How to Generate a JavaScript AST With Babel Plugins
Exploring tools to convert (or parse) JavaScript into a real AST
This lesson preview is part of the Practical Abstract Syntax Trees course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Practical Abstract Syntax Trees, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
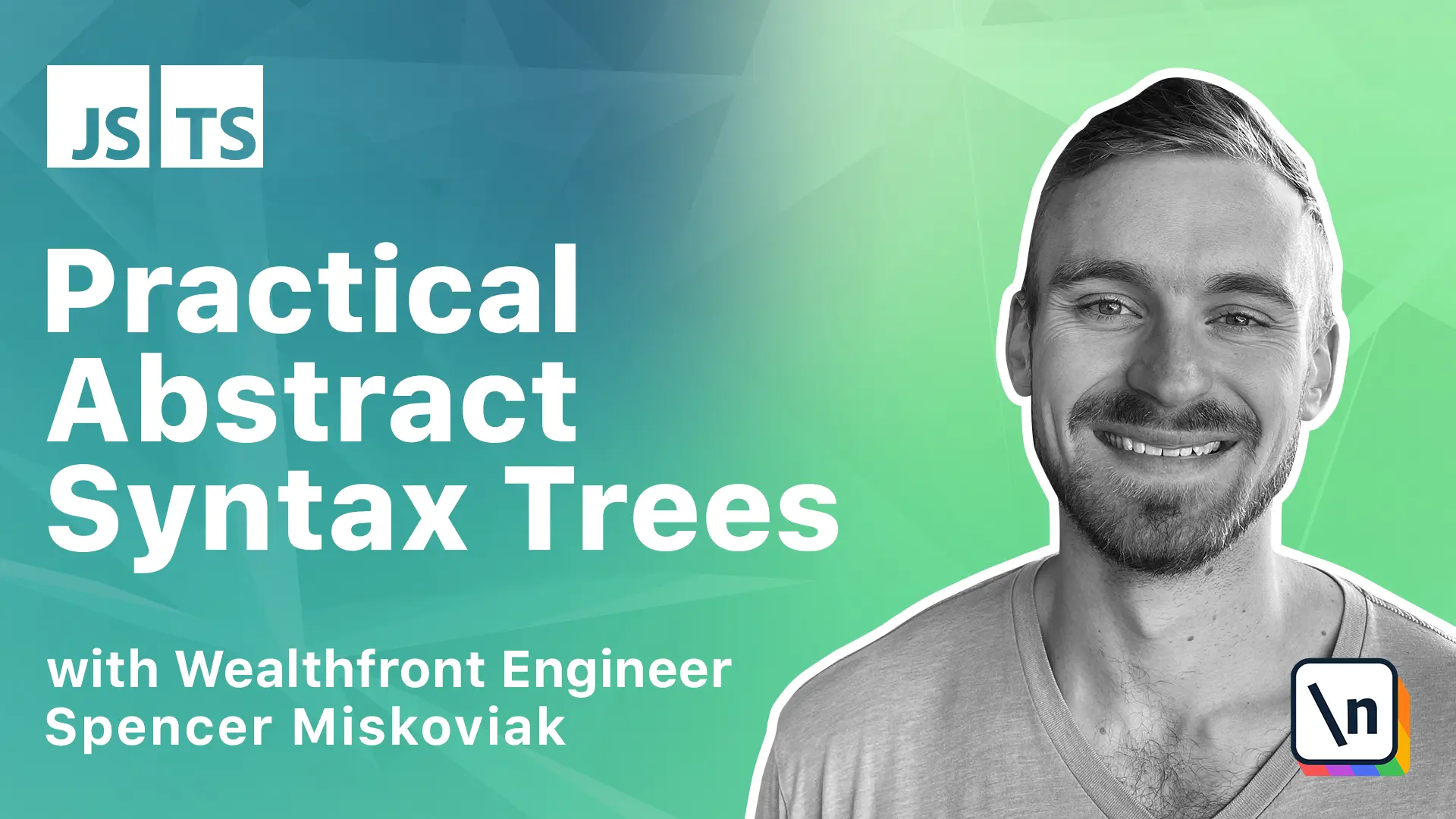
[00:00 - 00:13] Now that we have an understanding of what abstract syntax trees are, we can start generating ASTs from source code and programmatically traverse through the different nodes in the tree. When previously looking at AST Explorer, the parser option was set to Babel parser.
[00:14 - 00:30] This is a package that's available on MPN, which means it can be installed and used to parse any source code string into an AST. At a high level, Babel is a JavaScript compiler commonly used to transpile modern JavaScripts syntax to compatible but older JavaScript syntax.
[00:31 - 00:40] This allows early adoption of new syntax and features before there is sufficient browser support. It has a robust plugin system that can be used to add support for many different syntaxes.
[00:41 - 00:49] For example, the optional training plugin. This adds support for optional training syntax, which is new syntax that isn't widely supported by browsers yet.
[00:50 - 01:02] This will transpile this new syntax in two equivalent but older syntax. For the React JSX plugin, which adds support for JSX, which is XML-like syntax and not intended to be implemented by browsers.
[01:03 - 01:13] This plugin transpiles the syntax to an equivalent and supported syntax, specifically functions and objects, before it can be ran in the browser. And the final example is the TypeScript plugin.
[01:14 - 01:23] It adds support for TypeScript syntax, specifically static types. This plugin strips the types and does other transformations to transpile to valid JavaScript.
[01:24 - 01:40] These are only a few of the many plugins that Babel supports. Babel performs these transpilations by converting the source code into an AST and manipulates the nodes and converts the manipulated AST back into source code.
[01:41 - 01:49] These plugins can extend and modify the parsing and generating of the AST. This means it's also possible to write custom plugins.
[01:50 - 02:01] The Babel core package wraps up most of this functionality in a single package with minimal configuration. However, Babel also exposes the different internal operations and functionality in a series of packages.
[02:02 - 02:11] We'll be using several of these packages in this lesson and throughout the rest of the course. The Babel parser package parses a source code string into an AST.
[02:12 - 02:20] The Babel traverse package allows us to traverse different types of nodes within an AST. The Babel generator package converts an AST back into a source code string.
[02:21 - 02:34] The Babel types package creates new nodes to add or replace existing nodes in an AST. All of these lower level packages can be used to create flexible custom scripts to parse, generate, and manipulate ASTs.
[02:35 - 02:43] To get started, we'll focus on the Babel parser package to parse the previous code snippet into an AST. First, create a new Babel parser demo directory.
[02:44 - 02:56] Then change into that new directory. Next, we'll initialize a new package.json file.
[02:57 - 03:06] And finally, we're going to install the Babel parser package. Now we can open this project in our editor.
[03:07 - 03:16] Next, create a parser.js file that we'll use to execute the parser on the source code. First, import the parse function from Babel parser.
[03:17 - 03:34] Then, we can create a variable with the same example code from previous lessons . Now we can use the parse function to parse the code into an AST.
[03:35 - 03:42] Now that we have the AST, let's print it. Switch back to your terminal and now we can run this with node.
[03:43 - 03:48] We can see that this printed the same AST. This AST is a single file node with several properties.
[03:49 - 03:59] One of the properties is program, which is a program node. This program node also has several properties, one of which is body.
[04:00 - 04:07] The body property is an array that can contain various types of nodes. This is where the nodes that represent the code we parsed will be.
[04:08 - 04:20] Note that ASTs are deeply nested so the output is truncated after a few levels. Let's switch back to our editor and we can update the script to log the first node in the body of the program to see the part of the tree that represents the example code.
[04:21 - 04:30] As we just saw, the AST had a program property, which itself had a body property, which is an array of nodes. And we want to specifically look at the first node in that body array.
[04:31 - 04:41] Now we can switch back to our terminal and run this again. This time, the parser script only printed the first node in the program body, which is an expression statement.
[04:42 - 04:55] This node has an expression property, which itself is a binary expression. A binary expression node has three important properties, left, operator, and right.
[04:56 - 05:04] In this case, the operator is the addition sign. The left property is a numeric literal node with the value of 2.
[05:05 - 05:15] However, the right property itself is another binary expression node. This second binary expression operator is a multiplication sign.
[05:16 - 05:26] In both its left and right properties, each point to a numeric literal node representing four and ten. Compare this output with what you saw in AST Explorer.
[05:27 - 05:44] AST Explorer and this script are both using the Babel parser package, so the output should be identical, assuming all of the configuration is the same. Now that we know how this part of the tree looks, let's update the script to print only the first binary expressions left numeric literal value, which is 2.
[05:45 - 05:55] The script is already printing only the expression statement. As seen earlier, the expression property is a binary expression which has a left property that is a numeric literal node.
[05:56 - 06:05] The numeric literal node then has a value property with a value of 2. Now we can switch back to our terminal and run the script again.
[06:06 - 06:20] This time, only the value 2 is printed. Instead of logging only the leftmost value, take a few moments to try to update the parser script to log all of the numeric values in the expression 2, 4, 10.
[06:21 - 06:28] For now, assume the tree never changes. In the next lesson, we'll explore how to do this in a programmatic way to handle any arbitrary tree.