The Pull Payment Pattern - Withdraw payments when outbid
Also note that any sort of math like this _should_ be checking for overflows, which we'll talk about later.
This lesson preview is part of the Million Ether Homepage course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Million Ether Homepage, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
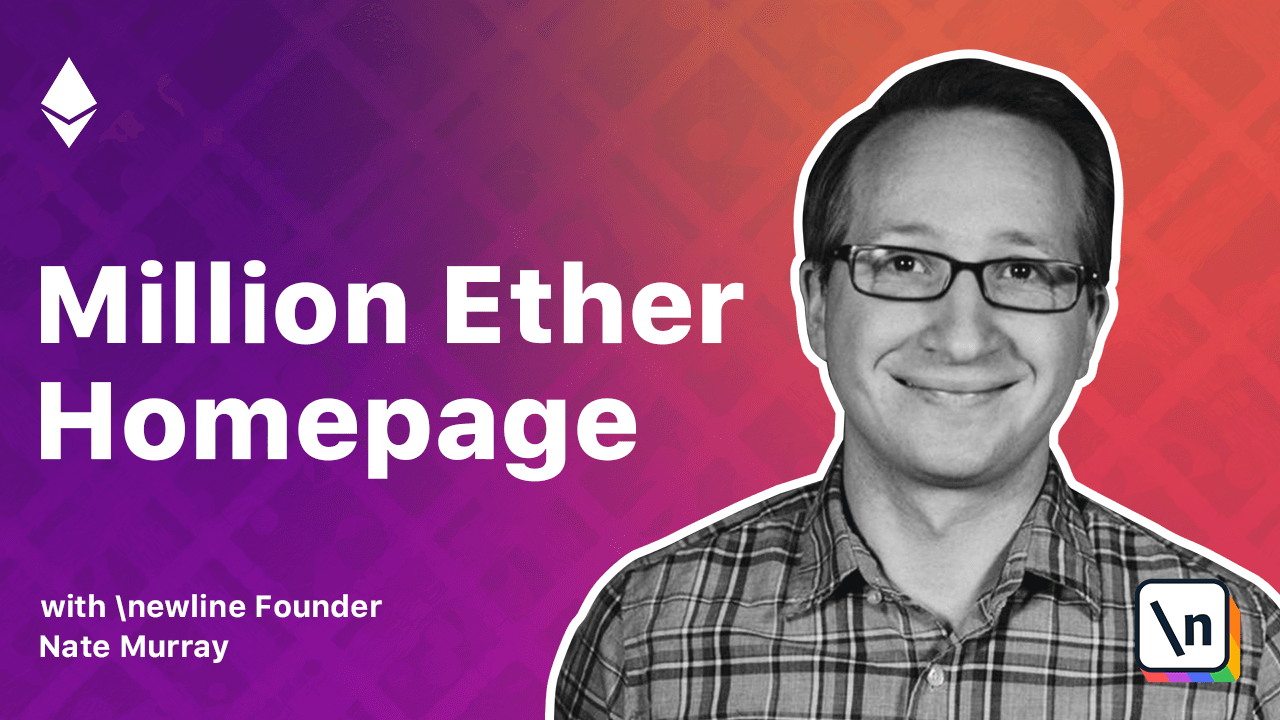
[00:00 - 00:06] Let's get back to the million EtherPage contract. Now that we have a grasp on how to send payments, let's issue refunds when a pixel is outbid.
[00:07 - 00:18] Taking a look at our color pixel function, the obvious solution would be after we verified that the new bid price is higher than the sold price, we should dot send the funds back to the previous owner. That code might look like this.
[00:19 - 00:27] So we check that the message value is greater than the pixel sold price, and then we call pixel dot owner dot transfer pixel dot sold price. That looks pretty clean.
[00:28 - 00:35] But wait a second, what do you think about this code? Are there any problems or potential attacks?
[00:36 - 00:53] Remember that dot transfer will revert all changes if sending the funds fail. So if an attacker could craft a contract, which buys the pixel, and then always deliberately fails when you try to send it value, the result would be that no one could ever buy that pixel again.
[00:54 - 01:04] Every time someone outbid them, this contract would try to transfer the funds. But the transfer would fail, which means the contract would fail to instate the new bid and recolor the pixel.
[01:05 - 01:23] This sort of situation also comes up whenever you're looping over sending payments. For example, if you have a for loop where you need to send payments to several different contracts, make sure one contract failing won't prevent the addresses later in the loop from receiving their funds.
[01:24 - 01:30] This problem shows up in Ethereum smart contracts all the time. So much so that the solution has a name called the poll payment pattern.
[01:31 - 01:45] The idea applied here means that instead of sending the Ether refund in line, that is when a new pixel is purchased, we keep track of pending refunds internally. Then the account that receives the refund must submit a separate transaction to pull the funds out.
[01:46 - 01:52] Let's implement this pattern in our contract. We'll start by adding a pending refunds property, which is a mapping from an address to an amount.
[01:53 - 02:01] This is a lot like the list of accounts we had in our bank contract in the earlier video. It's a mapping from an address to a Uint, it's public, and we'll call it pending refunds.
[02:02 - 02:12] When we color a pixel, instead of sending the Ether directly, we'll credit the pixel owner's account with a refund. First we check that the pixel owner is not an empty address.
[02:13 - 02:21] And then we add on depending refunds, the old pixel owner's original price. Again, remember this doesn't transfer any Ether out of the contract.
[02:22 - 02:29] This is just internal bookkeeping. And also note again that any sort of math like this should be checking for overflows, but we'll talk about that later.
[02:30 - 02:38] And lastly, we'll write the withdraw refund function. And also, I want to mention that this function is based off of the Open Zeppelin Poll Payment Library.
[02:39 - 02:48] You can find the URL in the notes. So the function withdraw refunds, first, takes the pay as the message dot sender, and we look up the payment in pending refunds.
[02:49 - 03:01] We require that the payment not be zero, and we also require this dot balance be greater than the amount of the payment. This dot balance is the amount of Ether that belongs to this contract.
[03:02 - 03:14] Next, we zero out the pending refunds for this payee, then we send the payee the payment and require that it succeeded. If it fails, we revert all of our state changes so far.
[03:15 - 03:20] Let's try it out. First, we can deploy this contract.
[03:21 - 03:32] Now let's buy a pixel for one Ether. Now let's try buying that pixel for fewer Ether from the other account.
[03:33 - 03:52] Notice that it doesn't run, and it returns quickly too. And that's because a remix will try to run the transaction locally before sending a real transaction to the network.
[03:53 - 04:16] Now let's check the pending refund for our original account. Great, we can see our refund here.
[04:17 - 04:27] Now let's try to withdraw our refund. It worked.
[04:28 - 04:32] Notice the balance of Ether in our account went up. Let's check our refund balance now.
[04:33 - 04:35] It's zero, great.