How to Interact with Smart Contracts on Ethereum Blockchain
In this video, we'll write our first Solidity smart contract
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Now that we know we have a connection between our browser and our Ethereum node Let's make a connection to our contract
Remember that in order to create a JavaScript representation of our contract, we need to load the abi
Let's copy the abi that we compiled earlier into this project. Only I'm going to rename it to counter_sol_Counter_abi.json
In the code, we can require this .json file -- this is possible because of the nwb config, if you're building using another method you could always just copy and paste the abi here in the code t counterAbi = require('./counter_sol_Counter_abi.json');
We can log it out and make sure it loaded. Great nsole.log(counterAbi);
Also remember that our particular instance of the counter contract lives at a specific address on the blockchain. I hope you saved the contract address from last time.
let contractAddr = '0x4575e7fcf12a110060ebc1e3b2b85706ddfbc97e';
This lesson preview is part of the Intro to Programming Ethereum ĐApps course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Intro to Programming Ethereum ĐApps, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
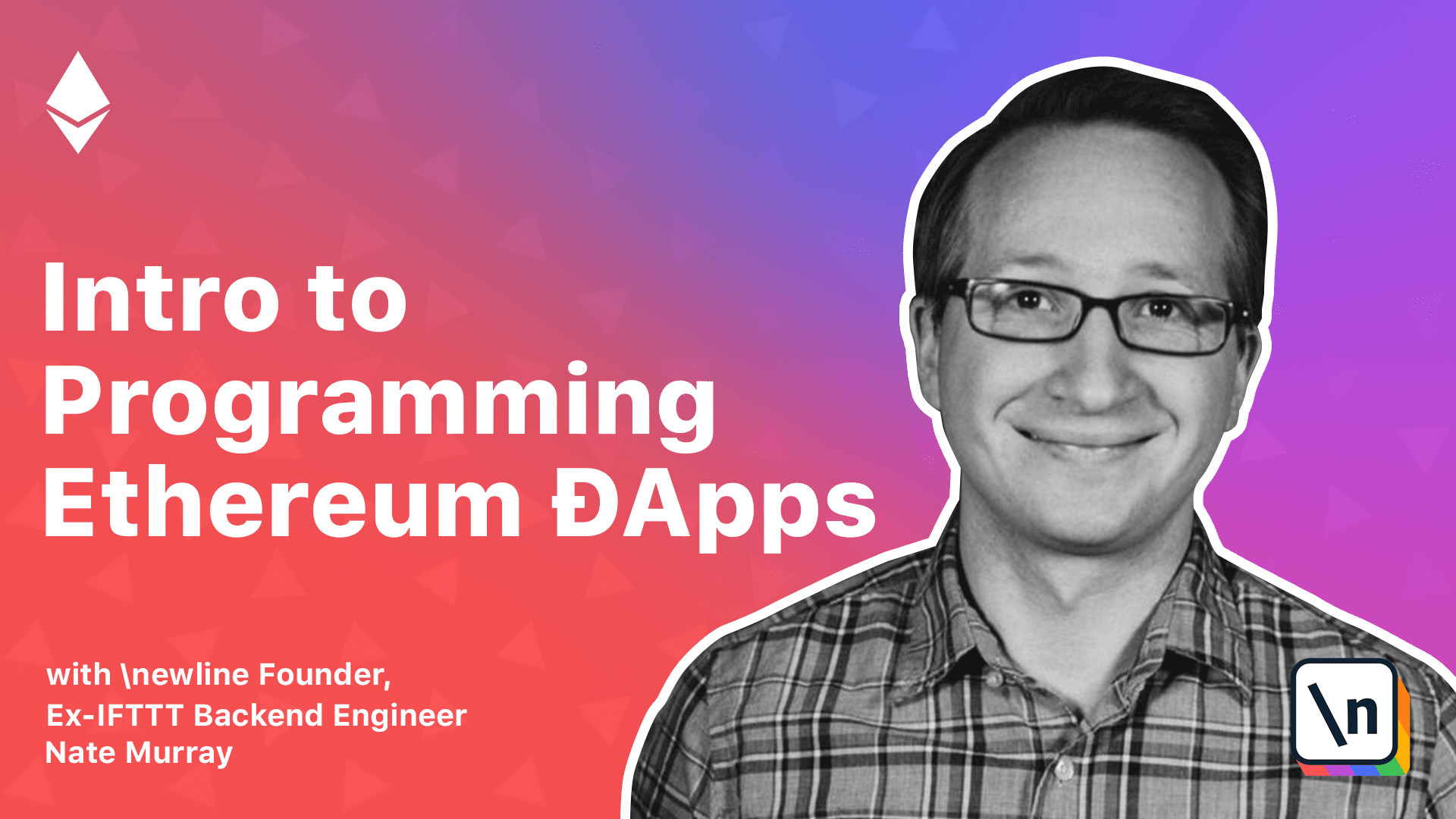
[00:00 - 00:52] Now that we know that we have a connection between our browser and our Ethereum node, let's make a connection to our contract. Remember that in order to create a JavaScript representation of our contract, we need to load the ABI. Let's copy the ABI that we compiled earlier into this project. Only I'm going to rename it tab.json at the end, so I'm going to rename it to counter_soul_counter_abi.json. In the code, we can use a JavaScript require to load this .json file. This is possible because of the NWB config. If you're building using another method, you can always just copy and paste the ABI here in the code. I'm going to type let counter_abi equal require, and I'm going to require the file. We can log it out to make sure it loaded. See it in the log? Great.
[00:53 - 01:47] Also remember that our particular instance of the counter contract lives at a specific address on the blockchain. I hope you saved the contract address from last time. I'll copy that address, and I'm going to define a variable, let contract address equal, and then paste the hash in. And remember, this is an important point. When you deploy your website, it lives on a specific domain name. When you deploy your contracts, they'll live at a specific address. So you'll have these addresses hard-coded, or at least in a config file, committed into your code. So now that we have our ABI and our contract address, we can create a new contract object. We do that by saying var counter contract equals new web3.eth contract. We pass the two arguments counter_abi and contract address. Let's assign this to window so that we can inspect it in our console.
[01:48 - 02:49] So looking at counter contract, notice that the functions that we want to call are in methods on this object. In order to get the value of our counter, we have counter contract dot methods dot get. But notice here there are two important functions on get send and call. There's a difference between them. We use call when we want to call a function locally and send when we want to send a transaction to the network. Getting the value of the counter can be done locally, so let's use dot call. However, calling out to our Ethereum node is async, so dot call will return a promise. So we call counter contract dot methods dot get dot call dot then and we take the count and we'll log out the count.
[02:50 - 03:04] Great, we got our count. Now let's add a function for this and show the value in our view. First thing we'll do is we'll add a div and placeholder to show our counter value and we'll update our button to call check counter.
[03:05 - 03:32] Let's define our check counter function. First we'll type window dot check counter equals function. Then on the contract, we'll call counter contract dot methods dot get dot call dot then, which will be given account. Then we find the counter value element by ID.
[03:33 - 03:39] And we set the inner text equal to the count. Flip back to our browser and let's try it out.
[03:40 - 03:58] Great, it works. The next thing that we need to do is send a transaction from our browser to mut ate that counter value. Now let's mutate our contract state by incrementing the counter.
[03:59 - 04:09] To do this, we'll need to send a transaction to the blockchain. We can check this out in our console. The counter contract object has functions that help us build this transaction.
[04:10 - 04:29] It looks like this. Counter contract dot methods dot increment. We'll call dot send on this. Dot send means send a transaction. So recall that to send a transaction, we need to specify the sending account as well as the amount of gas that we're willing to spend to run this transaction.
[04:30 - 04:41] Let's grab our first account address and try it. We'll set our account to a variable.
[04:42 - 05:00] And then call counter contract dot methods dot increment dot send passing from our account. Gas will just put one million dot then and capture the error and response and log it out.
[05:01 - 05:14] Hmm, the promise that returns isn't resolving. Let's check our logs to see if we can figure out why. In the network tab, we can see the response that says authentication needed, password or unlock.
[05:15 - 05:29] We forgot to unlock our account. So let's do that first. We'll type web three dot ETH dot personal dot unlock account pass our account address and then the password ABC 123. Looks good. Now let's start re-setting our increment transaction.
[05:30 - 05:39] Looking at the network tab, it looks like it went through. Let's mine this transaction. That can get we'll do minor dot start.
[05:40 - 05:49] Got our transaction and minor dot stop. Let's check our counter again.
[05:50 - 06:02] It works. All right, let's add a button which will increment this counter for us. We'll set our from account.
[06:03 - 06:26] So we're going to add a new button where on click calls increment counter. And let's define our increment counter function.
[06:27 - 06:50] We'll call window dot increment counter equals function counter contract dot methods dot increment dot send from from account gas one million. And let's look back over to our browser and press the increment counter button. And we need to mine the transaction. Now I don't know about you, but I'm getting tired of starting and stopping the minor manually.
[06:51 - 06:58] So let's just leave the minor running. Now let's increment the counter. And if it doesn't work, you might need to unlock your account again.
[06:59 - 07:09] Let's wait a couple seconds for it to be mined. Then check the counter value. It works. Let's try that again. Wait for the minor.
[07:10 - 07:26] Click it again. It works. Fantastic. So there's a really basic web three Ethereum tutorial. I think the most obvious improvement I'd like to make here is to have the counter value automatically update in the view once the transaction has been mined.
[07:27 - 08:56] We'd use web sockets to push the new information from the RPC server to the browser. And then we could update this value automatically without the user having to check manually . But using web sockets is something we'll cover in a later video. In fact, we have a lot to cover in other videos. This tutorial shows you the key connection and how to build D apps. But there is so, so much more to cover. For example, I want to talk about the specifics of writing real world solidity contracts, taking performance and security into account. I want to talk about building apps where our users can trade collectibles or vote on resolutions and make provably fair agreements. I want to go beyond Ethereum and talk about distributed storage with IPFS and talk about high throughput transactions with sidechains and state channels. I want to talk about how to accept Bitcoin payments on your website without any bank or credit card intermediary. And of course, we're going to talk about how to build your own cryptocurrency. We'll discuss what it means to create your own tokens and how to integrate them into your own apps. For now though, let's celebrate our success connecting our Ethereum network to a browser app. We're entering a brand new world and we've got a lot to build. (upbeat music) (ambient music)