Frontend
Setting up the frontend with Vite, React and Tailwind
This lesson preview is part of the Fullstack Typescript with TailwindCSS and tRPC Using Modern Features of PostgreSQL course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Typescript with TailwindCSS and tRPC Using Modern Features of PostgreSQL, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
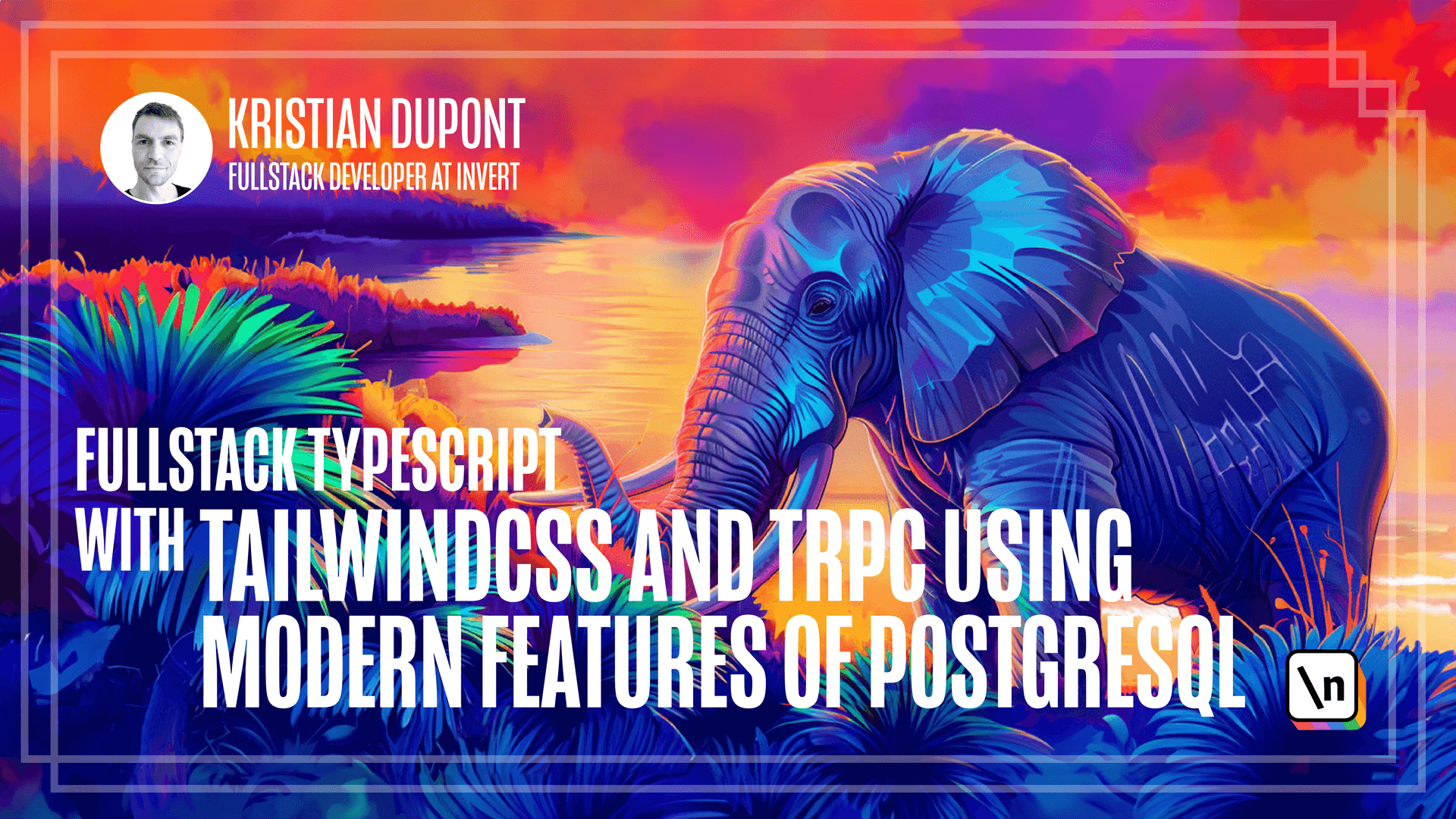
[00:00 - 00:12] Now, the final missing piece of our architecture is our frontend. And the reason why we haven't created any frontend folder or package JSON is that we're going to be using a bundler called VIT.
[00:13 - 00:21] And VIT has some templates that we can use to help us create the basic scaff olding of everything we need. So let's try and invoke that.
[00:22 - 00:39] If we go to our services folder, we can run npm create VIT at latest and we can call our project frontend. And it used to be that you had to specify a template here, but at this point, V IT just asks a series of questions.
[00:40 - 00:50] So we're going to tell it that we want the React template and we want it with TypeScript. We're going to be using the regular ESBilt bundler rather than SWC.
[00:51 - 00:58] I think it should probably be fine with either. And SWC might indeed be faster, but at this point, let's just go with the default one.
[00:59 - 01:10] So we will now have a frontend folder for us and we can run npm install in this . And you can see that VIT will have created a folder for us with quite a few files in it.
[01:11 - 01:22] So there's a whole bunch of stuff in here, including a package JSON that has quite a few dependencies. And one thing to note here is that it has set up a ES lint for us.
[01:23 - 01:33] And that is actually not really what we want because we already had that set up in the root and that's where we want to do our linting from. But it does add some nice plugins and rules, like the React hooks and so on.
[01:34 - 01:45] So we'll keep it just for now and that is something we can refine later on. One thing we do want to adopt a little bit though is that you can see that it has added a TS config file for us.
[01:46 - 01:57] In fact, it's added too. And the reason for this is that the TS config file itself is where we configure TypeScript for our code, like the code that will become the frontend.
[01:58 - 02:14] The TS config.node.json, which is in my opinion a little bit misleading, it should probably be called something like .vit.json. But that is the configuration for the VIT engine itself because that is a node project that runs in your terminal.
[02:15 - 02:19] And that needs to be using TypeScript as well. You can see here that it has a configuration file that is TypeScript.
[02:20 - 02:32] So it's running TypeScript as it runs and that is configured in this file here. We're not going to care too much about that one right now, but we do want this configuration file to be updated a little bit.
[02:33 - 02:40] We can keep these settings as they are for now. So you can see it includes source in SRC the way we've done in our other projects and that's fine.
[02:41 - 02:56] And the only thing we want to add is that we wanted to extend our primary configuration. And the reason for this is that we want to make sure that the references to the schema package are kept in our frontend.
[02:57 - 03:04] And that's all we really care about at this point. We might refine some of these other settings as we go along, but for now that will all be fine.
[03:05 - 03:25] But let's reference this or extend this basic TS config file and see if everything works. So I'll say NPN run dev and beat spins up and it says this is server running now on localhost.
[03:26 - 03:40] Let's try and open that and look we have a little app running react and we can click on this button and account will increase. So everything seems to be working fine even with our specific TS configuration.
[03:41 - 03:50] Let's stop that. We're going to be using tailwind CSS for styling of our frontend.
[03:51 - 04:03] And if you're not familiar with tailwind CSS or if you haven't tried using it you might feel similar to how you might with prettier. It is definitely controversial and personally when I first saw it I didn't like it at all.
[04:04 - 04:16] But I found that after I've worked with it for a bit I absolutely would hate to not use it on a new project. It is very addictive and it's a very nice way to work but it is something that is somewhat controversial.
[04:17 - 04:30] So you might find that this isn't for you and then you shouldn't be using it in future projects but that is what we're going to be using in this course so now you'll have to bear with me a little bit. Let's install tailwind by adding it to our frontend.
[04:31 - 04:39] So we do that by adding some developer dependencies. Tailwind CSS, post CSS and auto prefixer.
[04:40 - 04:55] Post CSS, auto prefixer are dependencies of tailwind CSS so you just need to add those at the same time. And then tailwind has a little script for initializing it so we'll run that and PX tailwind CSS in it -p.
[04:56 - 05:08] The -p I believe creates the post CSS configuration file. So we run that and we now have a post CSS configuration file and a tailwind configuration file.
[05:09 - 05:18] Let's open the tailwind config file. So we want tailwind to look at all of the files that we create that might have CSS classes in them.
[05:19 - 05:38] So the way it works is that tailwind will provide a whole bunch of utility classes and you don't want all of those to be included in your CSS bundle. So what it does is that it scans all of your source code for any string that looks like one of these classes and if it finds it, it will include it in the bundle.
[05:39 - 05:55] And it's a confusing error situation to have that you are missing one of these classes because tailwind didn't discover it in your source code. So it's pretty important that you get all the source code included here that you might be adding CSS classes to.
[05:56 - 06:13] And we may not be adding anything to index.html, but let's just include it to be absolutely sure that we have everything. So one thing to note here is that VIT puts the index.html in the root folder of the project, not in the source folder.
[06:14 - 06:27] If you're not familiar with VIT, that is something that might trip you up, but just to keep in mind that it sits there. And then we're going to include all of our component files and all of those are in the source folder.
[06:28 - 06:37] And they're called either, let's just include JavaScript. So JS, JSX or TS or TSX files.
[06:38 - 06:59] And the reason why we're including JS and TS as well as the X files is that we might have some logic in just a plain type script file that concatenates some string and returns as CSS class from that. I don't know if we're going to have that, but just in case we do, we don't want Tailwind to miss it.
[07:00 - 07:09] That's why I'm adding JavaScript as well here. We're not actually going to be writing any JavaScript code, I don't think, but just in case we do create a JavaScript file, it's nice that it is automatically included.
[07:10 - 07:19] So now that we have that set up, Tailwind should find it. We will then change the index.css file.
[07:20 - 07:26] So this index.css file refers to all of that that we had on this page here. And we're not going to be using any of that.
[07:27 - 07:35] So let's just get rid of all of this and just add the Tailwind directives. And there are three of them and we'll just add those.
[07:36 - 07:50] Those are just, there are some more I think, but these are what the basic configuration is. These components and utilities.
[07:51 - 07:56] You can see I get little error or warnings here. That's just because VS code doesn't know about Tailwind.
[07:57 - 08:15] I'm not actually sure why that is, but it's a warning that can safely ignore because post CSS will pick this up. We will then, we can get rid of this stylesheet file and the main just renders an app.
[08:16 - 08:19] So that is fine and that opens index.css. So that is fine.
[08:20 - 08:33] The app file, we can just clear and we will just add, let me just copy this from the article, this very, very simple component. We can also get rid of, let's see, the assets folder.
[08:34 - 08:42] We don't need that and we also don't need this logo here in the public folder anymore. There.
[08:43 - 08:58] So now what we should have is just a basic div with an H1 that has text for Excel and text indigo 700. So the text for Excel will set the text size, the font size, and this one will set the color.
[08:59 - 09:06] And you can see I have little hints here. That is from a VS Code plugin called Tailwind CSS IntelliSense, I think.
[09:07 - 09:13] And if you're using VS Code, I highly recommend that you install that. It is tremendously helpful when you're using Tailwind CSS.
[09:14 - 09:22] So let's try and run our server again and see if we get a page that now reflects this. Hello world.
[09:23 - 09:25] That looks indigo to me. Perfect.