Adding a domain library for RoomList, RoomBackground and RoomTimelineList that I missed in previous lessons
Add a domain library for the components
This lesson preview is part of the Next-Level Angular Apps with NX course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Next-Level Angular Apps with NX, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
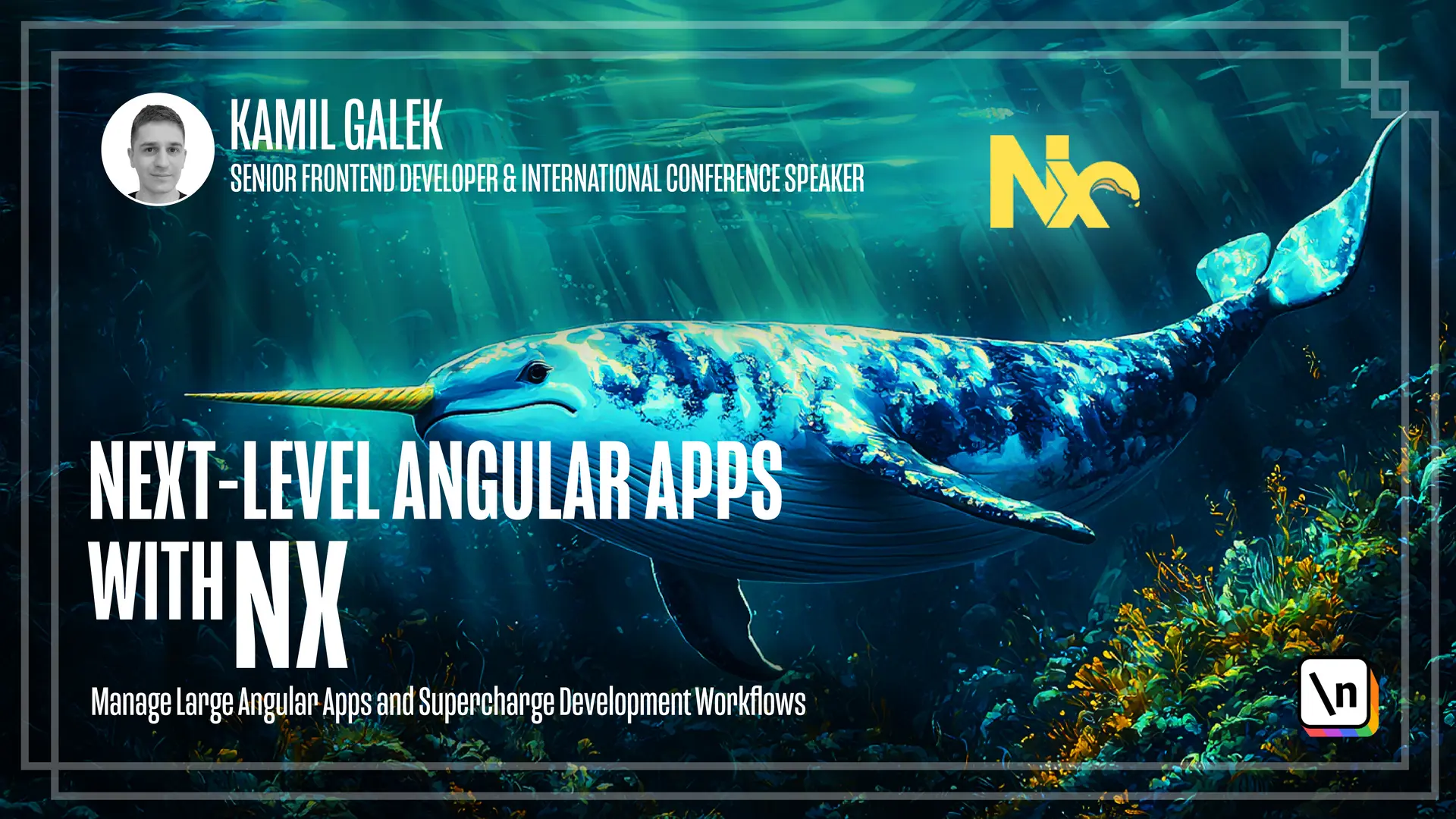
[00:00 - 00:07] To compose our applications, we need three more components. First one is grid of rooms and each room is presented using card component.
[00:08 - 00:13] This component will be used in both fronted applications. The next one is room background component.
[00:14 - 00:20] At Repound, our application will contain a predefined list of backgrounds to choose from. We need the component to handle that.
[00:21 - 00:29] And the last component is room timeline list component, which will present all meetings for selected room. So let's start with room background component.
[00:30 - 00:37] First of all, we need 10 nice photos. I'm using r/images, but if you have your own favorite pictures, just use it.
[00:38 - 00:59] The plan for this component is to separate logic from the visual part of components, so we will create room background image series that will provide an order list of available images. The component input will receive an index of the image to display, and then it will use room background image service to get the URL for selected image.
[01:00 - 01:11] We're using indexes instead of URLs or names, because it will allow us to change photo URLs in the future. So room background image service is exposing three methods.
[01:12 - 01:27] Get all, get URL by index that gets index as argument, and of course get URL by room, which returns URL for given room. Before we jump straight to the code, we need to speak about contracts.
[01:28 - 01:35] So method get URL by room is using room as parameter. And of course, it's not the DTO that we extracted in previous lessons.
[01:36 - 01:53] You want to avoid using the same objects that you get from HTTP later in the application. Because if you do this, it means that if the DTO will change, for example, someone will remove a field or add a field, you need to go through the whole application and fix all the usages in that object.
[01:54 - 02:07] The idea here is to map DTO to some other object that will not change. So even if the HTTP is changing and you have to fix your code, you will need to fix it only in one place.
[02:08 - 02:22] And by the way, you probably should consider mapping this object more than once . Basically, every time when you need to use something, you should create a separate object that sets the contract for the whole library or whole part of your application.
[02:23 - 02:30] And I would recommend keeping at least three types of objects on the front-end side. DTOs as a contract between front-end and back-end.
[02:31 - 02:41] UI read-only objects. So you use these objects only to display and immutable objects that you use for data processing.
[02:42 - 03:05] So for example, you receive DTOs from HTTP, then you map it to domain objects, and you can perform a lot of operations on that, then you map it to UI read- only objects to display it. I have put in the description to this video all necessary details that describe these three kinds of objects. So now we are ready to create room background image service.
[03:06 - 03:12] Give me a second and I will show you the code. On the top of the file, I have an interface called room.
[03:13 - 03:24] It's my contract, so if you are going to use this service, you need to fill that contract. You need to provide me a room object that expose getEMG method that is returning string.
[03:25 - 03:36] Next, I have an array of images that I'm going to use and three methods. So I have get all, which basically goes through images and returns an index of each image.
[03:37 - 03:45] Then I have getURL by index, so you're providing me an index. I'm checking if I have an image for that index, and I'm returning the URL.
[03:46 - 03:56] If not, I'm throwing an error, and the last one, getURL by room. So I'm using that room interface to get image index a string.
[03:57 - 04:06] I'm converting it to the number, and then I'm returning the URL. So nothing really complicated, very simple service.
[04:07 - 04:21] And with that construction, I'm able to change these URLs later. If necessary, for example, we have new images that we are going to use, or something, I'm storing only indexes of these images.
[04:22 - 04:29] So the only constraint for me is to provide 10 images. And of course, I need to expose room service from index.ts, so let's do this.
[04:30 - 04:40] So room background image service.ts. And we are ready to create a storybook story.
[04:41 - 04:55] My storybook component is quite simple, so I'm injecting that room background service, and I'm using getURL here to get list of all available images. And then I'm iterating through it to display all of them, using shared room background.
[04:56 - 05:09] And let's switch to the browser and check how it looks like. So as you can see, nothing really impressive at the moment, because we have only room background works, tags here, multiple times.
[05:10 - 05:16] So let's implement the component and check again. Okay, so shared room background component is quite simple.
[05:17 - 05:24] We have a div that is using style with background image to show the image. And then we have a required input called index.
[05:25 - 05:37] So we have to provide that index to display. I'm injecting room background service, and then I'm using it in getURL method to construct the URL string for that background image here.
[05:38 - 05:47] So let's switch back to the storybook and check how it looks like at the moment . And as you can see, we have a very nice overview of all images that we can use as backgrounds for our rooms.
[05:48 - 06:06] And this component is a bit more complicated. It's still simple, but the main idea here, why I'm not calling it as a basic component, is that this component keeps an information, the main knowledge about available images, how to display them, how to map them into indexes, and so on.
[06:07 - 06:09] That's why it's more complicated. That's why it's separated.
[06:10 - 06:16] I don't want to repeat this code, and I'm going to use it in both applications. So I decided that I want this component.
[06:17 - 06:22] The next component we are going to implement is a room list component. Okay, so I have the code.
[06:23 - 06:26] Let's go through it and check what is inside. So let's start with storybook stories.
[06:27 - 06:34] So I have a room list story that is very simple again. And I have my storybook room list component.
[06:35 - 06:40] So this is the component that is configured to display whatever I want in story book. And I have two blocks.
[06:41 - 06:51] So first block is playing shared room list component without add button. And I have rooms because it's required input and one output assigned.
[06:52 - 07:01] In my class, I have a hard-coded list of rooms. I have some mocked methods like on click that will display only another and so on.
[07:02 - 07:15] And of course, I have a get room method to create that mocked room list. So the second block is displaying the same list, but with new room button.
[07:16 - 07:28] In the component file, first of all, I have a new contract called again room. So I have a room that should expose get ID, get image and get name.
[07:29 - 07:35] The template is not very complicated. So I'm using shared card components to display it.
[07:36 - 07:48] So first shared card component is repeated using ng4 for all the rooms that are provided using input. Then I have an image that is passed through input.
[07:49 - 08:00] And then of course, I have a click listener that emits a new event on the room selected output. The second shared card is displayed only when the new room button is visible.
[08:01 - 08:04] So you have ngif here. I have a class for that.
[08:05 - 08:13] And of course, I need to assign output. So I have new room clicked output and I emit a new value and someone is clicking on that card.
[08:14 - 08:24] Inside of that component, there is nothing really complicated. So we have two inputs to outputs and room background service because we need to call get URL by room.
[08:25 - 08:29] Let's check how it looks in the storybook. So we have a new component on the list.
[08:30 - 08:33] Let's check how it looks like. And as you can see, it's a simple grid.
[08:34 - 08:42] We can click on that card components and we should get an alert. And the second option here is a list with add button.
[08:43 - 08:53] So we can click on add button and then we can go to the add screen. This component will be used in both application room front end application and admin front end application.
[08:54 - 09:05] The first option without add button will be used in the room front end application because we want to display all available rooms. And you can select one that you're interested.
[09:06 - 09:11] And the second option with add button will be used in admin application. So we can add a new rooms.
[09:12 - 09:20] And the last component for this lesson is room timeline list component. It's a list of all meetings in the selected room.
[09:21 - 09:28] I forget to add it in previous lessons where we created all these libraries in advance. So let's add it now to generate that component.
[09:29 - 09:34] I'm going to a similar command as previously. So yarn and next generate and extangulate library.
[09:35 - 09:47] And the path is leaps, shirt, components, room timeline list with prefix, build double, publishable and import path. And basically that's it.
[09:48 - 10:02] As you can see, I have the implementation and shirt room timeline list component is the most complicated component that we are going to create in this lesson. So first of all, as always, we have our contract here.
[10:03 - 10:14] So we have a meeting that has get name, get date from, get date to. Then we have a template, but maybe before we go to the template, we will go to the implementation of the class.
[10:15 - 10:22] So we have only one input called meetings and it's required. It's a right of meeting and meeting is our contract.
[10:23 - 10:48] Then we have a filter meetings method that gets the today date and filters out all the past events that are not for this day. We have three more methods is passed that should return true if the meeting was in the past and false if it's not is currently on for meetings that are currently running in the room.
[10:49 - 10:55] And of course, get formatted date because we want to show formatted dates. Let's go to the template.
[10:56 - 11:03] And as you can see, we have shirt list. So our basic component with shirt list item with ng4 here.
[11:04 - 11:10] So we are displaying all the meetings that are a result of filter meetings method. So only meetings for today.
[11:11 - 11:21] I'm going to add a class called pass when his pass is returning truth. So when the meeting has ended, I'm going to show that it was in the past.
[11:22 - 11:31] So it's going to be grayed out inside of list item. I have to spans first one is for meeting name and I will add class life if the meeting is currently on.
[11:32 - 11:38] And of course, I need to display a date from and date to. And that's it.
[11:39 - 11:45] And I've created a storybook story for this component. So let's go to storybook room timeline list component.ts.
[11:46 - 11:51] It's a very simple component. So we have our template that is shared room timeline list.
[11:52 - 11:58] We have some meetings that I decided to mock using get meeting method. It's nothing really complicated.
[11:59 - 12:06] So basically what I'm doing here, I'm generating a name, a date from and date to using offset. So I will have some past events.
[12:07 - 12:14] I will have some future events. Everything is marked like in unit test because I want to show all the options for this component.
[12:15 - 12:25] So let's check how it looks like in the storybook. And as you can see, we have room timeline list here component.
[12:26 - 12:30] And it's a list. All the past events are grayed out.
[12:31 - 12:40] The current event is red and future events are with no additional indicator. So to sum up, in this lesson, we created more components.
[12:41 - 12:55] What is important to notice is that these components were composed using components from a previous lesson. So for example, room timeline list component was using shared list, room list component was using cards and so on.
[12:56 - 12:59] What is important? I did everything using Starbucks.
[13:00 - 13:06] So I don't have to care about logic of my application. I'm preparing the ground for further work.
[13:07 - 13:15] And with this approach, I'm sure that my components are reusable and not strictly connected to the business logic of applications.