How to Quickly Import Custom React Hooks With an Index File
This lesson preview is part of the The newline Guide to Creating a React Hooks Library course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Creating a React Hooks Library, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
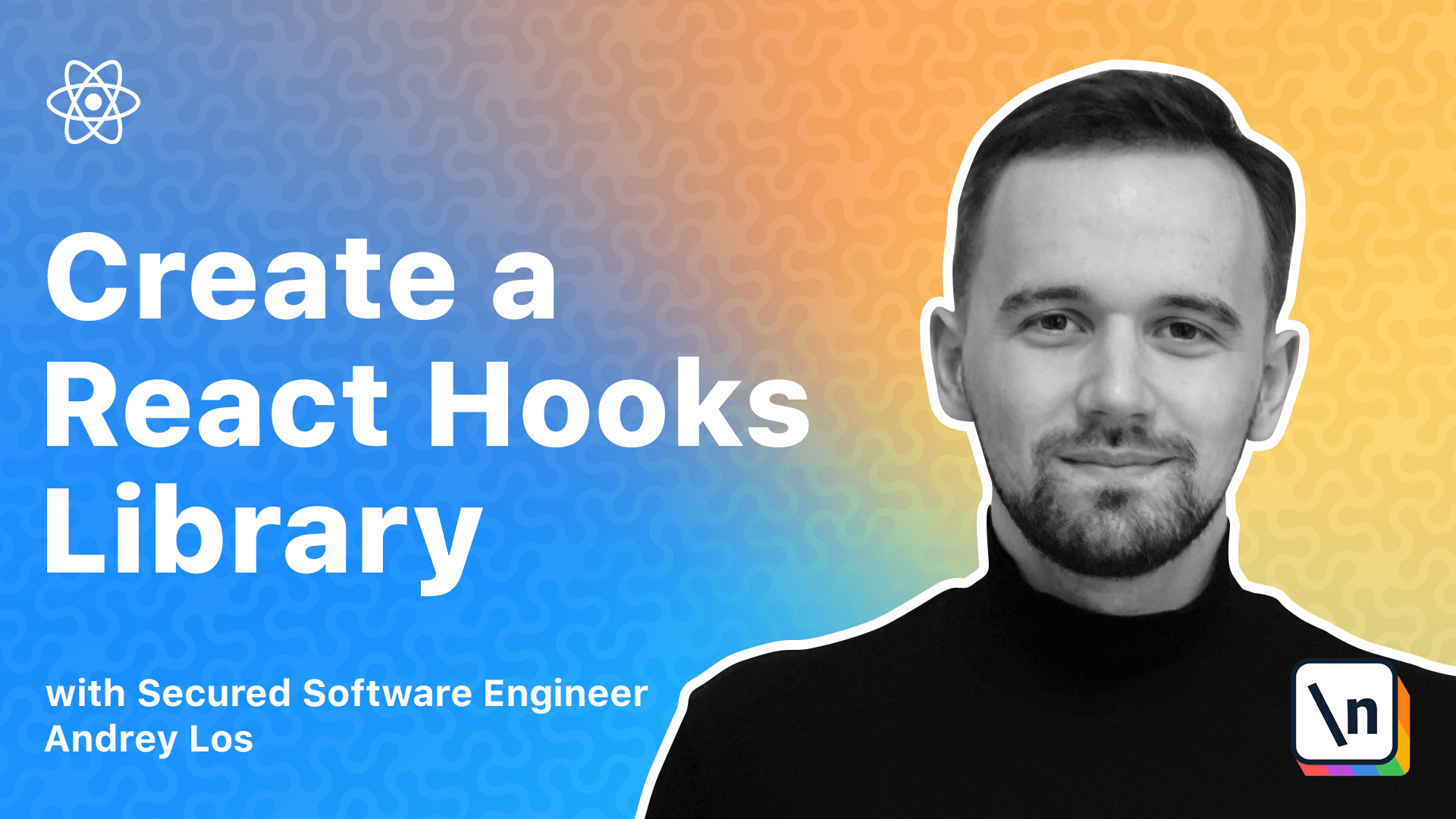
[00:00 - 00:57] All right, so this one is not super important, but I think it's still you need to keep this thing in mind that everything that you develop, every single library, your internal API should be pretty hidden somewhere and you want to usually expose your APIs, your types , etc. In your index file. So what we want to do, we want to re-export everything, all of our functions and types that we want to share with our users that are, you know, it will be our public API. And what we're going to do, so we're going to use an export from syntax to do so. So let me just quickly copy paste the code. So we have our use, build in, you have use, build in, use, build in actions, use map, use map, use map, whatever.
[00:58 - 01:47] So we basically export everything that our user may like to use out here. And you might say, okay, why would we just use the star? And I don't like the star on the, you know, there's a lot of reasons why I didn't like it. First, when you export something, sometimes you don't want to export it. You don't want to, it to become a public API immediately. You want it to be hidden somewhere, or you want it to be exported only because you want to test this and you want to import it in unit tests or something like that. You may have even test data in your files. Sometimes, I mean, which is probably bad, but with the star , it will immediately appear here and it will automatically be seen by your customers, by your consumers.
[01:48 - 02:31] And it's really not a good thing to do. So it's better to keep the list clear and explicit what you expect. What also great about that is that VEPAC roll up in all other tooling that does bundling and actually scans everything that you're using and then removes everything that is unused is really in love with this thing. It will clearly see what things are exported, which things are not exported and it will just understand what is the name of these functions, etc. So for them, it'll be way easier to understand what's happening. Probably even faster your bills will be probably even faster because of that because star.
[02:32 - 02:48] Also, I mean, if we think about the Babel, if you don't know that I will explain later, no problem. But for some people that already know that, it will compile down. If it's a common JS, if it's if it's ES6, of course, it will just keep this syntax as is.
[02:49 - 03:33] But if it is, will be compiled to common JS modules, the modules that are used in node JS, you will have a big problems because all these stars will be compiled into something like for in key, whatever in use map or the name of this requires. So it'll be, I think, a require and it will iterate over keys that requires actually returns. So it's almost impossible. I think, of course, Webpack is not that stupid anymore. And they know how it all looks, etc. So they may probably do this corner cases and handle them. But it complicates things a lot because it's basically a runtime thing. It's not a static thing.
[03:34 - 04:36] Here, you can see by the code, you don't need to execute it to clearly see what is getting exported. If you see four keys from the require, use map something something, imagine you develop a tool and you want to statically kind of check it. It will be just a headache for you because you will have to look up to the file, check what kind of keys are there , which keys are exported. So it's just a lot of heavy lifting for no reason. So explicitly name everything. One also important thing that was added to TypeScript recently is that you can now use an export type. I mean, before you can, it was possible to do just that, you know, export type. But right now you can do this bulk exports like export type. And then here you list all the types you want to expose. So it's also nicer to read because it clearly shows where you have your TypeScript APIs and typings, etc, where you actually export your types.
[04:37 - 06:24] What is also great again is this will never, would say, fool the tool like a backpack or Babel or whatever, because they will see the expert type, they would say, okay, it's a sync not for me, it's a sync for the TypeScript compiler. Family just, of course, it should be a villain. So I can, I will just wipe it out. So it'll be wiped out and you will left out only with these two beautiful functions that are really important for JavaScript because TypeScript stuff will be wiped away completely. And yeah, in case of use map, map entries, or that, etc, it'll be pretty complicated for Babel or for the Webpack or any other tool to understand. Is it actually type or is it the class or is it something that is in the runtime or just something of a TypeScript? So you definitely want to do that. And another thing when you import, when you import your types, let's say from the React hanger in the future, if it's only a type, you can say import type name of the type. All right, so this is a pretty small thing, but it may two wonders for you because it may actually make your bundle smaller because it will also probably make your Webpack another tool and can be faster and more efficient. So that's pretty much it for this one. In the next one, we will quickly implement object-based API, the one that I've been discussing at the very beginning about the decisions about our API decisions that we need to make. And yeah, we'll quickly implement them because it's super easy once you have this area-based one. And we will export them in the same way . Good. So let's go to the next one.