Using Svelte's Dimension Bindings for Responsive Scatterplots
Using Svelte's dimension bindings to make our chart visible on all screen sizes
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Better Data Visualizations with Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Better Data Visualizations with Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
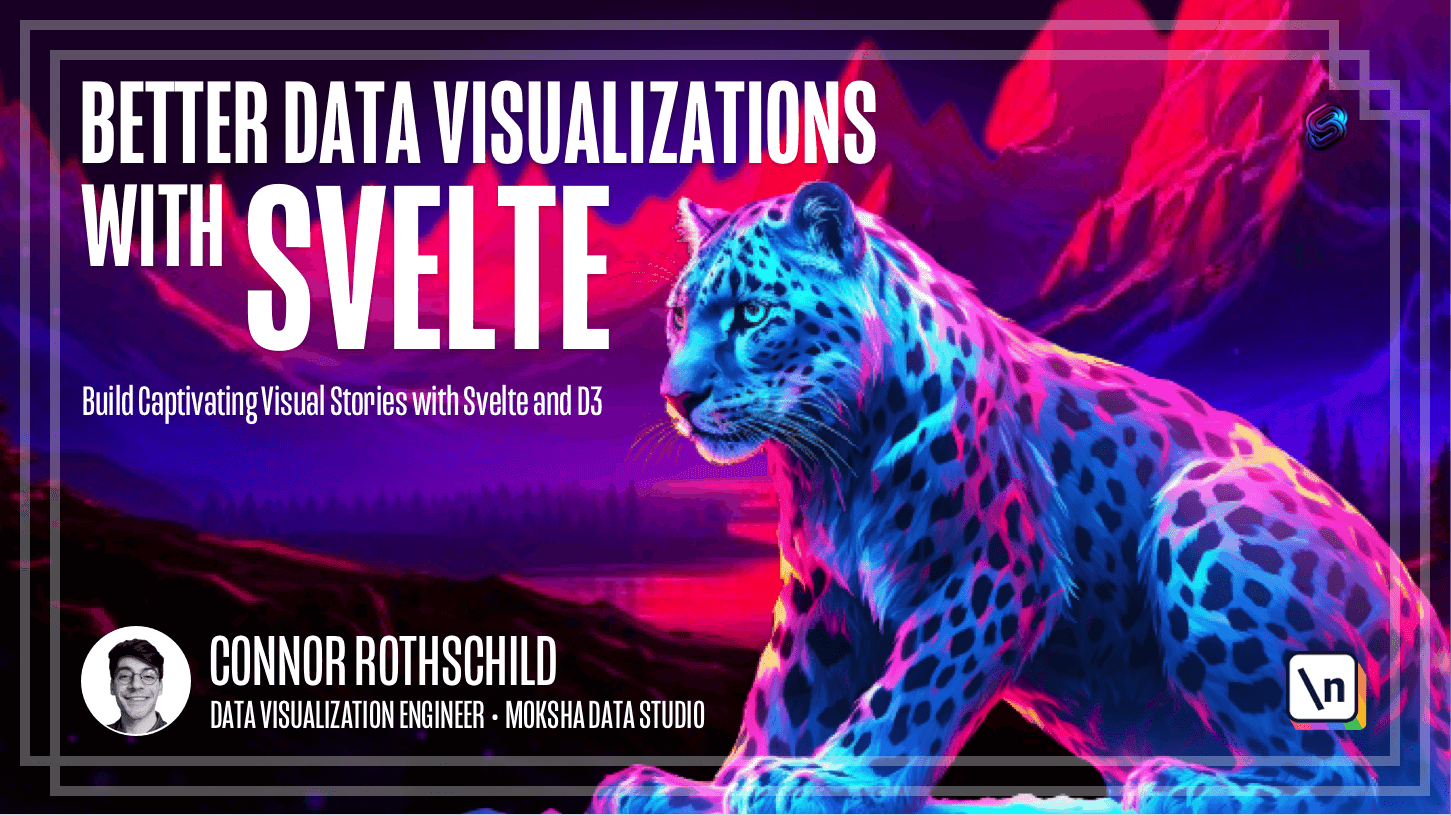
[00:00 - 00:05] Hey everyone, in this lesson we're going to be making our chart responsive. Now what does that mean?
[00:06 - 00:10] Right now we have a 400 by 400 SVG element. You can see it right here.
[00:11 - 00:19] And it looks great, but if I were on a large screen, it would look quite small. If I want a very small screen, it might not look that good.
[00:20 - 00:26] Right? And so what we want to do is make our chart responsive to whatever screen size we're currently occupying.
[00:27 - 00:44] And thankfully in Sfelt, there's some built in tools that make responsiveness really easy right out of the box. So compared to last lesson, which was almost an hour long, this will be much, much shorter as there's only a few steps that are really necessary to get this chart responsibly up and running.
[00:45 - 01:00] So the first thing that we're going to need to do is wrap our entire chart, which is currently this SVG element in a container div in a div parent element that contains the SVG and nothing else. And it will become clear why we need to do this in a second.
[01:01 - 01:11] But for now, just follow my lead. We'll type div and give it a class of chart container or whatever you want to call it, but I find chart container to be pretty handy.
[01:12 - 01:24] Let's go ahead and close that div after the SVG finishes and click save. Now you'll notice that there is a div around our chart container and within it is this 400 by 400 SVG.
[01:25 - 01:31] Now what we need to do with this container div is add a dimension binding. Now what is a dimension binding?
[01:32 - 01:54] I'm going to bring over the text for this lesson and click on this link right here, which has spelt tutorial showing what dimension bindings are. And what you'll notice is this edit me text at the bottom and as I decrease or increase this edit me font size, you'll notice the size numbers directly above it also update in real time.
[01:55 - 02:09] And those size numbers are dynamically linked to the size of this text element. So in some ways, what that means is that at any point in time, we can basically see how many pixels wide is the text edit me?
[02:10 - 02:17] How many pixels tall is it? And these numbers will update in real time thanks to what are called dimension bindings.
[02:18 - 02:39] Now practically, these dimension bindings could be one of a few values, client width, height, offset width and offset height. I believe the key difference here is whether or not these widths or heights also include the margin and padding around and within element or if it's only the intrinsic element height or width.
[02:40 - 03:09] But as you can see here, the syntax for binding an element's width or height to a given variable is like so, where we write bind colon and then client width or whatever we want to bind and the name of the variable that we want to bind it to. So in this example, on line two, W is declared to be undefined by default and on line 13, we then update it dynamically to be equivalent to the client width of whatever 's inside this.
[03:10 - 03:25] And so these two lines of code alone are what are creating dimension bindings. So now that we know what dimension bindings are, let's bring them over to our chart and using that exact same syntax, we're going to write bind client width equals.
[03:26 - 03:32] Now what do we want to set it equal to? We want to set it equal to the existing width variable that we already have.
[03:33 - 03:45] So if I type this and click save, and then to see if this is working, let's go ahead and console dot log width. But we also want to console dot log with anytime it changes.
[03:46 - 03:59] So we're going to prefix it with this reactive dollar label, which I'm going to explain what this is in just a second. But for now, we see with this 564 and if I increase with three logs over and over again, and it is in fact updating.
[04:00 - 04:04] So we're definitely doing something right. But the chart itself isn't updating at all, right?
[04:05 - 04:09] It's only the width variable that's updating. And the question is, why is that?
[04:10 - 04:22] How do we get the rest of the chart to update? Well, let's look at what's directly below width, which is our declaration of inner width, another variable that depends on width, but also depends on margin left and margin right.
[04:23 - 04:34] See, in this code, we are updating width whenever chart container resizes, because it is dimension bound, right? But line 16 is not updating alongside it.
[04:35 - 04:47] So although width is in fact changing, inner width, which is what we use throughout this chart is staying static. It's staying 400 minus 20 minus zero, right?
[04:48 - 04:57] And we wanted to also update in real time. Now in order to achieve that, we're going to need to introduce what is called the dollar label.
[04:58 - 05:11] So as you can see in this documentation right here, Svelte's key, one of its key things that it advertises why it's such a great framework is its reactivity. And one key tool for achieving reactivity is called the dollar label.
[05:12 - 05:22] Here on line three, you can see the first instance of this in a Svelte application. Now in this dummy example, count equals zero and doubled equals count times two .
[05:23 - 05:33] Now in traditional JavaScript, if you just had let doubled equals count times two, it would never update. See how here it still renders as zero, no matter how many times I click it.
[05:34 - 05:50] But Svelte says, if you want to keep doubled in sync with count, right? If you want the left side of this equals sign to update anytime the right side changes, you just replace the left declaration with the dollar colon label.
[05:51 - 06:00] And so it's called the dollar label. It can be thought of as the reactivity label, anything that you want to call it , but it is how you construct a reactive declaration.
[06:01 - 06:10] And so in this example, if I click, you'll notice that the number does in fact update any time that count updates in the example. So that's how reactivity works.
[06:11 - 06:23] And it's actually a very simple concept. Any time you want the left side to be responsive or reactive to the right side of the equal sign, just prefix it with a dollar colon instead of a let.
[06:24 - 06:32] So that's what we're going to do back in our example. And rather than inner width being instantiated with let, we're going to instant iate it with a dollar colon.
[06:33 - 06:47] It's safe and you'll notice we do start to see some changes, but maybe not the changes we wanted to see. The reason for this is because although inner width is instantiated with the dollar label, meaning its reactive, X scale is not.
[06:48 - 06:57] So here you'll see X scale is still instantiated with let. And actually at the point in time in which X scale is created, we don't even know what inner width is.
[06:58 - 07:05] It might be null. And that's why X scale is creating all of these on the zero position of the X axis.
[07:06 - 07:17] So the solution that you might have come up with in your head and the correct solution is just to replace let with a dollar label once again and hit save. Now all the circles look like they're in the right position again.
[07:18 - 07:28] And what happens as I resize my screen, everything is working as we intended it to. And so already we have a responsive chart right out of the box that simply works.
[07:29 - 07:37] And so there's something really refreshing about responsiveness that works with no additional overhead. You're just changing literally three lines of code.
[07:38 - 07:48] And now everything is working as it should. So we have a responsive chart and I'm really excited to see you in the next lesson to talk through interactivity and tooltips in our scatter plot.
[07:49 - 07:49] See you then.