How to Write an Atomic Component in Storybook
Writing the atomic components.
This lesson preview is part of the Storybook for React Apps course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Storybook for React Apps, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
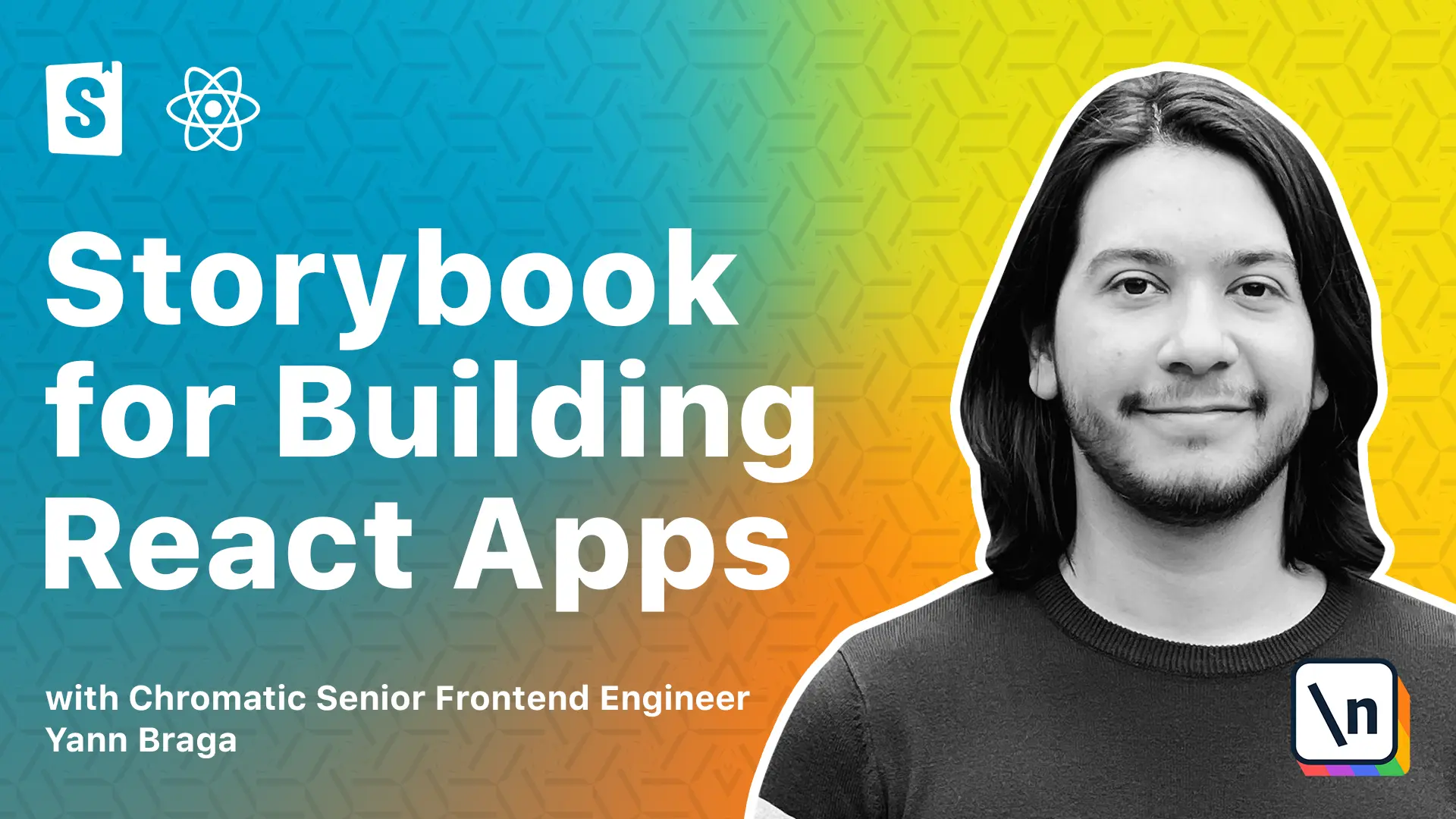
[00:00 - 00:12] Given the components we identified in the previous lesson, let's create the most atomic ones, the review and the badge component. The badge is a pretty straightforward component that does not contain any variation, so let's start with that.
[00:13 - 00:25] You will find a base template for the component already done for you under source, components and badge. Because we don't want to just put this anywhere randomly in the application just to develop it, let's use Storybook for that.
[00:26 - 00:46] We should collocate the stories file right beside the badge component, so let's add a badge.stories.tsx file, and let's add a minimal template for us to see the badge component there. So we first of all import from Storybook React because we're using TypeScript and there are helpful types called component meta and component story.
[00:47 - 01:04] And Storybook needs a meta which is a default export that contains a title as well as a component. The component we passed the badge component which comes from our file.
[01:05 - 01:22] And the title should be reflecting the folder structure of where the component is in, so that would be components/badge. Then in order to make everything more type safe, we could cast the default export as component meta.
[01:23 - 01:32] And as a generic, we pass the type of the badge component. This will automatically read the props from our badge component and provide them in the ARX property.
[01:33 - 01:42] So let's just add the text here and call it "comfort food". And this arc will be passed down to every single story in our file.
[01:43 - 02:01] So let's create a template which is going to be receiving ARGS and passing those ARGS down to the badge component. But in order to get type safety here, we should also cast this to a component story of type of the badge component.
[02:02 - 02:13] This way the ARX will be inferring the correct props. And then once we create our first story, so let's export a named export called default which is a cloning of the template.
[02:14 - 02:25] And then if we ever wanted to add some ARGS and such, we could do defaulted ARX , for instance. And then because of this type, we get type safety here.
[02:26 - 02:36] We could also get automatic type safety and documentation for these properties, which could be pretty helpful for us. But in this case, we don't really need that.
[02:37 - 02:49] So let's open up the terminal and run Storybook. Now on Storybook, on the left side you'll see in the sidebar there's a new story, which is the badge component, which is rendered correctly.
[02:50 - 02:59] And on the right side you should see an add-ons panel with the controls. If you don't see it, you can press the A key in your keyboard, or you can click here and there will be an option to show the panel.
[03:00 - 03:16] The control add-ons allows us to do adding some stuff, and then the component will react to these changes. And by the fact that we added the component to the Story meta, it also means that Storybook is able to get information from the story, such as the props and their types.
[03:17 - 03:28] And also be able to show a very interesting snippet on how this component actually is used. So if you actually change the values here, it will also update the JSX for people to use it as a reference.
[03:29 - 03:46] Now let's get back to the component and add some styles to it. I'm going to be pasting the snippet, which is also part of the lesson description, and I'm importing style components, creating a container, which is just collared with some fun styles and such, and then wrapping our text with that.
[03:47 - 03:53] And once I come back to Storybook, we see that the badge is done. So that's pretty much it for the badge component for now.
[03:54 - 04:05] So let's go ahead and implement the review component. Just like the badge component, we start with the base template for the review component, under source, components, and then review.
[04:06 - 04:17] In order to see the base template, let's add a story to it. Once again, we add a collocated story called review.stories.tsx, and we do the same kind of boilerplate we did before.
[04:18 - 05:01] So we're going to import some types from Storybook React, which are component, meta, and component story. We are going to import the component, as well as export our default, which is the meta, which is going to contain a title of components/review, a reference to the component, and we're going to cast it as a component meta of type review component. In this example, we're not going to be using the arcs in the meta level, because we don't want the same arcs for every story, because the review component might have different stories.
[05:02 - 05:25] So let's create a template of type component story, type of review, which receives arcs and passes down to the review component. We start by creating our first story, so export const default, which is just going to be template.bind.this.
[05:26 - 05:51] So we save it, and then coming to the browser, we see there's a new story, which has our review component, although it just says hello world, so let's work on the template. So let's check the review component and add some logic to it. I'm going to collapse the terminal because it's not really helpful, and I'm going to paste the logic here that you can also find in the course description to explain what 's going on.
[05:52 - 06:11] So essentially, we now have a method called get review, which if there is no rating, it just says no reviews, but then depending on the rating, the review will slightly change. So once we save this file, and we go back to storebook, we see that the component now has no reviews yet.
[06:12 - 06:37] And on the control panel, we can set a number value, and once we keep changing, you'll see on the left side that it responds to those, which is pretty cool. However, having this control is not really that interesting because we have to set the number directly, and we don't know the limits, but thankfully storebook has the concept of R types, which allows us to customize the type and behavior of each arc in the controls panel.
[06:38 - 07:12] So let's go back to the stories from the review components and add our types there. We can add our types either on the level of the story or the component in the meta, and because we want this applied to every story, we're going to add to the meta. So we type here are types, and then we define that for the rating property. We want the control to be of type range, so that we have a slider that has a minimum value of zero and maximum value of five, and a step of, let's say, 0.1.
[07:13 - 08:05] So once we save this and go back to our component, you will see that the rating has changed its type, and we have this slider, which is very interesting now because first we get up to the maximum of the review and the minimum, and we can quickly see how the component behaves just by playing with that. Now you might be asking, if I can reproduce every use case of this component by using the control panel, do I even need to create more stories? Well, even though we technically can see every use case by playing with the controls, it's important to document the use cases as stories for a component, so that anyone accessing our storebook can easily identify them without having to figure out by the control panel. So every story should be reflecting a very important use case of a component. If we go back to the review component stories, we already have the default. So let's add a couple more.
[08:06 - 08:42] I'm just going to be cloning this line and calling this excellent. The excellent story should have a rating. So we define the arcs for the rating of five. We do the same for a couple more, and essentially the first is excellent. The second is very good, and that should be of maybe 4.3. The next one should be adequate, which should be around 2.5, and then very poor of one. We don't need this.
[08:43 - 09:19] So once we save this, we make sure that we have one story per scenario that reflects how the rating component should look like. And if we look at this, the default one kind of looks weird because it's actually using the values we played with in the controls panel, but we can just click here to reset it. So this is the correct way to look like. Then we have excellent, very good, adequate, and very poor. And this is great because anyone that checks your storebook will be able to see exactly all of the scenarios that the component should take care of, which is very important in terms of documentation for anyone.
[09:20 - 09:43] And this should be it for the review component. We have successfully created two components and worked on them in isolation by using storebook stories without having to even run the application. But the thing is, we still are not done because as you can see, the styles are kind of wonky and the fonts are missing. So normally applications, they have global styles that are applied across the entire application, and in your drop, it's no different.
[09:44 - 09:56] However, storebook does not know about these styles, which is why we don't see the correct font in those components. So in the next lesson, we will learn how to apply global styles to storebook by adding decorators to provide them in support. I'll see you then.