How to Build Tests For React Button Components
This lesson preview is part of the The newline Guide to Building a Company Component Library course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Building a Company Component Library, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
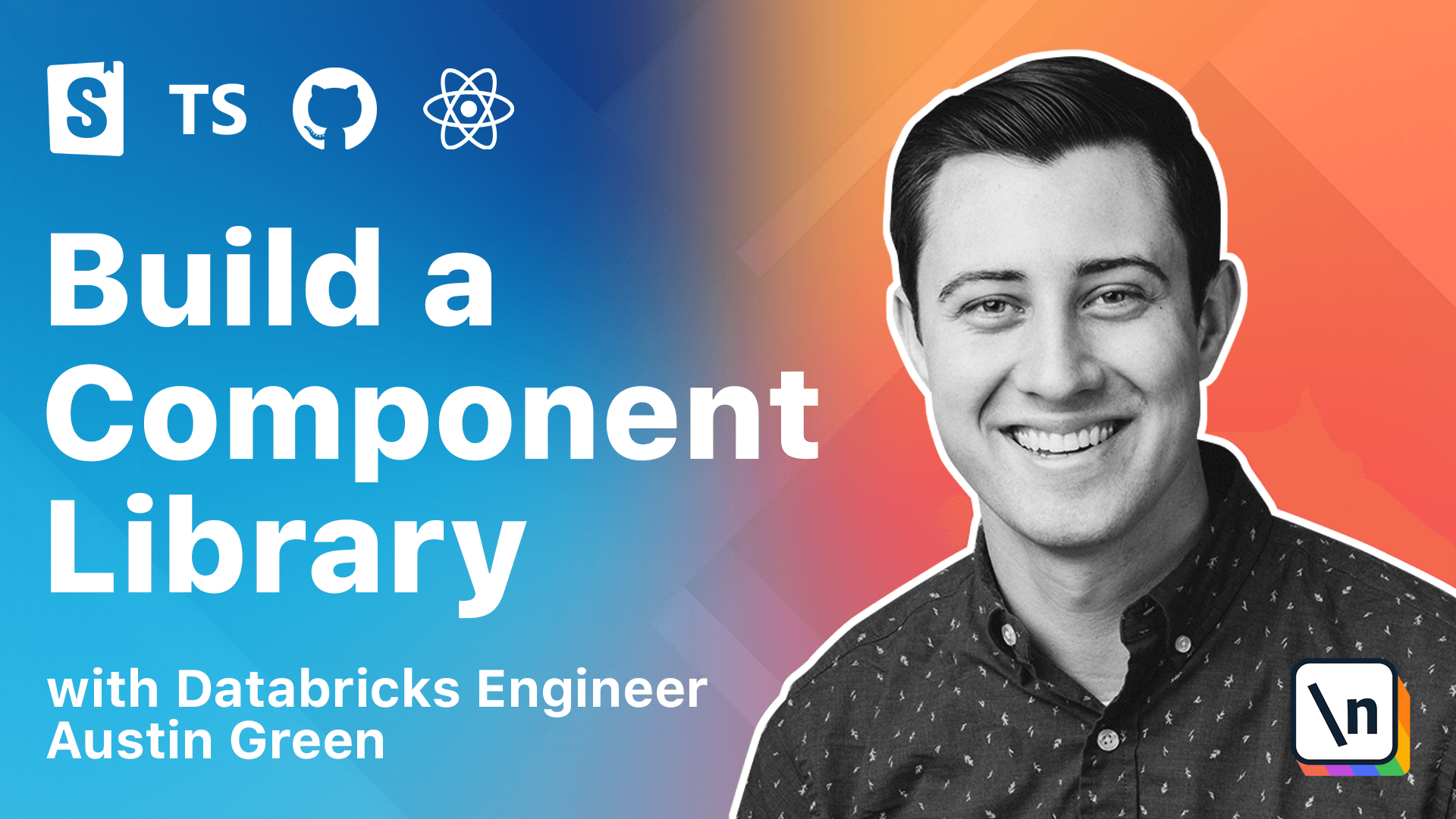
[00:00 - 00:20] Even though our button component has a simple API, there are several features that we can test. Some possible test cases include making sure that the component defaults the type attribute to button, if it allows consumers to override that default type, and if the button allows all ValaProst to be spread onto the underlying button element.
[00:21 - 00:31] In this course, we will be avoiding testing any of the individual styles of our components. These types of tests can become rigid and hard to maintain over time.
[00:32 - 00:50] Without rendering these components in a real browser and visually checking the styles, we are unable to assert that the styling is fully correct. There are several testing methodologies and tools like snapshot testing and component image snapshots that will help with style assertions if that's something that you would like to pursue.
[00:51 - 01:00] To start, let's create a new spec file for our button. If we go to source buttons, we can create a new button.spec.tsx.
[01:01 - 01:15] We are going to create a new describe block for our button. This is going to include three separate tests within it.
[01:16 - 01:38] The first one, we are going to test if it applies the default type of button. We are also going to check if it applies the specific type if provided.
[01:39 - 01:55] We are also going to ensure that it applies a valid attribute to the element. To start, let's create a new import react from React.
[01:56 - 02:09] We are going to be using two utilities from @Testilibrary react here. That is going to be render and screen.
[02:10 - 02:27] We are going to see some errors here while there are some empty assertions. Let's start by calling npm run test watch.
[02:28 - 02:38] This is going to start just in watch mode to watch as we change the testing files. Because we are not asserting anything, everything is passing.
[02:39 - 02:54] Our first test, we are going to be rendering a simple button implementation. We can do that by calling render and then directly calling our button with some sample text like holo.
[02:55 - 03:07] Once this component is rendered, we are going to be able to retrieve that underlying button element using the getByRollUtility method. There are a variety of queries that we can use for treating elements with React testing library.
[03:08 - 03:19] Each of them are designed to make our test as flexible and semantically correct as possible. What we want to do is ensure that the type attribute on our button is equal to button.
[03:20 - 03:31] We want to expect that the screen has an element with a roll of button inside of it. That will return that HTML button.
[03:32 - 03:45] We want to expect that to have an attribute of type that is equal to button. That test should pass.
[03:46 - 04:05] Next, we want to have a similar test, but we want to ensure that a custom type is able to override that. We can override this with a custom button type.
[04:06 - 04:16] It has an attribute of type submit. Let's see if that test pass.
[04:17 - 04:31] For this last one, we want to assert that any valid attribute is spread onto the button element. A common one for this could be, let's maybe try something like a hardware label , for example.
[04:32 - 04:39] Our label is a valid attribute for button. Let's include the value test.
[04:40 - 05:01] We want to ensure that the button within the screen has an attribute of ARIA l oping that is equal to test. Perfect.
[05:02 - 05:12] Now we have three tests that cover all of the custom functionality that we've included in the button component. In the next lesson, we're going to be creating a new test suite for our fuel component.