How to Clean Up and Componentize Svelte Code
Componentizing our code for a more readable project
This lesson preview is part of the Better Data Visualizations with Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Better Data Visualizations with Svelte, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
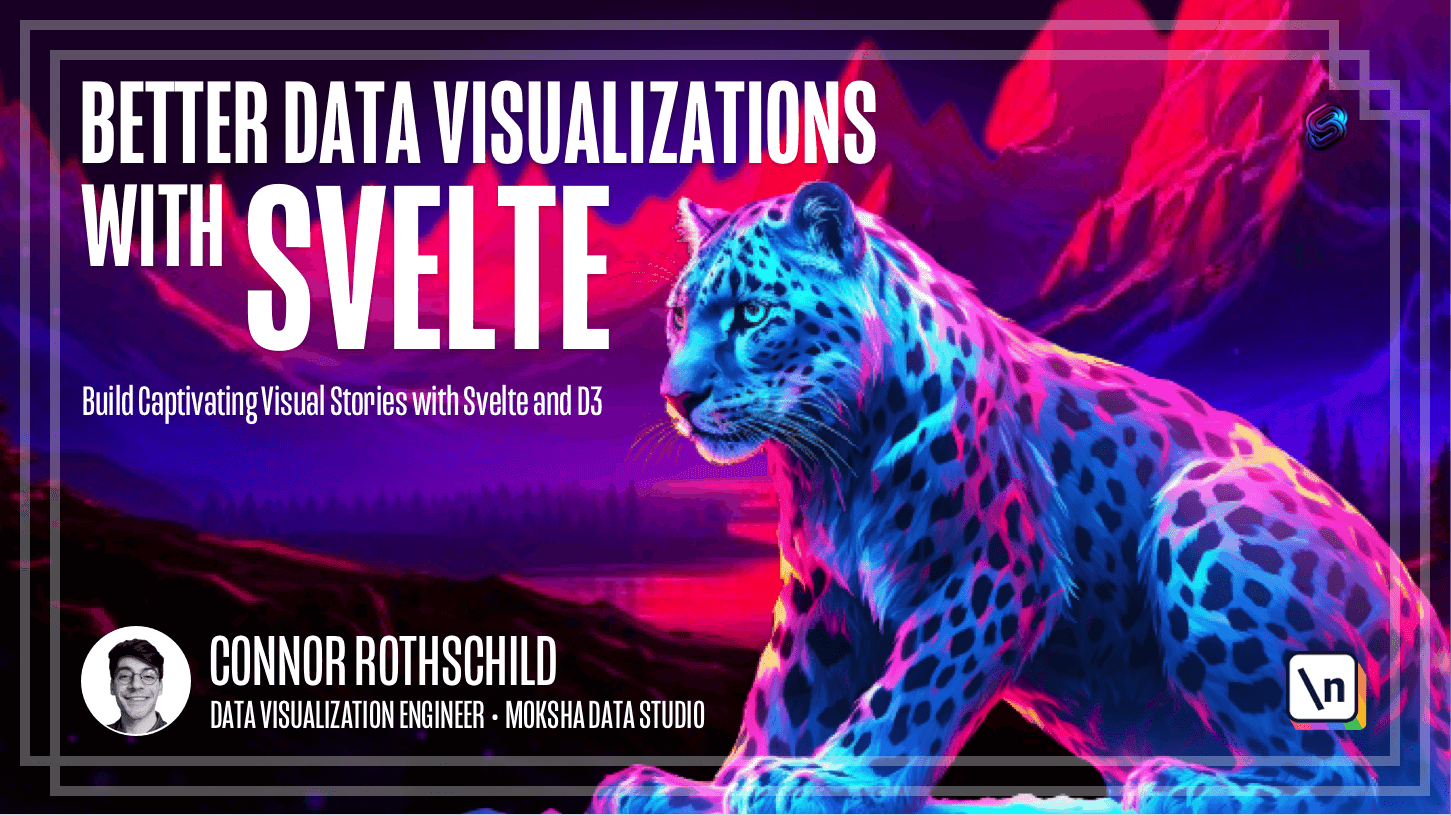
[00:00 - 19:45] Hey y'all, welcome to the final lesson of this module in the course more broadly and sorry for you know speaking slower I'm just honestly getting emotional it's all coming to a close no I'm just kidding I'm really excited that you're here if you've made it this far thank you so much for tuning in and watching the entire course it literally means the world to me so thank you and if you know anything came up throughout these four modules and you have questions you deserve it just for sitting through all of it you deserve like all the answers in the world so just DM me with any questions reach out to me however you'd like and I will answer any question because you deserve it for making this far so yeah awesome but we're not done yet what do we have to do now we're basically going to spend our final module refactoring a bit of our code base and what I mean by that is there's a lot happening in app.s felt right now there's 214 lines of code and obviously they are split up into the script tag our markup and our style tag but even within those three there are definitely characteristically distinct bits of code for example here we have all of our chart code right this div of sticky in the chart container with it here we have all of our steps and everything within there and then you know within the style tag there are certain styles which are only being applied to steps or only being applied to the chart the sticky element that surrounds it or the chart container so rather than having all of this code live in one file what if it lived in components and essentially you know whether you're making a data visualization or you're working in any other web project components are a great way to keep your code base concise and clean right if you're visiting this again two years from now and you're like man where is that article that I made with Conner about students who studied longer scoring higher on their finals exams and you pulled this out of the the archives two years later obviously by then you're a visualization expert but back then you know you were just learning alongside me and you're trying to understand how you even built this old article because it's important because maybe you're building a scrolly telling article and imagine how much you would think your past self if you came back two years later and everything was cleanly named and clean components that had characteristically separate purposes from one another and those components were not too lengthy and they got the job done and they did exactly what they were supposed to do you would thank yourself a lot now imagine you came back two years from now and you had 214 lines of code and you're like what's happening it's probably not ideal right so for your future self for other people on your team and you know just to be a good programmer let's go ahead and refactor our code base to make it cleaner and work in size and candidly maybe we should have done this on previous projects too but the truth is I kind of wanted to make sure that you got the patterns down and I didn't want to distract you with some of the quote-unquote best practices of component organization if it got in the way of you understanding and inculcating these kind of patterns that are replicable across every project that you work on so we're going to wrap up by doing something we haven't done before we're just kind of refactoring our code base into nice characteristically distinct components the first thing we need to do in order to refactor is identify what's actually happening in our large app.s felt file and the best place to do this is the markup because although the script tag contains different bits of logic and the style tag different styles the markup is that basically the referential center of all of this right the markup has the classes you know the CSS elements below and it has the logic like binding the value of current step and you know some of like iterating through and these props like the the markup itself is where we center our focus and it's where we're going to begin the refactor so what's happening in our markup we have one large main tag which is basically the page wrapper within that we have a title some sample text and then section is really where the main meat of our project lives we're doing three things okay first we are rendering a chart which you can see we have chart container here and we're rendering all these elements within an SVG tag and included in the chart is also the axes and we have all of these attributes within those circles okay the second thing that we're doing is scroll handling so that is lower down we have step rendering in this div that has a class of steps we have this scroll component from Russell Samora and each block which renders each of the steps and then third and finally we have hover logic so this is kind of nested right it's right here but we have this if hovered data and this is how we render a tooltip which you'll recall I can do by hovering within my chart so those are the three things that we're going to do and let's start off by moving the initial chart logic this first bit of code that lives in sticky but we'll move that into a brand new component and we'll call it chart dot felt so let's move the div of class the div with the class of chart container and let's find it's open and closed the way I'm going to do this is toggle 72 shut toggle all of its children shut until I get to what seems to be the bottom of it so it looks like my guess would be the bottom is here and go ahead and confirm this works by copying it and hitting save and if your code formatter auto completes that means you did it correctly if it doesn't save if it doesn't auto format that means you probably got the wrong diff okay so these were the divs I wanted so I'm going to copy all of chart container and I'm going to move it over to a new component which I'm going to call chart dot felt so here I'm going to go ahead and paste this in as a div now at first you're going to notice that nothing renders there's no chart there and the simple reason for that is because we don't have this chart component imported so let's go ahead and import chart and we obviously need to import it in our script tag as well components slash chart dot felt now what do we see we're going to notice that the the compiler the plugin is going to tell us hey this won't work because these variables that you're declaring don't exist and the plugin is right they don't exist because they exist in the app dot felt parent but not in the chart dot spelled child so what we need to do is basically find each of these variables with height inner height inner width and import them as props into chart dot felt okay so there are definitely easier ways to do this but what I found is a consistent and safe way is just to see what the compiler complains to you about and then update it accordingly so what do I mean by that it says that width does not exist so I'm going to write export let width okay now I see height is not declared so I'm going to write export let height what do I see now maybe nothing but I do notice that the compiler is still complaining that all these things don't exist hovered data does not exist I'm going to import hovered data okay what is it complaining about now margin current step I saw now what these components which will be totally separate so we'll get into that in a second rendered data x scale okay so let's get those two export let rendered data export let x scale y scale that looks like all of the variables that we need to bring in so we know these are the props that we need to accept from app not felt so let's go ahead and I'm going to go and do something kind of funky here where I'm going to paste all of them I'm going to bulk select by doing shift option and then selecting like clicking my cursor so you'll notice that has a multi-line cursor now and I'm going to delete all text around and then add brackets like this add these mustache curlies which basically is going to pass each of these as variables into chart dots felt now you'll see the chart actually reappears so as simple as this refactor to basically pass the relevant variables into the new chart component using the same syntax as always and now it works as expected but you're going to notice some things don't work like axis x still has some underlying because it's not defined same with axis y in dot fly is not defined tool tip is not there either so what do we need to do we need to go ahead and import those components as well so I'm actually going to just write import and then the names of these components axis x from the components folder axis y from the components folder and tooltip let's see if I can prompt it tool tip from the component folder now actually nothing is complaining to me anymore except this in fly does not exist so we'll go ahead and import fly from spell transition now this entire app is working um as far as we can tell so everything seems like it works but you might notice that some things aren't exactly as we would expect for example as I scroll you'll notice that the transitions are abrupt yet again um in that on step three for example there was supposed to be this hover event that didn't occur you'll also notice if you try to resize the resizing does not happen this is now a fixed chart at 400 by 400 and the reason for that is because some of our variables that were updating in chart dots felt we need to propagate those changes upstream to the parent component as well and a good example of that is width and height right because these are bound to the client width and height and so we need to pass those backup to app dots felt so that our existing x scale and y scale will also update in line so you might recall we did this last module but the way that we want to handle this is by binding our variables to the parent uh component and specifically we're actually going to bind these top three width height and hover data why is that it's because width is a dependency within app dots felt for other things like inner width and x scale height is too for inner height and y scale and then um hover data is rendered down in the if block as well in our dynamic if block and so we also want to update that from the child to the parent so this syntax is pretty simple you write bind the name of the variable in the parent equals the name of the child value so bind height to height bind hover data to hover data you'll then notice it simplifies the shorthand to just bind bind bind and now you'll notice for example as we resize um you might notice there might be a difference but there's still one other thing we need to do to make these variables actually update and that is add some styles so certain css properties need to be applied to chart dots felt because right now there are no styles whatsoever and there are a lot of styles in app dots felt that we just got rid of that are no longer applicable like the circle like the chart container etc so let's go ahead and move these styles over anything that's underlined with that yellow underline is definitely a key candidate for what needs to be copied over right because it's literally being unused in the parent so we'll copy in this chart container and this circle and what these styles enable is now the same transitions you saw the same responsiveness you saw is now going to be applied to this chart so now as we resize the window our width value is being updated that is propagating upstream um and because of these new css rule sets everything is transitioning smoothly so those are the things that we needed to target um and you might also notice that there was this other rule that targets our ticks tick and access title technically because this is a global declaration it could stay in app dots felt but for consistency sake you might want all chart related to code to live in the same component in chart dots felt so we could add this here it's going to be functionally equivalent but it might be nice to keep that in the same style as well now what do we have we have our sticky element and the chart within and then we have um the sticky rule set if you wanted this again is optional like this is the thing about refactoring because there's plenty of ways to be robust about it just about how you want to thank your future self you could also move this sticky parent also to the chart component so maybe we just move the sticky tag here and move the sticky div that wraps around chart as well so then if i wrap our entire application our entire chart dots felt in this sticky div and hit save we're going to notice that this is going to have the exact same behavior everything is working as it should um and what we've done is we basically taken app dot spell from i believe 214 lines of code to now 140 um an app chart dot spell has its own style and its own um length of code which is also long but at least it serves its own purpose and that's kind of the key point of this refactor so our chart dot spell component is now complete and you'll notice everything within here pertains to the chart itself there's no weird step rendering there's no anything odd this is just a regular scatter plot this is almost identical to the scatter plot that we made in module one together right let's move the second bit of code which is going to be scroll handling and step rendering so we're going to create a new component and we're going to call it steps dot felt and this is going to contain all of our steps logic so we'll grab this div copy we'll add steps as the replacement and paste this into our component now we're going to need to import steps like so import steps from the component folder and now let's go through the same treatment so what's what's going wrong well current step doesn't exist so let's pass in current step and accept it downstream i'll open and close a script tag export let current step cool thing here is this is actually the only value that we needed to import now obviously this scrolly component doesn't exist so let's import scrolly from source dot helpers dot scrolly dots felt and you could you know find the exact path right here for example it was dot helpers but recall the components is in its own folder so you'd actually want to go one level further back to find scrolly dots felt now scrolly dots felt exists let's see what happens well something's obviously broken and what's broken again is the styling so we need to go into our css rules for app dot felt find all of our step content so you know these rules and this one below so i'm going to copy these over and move them into our style tag so now that i've copied those over i'll delete them from app dot felt and on ninety nine through one oh three this steps as well i'll move that over and i'll hit save now what happens well as i scroll everything looks identical to how it did in the last application it seems like the problem now is that those changes remember our cool changes between step zero one and two they're not propagating right they're not updating and the reason is because we need a two-way component binding i pass this as a prop but remember the current step is this variable that affects this if block up here so we actually need to use a component binding property binding once again which we'll do with bind current step equals current step hit save and now you notice the application is actually working and it seems like this is functionally equivalent to the old code the differences our app dot felt is cleaner it only has 103 lines of code and all of our steps handling is in its own component all of our chart handling is in its own component now you know you can go through the most satisfying part of any coding refactor and basically delete the unused code so thankfully the s code will tell us if something is unused but we obviously don't need the access imports and app dot felt nor the tooltip import nor the fly transition nor the scrolly component and now see if anything else is grayed out doesn't look like it so in final in our final application we are under 100 lines of code which is a great improvement from our 214 or 41 or whatever it was and we've moved that code to two separate components steps dot felt which is 45 lines of code and chart dot felt which is 104 so we have completed our refactor just to go over kind of what we've done i've put together this final final demo final overview and i've actually had my own spin on the language so here's your little treat for sticking around this long i just wanted to say thank you for sticking with me in this course it means the world and i hope it was helpful and just remember that learning is a process and so if you're overwhelmed by this course don't worry it's just the tip of the iceberg if you thought it was too easy then like good continue learning i just hope that i helped a little bit along the way so thank you for tuning in um and sorry for being cheesy but i thought that would be funny to kind of throw it into the scrolly telling story so thanks so much y'all for attending this course for tuning in hope it was helpful and if you have any questions or complaints or comments or concerns i want you to reach out like i said you deserve it for sticking around this long so the easiest way to reach me is going to be twitter so you can go ahead and find me on here and DM me as you'd like um there are other ways to reach me on like the new line discord but feel free to like open up these direct lines of communication and i just want to wish you the best of luck as you enter this spelt journey and i wanted to thank you for letting me be a part of the beginning of the journey so thank you so much thank you for visiting and feel free to reach out with any questions or concerns and i wish you the best of luck in your spelt and data visualization journey all right thanks so much bye