Optimizing Web Application Performance with Page Architecture in Next.js
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Next.js Complex State Management Patterns with RSC course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Next.js Complex State Management Patterns with RSC, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
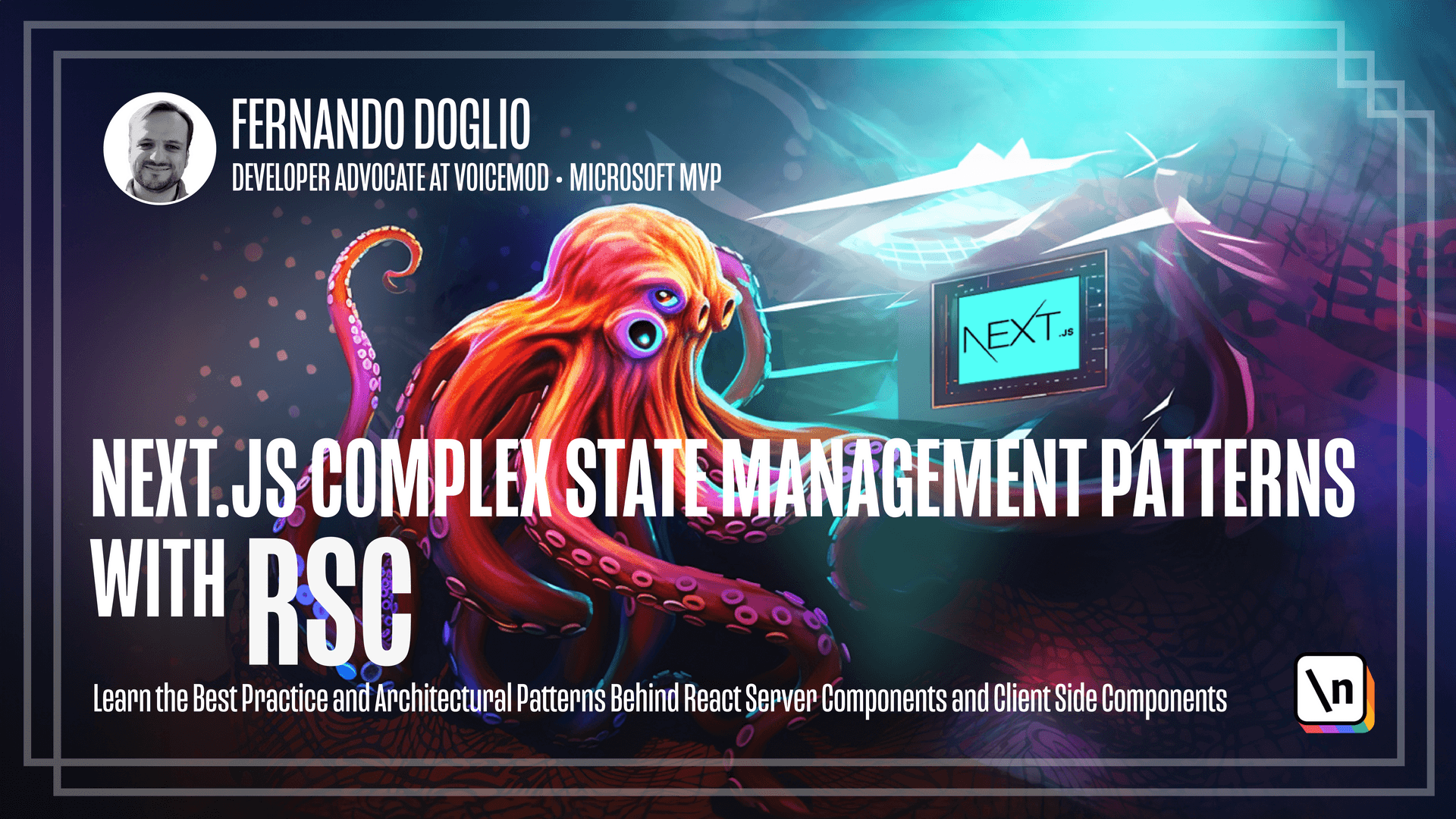
[00:00 - 00:24] We're at the brink of discovering how page architecture, or should I say "page architecture", in NextJS can transform, the way we build applications. In this lesson, I'm going to show you how to organize your code into server components and client components in a way that makes sense and optimizes both the developer experience and the user experience.
[00:25 - 00:34] So what exactly is page architecture? Imagine constructing a building where each piece that you put has a specific function, location, and purpose.
[00:35 - 00:46] A sturdy page architecture similarly places the bulk of the HTML or JSX within the walls of server side component, essentially. Laying a strong foundation for the entire application.
[00:47 - 01:19] What I'm trying to get at here, and this is something that I will be repeating throughout the entire course, is that server components should be the building blocks of your web application. If you're coming from previous versions of next or just traditional React, you're used to building client-side components and putting all that logic, your business logic, or at least your UI-related logic inside them and mixing and coupling potentially some aspects of that logic that should not be on the client side.
[01:20 - 01:34] Now with a new version of next, 13, and even now with the latest release of 14, and the incorporation of server components as the default building block when you're creating an application, when you're building new components. We want to shift the bulk of that UI code.
[01:35 - 01:51] We want to shift it into the server, if it makes sense. That way, and we're going to get into more details in further lessons, you optimize the developer experience by simplifying the way in which you write and you envision the interactivity with the users.
[01:52 - 02:11] And also improve the user experience by providing an optimal and more performant output by improving metrics related to the speed at which the page is loading. Because as we're going to be looking at further videos, server components allow for much faster and much more dynamic rendering of your pages.
[02:12 - 02:36] And coupled with the concept of page architecture, you will be able to properly optimize the way in which you write the code so that the minimum amount of JavaScript gets shipped to the client and as much work as we can do on the server, gets done on the server. Here you can see an approximation of what I mean by improving the experience of the user.
[02:37 - 03:07] The main thing that we want to reduce here is not code because you're still going to be writing probably a similar amount of code, but the amount of that code that gets shipped to the client is significantly reduced when you're using server components because a lot of that code gets executed and stays in the server. It's no longer shipped to the client where it needs to be executed in order to hydrate the components like we used to before.
[03:08 - 03:23] Now the majority of that code is going to be executed on the server and we're just going to get the output of that code, essentially the render components. There are many bad practices when it comes to architecting your page, now that we have a building block such as the server components.
[03:24 - 03:50] One of them, and it's one of the main reasons why I thought about creating this course, is when developers are just faced with blockers trying to use server components and decide to just go with client components for everything. That means you cram every piece of code into your client components including the code that doesn't really change or require any interactivity from or with the user, essentially.
[03:51 - 04:11] If a piece of code is not doing anything dynamic, essentially updating its data or interacting with a user, then it's definitely something that should not be in a client component and we'll get into more details about that. Another very common bad practice when it comes to architecting your page is performing data fetching within a client component.
[04:12 - 04:25] Again, this is a very common practice if you come in from previous versions of this technology, but now we have more reliable and easier tools to use. Here is an example of a component that is trying to do everything.
[04:26 - 04:36] It's trying to fetch the data from the API, process it and also show it. However, there are different ways in which we can solve this by properly architecting our page.
[04:37 - 04:46] One potential solution would be to split that client component into two, a server component and a dynamic client component. The server component is in charge of data fetching.
[04:47 - 04:59] In this case, I'm simply putting a URL there, but I could be performing a database query, for example. The point is that this component is pre-rendered the first time it gets fetched .
[05:00 - 05:11] So that query is executed within the server. The data is received within the server and the HTML generated is also generated inside the server.
[05:12 - 05:34] You don't see any client specific code here and we'll get into that down the line as well, but the point is that this server is performing the data fetching and pre- rendering of the static portion of the component. We then can use this component within a client component, like we see here, and the client component would fetch a pre-render version of our profile.
[05:35 - 05:47] So we're no longer adding data fetching code to our client component. We're no longer dependent on an API endpoint that we created within our app simply to perform data fetching.
[05:48 - 05:59] And our client component is slimmer, easier to maintain and only focused on the interactivity part. In this case, simply adding a button to set a fake editing option.
[06:00 - 06:15] By focusing client components solely on interactivity, we avoid the common pitfalls, like mixing presentation code with business logic or coupling data handling with UI transitions. This is something that we want to avoid at all costs.
[06:16 - 06:25] Link client components mean better maintainability, clearly, less code to write, less code to maintain, and are easier to develop. They just need to focus on one thing.
[06:26 - 07:04] Picture, if you will, if you want to still try to figure out a way to visualize these two types of components, picture a cross-section, picture a cross-section of a building, where each floor is bustling with people going about their day, just doing whatever they're doing. Those are the client components, interacting with users, trying to show dynamic behavior, trying to show dynamic data and underneath it all, the foundation that keeps the whole building standing, those are the server components, static, never changing and not requiring any interaction with the users.
[07:05 - 07:16] To wrap it up, a solid page architecture isn't just about performance. It's about creating an application that it's leaner, more maintainable, and ready to scale.
[07:17 - 07:28] We avoid the classic pitfalls of bad practices by separating concerns and delivering content Giving our users and developers the best experience possible. So great work so far.
[07:29 - 07:44] We're now equipped to start understanding how to architect our page. We still need to go through other basic concepts before we get to a proper understanding of how these components interact with each other, but we're going to get there.
[07:45 - 07:58] So in the further modules, we're going to get deeper into the world of server components and client components, and we'll compare each other to make sure that we understand fully the difference. So, until then, happy coding and see you in the next one.