchable opacity. Save and refresh.
[01:39 - 01:58] We can see our simulator says, "Your device doesn't support NFC", which is expected. The next step is, of course, to scan our NFC tags.
[01:59 - 02:14] Remember, in our previous Tech Countergame app, we use register tag event and set event listener to scan our tags. Here, we will use a different approach.
[02:15 - 02:32] Let's call NFC manager.request_technology. As you can see from the type hint, the first parameter is called tag, which is the technology type we'd like to request.
[02:33 - 02:51] And n-depth itself is also an NFC technology type. In order to use the correct tag type, we should import NFC tag from our library .
[02:52 - 03:13] Once this API is resolved, it means the NFC tag with our expected technology has been discovered. So at this point, we can call NFC manager.get tag to retrieve the NFC tag object.
[03:14 - 03:39] If the NFC tag contains valid n-depth data, there will be a n-depth message property inside the tag object, as we saw before. After scanning, we will have to call NFC manager.cancel_technology request to clean up.
[03:40 - 04:01] Then what we'd like to do is to pass the tag object as parameters into the tag detail screen. And we can accomplish this by using the second parameter of the navigation.nav igate function.
[04:02 - 04:13] We also wrap our code in a tri-cache block to prevent unexpected errors. In this case, no critical exceptions will be thrown.
[04:14 - 04:44] So we can simply ignore them. We also move the cancel_technology API into the finally block, because it is the clean up operation and should always be called.
[04:45 - 04:57] Then call the read_end-depth function from our tag button. Let's quickly see our read_end-depth function again.
[04:58 - 05:20] This request_technology pattern will be our primary method for NFC tag scanning for the rest of the course. Here, this API is an async function and only resolves when the tag is discovered.
[05:21 - 05:35] Before testing, we still need to update our tag detail screen so that it can display the tag object we pass. First, destructor the route from props.
[05:36 - 06:02] And the data we are looking for will be in the route.parameters. For now, we simply wrap the stringified tag object inside a text element.
[06:03 - 06:13] This works pretty well. It's time to actually parse the end-depth.
[06:14 - 06:29] Import end-depth from React Native NFC Manager and declare a variable called ui . We first check if the tag object contains only valid end-depth record.
[06:30 - 06:53] Call from our previous lesson, end-depth message is a container which consists of multiple end-depth records. So the end-depth message property here will be an array and each element of this array is an end-depth record.
[06:54 - 07:05] To simplify things, we only handle the first end-depth record here. By the way, it's true for most cases.
[07:06 - 07:24] Then since we'd like to parse the ui, the tnf property should be well-known and the type property should be RTD ui. The tnf comparison is straightforward.
[07:25 - 07:42] But for the type property, we will have to perform array comparison since it's actually an array of bytes. Now we have confirmed this record is a RTD ui.
[07:43 - 07:59] We can decode the payload property via end-depth.uri.deco payload. We also need to update our render logic to display the parsed ui.
[08:00 - 08:23] Let's give it a try. Besides showing the parsed ui, we can use linking from RAN native to ask our operating system to perform corresponding actions according to our ui.
[08:24 - 08:38] We first wrap the text element inside a touchable opacity. For the unpressed handler, we simply call linking.open URL.
[08:39 - 08:49] Let's test it again. It's worked pretty well.
[08:50 - 09:08] Let's create a new screen called end-depth write screen. I will paste the ui code directly to save you some time.
[09:09 - 09:17] Here we have two states. One is selected link type and another is value.
[09:18 - 09:34] We iterate through web, tell, SMS and email. Set unpressed handler for each of them so that it can update the corresponding state into selected link type.
[09:35 - 09:54] We also have a text input to update the value state. Next, let's quickly hook up this new screen into our app navigator.
[09:55 - 10:27] And trigger the navigation from whole screen into end-depth write screen when the user clicks the link button. Let's give you a try.
[10:28 - 10:42] Now it's time to transform the user input data into a valid end-depth message. Again, import end-depth from native NFC manager.
[10:43 - 11:08] Add a skin to the correct value according to selected link type. Then create our ui record via end-depth.ui record.
[11:09 - 11:24] This function receives a ui stream and returns an end-depth record object. Next, we need to generate the end-depth message from the end-depth record.
[11:25 - 11:37] This can be accomplished by calling end-depth.encode message. The input is an array of end-depth record objects.
[11:38 - 11:49] And the output is an array of bytes, which represents the entire end-depth message. Let's observe the data now.
[11:50 - 12:26] Let's decode the first byte to see if it matches the end-depth record structure we just learned from the previous lesson. 2009 means D1 in Hacks and 1101, 0001 in binary.
[12:27 - 12:46] Once it is in the binary form, we can easily find the meaning for each bit by mapping it to the table here. So mb and me means it's the first and only end-depth record in current end- depth message.
[12:47 - 13:01] Then cf or chunk flag, we don't care about it. For the sr1 means it is a short record and tnf1 means it is a well-known record .
[13:02 - 13:20] Now I hope you get the feeling of what happened under the hood. The last part is to actually write end-depth into our tag.
[13:21 - 13:41] So import nfc-manager and nfc-tag from native NFC-manager. And we will use the same request-technology API and select end-depth as the expected NFC-technology as before.
[13:42 - 13:58] Then we use nfc-manager.end-depth-handler.write-end-depth-message to actually write end-depth into tags. This is the first time we have used an nfc-technology-handler.
[13:59 - 14:14] In our nfc-library, the technology-specific operations are grouped into their own handler. So the write-end-depth-message belongs to n-depth-handler.
[14:15 - 14:33] You might wonder why we don't need to use n-depth-handler when we read the nfc- tags. That is because the operating system will discover n-depth-message automatically, regardless of their underlying nfc-technology.
[14:34 - 14:54] So we can later retrieve it using get-tag API. Finally we call cancel-technology-request and wrap the code in a try-cache block like before.
[14:55 - 15:11] Let's test it. It's worked pretty well.
[15:12 - 15:23] Now we have both the read and write functionalities. Let's check if other URL type works.
[15:24 - 15:41] First write. Then read it back.
[15:42 - 16:03] Write. Read it back again.
[16:04 - 16:20] Write. Read it back again.
[16:21 - 16:41] Now it's time for our Android app. The only thing we need to do is integrate our previous Android prompt component into tap and go app.
[16:42 - 16:53] First make a src-components-directory and create a file name and write prompt inside it. Then copy our previous code into this file.
[16:54 - 17:09] Back to our home screen. Import the Android prompt component.
[17:10 - 17:28] Create a ref called Android prompt ref via react.use ref. Enter the component and pass ref and un-cancel-press into it.
[17:29 - 17:51] Inside un-cancel-press we simply call nfc-manager.cancel-technology-request. Finally in our read_m-death function we should set Android prompt to be visible in the beginning and set it back to invisible in the end.
[17:52 - 18:06] And of course we should only show this prompt on the Android platform. Okay, home screen is good to go.
[18:07 - 18:14] I will leave the end-death write screen to you as an exercise. (swish) (swish)