What is OAuth and How Does it Work? [with examples]
Learn how OAuth works and how it will work with the Spotify API
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Build a Spotify Connected App course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Build a Spotify Connected App, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
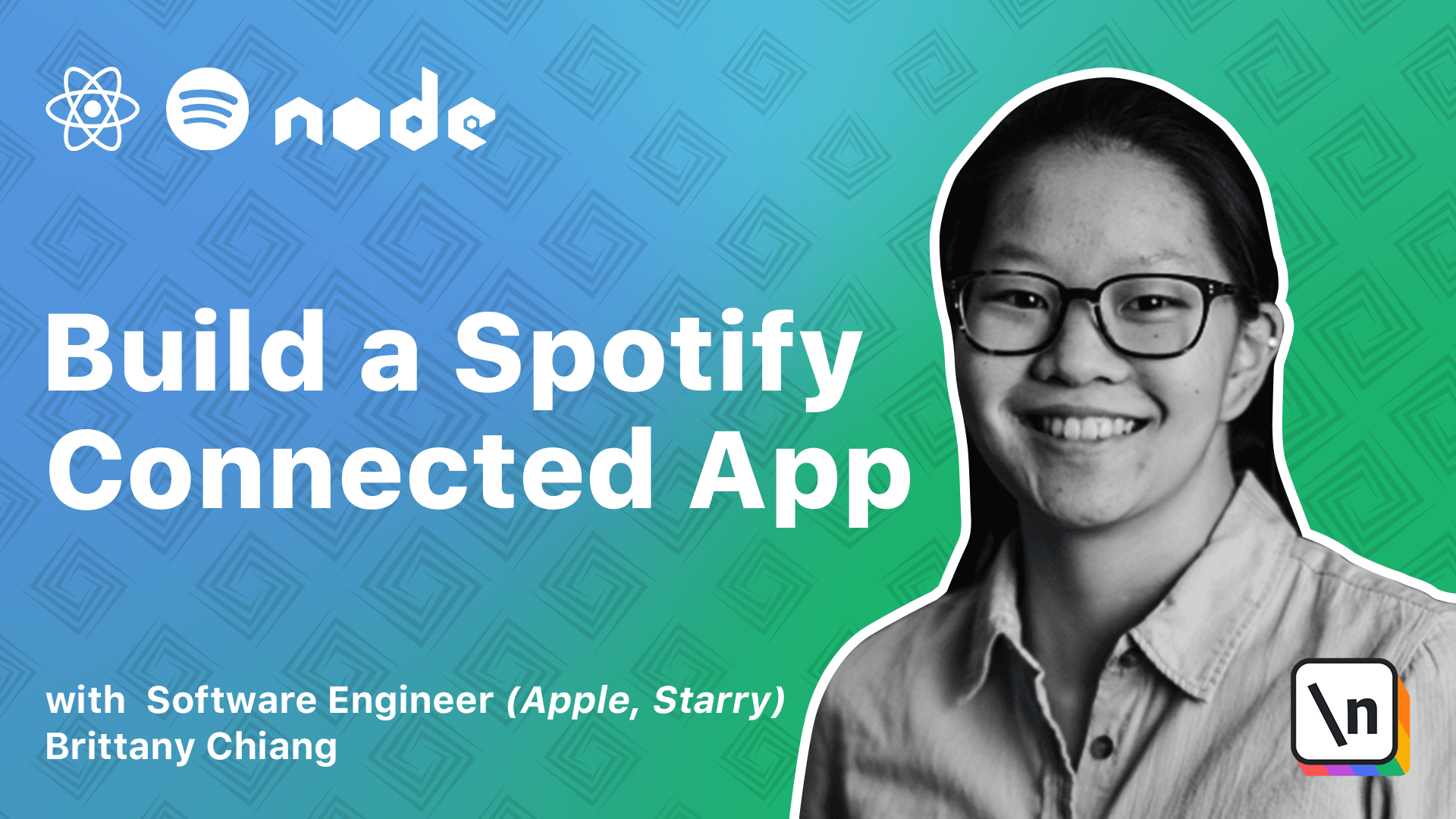
So we're planning on building an app where a user can log in to Spotify and give us access to their data through the Spotify API. We need to use something called OAuth to do that. So what is OAuth and why do we need it? OAuth or Open Authorization is a secure industry standard protocol that allows you to approve one application interacting with another on your behalf without giving away your password. So instead of passing user credentials from app to app like your username and password, OAuth lets you pass authorization between apps over HTTPS with access tokens, kind of like a special code. So for example, you can tell Facebook that it's okay for Spotify to access your profile or post updates to your timeline without having to give Spotify your Facebook password. This way, it's less risky for both you and Facebook. In the event Spotify suffers a breach, your Facebook password remains safe. Similarly, when a user authorizes our app to access their Spotify account data, we won't be storing their username or password anywhere. We don't want to be responsible for sensitive information like this, so instead , we'll rely on the secure OAuth protocol to take care of the authorization flow with access tokens. As you'll learn, the OAuth authorization flow is a bit tricky. It requires some back and forth between client server to be completely secure, but never fear. We'll go through how to handle this in our node server step by step. Let's try to grasp the OAuth flow. When trying to understand OAuth, it's important to remember that it's about authorization, not authentication. Authorization is asking for permission to do things. Authentication is about proving you are the correct person by providing credentials like a username or password. OAuth scenarios almost always represent two unrelated sites or services trying to accomplish something on behalf of users or their software. OAuth 3 have to work together with multiple approvals for the completed transaction to get authorized. Since OAuth is a way to authorize an app without giving away your username and password, you can picture it like a series of handshakes. If all handshakes are completed, then your reward is a unique access token that only your app can use to access resources from a service. There are four main roles that need to shake hands to get that token. First, the resource server. This is the API which stores data the application wants to access. In our case, the Spotify API. The resource owner. This person owns the data in the resource server. And in our case, this is the owner of a Spotify account who wants to log into our app. Third, the client. This is the application that wants to access your data. In our case, it's our client-side app. Fourth, the authorization server. This is the server that receives requests from the client for access tokens and issues them upon successful authentication and consent by the resource owner. Fourth, the authorization server. This is the server that receives requests from the client for access tokens and issues them upon successful authentication and consent by the resource owner. In this case, it's the Spotify account service. In addition to different roles, there are also different scopes in OAuth. Scopes are used to specify exactly which resources should be available to the client that is asking for authorization. They provide users of third-party apps with the confidence that only the information they choose to share will be shared and nothing more. The resource server, in our case, the Spotify API, is in charge of defining these scope values in which resources they relate to. As you can see, the Spotify API has many scopes for many different purposes. For example, the user read private scope is for read access to a user subscription details and is required if you want to access the /me endpoint of the Spotify API. This endpoint gets the currently logged in user's profile information. There is also a scope that's called Playlist Modify Private that is required if you need right access to a user's private playlist. This scope allows you to do things like add items to a playlist or upload a custom playlist cover image. You can check out all of the available scopes for the API in the docs. Like I mentioned before, all of the back and forth handshaking that OAuth requires us to do is for one thing, an access token. Our app needs this token to successfully access resources on the Spotify API. With every API request we make, we'll include our token in the HTTP request headers. If we don't, the Spotify API won't know that our app has been authorized by the user and will reject our requests for any data. You can think of access tokens like two-factor authentication codes that some services send you via text message for you to log into a website. Just like two-factor auth codes, OAuth tokens have a limited time in which they are valid. After a while, all tokens expire and you'll need to request another one or refresh it. In the end, this is all for security. In case someone gets a hold of your unique access token, they can only access your private data for a limited amount of time before they're locked out. Before any client or server requests are even made, there are two things that the client or app needs in order to kick off the OAuth flow, the client ID and the client secret. These are two strings that are used to identify and authenticate our specific app when requesting an access token. With Spotify, your app's unique client ID and client secret can be found in the developer dashboard. Once we have these two values, we're ready to initiate the auth flow. Step one, client requests authorization to access data from Spotify. So first, our app sends an authorization request containing the client ID and client secret to the authorization server, or in this case, the Spotify account service. This request also includes any scopes the client needs and a redirect URI which the authorization server should send the access token to. Step two, Spotify authorizes access to client. So second, the authorization server, the Spotify account service, authenticates our app using the client ID and client secret, then verifies that the requested scopes are permitted. Step three, user grants app access to their Spotify data. After step two, the user is redirected to a page on the Spotify authorization server where they can grant the app access to their Spotify account. In our case, the user will have been sent to a page that belongs to the Spotify account service. Note the accounts.spotify.com URL. This is where the user can log in to Spotify with their username or password, or use Facebook, Apple, or Google auth. Step four, client receives access token from Spotify. So once the user grants access by logging into Spotify, the authorization server redirects the user back to the client or our app with an access token. Sometimes a refresh token is also returned with the access token. And finally, step five, the client uses the access token to request data from Spotify. So this is when we can use the access token to access resources from the Spotify API. If all of this feels like a lot, don't worry. It's because it is. In the next lesson, we'll go over how to exactly handle the OAuth flow with our express JavaScript code. For now, all you need to remember is this. OAuth is an authorization protocol that lets you approve one application interacting with another on your behalf without giving away user credentials, like your username and password. Instead of storing usernames and passwords in our app, we'll be using an access token that we obtain via OAuth to send authorized requests to the Spotify API and retrieve data. There are five basic steps of the OAuth flow. First, the client requests authorization to access data from Spotify. Second, Spotify authorizes access to the client using the client ID and client secret. Then, the user grants the app access to their Spotify data. This is the accounts.spotify.com screen we showed before. Fourth, the client receives the access token from Spotify. And then finally, we can use the access token to request data from Spotify.