Okay, but what is a REST API?
Learn the basics of REST principles and HTTP requests & responses
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Build a Spotify Connected App course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Build a Spotify Connected App, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
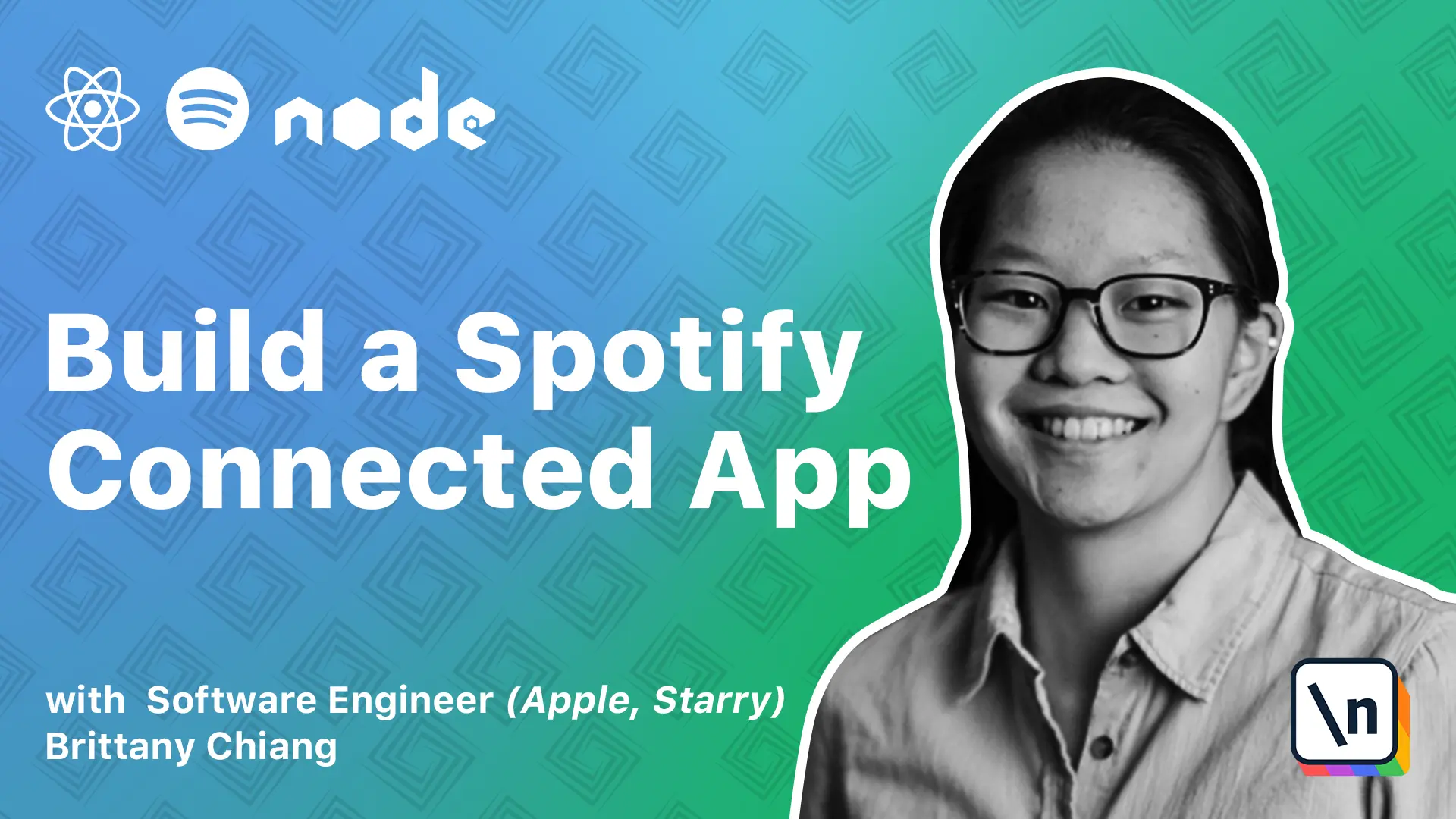
[00:00 - 00:12] So when people talk about third-party APIs like the Spotify API, the Twitter API, or the GitHub API, what they're really talking about is a REST API. REST stands for representational state transfer.
[00:13 - 00:26] So if an API is a set of rules that allows one piece of software to talk to another, then REST is a standard set of rules that developers follow to create an API. These rules determine what the API looks like.
[00:27 - 00:36] So for an API to be considered RESTful, there are certain criteria that need to be met. So the first of these criteria is client server.
[00:37 - 00:57] The API should be able to enable a client server architecture made up of clients, servers, resources, etc. With requests managed through HTTP. So when system A makes an HTTP request to a URL hosted by system B, system B server should return a response.
[00:58 - 01:10] The second criteria is stateless. All requests should be independent of each other. It should be possible to make two or more HTTP requests in any order with the same responses being received.
[01:11 - 01:27] Third, it should be cacheable. The API should make data cacheable to streamline clients or interactions. Fourth, uniform interface. There needs to be a uniform interface between components so that information is transferred in a standard form.
[01:28 - 01:49] Fifth, it needs to be layered. The request in client doesn't need to know whether it's communicating with the actual server, a proxy, or any other intermediary. And lastly, code on demand. The API allows executable code to be sent from the server to the client when requested, extending client functionality.
[01:50 - 02:10] So first, let's talk about restful requests. In simple terms, the clients of a rule of restful APIs means that you should be able to receive a piece of data, also known as a resource, when you access a predefined URL. Each of these URLs, also known as endpoints, are part of what is called a request.
[02:11 - 02:21] And the data you receive from the request is called a response. The resources provided by these responses are usually in the form of JavaScript object notation or JSON.
[02:22 - 02:34] So a restful request is composed of four parts. First, the endpoint URL, then the HTTP method, the HTTP headers, and then the body data.
[02:35 - 02:47] Let's dive a little deeper and look into these four parts of requests. So the first thing we have is the endpoint. The endpoint is the URL you request .
[02:48 - 02:58] All rest APIs have a base URL, also known as the root endpoint. For example, the base URL of Spotify's API is api.spotify.com.
[02:59 - 03:12] And other APIs like the GitHub API have root endpoint that are api.github.com. Additionally, each endpoint in a REST API has a path that determines the resource being requested.
[03:13 - 03:23] So in this case, this endpoint from the Spotify API returns data for a playlist based on the ID you provide. In this case, it's this 5.9z string at the end.
[03:24 - 03:46] And note that v1 in this Spotify API base URL is not part of the path. It's a common convention for APIs to add a version indicator like /v1 to their base URL, so that if there's breaking our major changes in the future, a new v2 base URL can be created, leaving the v1 API available for use.
[03:47 - 04:16] Some endpoints also support query parameters, and query parameters give you the option to modify your request with key value pairs. They always come after the main URL you're requesting and begin with a question mark right here. Each key value pair is specified with an equal sign and each parameter pair is separated with an ampersand. So query 1 and value 1 is one pair and query 2 and value 2 is another pair.
[04:17 - 04:56] Query parameter values can sometimes be a list of things, and in these cases, it's common to use comma separated lists. So endpoints are just like any other URL you visit on the web, but instead of returning data for a website, they return resources, data like JSON or XML. The second part of the RESTful Request is the HTTP method. This method is the type of request you send to the server, and there are five common types of HTTP methods. There 's GET, POST, PUT, PATCH, and DELETE, and they're used to perform four possible operations.
[04:57 - 05:08] Create, read, update, and delete. CRUD, as you may have heard before. So first we have GET. GET retrieves or reads resources.
[05:09 - 06:31] POST creates resources, PUT and PATCH both update resources, and DELETE, as you can imagine, deletes resources. When working with a REST API, the documentation should tell you which HTTP method to use with each request. So for example, this GET playlist endpoint on the Spotify API will tell you that this endpoint is accessed with a GET request. The resource that this endpoint returns is some JSON about a specific playlist, and we'll touch more on this below, but there is a ton of data in this JSON object that we can use on the front end. The third part of RESTful requests is the HTTP headers. HTTP request headers are used to provide information to both the client and server. You can store things like authentication tokens, cookies, or other metadata in HTTP headers. In our app, we'll be sending along basic authorization headers in our HTTP requests so that the Spotify API can authorize our requests. Headers are key value pairs that are separated by a colon, and the example below shows a header that tells the server to expect JSON content. The key here is content type and the value is application/json.
[06:32 - 07:42] If you want to learn more about HTTP headers, you can visit the MDN documentation. There are a ton of headers such as authentication headers, caching headers, client hints, etc. The fourth and final part of RESTful requests is the body data. So the body of a request contains any information you want to send to the server. And since GET requests don't need any extra information from you to return existing resources, only post, put, patch, or delete requests can have body data. And the body data you send along with these requests is usually the data you'd like to create a new resource with, for example, with a post request, or the modified value of a resource you'd like to update, for example, with put or patch requests. And data is normally transmitted in the HTTP request body by sending a single JSON encoded data string. And if this doesn't make sense to you right now, that's totally fine. We'll go over some of the more hands-on examples soon. So now that we've covered RESTful requests, let's talk a little bit about REST ful responses.
[07:43 - 07:55] So like we mentioned before, REST API responses usually return resources in the form of JSON. Like we saw before, this is the GET a playlist API endpoint on the Spotify API.
[07:56 - 08:39] And the JSON that this endpoint returns has a bunch of data that is really interesting that we can use on the front end. For example, there is the playlist image, the name, the different tracks on the playlist, and each track has its own information, such as when it was added, the available markets, and each tracks specific image. And this is all information we're going to leverage and use to display our front end when we start building out our app. The last thing we need to know about RESTful responses are HTTP status codes.
[08:40 - 08:57] So every REST API response includes an HTTP status code in the response header. These status codes are numbers that indicate whether a request was successfully completed and range from the hundreds to the 500s. In general, the status codes fall into these categories.
[08:58 - 09:37] So 100 plus is an informational response. 200 plus is a request has succeeded. 300 plus is request has redirected to another URL. 400 plus is an error that originates from the client, and 500 plus is an error that originates from the server. All right, so now that we've learned a little bit about RESTful requests and responses, in the next module, we're going to start looking into Express, a framework in which we can actually start writing our own RESTful APIs.