React Native dotenv / Babel Setup
This lesson preview is part of the Scaling Web App Configuration with Environment Variables course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Scaling Web App Configuration with Environment Variables, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
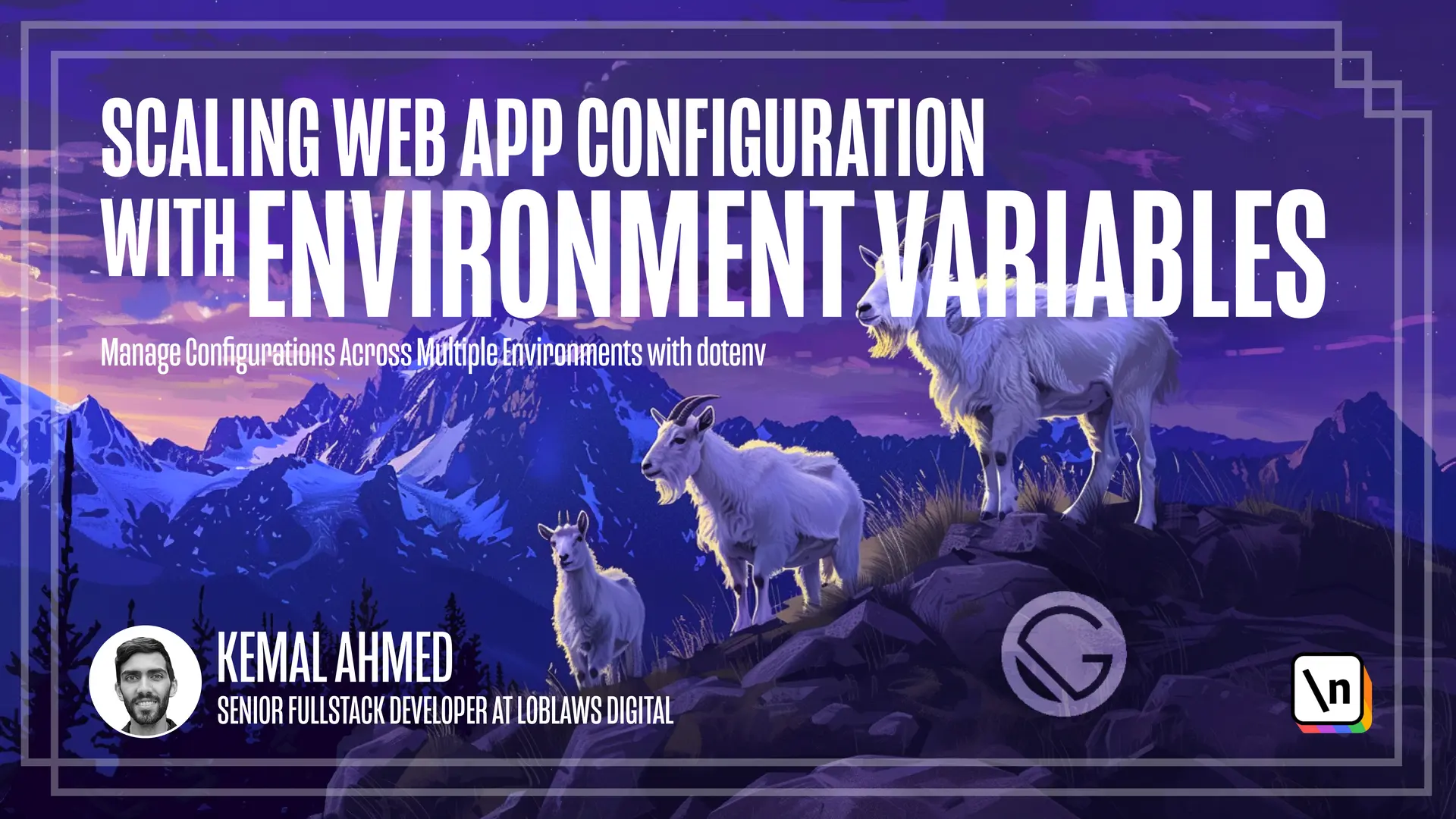
[00:00 - 00:06] Right, testing, one, two, three. Testing, one, two, three.
[00:07 - 00:13] Welcome to lesson 4.2. This is our last lesson together and it really packs a punch.
[00:14 - 00:23] This time I'm filming during the day, so sorry about the light mode. This demo will be setting up React Native dot end without using Expo.
[00:24 - 00:35] We'll start by creating a brand new project from scratch. Npx react native init my app.
[00:36 - 00:49] Make sure that these two are capitalized and let's build it. Now that that's built, you'll notice this has created a TypeScript project.
[00:50 - 01:02] We're going to make an Android build for this project. In order to do that, we have to first install the right versions of Android, Java, and a few other things that will make it run.
[01:03 - 01:14] I've included a link to the React Native documentation that goes through how to do that in the description. If you're following along using Expo, most of the steps are the same.
[01:15 - 01:23] If you haven't already set up your computer with React Native, pause this video and go get those set up. Done?
[01:24 - 01:29] Great. Now that we've set that up, let's build the Android app.
[01:30 - 01:53] To do that, all you have to do is first move into the directory of your project , my app, and then run npm run Android and it will run the script that has been defined over there in the package JSON. I see a lot will come up and just wait for both of them to continue loading before we continue.
[01:54 - 02:04] If an error comes up like this, make sure that you've set up your Android emulator properly or just rerun it again. There we go.
[02:05 - 02:14] Pressing the A button, ran the Android emulator, opened this QEMU and it ran the app. That's awesome.
[02:15 - 02:28] Now that it's working, let's install React Native dot end. npm install, it has to be installed as a dev dependency, so dash D, React Native dot end.
[02:29 - 02:50] Now if you try to install it as a regular dependency, it's going to start trying to add things like path and FS and things that are not really supposed to be added to your React Native project. But since it's a Babel library, we do need to add it as a plugin here.
[02:51 - 03:17] So plugins, module, add, actually don't forget there's two sets of brackets for plugins. So before we add that, we'll do that, react native dot env Next, let's create our dotenv file.
[03:18 - 03:33] Save that dot envy. And we'll say test var equals testing.
[03:34 - 03:43] And actually we'll call test message. So test message, hi.
[03:44 - 03:53] And then in our app dot TSX, I'm going to create another text object. Remember, this is React Native.
[03:54 - 04:11] So it's not regular HTML, and we'll do process dot env dot test message. And then we'll compile that and see what happens.
[04:12 - 04:24] So the first thing we'll see is that there is an error, it's not showing up. The reason why is that Babel does not look for changes in your dot end file.
[04:25 - 04:43] What I mean is Babel actually caches everything that you write, JavaScript, TypeScript, etc. And when you make changes in it, then when you recompile, it makes sure to recompile those parts and it caches the rest.
[04:44 - 04:55] Unfortunately, dot end files are cached. So in order to actually tell Babel to check for changes, you have to invalidate the cache.
[04:56 - 05:07] So let's go do that. First thing we have to do is let's close our current server instances that are running.
[05:08 - 05:19] And let's find our package JSON and where we have our start and Android, well, we'll do through start. So I'll make a copy of start.
[05:20 - 05:31] And then I'll actually just add a short word to say reset. And at the end, I'll add a flag reset cache.
[05:32 - 05:47] Now let's run that NPM run start reset. And it should bring up the server and we can rerun the app.
[05:48 - 05:52] You'll notice it says cannot connect to Metro. That's because we ran start, not Android.
[05:53 - 06:00] So all we have to do is press A and it runs on Android and that should reload shortly. And it shows up.
[06:01 - 06:06] It says hi. I did add a style attribute right there just to make it more visible.
[06:07 - 06:18] And I did make a few mistakes, but I'll show you how to do with that. So over here, if you see any mistake, just make sure to auto fix all your problems as much as possible.
[06:19 - 06:29] And sometimes you'll get some strange ones. All you have to do control shift P like open the command panel and restart your ESLint server.
[06:30 - 06:50] Oftentimes that'll fix a lot of pesky issues that are stuck in cache. If for whatever reason that reset does not work for you or you're not able to do that, then you can also go into your Babel config and change it so that way you disable the cache.
[06:51 - 07:02] So the way to do that is turn this into a function module dot exports equals function. We'll say API as a parameter.
[07:03 - 07:13] And in there we do return an object. And in that object will be this configuration.
[07:14 - 07:24] And before we return the new line and we'll do API dot cache balls. So that disables the cache.
[07:25 - 07:37] Jack native dot also supports some type of flow. So we can make a development version of this since we're in development mode, dev dot development sorry.
[07:38 - 07:59] And then we'll just do internal message. And just so that way we can demonstrate something new, there's a second way which we can access variables other than process dot end in case you're not able to access process dot end.
[08:00 - 08:08] And that is through a special type of import. So we can import our variables.
[08:09 - 08:26] And we say from at env. So basically what will happen is Babel will convert this into the object and it will extract the value so that way we can use it where we want to.
[08:27 - 08:42] And at the bottom, I'm just going to add that to our text object that we had before. Process dot end, test message, whoops.
[08:43 - 08:48] And then we'll do that. And then all we do is internal message.
[08:49 - 08:56] Okay, we're definitely going to have to clear the cache there. Well, sorry.
[08:57 - 09:07] Plus this line just to separate the values. And yeah, let's try that.
[09:08 - 09:13] Okay, so that didn't work, but that's okay. I'm going to tell you why.
[09:14 - 09:27] The message says cannot find module at end or its corresponding type declarations. So since we're using TypeScript, we actually need to define this so that way the linting knows what we're looking for.
[09:28 - 09:36] And it's actually not too bad. First, I'll create a types folder.
[09:37 - 09:45] Types. And in there, we'll create a new file, which is where we'll put our type def.
[09:46 - 09:54] In there. nb dot d dot TS, you can call it whatever you want to just find.
[09:55 - 10:09] Env is a good name for that. And then what we'll do is we'll say declare module.
[10:10 - 10:20] Env. We'll say export const test message string.
[10:21 - 10:37] And then the second one export cuts internal message string. Next, let's restart the app.
[10:38 - 10:42] We'll stop the server. Run start.
[10:43 - 10:54] Since we have the API to cache false going, we don't need to use the reset version. You can just use the regular start.
[10:55 - 11:04] And it should actually reset the cache to include both of these two variables. Well, it does say hi twice.
[11:05 - 11:12] So maybe it'll change that hello so that way we can see the difference. And it works.
[11:13 - 11:23] So we went over how to create a development config.n dot development. But I didn't show you how to actually set your end type.
[11:24 - 11:36] When using the start commands, you can set your end type using app end. Set app end equals.
[11:37 - 11:48] And you can use it just like we have done in the past with node end, except the React native tool chain overrides this value. So app end is the way to work around it.
[11:49 - 12:02] You can even use non-standard end names like staging in this start command. You do need to use reset cache every time you use app end.
[12:03 - 12:13] But for this demo, we're going to use production. So let's create a dot end dot production file.
[12:14 - 12:24] And I'm going to change these both to say something that actually makes sense. Well, this one says production.
[12:25 - 12:35] So we'll say production. And we need to rerun that.
[12:36 - 12:50] So let's close the server. End npm run start reset.
[12:51 - 13:03] And it works. One thing I will note, it's a lot harder if you're trying to set the dot end values for the run platform commands.
[13:04 - 13:14] Firstly, for Android, you have to make sure you're adding a clean task. There's another attribute that you need to add tasks clean.
[13:15 - 13:22] And I like to put install debug after that. There's actually two modes for platform.
[13:23 - 13:27] There's debug and release. Their default is debug.
[13:28 - 13:41] So I'm also going to copy that and I'm going to make a production one or just prod now because it's easier. And then we'll say mode release.
[13:42 - 14:07] And then we have to change this one to release. Note that for production, when this is basically the equivalent of app end equals production, except the problem is that app end equals production and app end equals development don't really work for the platform scripts.
[14:08 - 14:21] So it's better to just use what it gives you. So basically what I mean by that is whenever you run release, the default node end is going to be production.
[14:22 - 14:41] And when you do debug, the default will be development. So it's better if you do want to do, if you're wondering how app end plays a role, you're going to have it plays a role for custom environments.
[14:42 - 15:05] So if I wanted a staging version, I could do app end equals staging and react native run Android. But before I show you, I will tell you this is a very nuanced, this is a very big problem.
[15:06 - 15:30] So unfortunately, every time that you use this, you have to clean the cache. So basically you can only use it once at a time after every time you run one of these Android commands, make sure that you run the start reset command.
[15:31 - 15:34] This way you'll avoid caching your end type. Let's do that first.
[15:35 - 15:53] Well, actually, sorry, we don't actually need to do that because we just ran that a second ago. But just make sure to get into the habit of doing your npm run start reset after every time you run one of these Android scripts.
[15:54 - 15:57] Okay. Well, let's try it out.
[15:58 - 16:00] So npm run Android. Oops.
[16:01 - 16:05] I forgot to actually make the staging file. Let's do that.
[16:06 - 16:23] Rename staging and Android staging. And we'll see what happens.
[16:24 - 16:33] You may be tempted to press a button in the thing that comes up. Sorry, it didn't do set.
[16:34 - 16:44] You may be tempted to click through the window that pops up when this happens. Basically don't touch it.
[16:45 - 16:50] Just let it go through to the end. I'll show you what I mean.
[16:51 - 17:07] This window, this node window that comes up, it'll say what commands do you want to do, Android reset, et cetera. Don't click anything on there, just wait until everything compiles and then see what happens.
[17:08 - 17:11] And there we go. It says staging.
[17:12 - 17:23] So from this point, you will be able to publish it in Android Studio and it should contain the values that you've built into it. And you should be good from there.
[17:24 - 17:25] ( cause of "the sun is the sun" )