How to Call An Ethereum Smart Contract Code From Javascript
In this video, we'll write our first Solidity smart contract
Now that we have our contract on the blockchain, we can start using it.
To do this, we're going to make a JavaScript object that acts as a proxy to our smart contract. Remember that our contract code doesn't describe, it's own API, so we need to use our ABI file.
The process to get a handle of our contract instance, feels a little odd coming from JavaScript. It looks like this:
- First we'll parse our ABI into a JSON data structure
- Second we'll create a contract "class" using that ABI and
- Third we'll instantiate that class using our address
It's probably easier to just show you in code.
# First we're going to copy our abi file we compiled earlier
cat counter_sol_Counter.abi
cat counter_sol_Counter.abi | pbcopy
Next we'll go back to geth and parse the string with JSON.parse
:
var abi = JSON.parse('[{"constant":...';
// take a look
abi
// Next we're going to create, essentially, a JavaScript "class" for our contract
var Counter = eth.contract(abi);
// And finally, we need to tell our code _where_ to find this particular instance of the Counter contract on the blockchain
// So we type ...
var counter = Counter.at(contractAddr)
counter
// looking at counter, we can see we have our address, and our functions, get and increment.
Now we have an handle to our contract instance in JavaScript! This means we can now interact with our contract's code like a JavaScript object. Sort of.
This lesson preview is part of the Intro to Programming Ethereum ĐApps course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Intro to Programming Ethereum ĐApps, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
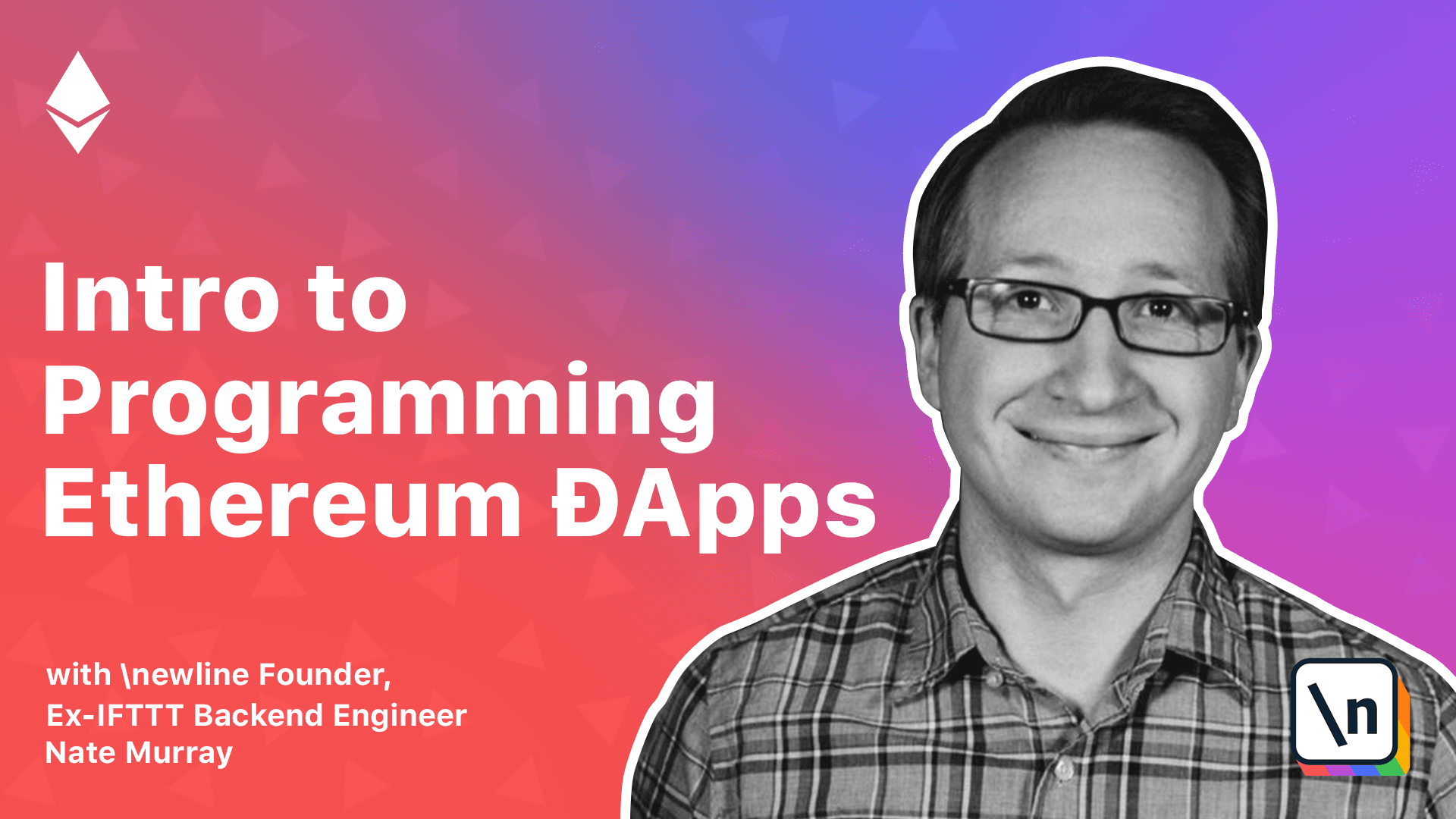
[00:00 - 00:09] Now that we have a contract on the blockchain, we can start using it. To do this, we're going to make a JavaScript object that acts as a proxy to our smart contract.
[00:10 - 00:22] Remember that our contract code doesn't describe its own API, so we need to use our ABI file. The process to get a handle of our contract instance feels a little odd coming from normal JavaScript.
[00:23 - 00:28] It looks like this. First, we'll parse our ABI into a JSON data structure.
[00:29 - 00:38] Second, we'll create a contract class using that ABI, and third, we'll instant iate that class using our address. It's probably easier just to show you in code.
[00:39 - 00:52] First, we're going to copy our ABI definition file we compiled earlier. Next, we'll go back to Geth and parse the string using JSON.parse.
[00:53 - 01:08] Take a look at the ABI. You can see that it's an object. Next, we're going to create essentially a JavaScript class for our contract.
[01:09 - 01:21] For our counter equals eth.contract passing the ABI. And finally, we need to tell our code where to find this particular instance of the counter contract on the blockchain.
[01:22 - 01:41] So to create an instance, we type var counter lowercase equals counter at the contract address. And looking at counter, we can see we have our address and our functions get an increment.
[01:42 - 01:49] Now we have a handle to our contract instance in JavaScript. And this means we can now interact with our contracts code like a JavaScript object.
[01:50 - 01:59] Sort of. Remember that all reads can be performed locally for free as long as they don't change any state. Let's try to use our read function get.
[02:00 - 02:06] Do you remember what return value should be? Great, it's one.
[02:07 - 02:18] We just read our first value from our first contract on the blockchain. Even though we just read a single number, it's worth noting that right now we have the pieces that we need to build JavaScript applications that read the contract.
[02:19 - 02:29] We'll talk about how to connect this to the browser using web three in a bit. But by making this connection between Solidity and JavaScript, we're now in somewhat familiar territory for a minute anyway.
[02:30 - 02:34] It gets more complicated when we want to update the state. Let's call our increment function now.
[02:35 - 02:40] Counter dot increment. Hmmm, error invalid address.
[02:41 - 02:50] Well, it might not be clear what that message means, but it's clear that we can 't use our state. Well, it might not be clear what that message means, but it's clear that what we were trying didn't work.
[02:51 - 03:02] And the primary reason is that you can't just call functions that change smart contract values locally. You have to submit a transaction that the rest of the network agrees is valid.
[03:03 - 03:09] So how do we do this? Here's another area where Solidity JavaScript API is not that intuitive.
[03:10 - 03:17] To send a transaction on a contract method, we call sendTransaction on that function. It looks like this.
[03:18 - 03:30] And you might need to unlock your account again. We'll call counter dot increment, but then on that increment function we'll call dot sendTransaction from ETH accounts zero.
[03:31 - 03:44] So here we're calling the sendTransaction function on the increment function. This API of calling functions on functions to change their behavior is something we'll see a few times when using JavaScript and Solidity.
[03:45 - 03:54] Our sendTransaction call returned the hash of our transaction and notice that the recipient is our contract address. And what will happen if we call counter dot get right now?
[03:55 - 04:06] You probably already know that we need to mine another block first, so let's do that. Mine are dot start, mine are dot stop, and now let's call counter dot get.
[04:07 - 04:12] Two, it works. We wrote our first change to our contract state in the blockchain.
[04:13 - 04:34] Now, if it didn't work or you want to dig in, you can use debug dot traceTrans action and pass the address of the mind transaction. You can also call eth dot getTransaction or eth dot getTransaction receipt.
[04:35 - 04:42] This might output quite a few logs, but you can just check for any errors for now. Of course, this is a simple contract with a simple application.
[04:43 - 04:48] The next thing we're going to do is bring the interface to this contract into our web browser.