Implementing Favicon Modification Functionality
This lesson preview is part of the Master Custom React Hooks with TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Master Custom React Hooks with TypeScript, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
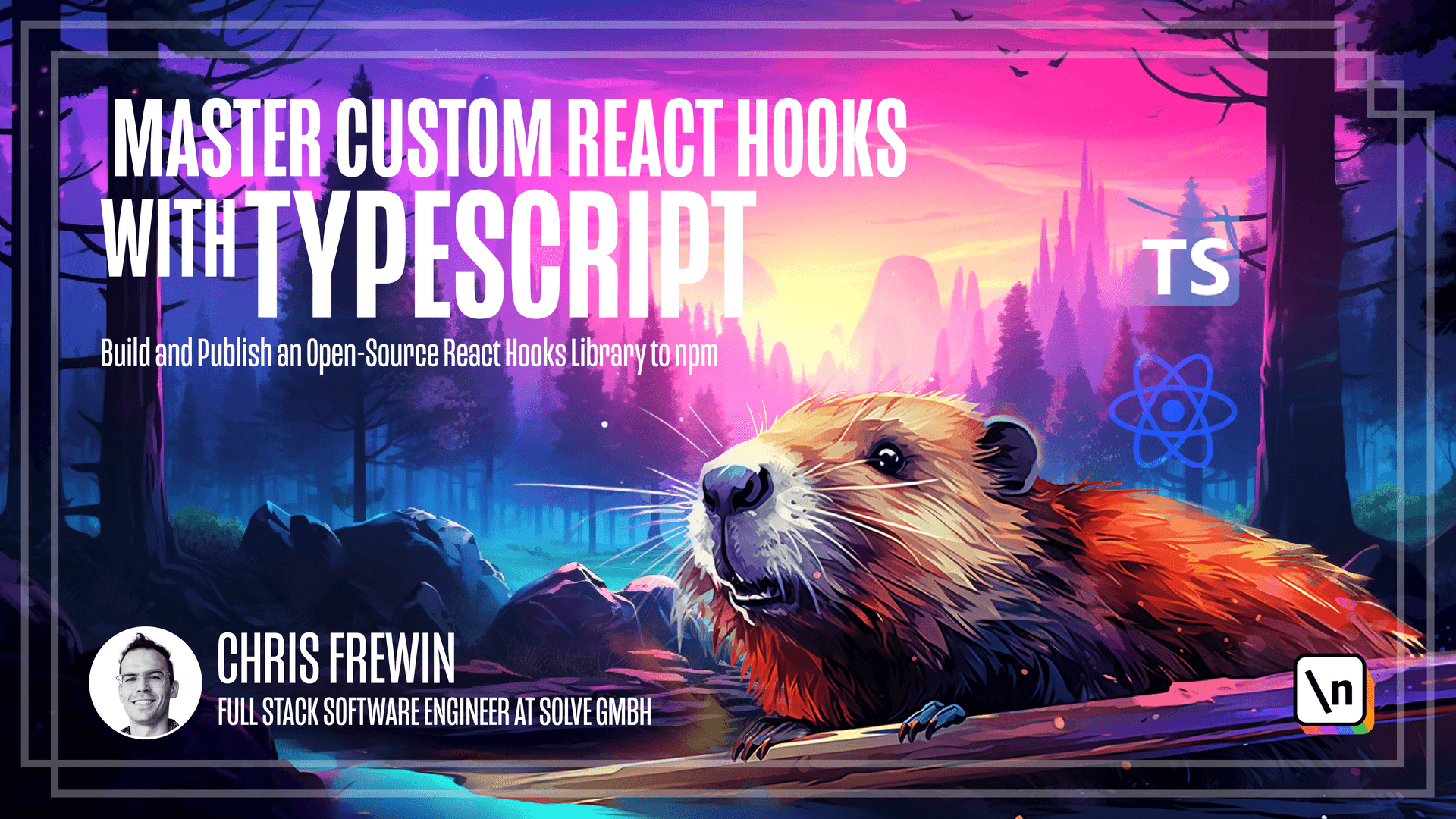
[00:00 - 00:13] Let's also allow iteration through favicons via the hook. Let's create a new hook called use favicon change effect.
[00:14 - 00:31] This will end up looking quite similar to use title change effect. We'll export const, use favicon change effect.
[00:32 - 01:03] This hook will take a parameter called favicon links, which is of type string array, and should iterate favicons, which is type boolean. We first need a state variable to keep track of the current favicon index, so I 'll just call that favicon index, and the initial value will be zero.
[01:04 - 01:22] We're also going to set up a ref for the favicon since every time we need to use the favicon, it's not very efficient to search through the entire DOM to find it. I'll just call it favicon ref, and use ref.
[01:23 - 01:52] This will be of type HTML link element within initial value of null. Similar to what we've seen in use title change effect, we're going to use interval, calculating the next index by incrementing the current favicon index.
[01:53 - 02:13] And if the next index is already at the end of our favicon links array, then we 're going to start back at zero. Otherwise, we can continue on with the next index.
[02:14 - 02:32] We'll also use a interval of 500 milliseconds, and we'll pass on this should iterate favicons parameter to toggle when the interval should run. favicons can be stored in a variety of ways and formats.
[02:33 - 02:42] We're going to create a custom function to try and find the favicon in the DOM. So we'll create a new folder called utils.
[02:43 - 03:02] And within this folder, we'll create a new function get favicon.ts. This function is going to hopefully return an HTML link element.
[03:03 - 03:15] But in the case that it can't find anything, it will return null. We would expect the favicon to be a link tag and also with attribute rel of icon or short icon.
[03:16 - 03:50] So first we're going to get all the nodes that are of type link. Then we're going to loop at this list and check if the link tag that we're currently looking at.
[03:51 - 04:27] If that tag has a rel attribute and if it's equal to icon or it's equal to shortcut icon. So if this is the case, we will return that element.
[04:28 - 04:45] If we come all the way down to here, that means we haven't found one and we'll return null. Now back in use favicon change effect, instead of passing null as the initial value for our favicon ref, we can call our get favicon function.
[04:46 - 04:57] And now we can write the use effect hook that actually updates the favicon. So this is use effect.
[04:58 - 05:21] And similar to what we've already seen in use title change effect, we're going to hook into the favicon index. And every time that changes, we will set the favicon ref current and the ref value of the favicon links passed in at that favicon index.
[05:22 - 05:33] We can import this from react and format this hook. And now let's finally add use favicon change effect to use please stay.
[05:34 - 05:53] Just like the other custom hooks we've written, I'm going to add a comment. Modifies the favicon of the page whenever should iterate titles is true.
[05:54 - 06:12] So use favicon change effect, favicon links, and should iterate titles. And of course we have to add these favicon links as a new parameter, which is type string array.
[06:13 - 06:21] So we're ready to test this new functionality in the example app. So first we'll rebuild.
[06:22 - 06:31] We'll move into the example app. And to just make sure it's a clear test, let's just do the loop animation type.
[06:32 - 06:47] And we'll do we heart react. And alternate that with we heart new line.
[06:48 - 06:54] And the corresponding favicons here. One comes from react.js.org.
[06:55 - 07:12] This also happens to be the default create react app, favicon, and the new line , favicon. Let's format this so it's a bit easier to read.
[07:13 - 07:29] And we can start up our example app. So we've got our default title here and we expect when we open this new tab for both the title and favicon to change simultaneously.
[07:30 - 07:38] And that's exactly what happens. So we successfully built a way to change the favicon dynamically along with the title.
[07:39 - 07:55] In summary, we created a new use favicon change effect that was largely formatted like our use title change effect. We created a custom get favicon function that helps us find the favicon in the DOM.
[07:56 - 08:16] We added use favicon change effect to our main use please stay function, which also required that we expose a new parameter to use please stay, which is this favicon's string array parameter. We then rebuilt our hook and tested it in our example app and we saw that it was working as expected.
[08:17 - 08:22] In the next lesson we're going to allow adding the interval time as a parameter to our hook.