Drawing Pixels from the Blockchain
This lesson preview is part of the Million Ether Homepage course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Million Ether Homepage, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
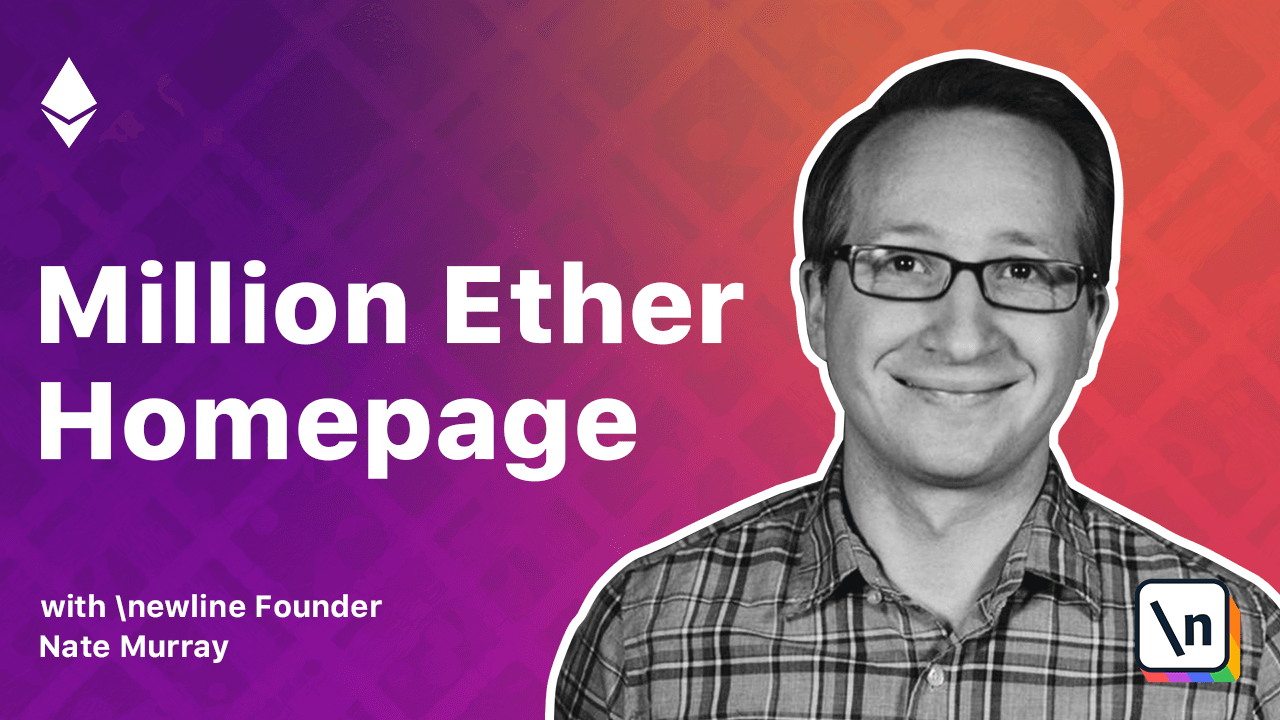
[00:00 - 00:09] We have pixel-changed events coming over web sockets and we need to draw them on our canvas. What we need to do is capture each event, parse the color string, and then write the pixel to the canvas.
[00:10 - 00:18] Let's take each step at a time. First, take a look at the event that comes through. The x, y, and color are all strings, but we want them all to be integers.
[00:19 - 00:32] Actually, x and y are plain integers and we need to parse the color into three integers, RGB, each one being between zero and 255. Let's write a function that, given an event, will parse these values and write the pixel.
[00:33 - 00:42] We'll name this function, write pixel with event, and it takes an event as an argument. And then from that event, we'll extract the x, y, and color from event.return values.
[00:43 - 00:53] Then we want to write the pixel. We haven't written write pixel yet, but we will. We'll type write pixel, we'll parse in x, parse in y, and we need to parse the color.
[00:54 - 01:02] We'll write parse color too. Let's write parse color now. Pars color will take this raw color string, split the bytes, and convert it to a three element array.
[01:03 - 01:11] Each element corresponding to a channel, red, green, or blue. You don't need to worry too much about how this function works. Just know that it returns a three element array.
[01:12 - 01:20] Now we need to write this particular pixel to the canvas. What we're going to do is read the current image data, find the index for this pixel, and update its color.
[01:21 - 01:31] Let's write the write pixel function. It takes three arguments, the x position, the y position, and the color. And let's log those out. First, we'll grab the existing image data.
[01:32 - 01:37] And then we'll grab the width and the height from the canvas. And notice that the canvas is in scope here.
[01:38 - 01:44] Now finding the index is a little tricky because the image data is a one- dimensional array. How do we find the index in a one-dimensional array?
[01:45 - 01:55] First, we'll take the y value times the number of columns plus the x value times four. Y four because we have one byte per channel per pixel.
[01:56 - 02:03] If that seems confusing, try to work it out on paper. But honestly, understanding the layout of this image array in memory is not that crucial to building this app.
[02:04 - 02:13] This is how you find the index. Now we will assign the pixel in data each channel of our pixel in color, the first one being red, then green, then blue.
[02:14 - 02:20] And I want every pixel to be opaque, that is no transparency. So I'll set the alpha channel to 255.
[02:21 - 02:25] If you don't do this, your pixels will be invisible. And lastly, we put the image data back onto the canvas.
[02:26 - 02:33] You might read this and realize that we're rewriting the canvas on every new pixel. You could certainly batch these updates, but we're not going to for this video.
[02:34 - 02:45] Now, all we have to do is change our event subscriptions to send the events to write pixel with event. All right, we don't need this anymore.
[02:46 - 02:59] And whenever a new pixel changes, we'll write pixel with event there. Here we'll type events dot map and then pass that to write pixel with event.
[03:00 - 03:10] If your page reloads and all you see is a white screen, look closer. A pixel is a pixel, no matter how small.
[03:11 - 03:16] And if you zoom real close, you'll see pink on the wall. But if we add more pixels, it's easy, you see.
[03:17 - 03:29] We just type 340x0c. We have two pixels rendering.
[03:30 - 03:37] Let's refresh the page and they should still reappear because we're using the same function to load old events as reading in new events. But these pixels are too tiny to see.
[03:38 - 03:41] So here's an idea. Let's buy an entire image.