Video Course Overview - Modernizing an Enterprise React App
Introduction to Modernizing an Enterprise React App
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Modernizing an Enterprise React App course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Modernizing an Enterprise React App, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
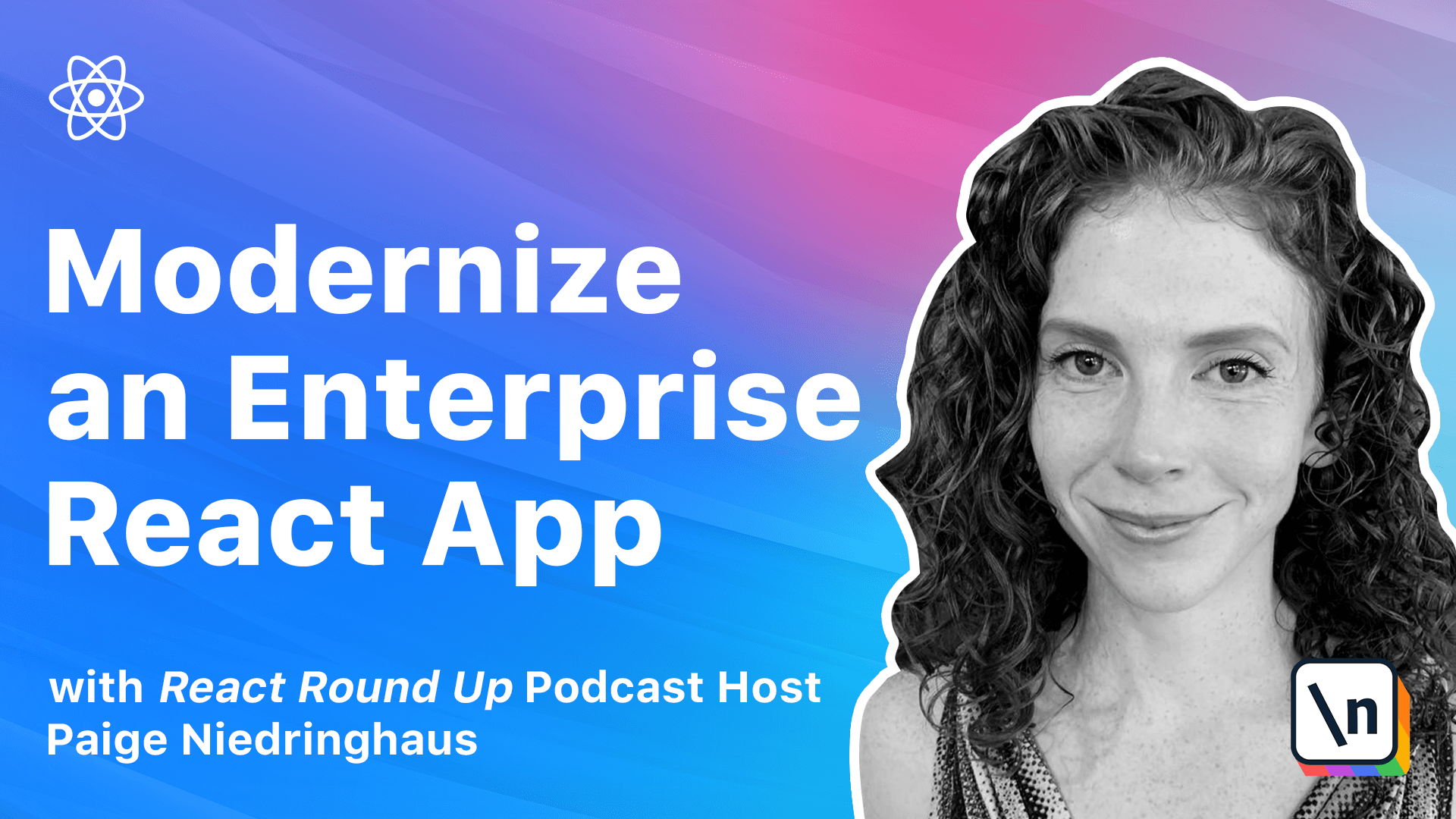
[00:00 - 00:07] Welcome to the New Line Guide to Modernizing an Enterprise React application. I'm the course author, page-neadring house.
[00:08 - 00:25] Many courses and tutorials begin building React applications from scratch, using the latest and greatest features that framework has to offer from the beginning. They also tend to gloss over things, like structuring an app's source code, so it can grow and be maintained in an organized fashion over time.
[00:26 - 00:49] How to organize and write automated unit tests and automated end-to-end tests. How to set up code standards in a project, so that the whole team adheres to linting and code formatting best practices, how to leverage component libraries to speed up development cycles, and most importantly, how to upgrade an existing application to take advantage of the latest advances that the framework has to offer.
[00:50 - 00:59] That is not this course, because that is not real life for many developers. Many developers join development teams and are introduced to an existing React application.
[01:00 - 01:10] The application may have been worked on by many other devs at the point that they inherit it, and none of those original developers may still be with the project. It may be out of date by a few versions or more.
[01:11 - 01:32] It may lack consistent structure or coding standards because it was built without an architectural design discussion. It may lack what are now considered industry standards, like automated testing, but that's okay. That's all okay, because we're here to help arm you with the knowledge of all of the above to improve this app and leave it better than when you found it.
[01:33 - 01:46] To get the most out of this course, there are a few prerequisites I would recommend you have. I think that an intermediate understanding of HTML, CSS, and modern ES6 JavaScript is pretty critical.
[01:47 - 02:02] Also, a basic knowledge of the React framework, at least up until the point hooks were introduced. Some familiarity with JavaScript automated testing frameworks, things like Jest , Enzyme, and Cypress will help you to get the most out of this course.
[02:03 - 02:11] Now, let's take a look at all you're going to learn in the next 10 modules. For module 1, we will start off easy.
[02:12 - 02:29] We will just do an introduction to React hooks because a lot of people, although they may have used hooks, may not feel very familiar with them, very comfortable with them. You may be very comfortable with classes, but maybe you just haven't had the opportunity to really dive into hooks and get a good feel for it.
[02:30 - 02:45] So, we're going to go over how hooks operate, especially the ones that don't necessarily line up in a one-to-one comparison with React's class-based components, like use effect or use state. In this module, we will learn about why hooks were introduced.
[02:46 - 02:56] We'll walk through some examples with various hooks that are simple and easy to get started with. We'll go over some of the most commonly used ones, like use state, use effect.
[02:57 - 03:25] We'll talk a little bit about use ref because that's one that I've found myself reaching for on various occasions, use context, and finally, we will talk about custom hooks, which everybody likes to talk about, but doesn't necessarily do a great job of describing, as well as help you understand some use cases where custom hooks really are the right choice to reach for. With module two, we will go into actually upgrading our sample application.
[03:26 - 03:35] We'll use the newest version of Create React app, and we will lock in project dependencies. You'll see more about what I mean when I say this when we get there.
[03:36 - 03:46] React 17, we're still not quite out of the beta stage for 18, so we will probably be upgrading to a version of 17. We will upgrade the version of React.
[03:47 - 04:06] We will upgrade the version of React scripts, which is what Create React app runs on, and we will troubleshoot any initial upgrade issues that we might encounter. Additionally, we will start to lock in our node and yarn versions, and we're going to use a tool manager called Volta to help us do that, as well as node engines.
[04:07 - 04:18] So whenever any developer downloads and runs this app, they'll have the same development experience that any other developer on the team might have. And you're going to be impressed with this.
[04:19 - 04:31] This is really a cool thing that Volta makes possible, and I'm excited to show you that. With module three, we are going to configure our prettier and our ES-lint setups for our application.
[04:32 - 04:49] So nothing, I can say this, honestly, changed my development experience for the better, like adding prettier and ES-lint to my normal development flow. I'm honestly not quite sure how I got along first long as I did before these two things existed, but I know that I never want to go back to that now.
[04:50 - 05:32] So in this module, I will show you how to create a prettier RC file that will be included in the project, along with any personal formatting preferences that you like, and I'll also show you how to configure VS Code, which I hope is your editor of choice to automatically format code on Save or when on switching focus in different tabs. Likewise, we're also going to have a lesson on setting up an ES-lint RC JSON file, which uses the standard ES-lint configurations that are developed by Airbnb for both React and React hooks, as well as how to modify the ES-lint rules to suit your team's preferences, needs, rules that you want to enforce versus ignore, things like that.
[05:33 - 05:46] It's really going to make everybody's code more standardized, and it's going to help you write better quality code. So for module four, we are going to actually dive into refactoring our class components and start using hooks.
[05:47 - 06:08] So a big part of modernizing an existing React app hinges on being able to take a traditional class-based component, maintain its current functionality, and convert it to use hooks under the hood. So taking advantage of all that the upgraded dependencies have to offer, but still keeping the same level of functionality that we had before.
[06:09 - 06:36] So this module will consist of going through about five or six of our files that are class-based components currently and upgrading them to use hooks, but still do the same things that they were doing before. And I think you will be pleasantly surprised at how smoothly this can go, especially if you have more of a strategic way that you approach this, and I will share with you mine so that you can figure out what works for you.
[06:37 - 06:49] With module five, we will create custom hooks for our app. So custom hooks are a key change that comes with the React upgrade, and it's time to make a few that will support this application.
[06:50 - 07:24] So for this module, we will be looking at some places in our app where maybe code is duplicated or it is more complicated than it probably needs to be inside of a particular component, and then we will pull that logic out, put it into custom hooks, and share it in the way that we need to so that all of the application pieces that need that info can get it. And with module six, we will build onto that, and we will start using the context API and the use context hook to make state access within our application easier.
[07:25 - 07:50] So we don't necessarily need a global state management library for this size of an application. Redux is the one that properly comes to mind. Recoil is another new one that Facebook introduced not that long ago, and there are still definite benefits that we can use with React's built-in state passing mechanism, not state management, but state passing mechanism, which is called context.
[07:51 - 08:43] So in this module, we will gain a better understanding of what React's context is good for and what it is not, and then we will add some contexts to our own application and refactor it so that there is less prop drilling, which is taking a piece of information from a very high-level parent component and passing it through several levels to get to the child that needs it, and we will create context providers to hold that state and use context hooks to consume it elsewhere in the application. We'll also learn how not just to pass state, but also functions that update that state in the parent component from a child component's actions, and you'll see more what I mean when we get to it. That was always one of the things that kind of tripped me up as I was learning about context, and so I really want to point that out to you so that you understand how to tackle it when it becomes necessary.
[08:44 - 08:52] So in module seven, we start getting into testing at this phase. Automated integration testing with just and React testing library.
[08:53 - 09:25] So as you know, getting an application to work correctly today is only half the battle because we need these applications to be stable, long-lasting, they need to be rock solid because they are enterprise-level applications that are potentially supporting hundreds of thousands of users or more. So the other half of that battle is ensuring that it continues to work as expected, even as new features are added, new system requirements come up, new levels of performance and scale are reached, and that is where integration testing comes into play.
[09:26 - 09:47] So these lessons, we will discuss the automated testing benefits and why React testing library and Jest have become the de facto unit and integration testing frameworks of choice. We will share integration testing best practices and advice for organizing tests in the app, like working from smaller components up to larger ones.
[09:48 - 10:05] We'll download all the needed libraries and dependencies to test the app and configure it to easily run tests and check code coverage with just a few simple NPM scripts. We'll learn how to set up mocks and supply test as needed, and we will write tests for our existing components, hooks and services.
[10:06 - 10:16] You are going to get a lot of hands-on testing in this module, so get ready. If you're at all on shaky ground with it, you won't be by the end.
[10:17 - 10:38] In module eight, we will dive into end-to-end testing with Cypress because while individual unit testing is fantastic and it's definitely an important piece of the puzzle, it is not the only sort of testing that can be done. End-to-end testing is critical because if a user can't use the application as intended, then what's the point of building it?
[10:39 - 10:49] This is what end-to-end testing does best. It simulates what a user would do by interacting with the browser as a user would, and Cypress is the best of the end-to-end testing frameworks available today.
[10:50 - 11:08] In this module, we will cover why end-to-end testing is also an important piece of the automated testing puzzle that enterprise applications need to live up to , and where it falls in the testing hierarchy, which I will get into in more detail in that lesson. An introduction to Cypress I/O is what will happen next.
[11:09 - 11:15] The current favored end-to-end testing framework of the JavaScript world, you will see why. It is fantastic.
[11:16 - 11:43] We will go through downloading all the necessary libraries for the app to run Cypress, and we will configure our application to run Cypress tests a couple of different ways, depending on what the needs are, whether you're doing local development or you have this running for some kind of a CI/CD pipeline. We will set up fake data, mock API calls, target DOM elements to trigger events , and debug any misbehaving Cypress tests with the Cypress test runner.
[11:44 - 11:57] We will explore the new Cypress Studio feature, which allows users, like us, to teach Cypress tests by having them observe a user interacting with the browser. Trust me, when I tell you, I hadn't tried this up until this course.
[11:58 - 12:02] It is awesome. It is so cool, and it is such a time saver.
[12:03 - 12:07] You will really, truly love it. Finally, module 9.
[12:08 - 12:21] Our last module where we teach actual new content, this is a bonus module that I wasn't sure I would be able to finish. With our bonus module, we are going to implement the ant design system to speed up our design and development.
[12:22 - 12:56] Ant design is one of a few extensive, well-designed design system libraries that allows designers and development teams to build and iterate faster because it provides flexible, attractive components right out of the box that developers can just plug into their React applications and be up and running. Design systems like this help reduce the amount of time that development teams spend hand-coding complex functionality, like tables, filtering, trees, things that a lot of people will end up using, but they don't have to reinvent the wheel.
[12:57 - 13:09] They also help the design team maintain consistency within the application because you already have a library of fully built components. You just need to plug them together almost like Legos and you're ready to go.
[13:10 - 13:23] So this bonus module will introduce design systems and we'll discuss some of the benefits that they can provide to designers, developers and larger organizations. We'll look at some of the more popular ones, including ant design.
[13:24 - 13:44] And then we will add ant design to our application and identify some components that would benefit from being switched out with the ant design versions. So we'll work from the more simple components, things like buttons, inputs and the like and up to the more complex ones that utilize the ant documentation to understand how.
[13:45 - 14:08] So it'll be a good learning experience because while ant design is extremely powerful, it's not always the easiest thing to get started with, so you will now know how to integrate as well as how to strategically start swapping out things that you need that you may have previously built with the ant design version. So by the end of this course, you should be ready to take on the world.
[14:09 - 14:37] Well, that might be a little bit of an overstatement on my part, but if you go through this whole course by the end, you should feel much more confident about taking any existing React application, no matter how new, how old, how large or small and maintaining and improving upon it. You should feel confident that you can set up project configurations, you can use hooks, you can write tests, you can basically quickly get up to speed with any React- based code that you might encounter.
[14:38 - 14:54] And as React is the most popular JavaScript UI framework currently available, this will make you an asset to almost any development team. So now we're going to get into some of the course downloads, code examples and local development requirements that I recommend as we're going through this course.
[14:55 - 15:08] So as I may have previously said, this course is going to focus on an already functioning React application, but it is built using a very outdated version of Create React app. I believe that it is version one dot something.
[15:09 - 15:22] We're up to version four or five now in Create React app, so this is definitely back there. So to run this application locally, you will need a relatively recent version of Node.js installed on your machine.
[15:23 - 15:37] I would recommend anything that is Node.js 12 or above, which you can download right here at the node.js.org. Just go ahead and grab whatever OS you are running and download that, if you haven't already.
[15:38 - 15:43] That will also automatically install NPM, which is the second requirement. So NPM or it's Yarn equivalent.
[15:44 - 15:57] Yarn is just another package manager if you're not very familiar with it. So as I said here, NPM is installed automatically when Node.js is downloaded, but I'll be using Yarn throughout this course because it's a bit newer, it's a bit faster.
[15:58 - 16:12] They're very interchangeable though, so whichever you feel most comfortable using, I'll try to give examples and command line commands for both. But if you want to download Yarn and haven't already, I am using the classic Y arn one, not Yarn two.
[16:13 - 16:19] So you can download it here at classic.yarnpkg.com. Go ahead and grab that.
[16:20 - 16:32] The project source code, which you can download right here if you just click this button. I have already downloaded it, but it is coming in a zip file and then you can unzip it and open it up on your local machine.
[16:33 - 16:41] You will need a code editor or an IDE, an integrated development editor of your choice. I hope that you're using Visual Studio Code, which is my favorite.
[16:42 - 16:54] It's free, easy to use. I have some plugins that I like to use with it from the VS Code marketplace, so I will be showing how to get set up with those, and I would encourage you to use it as well to get the most out of this course.
[16:55 - 17:12] You can download it from code.visualstudio.com and just find the OS download for your particular platform. And the last thing that you will need is a command line terminal like iterm2 for Mac OS users or Windows terminal for window users.
[17:13 - 17:29] The VS Code has a built-in terminal that works very well, which is what I prefer to use, but for those few times where you do need a separate command line integration tool, iterm2 is typically what I go for. And you can get that for free at iterm2.com.
[17:30 - 17:34] So go ahead and download something like that if you don't already have an editor. Okay.
[17:35 - 17:40] So I think now we are ready to get started. So you've downloaded all the dependencies that I listed above.
[17:41 - 17:51] You have downloaded and unzipped the source code, which I have another link to right here. So it is time to open up the first version of hardware handler in your IDE of choice.
[17:52 - 18:02] So now that we have hardware handler open, let's talk a little bit about what you will see in here. We have both a client and a server folder here.
[18:03 - 18:19] Since this course is focused almost exclusively on modernizing a React web app on the front end, I just spun up a very simple backend server that's filled with dummy data. So if we open this up, we see this db.org.json file.
[18:20 - 18:26] That is where all of our information that will populate into our application lives. So you don't really need to deal with that.
[18:27 - 18:38] Just know that that is acting as our backend so that we don't have to have a fully fledged express application running back there. So that's about all that's contained in the server folder at initial download time.
[18:39 - 18:48] But once the app starts up, you'll have a local file named db.json, which we can also see right here. That will be created within the server folder.
[18:49 - 19:00] And the data in db.json file is changed via the app. So for example, when new products are added to the app or products are removed from our checkout, which you'll see shortly, this is where that happens.
[19:01 - 19:09] So the client folder is really what we want to focus on. Now I'm going to cover the contents and structure of this folder in more detail in the next lesson.
[19:10 - 19:24] So the first thing that we're going to want to do to start our application is install our node modules and start the server. So once you have opened up your repo, open a new terminal, and check that you have the correct versions of node and yarn needed.
[19:25 - 19:46] So if I open up VS code, I can go up here, create a new terminal, and pretty easily, let me clear out that, make it easier. We can do node -v and yarn -v to check what we've got.
[19:47 - 20:01] And it looks like we have version 14.17.2 running for node and 1.22.10 for yarn . So that's great. That is perfectly fine. We'll work just fine with our application.
[20:02 - 20:16] So once we have confirmed those node and yarn versions, we are ready to install our project dependencies locally. So in the command line, we will just cd into the server first because that will be quicker and run yarn.
[20:17 - 20:26] And this will do a yarn install of all of our package dependencies there. And then we will back out and go to the client and run yarn once more.
[20:27 - 20:38] And this will install all of our React application dependencies for the front end. Okay, so now we have finished doing our React install and it is time to start up.
[20:39 - 20:49] So remain in the client folder and go to yarn start. And we will start to see things happening in the database.
[20:50 - 20:58] And it looks like everything has gone according to plan because we are now seeing the home page for hardware handler. So that's awesome.
[20:59 - 21:10] This is exactly what you want to see. We have links to our various other pages, my products, add new products, the checkout, all this good stuff. So we are up and running and we're ready for our next lesson.
[21:11 - 21:19] So in the following lesson, we will walk through what hardware handler can do and how it's structured under the hood. I will see you then.