How to Connect to Ethereum with Web3.js From JavaScript
In this video, we'll write our first Solidity smart contract
In the last video, we built a simple counter contract in Solidity, we deployed it to the blockchain, and called methods on it to update the state.
There's a lot more we have to learn about Solidity and smart contract programming, but I want to show how to make a connection all the way to our web browser. That is, I want to make a user interface for this contract.
I think it's helpful to see how an ethereum-based app is done end-to-end and then we'll go back and learn how to make more sophisticated apps.
To build a UI for our Ethereum app, the key idea is this: our Ethereum node has a JSON API and we will connect our browser to that API.
So the core architecture is that we have an HTML file, which loads JavaScript. And this JavaScript, instead of calling out to a say, "normal", "centralized" server, we'll call to our personally controlled Ethereum node instead.
This lesson preview is part of the Intro to Programming Ethereum ĐApps course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Intro to Programming Ethereum ĐApps, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
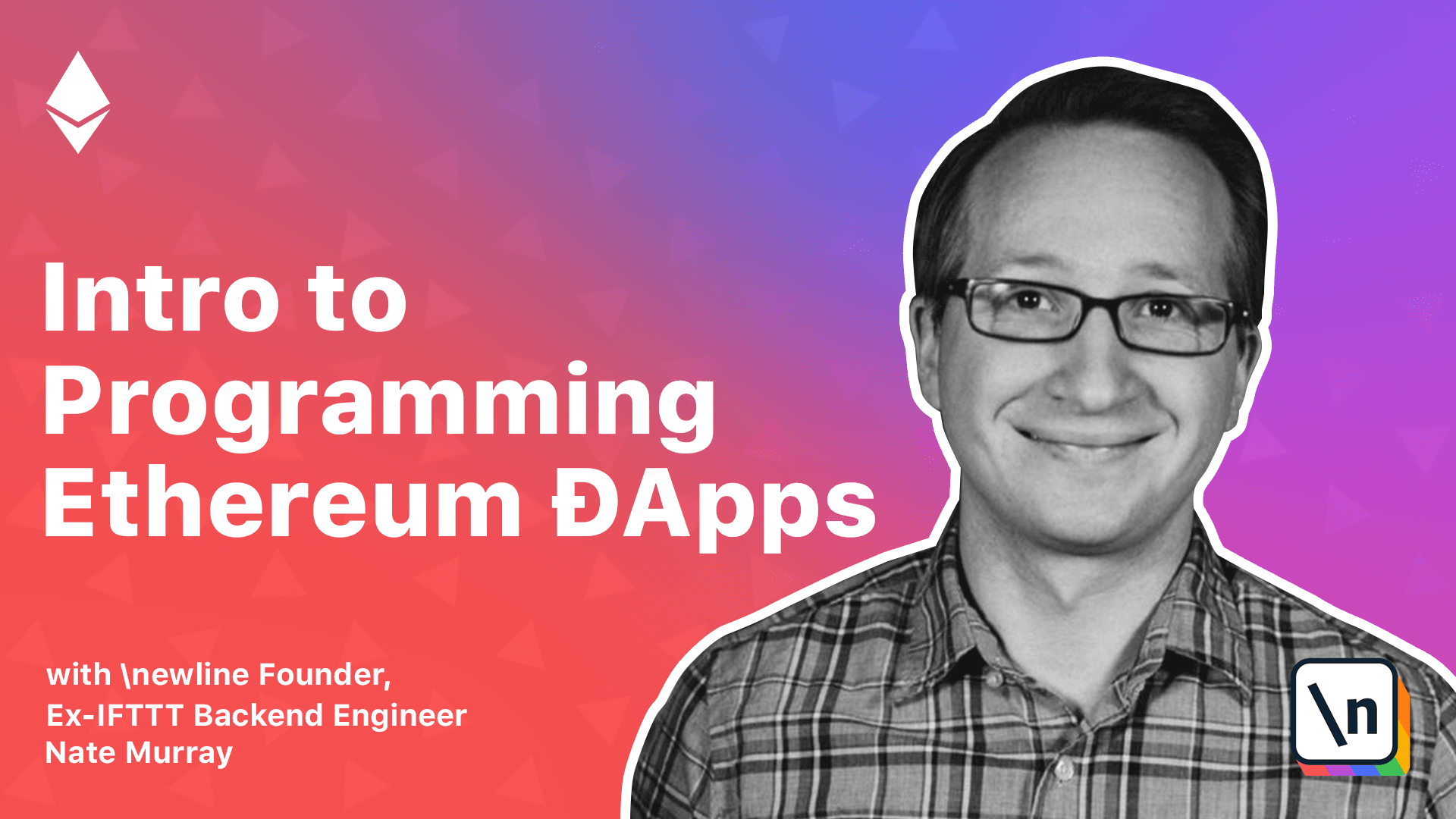
[00:00 - 00:07] In the last video, we built a simple counter contract in Solidity. We deployed it to the blockchain and called methods on it to update the state.
[00:08 - 00:18] There's a lot more we have to learn about Solidity and Smart Contract programming, but I want to show how to make a connection all the way to our web browser. That is, I want to make a user interface for this contract.
[00:19 - 00:29] I think it's helpful to see how an Ethereum-based app is done end to end, and then we'll go back and learn how to make more sophisticated apps. To build the UI for our Ethereum app, the key idea is this.
[00:30 - 00:50] Our Ethereum node has a JSON API, and we'll connect our browser to that API. So the core architecture is that we have an HTML file, which loads JavaScript, and this JavaScript instead of calling out to say a normal centralized server, we'll call to our personally controlled Ethereum node instead.
[00:51 - 01:01] Now, just to be clear, our web app is all just JavaScript. So you can call out to other servers if you want to, just like you can call lots of APIs in a normal JavaScript app.
[01:02 - 01:09] In fact, this really is just a normal JavaScript app. The only difference is that we're connecting to an Ethereum node for the API.
[01:10 - 01:22] The library that we used connect our JavaScript code to the Ethereum JSON API is called Web3. To build our UI, we need to start with a basic HTML, CSS, JavaScript boiler plate.
[01:23 - 01:26] I'm not going to teach web development here. We have other books and courses if you want to learn that.
[01:27 - 01:41] Instead, I'm going to assume that you know how to write JavaScript, install npm modules, and use promises. In a real app, I'd probably use a web framework like Angular or React, but I want to focus on what's new here, and so vanilla JavaScript will be fine.
[01:42 - 01:57] I also want to warn you that in this tutorial, we're going to take some JavaScript shortcuts, like creating global variables on Windows and directly manipulating the DOM. We'll cover how to properly structure larger Web3 apps later, but for now, let's just get it working.
[01:58 - 02:12] Everyone has their own favorite boilerplate, and I'm going to use NWB here, because it's an easy way to get a nice vanilla JavaScript app up and running. So we can install it first by doing npm install-g nwb.
[02:13 - 02:42] NWB new web app HelloCounter, then I'll cd into HelloCounter, do an npm install , and then I'm going to npm install Web3. We can start our server using npm start, and then open localhost 3000 in our browser.
[02:43 - 02:55] A great thing about this configuration is that it will automatically reload our app whenever we change these files. We can open up the code and see that the basic app we're given has an index.
[02:56 - 03:05] html and an index.js. The first thing we'll do is require Web3.
[03:06 - 03:18] And the way the Web3 API works is we're going to call new on this object, so we'll declare a lowercase Web3 equals new Web3. And so we can expose variables to our console by setting them on window.
[03:19 - 03:27] So let's make Web3 accessible to our browser console by typing window.web3 equals Web3. The next thing to do is to set the Web3 provider.
[03:28 - 03:41] In this case, we're just going to use the HTTP provider, but you could also use WebSockets if you wanted to get real-time updates. And notice that we're setting the address to localhost port 8545.
[03:42 - 03:50] This address is the address of our Geth RPC server. We haven't booted Geth in an RPC mode yet, so let's do that now.
[03:51 - 04:01] Flip back over to our Geth shell, kill it using ctrl C, and let's start it with new options. So I'll use bin_geth_datadir.
[04:02 - 04:13] I'm going to set the options, no discover, RPC, RPC API, and then a list of RPC APIs that we want to use, db_personal_eth_netweb3. There's several, these are the ones we'll use for now.
[04:14 - 04:25] And then there's a few other options, RPC cores domain, you don't need to worry about that for now. RPC address, I'll put localhost, RPC port 8545, and console.
[04:26 - 04:32] You can find this full command in the lesson notes. Feel free to adjust the RPC address or RPC port if you need to.
[04:33 - 04:41] For example, you might try using 0.0.0.0 for the RPC address if localhost doesn 't work on your machine. You might also need to change the RPC port.
[04:42 - 04:53] But essentially what this will do is launch Geth in console in our shell, but it's also opening up an RPC server in the background, which our web browser can connect to. Now we should be connected to our Geth node.
[04:54 - 05:08] We can try it out by typing web3, web3.eth, web3.eth.personal, and web3.eth. personal get accounts.
[05:09 - 05:19] Now notice here when we call the get accounts function, it returns a promise. So if we want to actually get the result of our accounts, we'll type web3.eth.
[05:20 - 05:30] personal.get accounts, and we'll call .then, and then we'll log out the results of that promise. If this didn't work for you, check out the network pane in your browser.
[05:31 - 06:03] But at this point, we should have web3 connecting from our browser to our Geth RPC server node. Now what we want to do is write a button on our page that will allow us to check the balances of our accounts. We'll do that by writing app.innerHTML= and then we'll put an h2 tag, show some text, and then a button, where on click we're going to call the checkbalance function. Now let's write a function that will check our account balances.
[06:04 - 06:27] Again, it's not a good practice to put functions on window, but we're just testing web3.eth.eth. Testing web3 here. So we'll type window.checkbalance= function, and first we'll call web3.eth.personal.get accounts.then, we'll log out those accounts, and then we'll get the balance of the first address by typing web3.eth.get balance.account0.
[06:28 - 06:44] That also returns a promise, so we'll call .then and log out the balance. Let's flip back over to our browser, try it out. Great, there you can see that we checked the balances of our Ethereum accounts in our browser.
[06:45 - 06:46] You