How to Build a Spotify Top Artists & Top Tracks Page in React
Build out and style the top artists & top tracks pages
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Build a Spotify Connected App course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Build a Spotify Connected App, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
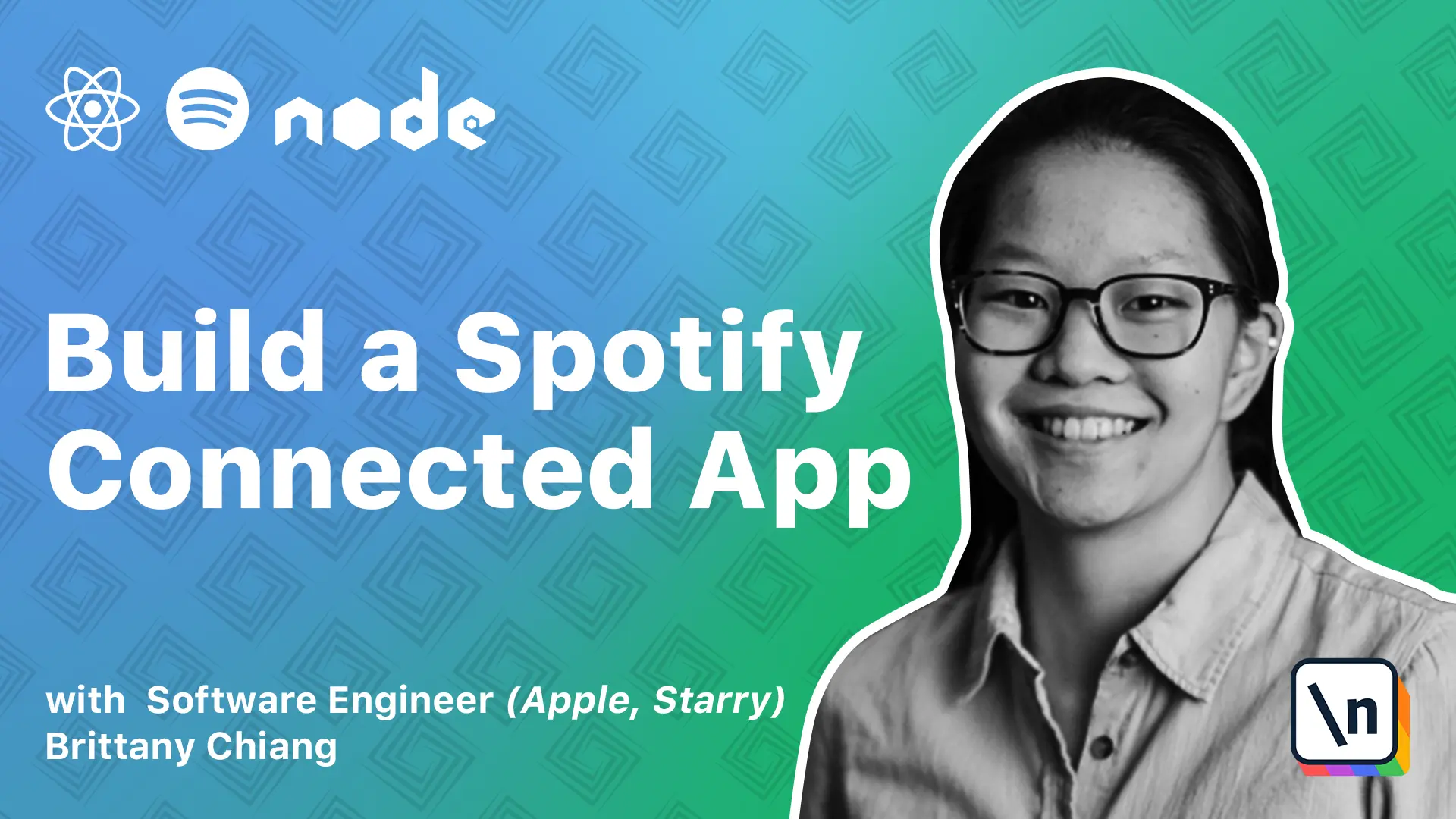
[00:00 - 00:16] Alright, so the next part of the app we're going to work on is the top artist page. This page is very similar to the top artist section we have in our profile page , but there are some buttons in the upper right hand corner to toggle between the time ranges.
[00:17 - 01:36] So let's scaffold out a top artist page component just like we have for our login page. So in App.js I'm going to add this top artist page to the imports and then down here I'll replace this H1 with top artists and then in our pages directory I'll add a top artist's file and I'll stub it out and I'll say const top artists equals function and I 'll just return an H1 for now saying top artist component and then I'll export it and then I will export it from the pages and next file as well. Alright, so if we go to our top artist page we'll see that our top artist component is being rendered. So now I'm going to just grab the fetch logic we have in our profile page and add it to the top artist page.
[01:37 - 07:07] So we'll have a use date hook, turn that into our return statement, I'll pull in use date from react and I'll pull in the use effect and here I can just get rid of all of this and then I'll add the rest of the imports I need. So looks like this. Alright and here I'll just console log top artist to make sure that data is coming through like we expect. Cool and now I can grab the artist grid like we have down here. I'll add this section wrapper and then import those components as well. Alright, so now if we take a look at our top artist page it should be really similar to what we have on the profile page. We have our top artist this month but here like we saw before we want the different time range buttons in the upper right hand corner and also we don't want to say top artist this month we just want to say top artists and we also don't need a see all link in our section wrapper if we take a look at what that looks like. Section wrapper takes a few different props it takes children title see all link and a breadcrumb and a breadcrumb is something we've set up here to just link back to our homepage so instead of a see all link here I'll just say breadcrumb true. Give that a save and we should see this breadcr umb is being rendered here we click profile we just go back to profile and I'll also add this main tag here so we can add some padding. So now what we want to do is we want to add these time range toggles in the upper right hand corner so we can go up here and above the section wrapper we can just add a list of three buttons so I'll say and in here I'll use an Emmit shorthand wrap this and I'll say I want three of them hit tab and here I'll say we want this month last six months and all time. Cool so those buttons are showing up and now we want to make them do something so we'll add an on click handler to them and what this on click handler is going to do is going to set a state variable called active range and that state variable is what we're going to use to pass the argument to get top artists. So if you take a look at this get top artists function again it hits the /me/top/artists endpoint and it passes a time range query parameter and this time range query parameter is what we're going to use to toggle between the different time ranges of top artists. So this function takes a time range argument that defaults to short term but there are three values we could pass to this time range query parameter and they are short term, medium term and long term. So in our top artist page here we'll add another use state hook and this will be for the current active range so our state variable is going to be called active range and set active range and here we're going to want to use our active range variable which is either going to be short, medium or long and pass that argument to the get top artist function. So we'll use a template string here and we'll say active range underscore term. So if active range is medium it'll be medium underscore term if it's long it'll be long underscore term and here we can just add active range as dependency and then if you take a look at the console there should be an error and it's saying this request failed with the status code of 400 and that's because we're setting the initial state of active range to null and we want it to be short in this case. So now that we've got that working all we need to do is add some functionality to these buttons.
[07:08 - 09:28] So for each of these buttons I'm going to add an on click handler and this on click handler is going to use our set active range function so I'll say anonymous function set active range and I'll set it to short for this month medium for the last six months and long for all time. Give that a save and refresh and now let's see if our buttons work. So this month last six months and all time it looks like it's changing. Cool. Now that the buttons are working the next thing we want to do is add an active state so we know what the current active time range is. So we can add a class for this that we will eventually target in a style component. Let's clean this up a bit. And here one of the props I'm going to add is a class name and we'll say if the active range is equal to a value then we 'll add an active class else we just render an empty string for the class name. So here it 'll be short, medium and long. Cool. So if we pop open our dev tools and we take a look at the class we'll see last six months is now active. All time is now active and last six months is not active anymore. Cool. So we got it working. Now all we need to do is add some styles for it. So since we're going to want these time range toggles in our top tracks page as well, we're going to move this to a separate component and we'll call that component time range buttons. So in our components directory we'll add time range buttons .js. We'll stub it out. Time range buttons equals function right now just render time range buttons.
[09:29 - 10:28] And we'll make sure to export it from our components index file. And here I'll just take this UL and I'll add it here. And don't worry we're going to pass the correct props soon. And then here I'll just say time range buttons. And I will import the time range buttons component from here. And now we can pass the variables we need in here which is the active range and the set active range function. So here we can just make sure we have them.
[10:29 - 11:15] And then in our top artist page here we'll just say active range equals active range and set active range. Cool, let's check if they're still working. Six months, all time. Awesome. So the next thing we want to do is add some styles to these buttons and make sure that the active button has a different background color than the rest. So we're going to be adding a style component for this and we're going to be calling it styled range buttons. So in our styles directory we'll add a styled range buttons.js file.
[11:16 - 14:48] And then here it'll look something like this. It's basically just a UL and we're going to absolutely position the buttons to the top right corner on desktop and then add a green background color to our active buttons. So if you export it from our styles index page and head back to our time range buttons we'll import that style component from styles. And then here we'll just replace our UL with styled range buttons. Give that a save and it looks like we're almost there. Oh, it looks like we need to add our time range buttons to be inside of our section wrapper. Let's add it right above artist grid. Cool. Now we're going to move on to building out the top tracks page which will be really similar to our top artist page. So just like top artists we will go to our app.js and we will update our top tracks route to use a top tracks component. We'll import it at the top here. And then under pages we'll add that top tracks page. We'll stub it out. We'll export it from our pages index file. Then just like top tracks we can basically copy what we have here, our imports. Instead of get top artists it'll be get top tracks. And then we'll add our logic down here. So instead of top artists I'll say top tracks. And let's give it a save and take a look at what our top tracks page looks like. And let's make sure instead of an artist grid we render a track list. And track list we'll say tracks equals top tracks to items. We don't need to slice it here. We want to make sure we get rid of this slice. And it looks like we're all set. It looks like our time range buttons are working. Our track list is rendering. And we can just get rid of this console log here to wrap it up. We did all of the hard work building out top artists. So all we need to do here is just render a track list and take advantage of the time range buttons component and style component we made before. Awesome.
[14:49 - 14:57] So now that we have our top tracks page working and our top artist page working , the next page we're going to build out in the next lesson is our playlist page.