How to Add Interactive Tooltips to a Svelte Globe Visualization
Providing additional context to the user on click
This lesson preview is part of the Better Data Visualizations with Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Better Data Visualizations with Svelte, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
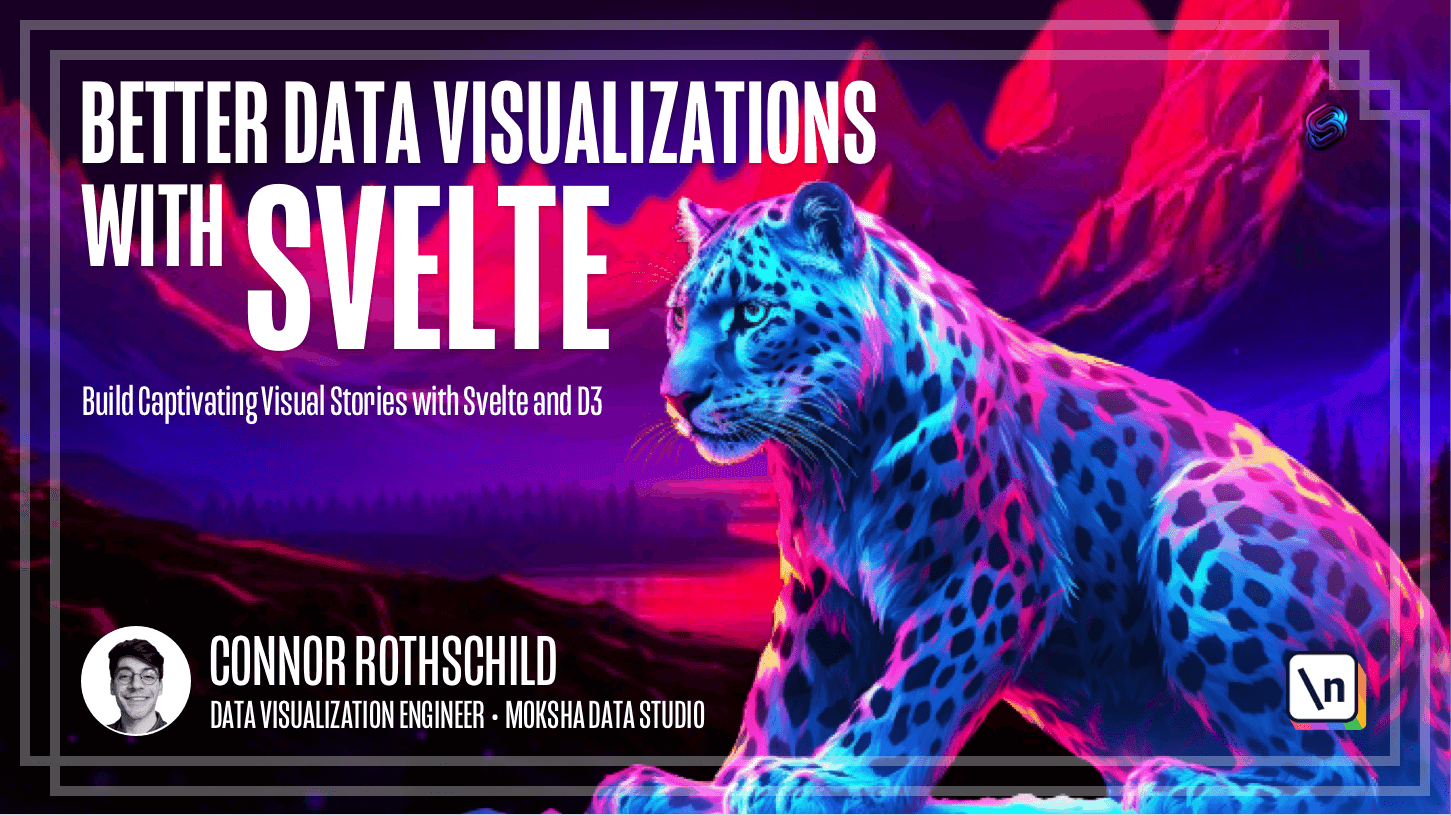
[00:00 - 00:17] Hey y'all, in this quick lesson we're going to be adding a tooltip to our globe . Right now we see all of the countries rotating one by one with auto rotation and we can rotate it with our own cursor like so, but it's still unclear, you know, the exact values for each of these individual countries.
[00:18 - 00:35] The shading gives us an idea of it, but it doesn't quite give all of the information we need. And for those of us like myself who are somewhat geographically illiterate, it might be important to see the name of the country if and when a user is interested in seeing which country has what population.
[00:36 - 00:47] So this lesson isn't going to be that different from prior lessons where we've been adding a tooltip. We're going to follow the same pattern like I said in the first module, spelt and dataviz is all about patterns, right?
[00:48 - 01:07] So we're going to be putting together a pattern that basically allows for us to add a tooltip by clicking on a country, storing that country as a variable, and then rendering dynamically a tooltip, a piece of information, if and when a country is in fact selected. So that's what we have to look forward to today.
[01:08 - 01:17] On the first step, like I just alluded to, is going to be click events and storing data. So recall that all of our countries live within this each block right here.
[01:18 - 01:36] This each block iterates through each of our 100-something countries. And for each of those lists one path, it renders one path with a de-attribute that uses our projection and our path generating functions, a fill equal to the country's population, a representative of that population, and no stroke.
[01:37 - 01:46] And just to show you if I wanted to change a stroke, for example, you would see this looks very different. Just to illustrate, this is in fact where our countries are being rendered.
[01:47 - 02:02] Now if we wanted to add some event, you know, we could add a mouseover event listener or a click event listener. It would be as simple as it has been in prior lessons where we basically do on click and then within the click we write a function that captures the behavior that we want.
[02:03 - 02:12] For our case, let's console.log country. Now I'm going to save this and I'm going to click on a country and you'll see that something does in fact get canceled.
[02:13 - 02:15] What is it? It is the country that I just clicked on.
[02:16 - 02:22] So this, for example, is Brazil. This, I believe, is the United States.
[02:23 - 02:31] And so we are seeing the country that we are clicking on is becoming rendered. It is rendered in the console, which means we're definitely doing something right.
[02:32 - 02:45] Notice that you can see, you know, all of its information, including its type, properties, population, ID, geometry, and country. And the reason for that is the transformation that we did right here in my code on lines 28 to 34.
[02:46 - 02:55] That's how we kind of added metadata from the geographic shape file data and the population data and merge them together, right? Just to remind you.
[02:56 - 03:07] So anyway, within our click event right now, we're console.logging country. You're going to notice a start off that it wants you to add a certain event for like key down, key up, key press.
[03:08 - 03:14] We're going to be addressing this with an on focus event. So we'll just do the same thing on focus.
[03:15 - 03:21] And then I believe from here we can just go ahead and ignore the compiler issue . I'm going to add that line to ignore it.
[03:22 - 03:30] But we will, in fact, make this tab indexable and focusable via the keyboard. So we'll address that accessibility concern that it will be a separate step.
[03:31 - 03:37] So for now, I'll delete this and we'll keep this as is with no compiler errors. So what do we want to do on click?
[03:38 - 03:41] We don't just want to console.log country. That is not very helpful.
[03:42 - 03:49] We want to keep track of that country in a variable. So up here in our script tag, let's add a new variable and call it tooltip data .
[03:50 - 03:59] By default, tooltip data will be undefined. But on click, we will reset data, or tooltip data, to be equal to the clicked country.
[04:00 - 04:07] So now, again, you've probably gotten used to this pattern by now. But if I console.log tooltip data up here, I'll destructure it so you can see the name.
[04:08 - 04:13] Notice how by default it's undefined. But every time I click, it updates to a new country.
[04:14 - 04:19] So this is, in fact, working tooltip data does exist. What comes next?
[04:20 - 04:35] The second step in rendering our tooltip is dynamically rendering a component that we'll call tooltip.sfelt if and when our tooltip data variable exists. So let's create a brand new component.
[04:36 - 04:42] And remember, we're going to open up this sidebar over here. And remember that the only component we have right now is glow.sfelt.
[04:43 - 04:47] Again, I'm going to move me. So right now we have glow.sfelt.
[04:48 - 04:52] Let's create a new component and call it tooltip.sfelt. Mine is not focusing.
[04:53 - 04:55] Here you go. tooltip.sfelt.
[04:56 - 05:03] Now, what do we want tooltip.sfelt to accept? Well, what we know by default is we definitely want it to accept some data.
[05:04 - 05:16] So right now, I'm just going to do export let data. And then I'm going to import the tooltip component right here from, and then I 'll find it in path components slash tooltip.sfelt.
[05:17 - 05:23] And I'm going to place this under my SVG element. The reason for this is because this is going to be a regular plain old HTML element.
[05:24 - 05:27] We're not going to write too much SVG. We're going to keep it in the document flow.
[05:28 - 05:35] And we're going to use a div for that. So we're going to render tooltip and we're going to pass in to accept the property data.
[05:36 - 05:42] We're going to pass tooltip data. So remember that the syntax here is this is the name that we accept tooltip.
[05:43 - 05:52] We accept data, but its value according to app.sfelt is tooltip data. So anyway, we're going to save this and save this.
[05:53 - 06:00] Refresh, nothing should change. You can confirm that this is in fact working by console.logging hello from tooltip.
[06:01 - 06:06] And then we can render the data to make sure that's working. Now if I click, you're going to need to prefix this with a dollar label.
[06:07 - 06:11] Now if I click, you'll see that it updates every time I click on a new country. Right?
[06:12 - 06:13] Great. So our tooltip exists.
[06:14 - 06:21] It works. And we are able to access the data that we click on from the app.sfelt parent.
[06:22 - 06:24] So what do we want to do? We don't want to console.
[06:25 - 06:29] We want to render something. And you might remember that the easiest way to do this in sfelt is going to be an if block.
[06:30 - 06:36] So we would simply write if and then what condition is met. We can do if tooltip data exists.
[06:37 - 06:54] But just in case the user were to click on a country that exists within the geographic shape file but does not exist within our population data set, one thing that we could instead do is, or sorry, we should have done if data. But what we should instead do is if data and then the property that we want to look for in particular.
[06:55 - 07:07] So I'm going to look for country because if the data existed in the geographic shape file from our very early lesson one, but it did not exist in our population data set , we don't want to render on the tooltip. So this is the easiest way to do that.
[07:08 - 07:13] We're going to look for the country property. And if it exists, we will render what is inside the block.
[07:14 - 07:20] For now, let's just do a simple div. And in it, let's do an h2 where we render the country name.
[07:21 - 07:31] And an h3 where we render population of and then data dot population. So this is going to be a very rough, rough kind of overview of what a tooltip could look like.
[07:32 - 07:44] So I'm going to click and then if you have very, very keen eyes, you might be able to notice right down here is where our data is rendered. So if I click on a country, China has a population of 1.4.
[07:45 - 07:53] I'm going to guess that's billion people. As you can see, these numbers incoming.
[07:54 - 08:06] Here, the United States has a population of 3.318 million, 331 million people. Wow, I almost exposed my knowledge of the world's population, but I do know the US has around 330 million people.
[08:07 - 08:12] So with that out of the way, you do have a tooltip. And what we definitely need to do now is some styling.
[08:13 - 08:17] We're going to do the three things. First, we'll place the tooltip in the bottom right corner of our globe.
[08:18 - 08:27] Second, we'll make the tooltip text white so that it's actually visible. And third, we'll make the country a larger font size and add some slight styling so it actually looks pretty nice.
[08:28 - 08:36] So the first thing we're going to do is place the tooltip in the bottom right corner of our globe. In order to achieve that, I'm going to open a new style tag and I'm going to target our div.
[08:37 - 08:48] Remember that because this is styled, scoped specifically to tooltip.s felt, I can target only div and only this div will be affected. Nothing on app.s felt will be affected because this is scoped styling, right?
[08:49 - 09:02] So I'm going to give it a position of absolute, which you'll recall from our earlier lessons. A position of absolute basically takes the div out of the document object flow and places it according to its pixel values, either top, bottom, right, or left.
[09:03 - 09:11] So I'm going to give it a bottom of 30 pixels. And the reason for that is I want it to kind of be in the corner but not all up against the edge.
[09:12 - 09:15] I'm going to give it a right of zero. I'm going to give it a text a line of right.
[09:16 - 09:20] Let's hit save and see how it changes. Now you see the tooltip appears here.
[09:21 - 09:29] It looks quite nice, but it isn't visible still, right? The position in the user, right, maybe, but the tooltip itself is kind of ugly.
[09:30 - 09:36] We're going to target our H2 and our H3 individually. We'll make our H2, which remembers our country name.
[09:37 - 09:44] I'll give it a font size of 1.5 rem and a font weight of 700. Then we're going to give it a color of white so you can actually see it.
[09:45 - 09:47] Now you can read the United States. It's actually visible.
[09:48 - 09:59] Let's give a similar treatment to our H3, where we're going to make it a font size of 1.15 rem, slightly smaller than the country, and a font weight of 200. So less bold, right, lighter than our other font.
[10:00 - 10:06] Here let's give it a fainter white. So let's do an RGBA of 255, 255, 255, 0.7.
[10:07 - 10:18] So what am I doing here? One would be pure white because it's 255, 255, 255, 255, and that RGB value corresponds to 1, whereas 0, 0, 0 corresponds to black.
[10:19 - 10:22] And so the 1 is the alpha value. So if I did a 0.1, it would be 10%.
[10:23 - 10:26] It would be super transparent. But at 0.9, it would be mostly visible.
[10:27 - 10:31] But I'm going to do 0.7. And so now you'll see that it's kind of fading.
[10:32 - 10:33] It's a little bit transparent. So just illustrate that.
[10:34 - 10:39] If I made it 0.2, it would be almost invisible. And then the only other thing we need here is some space between the elements.
[10:40 - 10:47] So on my H2, I'll say margin, bottom of 0.25 rem and hit save. I think that's looking pretty nice.
[10:48 - 10:52] I think our tool tip looks pretty good. So what else do we want to do here?
[10:53 - 11:00] I think the first thing that we want to do is make the tool tip disappear when the user clicks outside of it, right? The only thing I can do right now is update the tool tip.
[11:01 - 11:18] I can't delete the tool tip. And so the way that we're going to delete the tool tip is by basically putting a click event on the circle, which is the globe, that says if and when you click on the circle itself, none of the countries on top of it, but just the circle, go ahead and delete tool tip data or make it null.
[11:19 - 11:28] So we'll say on click, and then we'll do tool tip data is equal to null. And we'll apply that same disable right here.
[11:29 - 11:34] Perfect. So now if I click Brazil appears, if I click off, it disappears.
[11:35 - 11:39] See the difference? So slight, slight quality of life improvement.
[11:40 - 11:55] Let's also make it so that whenever I click on a certain country, say Brazil, the globe freezes right there and it focuses on it and it does not move. So this, we're going to bring in some old, you know, some of the rotation code and we're going to halt rotation if and when a country is focused.
[11:56 - 12:03] So what we need to do is use the centroid function from D3GO. So I would recommend, you know, to do some research on this.
[12:04 - 12:11] You can Google D3GO as you might remember. D3GO has a series of methods and functions.
[12:12 - 12:22] And what we're particularly interested in is what's called geo centroid, which returns the spherical centroid of the specified geo JSON object. What does that mean?
[12:23 - 12:33] Effectively, if we pass in a certain country that has geographic properties, it will find us the midpoint of that. And it will return the midpoints coordinates.
[12:34 - 12:37] Okay. So knowing that, this is what we want to use is geo centroid.
[12:38 - 12:47] And if you wanted to go one level further, you could always Google it and find some specific examples. For example, here you might find how somebody is using it in action.
[12:48 - 12:52] But lucky for you, I'm just going to tell you how to use it. So we'll go back to our code.
[12:53 - 13:11] And the way that geo centroid works is we want to pass in one of these country objects, which looks like this down here to geo centroid and it will return the center of that country. So easier said than done, let's just go ahead and import geo centroid from D3GO .
[13:12 - 13:26] And then we want to effectively, you know, rotate the globe if and only if a country is currently covered or is currently selected. So what we want is a reactive block.
[13:27 - 13:35] You may remember this dollar label basically says, if anything happens within the block, if anything changes within the block, carry out what is within the block. That is what we're doing.
[13:36 - 13:40] So we're going to say, we need a condition for this. I apologize.
[13:41 - 13:51] So we're actually going to say, if tooltip data exists, then carry out what's inside. Let's find the center of the geo centroid of our tooltip data.
[13:52 - 14:01] Right. So we want to rotate our projection like so projection dot rotate and then center of zero center of one.
[14:02 - 14:12] So what's happening here before we actually carry it out, let me go ahead and console dot log center so you can kind of understand what's happening at a high level. I'm going to make sure that this is not constantly.
[14:13 - 14:20] So if I click, you'll see an array of two items. So index zero represents its X rotation represent Y represents its.
[14:21 - 14:25] I apologize. I'm going to say this array item one represents its Y axis rotation.
[14:26 - 14:33] So given that we have this, we want to basically pass those values in to our rotation. And there's two ways to do so.
[14:34 - 14:44] So D three documentation would recommend you do this projection dot rotate, which then you pass in the values you want for X and Y and it will rotate there. Let's see what happens if I try this.
[14:45 - 14:50] It works, but it very abruptly transitions. And you might question, oh, well, how can we fix this?
[14:51 - 15:00] Well, remember that we already have spring variables using spelt built in physics engine. We can spring our values to the new X and Y rotations.
[15:01 - 15:10] So rather than, you know, writing projection dot rotate, we actually just want to reset X rotation and Y rotation. Now I believe that we do need these to be negative.
[15:11 - 15:12] I can't recall. Let me see what happens.
[15:13 - 15:18] But if I click, then you notice it does in fact work. Okay.
[15:19 - 15:25] It somewhat works, but what what's going wrong here? Why is it whenever I click, it doesn't complete the transition.
[15:26 - 15:34] Again, the culprit is going to be auto rotation. See how it tries to pan to China, but it can't make it all the way because the auto rotation takes over before it can get there.
[15:35 - 15:44] So again, this auto rotation is going to be something that we need to account for if we want this to functionally work. So thankfully, we already have an existing handler for this.
[15:45 - 16:01] For our timer that we created up above, all we want to do is say, if dragging is true, return, just like we already had, but also if tooltip data exists, then stop the timer. So here, notice that now if I click, it will pan to Brazil and stop.
[16:02 - 16:12] It has that spring animation, that little bit of jitter, which is intentional, but you'll notice that it goes to its final destination and it stops. Now if I click off, what's going to happen?
[16:13 - 16:24] Objective data, our variable is going to be reset to null, which means this condition will no longer be met, which means this line of code, 52, will in fact execute. Go ahead and see.
[16:25 - 16:34] Then the auto rotation continues and we're good to go. The final thing we want to do, the main, the final main thing we want to do is highlight the country that's being selected.
[16:35 - 16:46] So you select a really small country, Malawi, and you don't know which country it is, especially if you're geographically less proficient, like I am. In that case, you want to highlight using a border, the country that the user has selected.
[16:47 - 16:59] Now you might think that you would do so in the country's element. You could say, make the stroke white, if and when, tooltip data equals country.
[17:00 - 17:07] So you could try this. Let's see what happens.
[17:08 - 17:12] It works, but it doesn't work, right? So you see it, but not whenever it overlaps with another country.
[17:13 - 17:19] And the reason for this is our borders element down here, right? So remember that our borders are drawing borders between countries.
[17:20 - 17:30] So this would work, but we want our borders to render normally. And we don't want these overlapping shapes for each of the countries that share borders, which is why we're using this borders path in the first place.
[17:31 - 17:36] So we can't apply the stroke in the individual country path. So let's go ahead and bring this back to none.
[17:37 - 17:45] So where do we want it? We actually want it to live on top of the borders path because we want this white border to be drawn on top of the existing black border.
[17:46 - 17:56] So what we're going to do is say, hovered or selected country border. And from here, we want to write if tooltip data, remember that is in fact an important dependency here.
[17:57 - 18:00] Now let's go ahead and add a path. What do we want that path to have?
[18:01 - 18:08] A D attribute that's equivalent to path of tooltip data. So it's literally rendering just one of these countries just like last time.
[18:09 - 18:12] The difference is it's just on top of the borders. It's a very important note.
[18:13 - 18:24] So from here, then we want a fill of none or transparent because remember the country is selected already. We don't need to like re-render the green outline, although if you wanted to, you could make it like black or something.
[18:25 - 18:31] It's up to you. But I'm going to make my transparent and I'm going to add a stroke of white and I'm going to end the path.
[18:32 - 18:35] I'm also going to add a stroke width of two. That's slightly darker.
[18:36 - 18:40] So let's save this, save the if and then see what happens. Nice.
[18:41 - 18:49] So we see these beautiful paths being drawn around the actual country. It works perfectly and it disappears if I click off.
[18:50 - 18:58] But there's a feature I want to include from Svelte internals that makes this just look a little bit cooler. And that is Svelte's draw transition.
[18:59 - 19:08] So remember that we've already used properties or functions like fade and fly, which are Svelte's built in transitions. There's a third one that I like to use.
[19:09 - 19:13] It's called draw. And this is from the exact same place, Svelte transition.
[19:14 - 19:22] And this will give in a certain path, an SVG path with a D attribute. It will actually draw that D attribute from zero to finish.
[19:23 - 19:25] So it'll look really cool. It might be easier to just show you.
[19:26 - 19:32] So here on my path, I'll go ahead and write in draw. I'll hit save and click on a country.
[19:33 - 19:39] And now you notice this cool transition, but only the first time. So why is this?
[19:40 - 19:53] Well the reason is this if block, basically the if block, the condition is met whenever I select the first country. So if I select Brazil, this if block has been triggered, which means path has been drawn into place.
[19:54 - 20:05] So if I select a new country, because this if block condition has already been met and it will continue to be met, it's not going to re-render path. It will just move it to the new location.
[20:06 - 20:16] So what we actually need is what's called a key block. So this is another thing you should probably do some research on, but you could go ahead and look up key blocks felt and see what shows up.
[20:17 - 20:27] And you'll see that it's specifically used when referencing transitions, which is exactly what we're doing. So key blocks destroy and recreate their contents when the value of an expression changes.
[20:28 - 20:34] And the syntax looks like this, you just key and then the content inside. And that's actually exactly what we want here, right?
[20:35 - 20:49] As that prose just described, we want to render or re-render the transition if and when this inside changes. So let's go ahead and add a key block right here that says key of tooltip data.
[20:50 - 20:58] It is an object so we can just target a property that we know is always going to change, like dot ID. And then let's close the key block and hit save.
[20:59 - 21:05] Now if I click on Brazil, you'll notice it draws in. And if I click on the United States, you'll notice that it recreates the transition.
[21:06 - 21:12] So we have a perfectly transitioning border for whatever country is selected. You might ask why not use an entire transition.
[21:13 - 21:20] Well, let's go ahead and see why we click and then move. You see this lag where one country disappears and the next fades into place.
[21:21 - 21:28] That's definitely not the behavior we want. So we definitely just want to do in draw and let the other transition or let the other border disappear immediately.
[21:29 - 21:36] So notice how this border will disappear immediately as the new one draws into place. So great.
[21:37 - 21:39] So what else do we want? How does this look right now?
[21:40 - 21:43] Let's go ahead and talk about what's going well, what's not. Some final additional polish.
[21:44 - 21:48] The first thing that sticks out to me is that this population is ugly. It's a long string.
[21:49 - 21:58] And if you don't know populations, you don't want to have to read how many digits it is to know if it's millions, billions, whatever. So that's maybe one thing.
[21:59 - 22:09] Second, a transition. So just like the border fades or draws into place, the tool tip itself should transition in and out, fading nicely.
[22:10 - 22:19] And third, let's add a little cursor so that people know that they can click on a country, just using the pointer cursor property. The first thing we'll do, like I said, is formatting the tool tip population.
[22:20 - 22:22] We're going to do that in here. And there are a few ways you could do so.
[22:23 - 22:29] For example, you could write like two local string and see what happens. This is JavaScript's native way of kind of formatting numbers.
[22:30 - 22:36] So here you can see, yes, it's 1.4, 1, 2, whatever, right? You can see the actual comma separation.
[22:37 - 22:42] You can see, yes, the United States is 331 million, et cetera. But maybe this is still too many numbers.
[22:43 - 22:59] And you know, you should ask, what is your user gain from reading the exact digits in these places as opposed to just an M or as opposed to just a B? And in order to solve this, we're just going to render an M or a B. And the best way to do that is using D3's formatting library.
[23:00 - 23:07] So we're going to import format from D3 format. And the D3 format module that this library can be kind of confusing.
[23:08 - 23:20] So I would definitely recommend reading some documentation about D3 format. But there are good examples on its GitHub repo and on observable, the kind of illustrate how format works on a high level.
[23:21 - 23:33] As you can see, all of these are different ways to format certain numbers and they'll return a human readable output. So what you could do is go ahead and look for what most closely resembles the output that you want.
[23:34 - 23:46] For us, it's something like this, trimming the long digits and adding an M to replace them or a K to replace them. So we know we're going to be using this 2S or maybe 3S or maybe 1S.
[23:47 - 24:01] And so now that we know we want to use this, so the S, I would go back to the documentation and see what does that S even do. So I'm going to Google S and then quotation mark and then see how it ends and see what people or how they recommend using this.
[24:02 - 24:19] So here you see the available type values are S is decimal notation with an SI prefix rounded to significant digits. So it's basically going to return a decimal and use an SI prefix, whatever that means, rounded to significant digits.
[24:20 - 24:28] And if you're a math person, you're probably smarter than me and know what all of these mean. But for me, I know that this is the way that you abbreviate into human readable strings.
[24:29 - 24:42] So we're going to create a new format and call it suffix format because that's basically what we're doing is we're adding a letter as a suffix. And that's going to take in our value and return something that resembles that format.
[24:43 - 24:53] So we'll do dot 2S and then we will close it and then add the D. So this is going to have a bug right now by default. Oh, sorry.
[24:54 - 25:02] Let's go ahead and use suffix format and wrap our data dot population around it . So it's going to look good for most countries, Brazil, US, anything less than a billion.
[25:03 - 25:18] It's going to look good. But if I try to click on China, notice that it renders a G instead of a B. And the reason for that is because that SI prefix and whatever else, like this is how technically numbers above a billion should be rendered.
[25:19 - 25:30] But that's not what we want because we're writing to like a regular audience, not a scientific audience. So here we'll just do dot replace and replace any instance of G with B. So replace G with B representing billion.
[25:31 - 25:37] We'll hit save, click around, you'll see 1.4 billion renders. Everything is looking great.
[25:38 - 25:43] So now that we have our suffix format dealt with our strings are more human readable. Let's do something.
[25:44 - 25:52] Let's do the next thing that we were going to do, which is add a transition to the tooltip in and out. The way that we're going to do that is pretty simple.
[25:53 - 25:58] We have this outer div. Let's just go ahead and add transition fade.
[25:59 - 26:03] And then we'll need to import fade from spell transition. Now let's see what happens.
[26:04 - 26:10] You'll see that it doesn't fade between, but it does fade when it first enters and exits. This is personal preference.
[26:11 - 26:22] This could be totally fine. But if you wanted to also, you know, have it fade in every time a new one appeared, you'd want to do in fade and you'd want to add another key block just like we did last time.
[26:23 - 26:31] So you do key of data.country or maybe data.id, whatever you want, it's save. Now what would happen?
[26:32 - 26:38] Each time we click on it, it reappears. So that is something you could do as personal preference.
[26:39 - 26:49] I personally think that if it's already live and active, that's sufficient. But you could, you know, you could do in fade and out fly or whatever else.
[26:50 - 27:02] So personally, I think I'm just going to do transition of fade and I'm going to remove this key block because I think it's fine to just change the value abruptly if the country itself changes too. I'm going to click off and see.
[27:03 - 27:11] Great. So the final thing that we want to do, oh, the really small thing was add a cursor to each of our individual countries.
[27:12 - 27:19] So that's pretty easy. We can just go in here and add a new rule set for path and say a cursor of pointer should be applied.
[27:20 - 27:27] So now if I hover over Australia, you'll see pointer just begging me to click on it. Perfect.
[27:28 - 27:47] So now that we have that dealt with, the final thing we wanted to do is make sure that our map was accessible. So remember that the compiler was asking us to make sure that click events have key events and we're going to add focusable elements and make our countries tab indexable so that they can be navigated to using a keyboard.
[27:48 - 28:01] So what we're going to want to basically mimic is the click event where we add tooltip data to be equal to country. We'll do the same thing for on focus and then we'll need to add a tab index of zero.
[28:02 - 28:12] And then you can ignore this error because it is an interactive element. And we're going to do the same thing up in our circle, but instead of adding tooltip data to be country, we'll make it null.
[28:13 - 28:24] We'll ignore, hit save. So now if I tab on my globe and click in, you'll see that the first country to be present is Afghanistan because that is the first country alphabetically in our array.
[28:25 - 28:47] As I proceed, you'll see each of these countries appear one by one and the globe swings to each of them, including apparently Antarctica, which now you can see why we don 't want to render a tooltip for something like Antarctica because its population is either unknown or zero. So given all this, like we have a good functional tooltip, you could organize the countries by their population if you wanted to.
[28:48 - 28:54] So you could say each countries dot sort and then find their population and then render them. And what would this change?
[28:55 - 29:00] Well, I haven't actually checked this. So I'm going to delete this key block.
[29:01 - 29:04] What would this change now? The first thing to render would be China.
[29:05 - 29:07] The second thing would be India. The third thing would be the United States.
[29:08 - 29:12] So we're actually seeing the countries listed by their order of population. This is personal preference.
[29:13 - 29:19] You don't have to sort them this way, but it might be interesting. Final thing is this blue box around the globe countries.
[29:20 - 29:30] The reason for that is because for accessibility reasons, we want the path or whatever is being focused to be visible to keyboard users. But the thing is we're already highlighting these elements using this outline.
[29:31 - 29:37] So we actually don't need that focus. So what you can write is path of focus and give it an outline of none.
[29:38 - 29:48] You might want to remind yourself that typically removing focus is a bad idea of focus styling. It's a bad idea.
[29:49 - 30:00] But in our case, the focused country already has its own outline. And this reminder, just make sure you don't repeatedly use this whenever it is in fact needed for accessibility reasons.
[30:01 - 30:17] So now as I focus on new countries, there is no blue box, but we still see them highlighted. So voila, we've completed this lengthy and fun step where we've added a tool tip, we stop rotation, we've really focused on the selected country.
[30:18 - 30:28] In the next lesson, we're going to be adding a legend to add some final context for what that population means relative to the rest of the world. So thanks for bearing with me in this one, and I'll see you in the next lesson.