Component state and rendering data from it
How to render data from component state. Learn about Vue.js directives, and reactive rendering.
This lesson preview is part of the Interactive Vue.js Resume Builder course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Interactive Vue.js Resume Builder, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
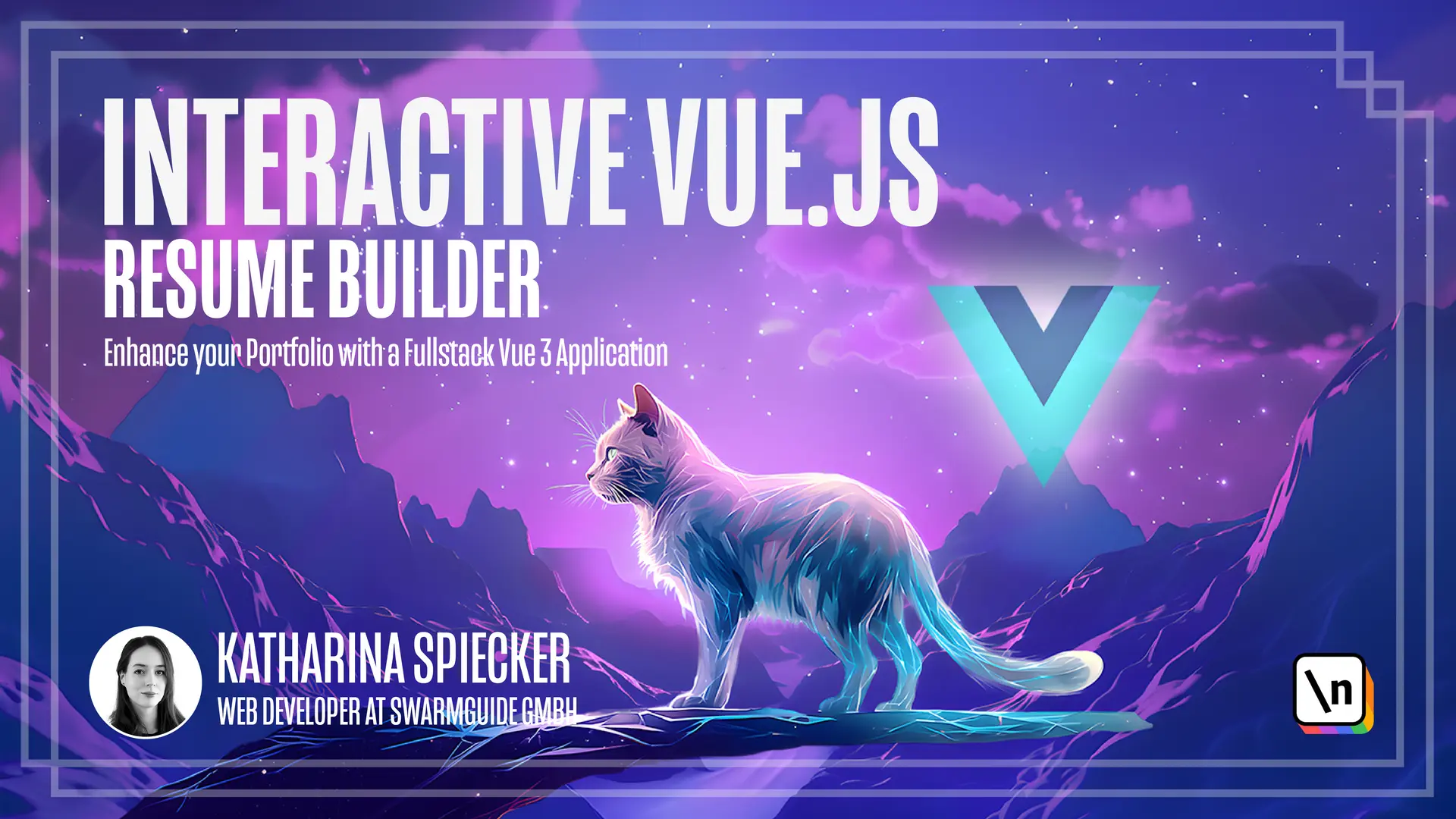
[00:00 - 00:31] In the previous lesson, we have created the template structure for the resume. In view, you don't write data that might change hard-coded into the template. Instead, you capture the initial value in the component's reactive state and display this value to the template. Reactive because view reacts to any changes in this data by re-render ing the part of the component where this data is displayed. This way, you are dynamically displaying data.
[00:32 - 01:03] If the state changes, the template will automatically update. In this lesson, we will get to know the data method that holds the component state and how one renders data in view. We will use this knowledge to eliminate the template's placeholders and move all the data to the component's state. So far, our app component only contains a template and some styling. As a first step, we need to add a script section containing the component state .
[01:04 - 02:05] Let's look up the structure in the documentation. The script section defines a view component using the default export syntax from ES6. You can include an object with various component options in the script section. Among these options, one crucial method is called data. The data method should be defined within this object and it returns an object itself. This in our object is where you capture and store the reactive data for your component. In other words, the data method returns an object containing the initial state of the component and view will automatically track any changes to this data for dynamic updates. Therefore, I will be referring to the reactive state of a component as the data object. Remember that it gets declared as a method that returns an object. Let's start by moving the name, title and intro text away from the template here.
[02:06 - 03:44] I will be using dummy data for this resume. You can use the same makeup different data or use your own data. Since this object is just a JavaScript object, it can contain any data type that JavaScript objects can contain. I point this out because you can add attributes containing any primitive data type as well as attributes containing objects and arrays. In order to render data in the template, we use the mustache syntax in view. The mustache syntax describes these two curly braces that would look like a mustache if turned 90 degrees. They are responsible for printing out the reactive data. You reference this data in the template simply by using the property names. View refers to this printing of dynamic data as text interpolation. We add the name and title. In the about me section, we add the intro text. Use reactivity system watches the value start and the data object and if anything changes, the associated template automatically updates. This reactivity of the DOM in regard to the data it contains is called data binding. It eliminates the need for manual DOM manipulation. The mustache syntax can also contain a JavaScript expression.
[03:45 - 04:05] A JavaScript expression can be anything that reserves to a value. As you can see, if I type a simple arithmetic expression here, it gets printed out as the view documentation states. View actually supports the full power of JavaScript expressions inside all data bindings.
[04:06 - 04:23] In addition to the text interpolation, view provides other forms of data binding. When you need to bind dynamic data to HTML attributes, you cannot use the mustache syntax. Instead, view offers the vbind directive for this purpose.
[04:24 - 04:45] With vbind, you can dynamically set HTML attributes based on the components data. For example, you can use vbind to set the source attribute of an image element or the href attribute of a link. To demonstrate this, we are going to add a picture to our resume.
[04:46 - 04:56] First, you must add a picture to the public folder of the project's directory. Next, you can reference the name of the image in the data object.
[04:57 - 05:15] The dynamic source attribute now references image URL. You can see the image being displayed, but it needs some styling.
[05:16 - 05:39] We made the source attribute dynamic by adding vbind in front of it. View provides a shortcut for the vbind directive. Instead of vbind, you can just write a colon.
[05:40 - 05:58] In many countries, adding a picture to the resume is common. We want to give users the option at least. Therefore, we added a placeholder image now, and let the user replace or remove the image later on.
[05:59 - 06:07] So far, we have moved two strings and the image pass and the data object. Next, I will move all headlines into an array.
[06:08 - 06:51] We render those headlines by accessing the index of the array. [silence] To represent the contact data, we will add a property of the type object that holds the property's phone, email, and address. To represent the data in the template, you just print out each property with the message syntax.
[06:52 - 07:13] Next, we move all the skills into an array. Let's also add some more skills to the array. In view, we can render iterative data with the v4 directive. You can think about it as a for loop.
[07:14 - 07:27] You add the directive to the element you want to repeat it with each loop. We create a list element since we want to render the skills data as list elements.
[07:28 - 07:51] The list element gets the view directive v4, skill, and skills. That way, we iterate over each item in the array. The v4 directive does not get added to the parent node, ul, for example, but to the exact node you want to repeat with each loop iteration.
[07:52 - 08:07] The current array item is then available within the element. That is why we can display the variable skill. So if we were to write v4, test, and skills, the test variable would be available within the element.
[08:08 - 08:39] What makes viewjs so effective is that once data changes, it only re-renders the part that is affected. In order to enable view to map the DOM elements with the correct item in the array, we have to pass a key attribute. You could use the index of the array item for this. View passes the index as a to the key second argument to the v4 directive if we change the syntax like this.
[08:40 - 08:56] Since the index is dependent on the current iteration, it is a dynamic value. with vbind. I am using the shortcut here.
[08:57 - 09:07] By adding the key attribute, we have enabled view to create a unique reference to each node. You can read more about that here.
[09:08 - 09:38] The second I change an entry and the data object, the DOM element where the entry is rendered updates. We can also inspect the data contained in the component with the view extension in the browser. I am using google chrome here with the vuejs def tool extension installed. If you open the def tools, you will find a new entry called view.
[09:39 - 09:53] This tool shows you which components are present on the page and the data that contain. So far, we only have one component. You could edit the properties and watch how the page re-renders.
[09:54 - 10:03] Note that you can only use this extension when the app is not in production mode. The app is currently running in development mode, so it works.
[10:04 - 10:32] We still need to move the experience and education sections into the data object. We will start with the experiences. Since there can be more than one experience , we need an area of objects. Each experience needs a title, a company name, a location, and a data stream. Each experience will be an object with these properties.
[10:33 - 10:43] Additionally, each experience gets the property description, which describes the experience further. Let's populate this experience array with three experience objects.
[10:44 - 11:11] Now let's run out the experiences from this array. We can use v4 here again. Each experience should be wrapped in a div. On that div, we need to add the v4 directive, so the code gets repeated for each item and the experience array. Inside this div, the item variable will be available and we can access the current experience through this.
[11:12 - 11:22] Let's add the title, the company name, the location, and the date. We need to iterate through this inner array to display the description items.
[11:23 - 11:41] Remember to add the key here, as we have learned previously. For this, we want to use a different variable, inner index, to avoid mixing up with the variable from the outer array. In between the list tags, we will print each description string.
[11:42 - 11:58] Looking at the app in the browser, we notice that there is currently no distance between the individual entries. We need to apply margin bottom to each education entry. For that, we add the class, inner section, to the wrapper div.
[11:59 - 12:53] To properly use the space and improve the resume layout, we want to align the company name, location, and date into the same row. We accomplish this by wrapping them in one parent div and adding our previously created Deflex class. Now, they are next to each other, but we want the date to be on their very right. We need to apply justify content space between to the parent element. Since we will be using this several times, I want to create the bootstrap like class for this and the main CSS file. The education part will be very similar to the experience part.
[12:54 - 13:23] An education object will need the following properties, title, university, location, date, and description. The description should be an array of strings, highlighting some achievements or aspects of this education. Now all that is left is to replace the placeholder content for education with the actual data from the education data property. We follow the same layout that we have used for the experiences part.
[13:24 - 13:39] The only difference is that instead of item company, we print item university. We wrap up this lesson about a components reactive state and rendering data from it.
[13:40 - 13:44] In the next lesson, we will learn about how to make the content editable.