How to Use GraphQL to Add Comment Features to a Frontend App
This lesson will show how to extend the hook to handle adding new comment logic. It will cover adding a new GraphQL mutation and using it from the frontend application.
This lesson preview is part of the The newline Guide to Full Stack Comments with Hasura and React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Full Stack Comments with Hasura and React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
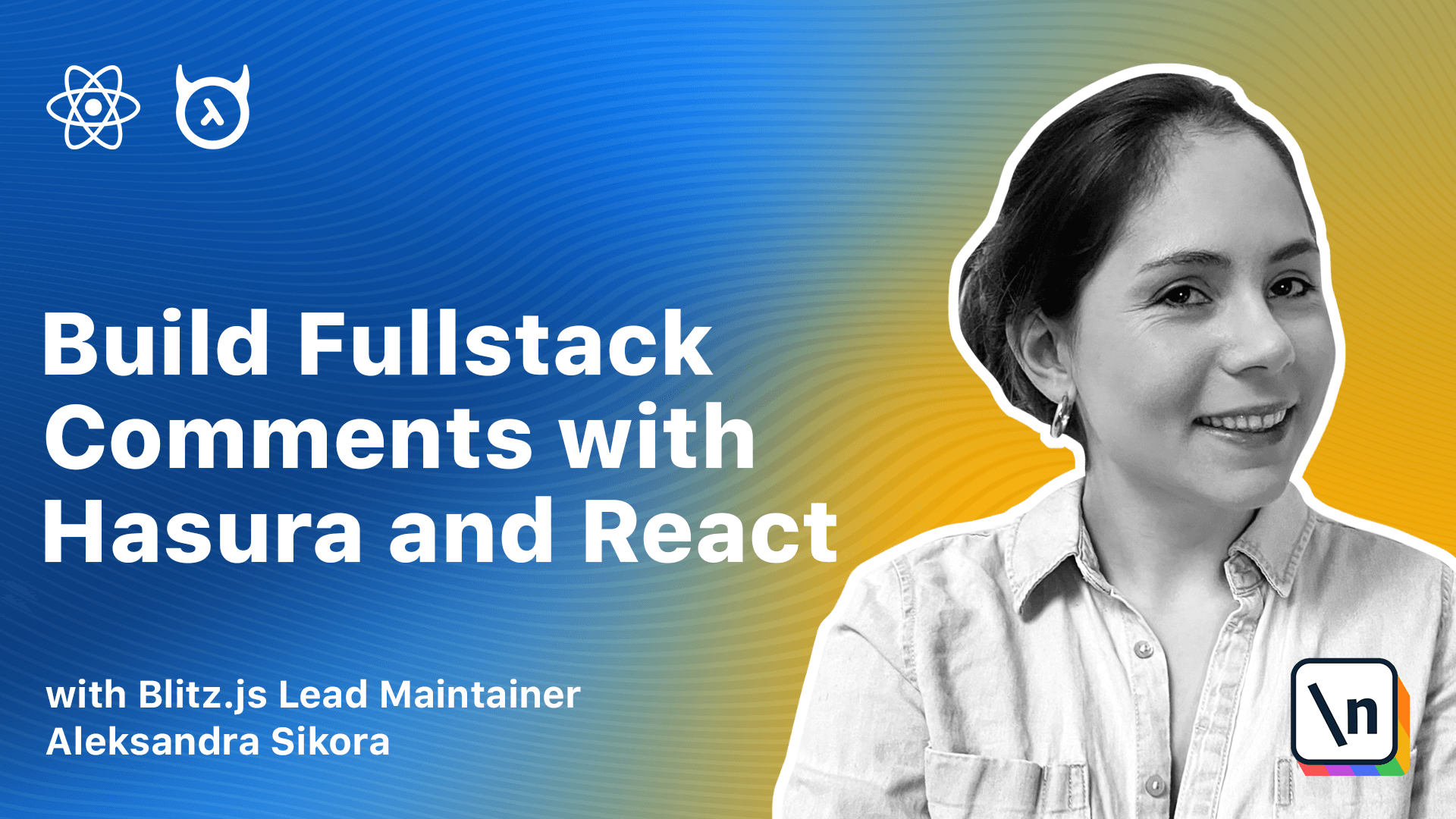
[00:00 - 00:08] In the two previous lessons, we implemented one part of the hook and we covered the UI. Now it's time to cover adding new comments.
[00:09 - 00:16] We'll write a graph computation for inserting a new entry to the database. And then we'll call it from the frontend.
[00:17 - 00:29] Firstly, let's go back to the Hasura console to create and test a graph computation. In the Explorer, we can see insert comment1 and insert comment mutation.
[00:30 - 00:37] The latter allows us to add multiple comments, but we'll only add one at the time. For that reason, we'll go with insert comments1.
[00:38 - 00:49] In the mutation, we're going to pass topic, author, and content as arguments. We'll store it as a constant in our code and then implement the add comment function inside our hook.
[00:50 - 00:56] It's going to be pretty similar to our fetch comments function. We also need to send a post request and handle the response.
[00:57 - 01:01] Let's start with the function signature. Our graph computation takes three arguments.
[01:02 - 01:09] Topic, author, and content. We already know the topic inside of the hook as it was provided as the hook parameter.
[01:10 - 01:15] Our function needs to take two remaining pieces of information. What's the type of the parameters?
[01:16 - 01:29] Instead of writing a new one, we can use a peak utility type. Now that we have the skeleton of the function, we can add the Hasura API call.
[01:30 - 01:41] We'll use a native fetch function, which arguments will look very similar to fetching the comments. We'll provide an URL to our Hasura instance and specify the configuration.
[01:42 - 01:49] Again, it will be a post request with the same headers as before. We're going to pass the X hasura all and content.
[01:50 - 01:58] In the request body, we'll provide created graph computation and variables. Topic, content, and author.
[01:59 - 02:06] Now we need to add response handling. In case of an error, we'll set the error message in the same way as we did in lesson 2.
[02:07 - 02:15] However, what should happen in the case of a successful call? We know that it means that a new comment was added and we need to display it along with the rest of the comments.
[02:16 - 02:22] There are two ways to do so. After a successful mutation, we can fetch all the comments again.
[02:23 - 02:38] The second option is that we can assume that the request was successful and since we have all the information about the comment, we can push it to the comment state variable. If the request was indeed successful, we don't do anything.
[02:39 - 02:45] Otherwise, we need to remove it from the list. This approach is called an optimistic update.
[02:46 - 02:52] Right now, we'll go the first option but we'll cover the second one in the following lesson. We just finished the hook implementation.
[02:53 - 03:05] Let's go back to the post component and try out the add comment function. We need to extract add comment from the hook and then we'll replace the console lock in on stop_mit with the add comment function.
[03:06 - 03:15] Now let's go back to the browser and see how it works. I will add a test comment and here it goes.
[03:16 - 03:24] Awesome! So we just implemented the logic to add new comments and tested it out in our sample block application.
[03:25 - 03:32] That means we have completed the basic commenting system. In the next module, we'll improve it by adding optimistic updates and pag ination.
[03:33 - 03:40] We'll check out a few other exciting ideas as well. In this module, we have one thing left to do.
[03:41 - 03:48] Is testing the hook? We'll see how to test react hooks and components and we'll write a few unit tests.
[03:49 - 03:50] See you in a bit!