Installing React and React-DOM as peerDependencies
Installing dependencies for the Scroller library.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Creating React Libraries from Scratch course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Creating React Libraries from Scratch, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
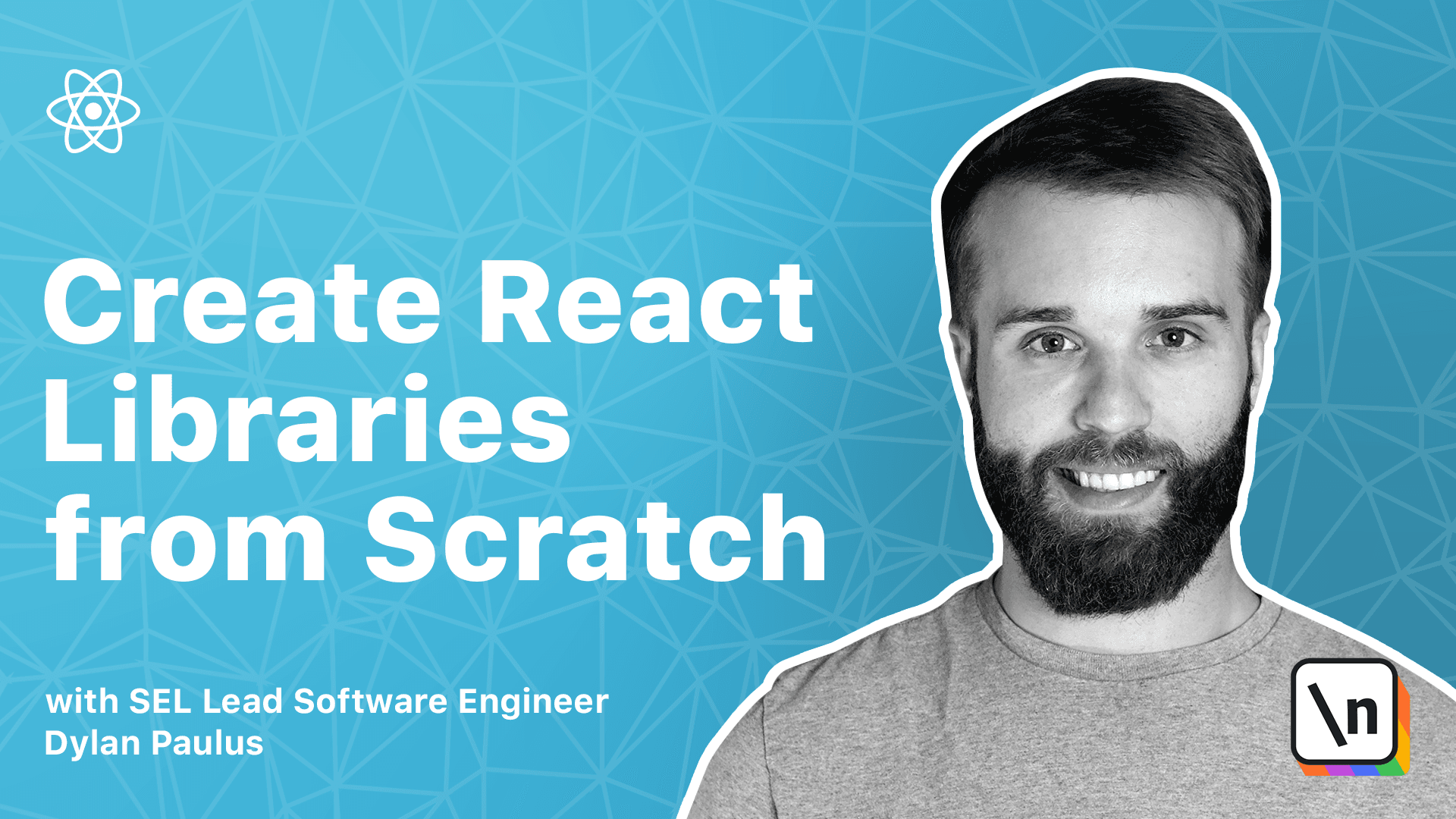
[00:00 - 00:11] Now we're ready to install dependencies for our library. We don't want to include React and React DOM in our package directly, but instead have whoever uses our library provided themselves.
[00:12 - 00:21] In this case, we'll be installing React and React DOM as peer dependencies. In the same directory as package.json, run YarnAddReact.
[00:22 - 00:29] React DOM --peer. Hit enter.
[00:30 - 00:41] As you can see, we don't need to run separate YarnAdd commands for each dependency. YarnAdd takes single or multiple packages to install by separating the package 's names by whitespace.
[00:42 - 00:49] Next, we'll need to install any development dependencies we'll be using. This may seem weird, but bear with me. In the same directory as the package.
[00:50 - 00:57] json, run YarnAddReact and React DOM. -dev.
[00:58 - 01:03] Yes, we're installing React and React DOM twice, but not really. Remember in the last lesson when we talked about peer dependencies?
[01:04 - 01:14] These are libraries we expect the consumer of a library to provide, a contract. This means we won't be including or unbundling React and React DOM in our library, so why do we install them as dev dependencies?
[01:15 - 01:19] Well, to be able to test the code we write is correct. We need a way of using React in the development setting.
[01:20 - 01:29] So we include React and React DOM as dev dependencies. Don't worry, both packages only get installed to the local disk once because dev dependencies don't get downloaded.
[01:30 - 01:37] Naturally, for a React library, we'll be needing React and React DOM. But what does ES build?
[01:38 - 01:49] ES build is a code bundler, which means it takes care of creating a published build over library. ES build will include any imported code, take care of building different module formats, and use ESM and common JS.
[01:50 - 01:59] Don't worry, we'll talk about these later, and provide optimizations like modification and dead code removal or getting rid of code that's not being used . There are a few other bundlers available, including Webpack and ROLA.
[02:00 - 02:05] Webpack and ROLA are more generally used and support a wide range of functionality. ES build is a new kid in the game.
[02:06 - 02:15] It's written entirely in the program language Go with a focus on speed. It's currently in the early stages development, but ES build has all the features we need for bundling our library.
[02:16 - 02:23] After running the Yarn add command, you may find some to audit our project. We have a new file called Yarn.lock.
[02:24 - 02:31] What does this do? Yarn.lock is a file that our package manager uses to ensure versions of the package don't change between installations.
[02:32 - 02:58] npm has a similar file called package.lock.json or shrink wrap.json. A Yarn.lock file contains things like a package hash or integrity that makes sure someone doesn't publish two separate instances of a package using the same version. It also stores the exact version of a package being used because we can define dependencies using version ranges, i.e. the carrot or greater than less than sign.
[02:59 - 03:06] Yarn.lock is an important file to prevent dependencies from breaking our code. As such, this file should be included in source control. In other words, git.