Handling Error Messages
Adding error message handling to the existing TextInput component
This lesson preview is part of the The Approachable Guide to Accessible Components course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The Approachable Guide to Accessible Components, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
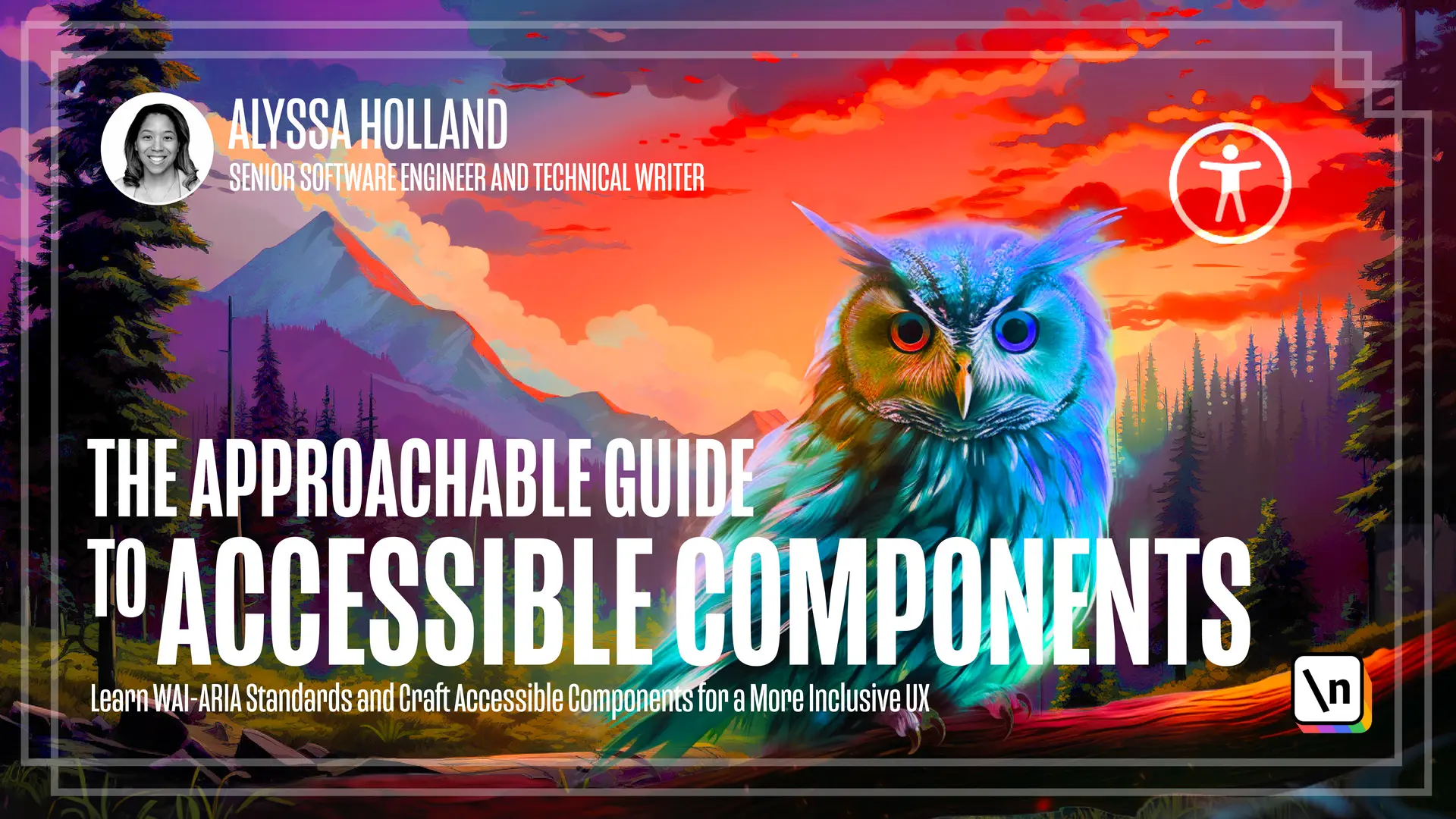
[00:00 - 00:15] Errors are inevitable in any application, and handling them gracefully is essential for a seamless user experience. In this lesson, we'll update our text input component to efficiently surface error messages, ensuring that users receive clear feedback when something goes wrong.
[00:16 - 00:28] To begin, we need to include JSX that will show the error message below the input. The text input component has an error prop that accepts a string value, which we will use to dynamically display the desired error message.
[00:29 - 00:43] Since we'll need to check for errors in a few places within this component, I've added a handy has-error variable that has been predefined and stores a boolean that tells us if there are any errors or not. The openup text input.jsx and add the following code below the input element.
[00:44 - 00:56] The code block does the following things. It conditionally renders the error content based on the has-error variable defined earlier in the file that checks for the presence of any error string.
[00:57 - 01:10] It applies an ID of error message that will be used later on in this lesson to link a specific ARIA attribute to this content. Thirdly, it applies a class name error, which would be targeted in our CSS later to apply error styles.
[01:11 - 01:30] Last but not least, it uses ARIA hidden equals true on the icon, since it is used purely for decorative purposes, and we intentionally want to hide it from the accessibility tree to avoid unnecessary content being passed to assistive technology. To get our error message to display, openupapp.jsx and update what's being passed into the text input component.
[01:31 - 01:43] Let's go under the on-change attribute and add error equals invalid format entered or whatever string you want to display. And this will trigger the error message to get shown.
[01:44 - 01:56] And now that we have our error message being surfaced, let's ensure that people using assistive technology get notified when there's an issue to address. And we'll achieve this by applying the appropriate ARIA attributes, ARIA invalid, and ARIA described by.
[01:57 - 02:13] Let's go back to text input.jsx and tack on the following two lines to the input element. First, we'll add ARIA described by, and if has error is true, we will apply the error message, and if not, we'll return back null.
[02:14 - 02:29] And for ARIA invalid, if has error equals true, we'll return back to the string true, and we'll return back to the string false. So we covered the ARIA invalid attribute earlier in the course, but as a quick reminder, ARIA invalid is used to indicate when the input value doesn't match what the application expects.
[02:30 - 02:37] So in our case, we conditionally set the attribute to true to show that the input failed validation. And when there are no errors, the attribute will be set to false.
[02:38 - 02:47] The ARIA described by attribute helps link descriptive content to the target element. It takes a space separated list of element IDs that describe the current element.
[02:48 - 03:04] In our example, when errors are present, we use the ARIA described by attribute to point to the error message ID, which we added to the paragraph tag holding the error text. When there are no errors, we set the value to null, which removes the attribute from the input element to no additional description is associated with it.
[03:05 - 03:13] Alright, let's do a quick recap. We've got the error message showing up when added and have used the right ARIA attributes to ensure assistive technologies know about the invalid input values.
[03:14 - 03:23] Now, the final part is supplied to the styles to indicate the error state. Since red is commonly used with errors, let's try using that in our error styles.
[03:24 - 03:33] The hexadecimal value for the red CSS name color is this. Let's run that through WebAIMS contrast checker to make sure the red color meets the ratio requirements.
[03:34 - 03:50] And after running the check, we can see that the red doesn't pass either WCAG, AA, or AAA performance for normal text. And since the error text is 16 pixels, falling into the normal text category, we need to pick a different color that meets contrast requirements while still conveying the error vibe.
[03:51 - 04:06] Let's consider a different color that has more of a maroon tint to it, and we'll replace a current foreground color with a following hexadecimal color . Now let's check that within the checker and see if the new color meets the contrast requirements for error text.
[04:07 - 04:19] Awesome, the new color successfully passed both guidelines, making it a good option for our error text and input border color. Now that we've selected our error color, open up text input.css to update the styles when an error state occurs.
[04:20 - 04:32] And in this case, we'll apply that maroonish color to the input text color and border input color. And we're utilizing CSS attribute selectors to target the input when rinvalid equals true.
[04:33 - 04:41] The beauty of implementing with an accessibility mindset is that it's not just for JavaScript and HTML. It seamlessly extends into CSS, making the entire workflow harmonious.
[04:42 - 05:02] And now you can see when the error styles are being applied, the input border color has that maroon tint along with the text color within the input. And when we inspect the accessibility tree, we'll see that rinvalid true is being applied, and the area described by message is being linked appropriately.
[05:03 - 05:10] Throughout this lesson, we aim not to rely solely on color to convey meaning. In this example, we employ two different techniques on displaying the error message.
[05:11 - 05:28] Textually, we surface the error messages strategically by positioning it near the location where the error occurred and ensuring proper association for screen readers by applying the appropriate ARIA attributes. Stylistically, we enhance the error presentation with features like a maroon border around the input and maroon text for the error message.
[05:29 - 05:36] Additionally, we included an error icon to make the error more visually noticeable for sighted users. A great job on implementing our accessible text input component.
[05:37 - 05:40] Now our component can gracefully handle a wide range of scenarios.