Refactor Home.js
We dip our toes into converting a class component to a functional one with Hardware Handler's home page.
This lesson preview is part of the The newline Guide to Modernizing an Enterprise React App course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Modernizing an Enterprise React App, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
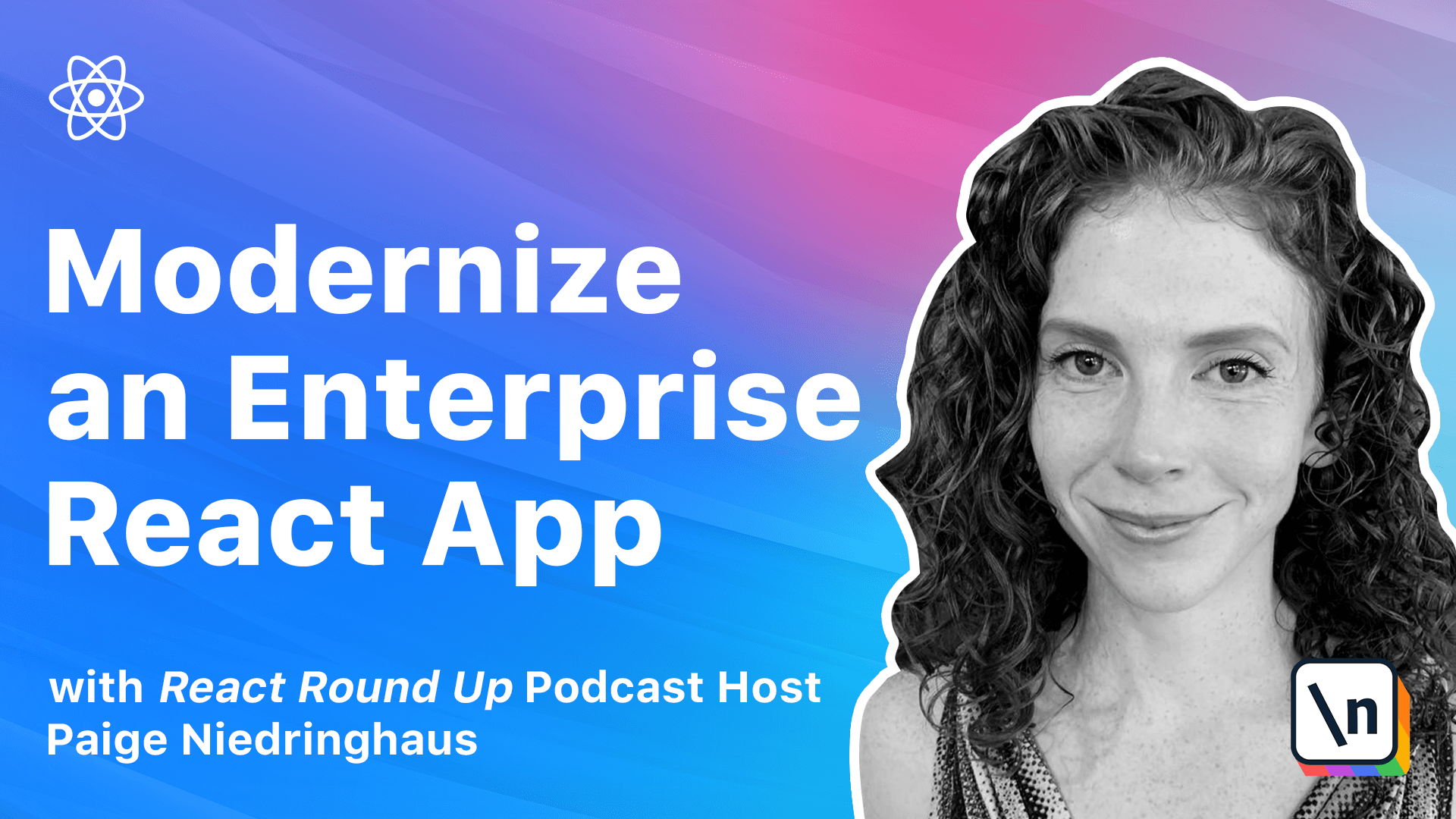
[00:00 - 00:13] I realized as I was looking over the code and my ES link config was working over time, that although I made the home component a class-based component, it really doesn't need to be. Why you might ask?
[00:14 - 00:18] Well, this component has no state of its own. It doesn't even accept props.
[00:19 - 00:27] Oops. I bet that you'll notice when you're looking through your own React code bases that you've got class components that don't really need to be that way either.
[00:28 - 00:31] But that's fine. It's not a big deal at all.
[00:32 - 00:41] This should make converting this component to be a functional version and easy win for us. It's a great way to get started on our journey to refactoring our app to just use hooks.
[00:42 - 00:56] So in this lesson, I'll teach you my recipe for converting class components to use hooks, giving us a roadmap to follow for every component we encounter thereafter. So open up your IDE and let's get to work.
[00:57 - 01:15] Before we start actually refactoring our app, component by component, let's talk a little bit about my recipe for refactoring components. As I've converted more and more class components to functional components in React applications, I've come up with a sort of recipe that I like to follow and I want to share that with you now.
[01:16 - 01:24] So the first step in my recipe is going from class to function. This is simply converting the class to a function and removing the render method.
[01:25 - 01:32] Second, I'll tackle the state. I'll take this.state objects and replace them with use state hooks and update any props.
[01:33 - 01:42] Next, I like to refactor the life cycle methods. So I update the component life cycle method to use effect or potentially to custom hooks.
[01:43 - 01:49] After that, it's class methods becoming regular functions. So any local functions that are present in our component.
[01:50 - 02:03] Then I fix the JSX, tidy it up so that it works with the components newly functional state and there are no this dot states left hanging around inside of it. Then regression test, we need to test the functionality to make sure everything still works as expected.
[02:04 - 02:18] Fix any ES lint errors that my code will be telling me about thanks to ES lint setup and rinse and repeat for however many components you need to convert. If you just follow these steps, it should go down pretty smoothly.
[02:19 - 02:43] So feel free to adjust this as you get more comfortable with React hooks but this works well for me and it's the way that I'm going to go through updating each one of these components in these lessons. So as I outlined above, when I'm converting class components to functional ones , I like to start by changing the class declaration to an ES 6 arrow function of the same name and removing the now unnecessary render method.
[02:44 - 02:49] So let's switch over to our application now and get started. Okay.
[02:50 - 03:01] So here we are in hardware handler. I have already installed everything and we are just going to start this up so that we can keep track of if anything breaks while we're doing this refactor.
[03:02 - 03:16] So we're already inside of the client folder. So go ahead and just run yarn start and we should see it open up in a browser and everything should work initially.
[03:17 - 03:30] Everything looks to be okay. And now let's navigate to our very first component which is actually going to be the home.js component inside of your client source containers home.
[03:31 - 03:44] Open that up and there is a giant red angry squiggly across all of this. ES lint is very upset but it's already telling us the problem that the component should be written as a pure function.
[03:45 - 03:51] It just so happens that that's the first thing we're tackling. So this error should disappear when we're done with this step.
[03:52 - 04:10] So the first thing that I'm going to do is get rid of this class home extends component and this render method. It's going to just become a const of home in the traditional arrow function way .
[04:11 - 04:22] We can also delete this extra curly brace that's now unnecessary at the bottom and we're going to save this. And thanks to prettier it has cleaned it up even further.
[04:23 - 04:39] So you might be wondering where did the return statement go? Well, our new home function doesn't have a return statement and that's because this particular function can have an implicit return because it doesn't receive any props which you can tell by looking at this.
[04:40 - 04:48] And there's no data manipulation before the component is rendered in the browser. This is purely a functional component that displays.
[04:49 - 05:06] If it did need to manipulate any data before displaying its JSX then the curly braces and the return statement would come back but in this case they can be completely emitted. So the last thing that we can do if you scroll up slightly we can delete both react and component from our import list.
[05:07 - 05:21] Since we're using react to version 17 the import from react line at the top of the component is no longer required for components that only render JSX which is awesome. It feels good when I end up deleting more lines of code from a file than I add.
[05:22 - 05:35] And with that we are done with this particular file and we are ready to move on . So before we go check the browser one more time, click around, make sure that the links still work and take you to different pages but everything should be in order still.
[05:36 - 05:37] So that wasn't so terrible right? It was a good warm up.
[05:38 - 05:50] It's a good refresher for how functional components look and it gives us a taste of what to expect as refactor our other class based components. So the next file that we'll cover is the app component at the root of our project.