Implementing a Marquee Functionality
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Master Custom React Hooks with TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Master Custom React Hooks with TypeScript, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
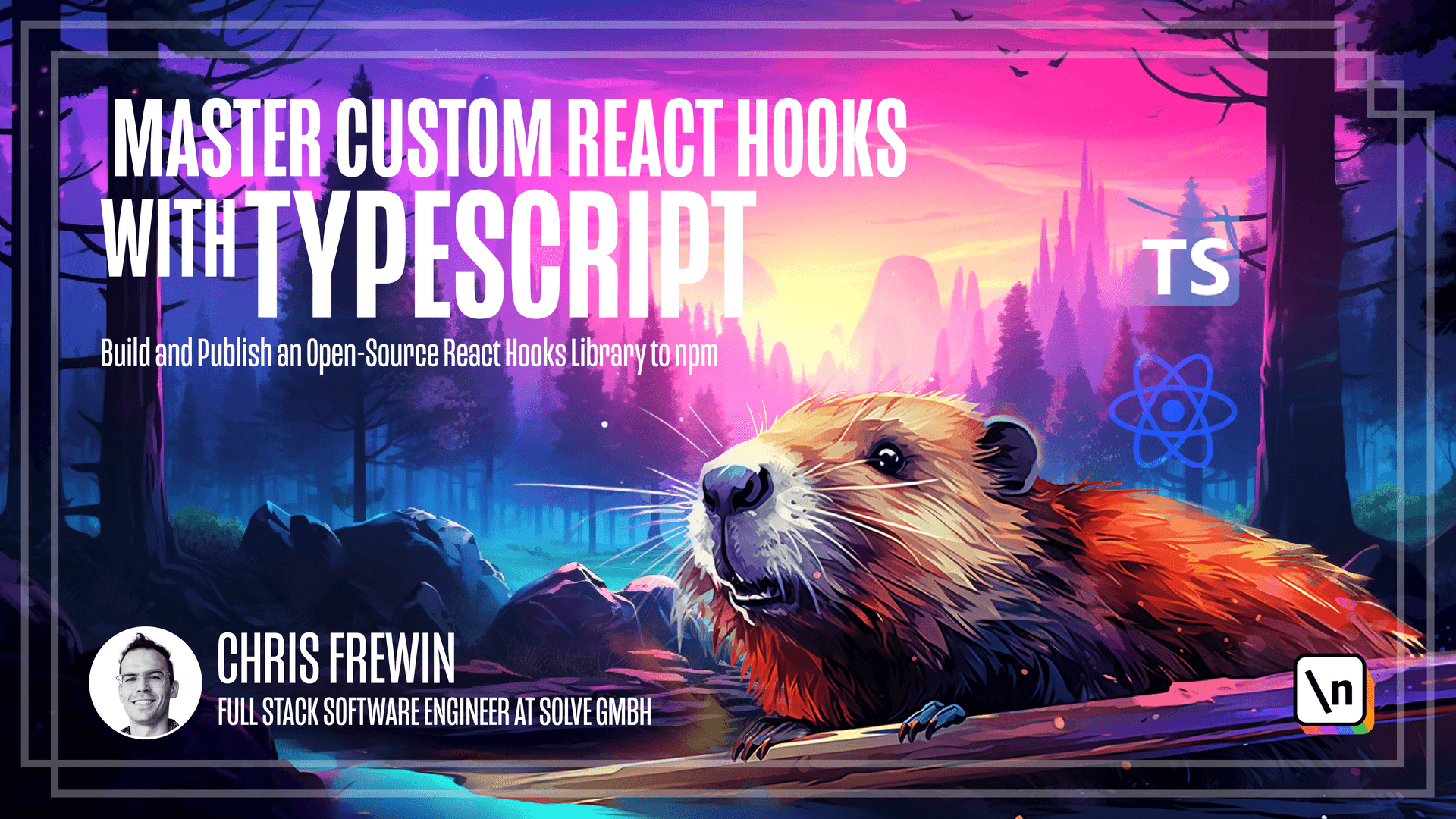
[00:00 - 00:08] Let's build a title marquee effect now. We will have an array of letters and we need to shift them from left to right in the browser tab.
[00:09 - 00:27] Just like the title looping and cascading letter functionalities, we will need to write both an iteration function and a title modification function within use title change effect. Now within Chrome, the maximum amount of letters that can fit onto a browser tab is about 30.
[00:28 - 00:43] For Firefox, it's a bit longer, but it's pretty similar and just a little bit longer at about 35 characters. We're going to take the shorter length for now to run our marquee as it'll also be compatible with Firefox.
[00:44 - 00:58] The first thing we'll do is define a new Constance folder. We'll create that under SRC, Constance, and a new TypeScript file called Constance.
[00:59 - 01:15] And we'll create a const here tab character count, which will be 30. In use title change effect, let's now write our iteration and title logic functions.
[01:16 - 01:31] I'm going to create a new function called run marquee iteration logic. And the logic here is fairly straightforward, like the other functions, just for improved readability.
[01:32 - 01:49] I'm going to save the next index as a const. And in the case of a marquee, we want to check if we are at that tab character count, which we just created in our Constance file.
[01:50 - 02:04] So if we are, we want to start back at the beginning. Otherwise, we are free to move on to the next index.
[02:05 - 02:19] We can then start to write our marquee title logic function. So that's run marquee title logic.
[02:20 - 02:34] And within run marquee title logic, we first need to check how many characters carry over beyond our character count. So I'll call that carry over count.
[02:35 - 02:51] And that's just the current title index plus the total length of the title. And then minus that tab character count.
[02:52 - 03:05] Now, if this is greater than zero, we need to do a bit more fancy calculation with our title. So first, we'll have a space text, which separates the start and end of our marquee.
[03:06 - 03:33] And that can be calculated by repeating the white space character. And it's tab character count minus the title length.
[03:34 - 04:10] And with that calculated, we can set the document title. So the document title will be the current title minus that carry over count all the way to the end of the title. So title, stop length. Then we have our space text.
[04:11 - 04:34] And then the rest of the remaining title. So that's substring again. But in this case, we're going from the beginning to what is left.
[04:35 - 04:52] Now, if carry over count is not greater than zero, then all we need to do is set the initial space in front of our title. So we'll first store an offset value again using this Unicode white space character.
[04:53 - 05:06] And we just need to repeat that the title index number of times. So the total offset is this current title index that we're offsetting by. And we have that many white space characters at the beginning of our string.
[05:07 - 05:23] And so the title becomes this offset plus the title itself. So we can clean that up. And we should be all set with the run marquee title logic function.
[05:24 - 05:40] Now, before adding these two new functions into our switch cases, let's hop into animation type and add this new animation type. Which we know is marquee.
[05:41 - 05:57] And back in use title change effect, let's add these new cases. So when it's marquee or the iteration logic, we of course want to run the marquee iteration logic.
[05:58 - 06:11] And the same for the title logic when our animation type is marquee. We want to run the marquee title logic.
[06:12 - 06:23] We should be all set with our new marquee functionality. So let's rebuild our hook. MPM run build.
[06:24 - 06:46] We'll move into the example folder. And within app.tsx, let's do a new marquee title setup. So I will change this to I am a marquee.
[06:47 - 06:57] And let's run the example app. And if we open the browser and we lose focus, we of course see our offset functionality doing its job.
[06:58 - 07:12] And as we get towards the end of the tab title, we see the carryover. So it looks like our marquee is working perfectly.
[07:13 - 07:22] As a summary, we first created a run marquee iteration logic function. A run marquee title logic function.
[07:23 - 07:38] We added a new animation type marquee and leveraged this new animation type value in both of our switch cases within use title change effect. And we rebuilt and tested our hook to make sure that everything was still working.
[07:39 - 07:44] In the next lesson, we'll see how we can also do favicon changes using our hook .